If you’re new to Python and want to learn the basics, practice with our free Python programming exercises for beginners. Our 40 problems cover Python numbers, strings, loops, functions, and other data structures, and they’re all designed to be easy to follow. So what are you waiting for? Start practicing today and build a strong foundation in Python.
Programming Exercises for Beginners in Python
Use our online Python IDE to run and test your Python scripts.
Python Basic Level-1 Exercises
Here are the first 5 Python exercises for beginners along with their descriptions and solutions. These are often given to interview candidates to assess their basic Python knowledge.
String Reversal
Description: Write a function that accepts a string as input and returns the reversed string. This exercise focuses on string manipulation using slicing in Python. You will learn how to reverse a string by leveraging Python’s indexing and slicing features.
Solution:
def reverse_string(s): return s[::-1] string = "Hello, World!" print("Reversed string:", reverse_string(string))
Also Try: 7 Ways to Reverse a List in Python
List Comprehension
Description: Write a program that takes a list of numbers and returns a new list containing only the even numbers. This exercise introduces list comprehension in Python, a concise way to create new lists based on existing ones. You will practice using conditional statements within list comprehensions to filter elements.
Flowchart: Here is a basic flowchart to cover the simple logic of finding even numbers.
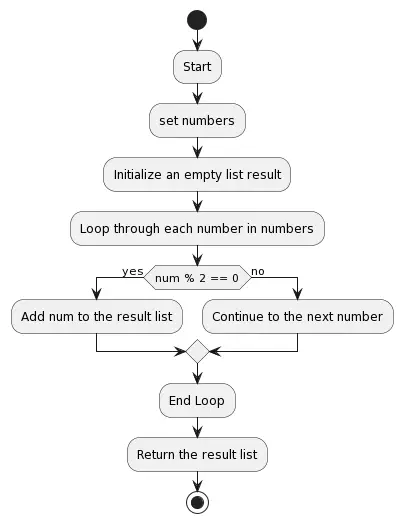
Solution:
def get_even_numbers(numbers): return [num for num in numbers if num % 2 == 0] numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] print("Even numbers:", get_even_numbers(numbers))
FizzBuzz
Description: Write a program to print numbers from 1 to 100! Here’s the catch:
For multiples of 3, print “Fizz”.
For multiples of 5, print “Buzz”.
If a number is a multiple of both 3 and 5, print “FizzBuzz”.
This exercise will help you learn how to use if statements in Python You’ll also get to practice with control flow and the modulo operator (%).
Flowchart: Below is a flowchart to illustrate the FizzBuzz algo.
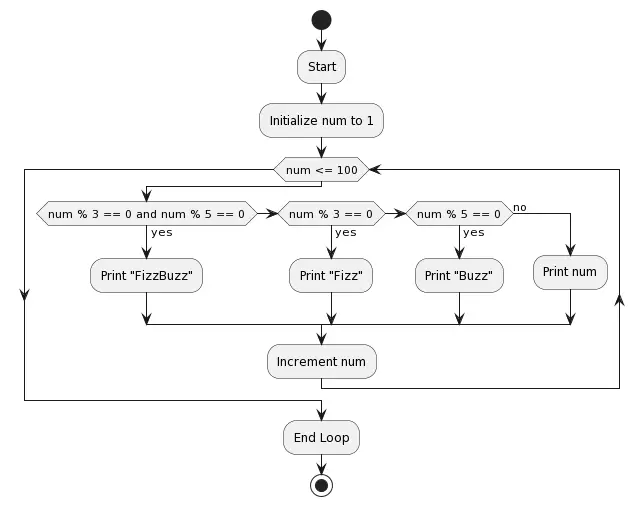
Solution:
for num in range(1, 101): if num % 3 == 0 and num % 5 == 0: print("FizzBuzz") elif num % 3 == 0: print("Fizz") elif num % 5 == 0: print("Buzz") else: print(num)
Check if Two Strings are Anagrams
Description: Write a function that takes two strings as input and returns True if they are anagrams (contain the same characters with the same frequency), False otherwise. This exercise involves comparing the characters and their frequencies in two strings to determine if they are anagrams. You will practice string manipulation, sorting, and comparison.
Flowchart: Refer to this flowchart to create a mind map outlining the steps to solve this problem.
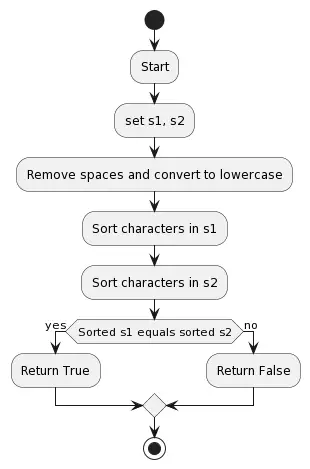
Solution:
def is_anagram(s1, s2): s1 = s1.replace(" ", "").lower() s2 = s2.replace(" ", "").lower() return sorted(s1) == sorted(s2) string1 = "Listen" string2 = "Silent" if is_anagram(string1, string2): print("The strings are anagrams.") else: print("The strings are not anagrams.")
Prime Number Check
Description: Write a function that takes a number and tells you if it’s prime. This exercise focuses on prime number determination using basic looping and mathematical operations. You will learn about loops, conditions, numbers, and Python range().
Flowchart: Refer to this flowchart to understand the logic of filtering prime numbers.
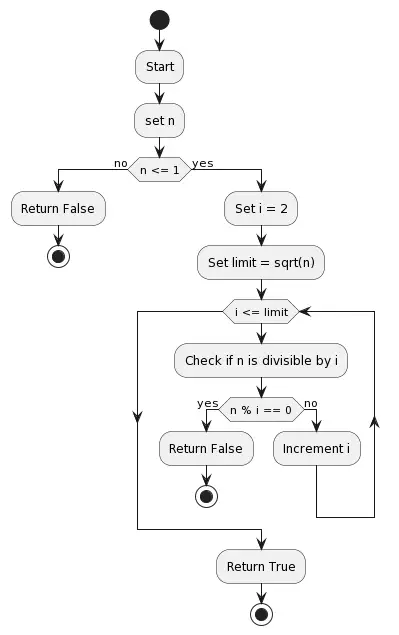
Solution:
def is_prime(n): if n <= 1: return False for i in range(2, int(n**0.5) + 1): if n % i == 0: return False return True number = 23 if is_prime(number): print("The number is prime.") else: print("The number is not prime.")
Level-1 Python Practice Problems
Good, now you must start feeling comfortable in solving Python programming problems. Next, we leave you with 5 basic Python exercises. Start building logic to solve these challenges.
Don’t miss to check out the next 5 Python exercises for beginners. These are often asked in Python’s technical interview rounds, along with their descriptions and solutions.
Python Basic Level-2 Exercises
The difficulty of the exercises will increase gradually in this section. They start with a simple exercise and get more challenging in the end. We encourage you to attempt all of the exercises.
Greatest Common Divisor
Description: Write a function that takes two numbers and returns their greatest common divisor (GCD). This exercise requires implementing the Euclidean algorithm to find the GCD of two numbers. You will practice using loops and conditional statements to perform repetitive calculations.
Flowchart: Before jumping on the code, try to solve it using pen and paper.
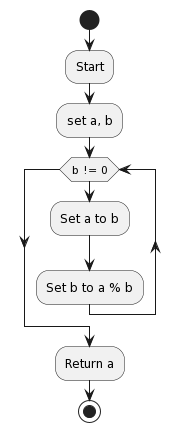
Solution:
def gcd(a, b): while b != 0: a, b = b, a % b return a num1 = 24 num2 = 36 print("GCD:", gcd(num1, num2))
Linear Search
Description: Write a function that takes a list and a target element and returns the index of the target element in the list, or -1 if it is not found. This exercise demonstrates the concept of linear search, which involves iterating through a list to find a specific element. You will practice using loops and conditions to perform element comparisons.
Flowchart: Let’s find out how the linear search works using the below flowchart.
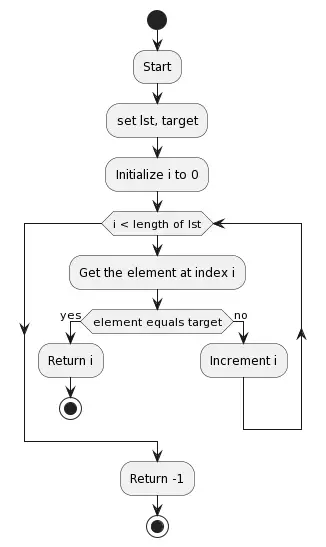
Solution:
def linear_search(lst, target): for i, num in enumerate(lst): if num == target: return i return -1 numbers = [2, 4, 6, 8, 10] target = 6 print("Index:", linear_search(numbers, target))
FizzBuzz with a Twist
Description: Write a program that prints the numbers from 1 to 100. For multiples of three, print “Fizz”. For multiples of five, print “Buzz”. Additionally, for numbers containing the digit 3, print “Fizz”. This exercise builds upon the FizzBuzz concept while introducing additional conditions based on digit presence. You will practice using string manipulation, modulo operations, and conditions.
Solution:
for num in range(1, 101): if num % 3 == 0 or '3' in str(num): print("Fizz", end='') if num % 5 == 0: print("Buzz", end='') if num % 3 != 0 and '3' not in str(num): print(num, end='') print()
Binary Search
Description: Write a function that takes a sorted list and a target element and returns the index of the target element using binary search, or -1 if it is not found. This exercise involves implementing the binary search algorithm to locate an element in a sorted list. You will learn about the concept of divide and conquer, and how to use recursion or iterative approaches to perform a binary search.
Flowchart: Binary search is fancy, and implementing it can be tricky. So, it’s better to draw the flow on paper to avoid too many hits and trials on the computer.
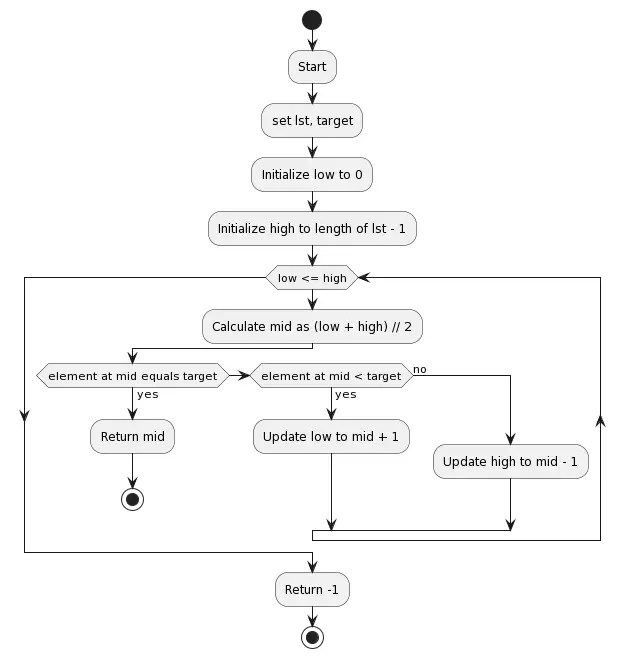
Solution:
def binary_search(lst, target): low = 0 high = len(lst) - 1 while low <= high: mid = (low + high) // 2 if lst[mid] == target: return mid elif lst[mid] < target: low = mid + 1 else: high = mid - 1 return -1 numbers = [1, 3, 5, 7, 9, 11, 13] target = 7 print("Index:", binary_search(numbers, target))
Matrix Transposition
Description: Write a function that takes a matrix (2D list) and returns its transpose (rows become columns and vice versa). This exercise focuses on working with nested lists and performing matrix transposition. You will practice using list comprehension and indexing to transform rows into columns and vice versa.
Solution:
def transpose(matrix): return [[matrix[j][i] for j in range(len(matrix))] for i in range(len(matrix[0]))] matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] transposed_matrix = transpose(matrix) for row in transposed_matrix: print(row)
Level-2 Python Practice Problems
Here are 5 intermediate-level Python exercises for you to practice.
So far, you have solved 20 (10 solved and 10 for practice ) Python exercises for beginners. Now is the time to take up a bit more challenging problems. You may now have reached the apex of problem-solving in Python programming.
Python Basic Level-3 Exercises
These exercises are all challenging, but their solutions comprise different concepts with a good understanding of Python. Solving these exercises will help you develop a sound knowledge of Python programming.
Armstrong Number Check
Description: Write a function that takes a number as input and returns True if it is an Armstrong number (the sum of cubes of its digits is equal to the number itself), False otherwise. This exercise involves decomposing a number into its digits, performing computations, and comparing the result with the original number. You will practice using loops, arithmetic operations, and conditionals.
Flowchart: Do you know that flowcharts are the magic key to solving many difficult problems? They are far away from any language barrier. So, you will get help irrespective of the language you use.
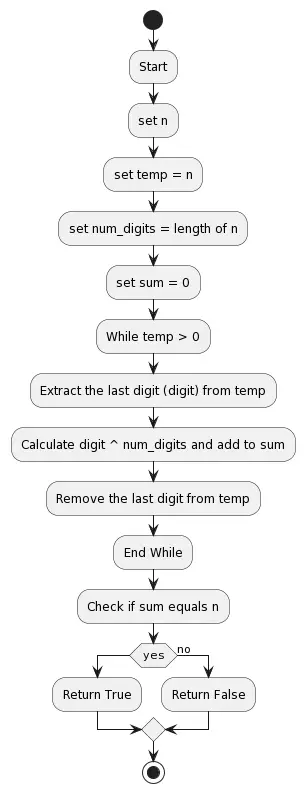
Solution:
def is_armstrong_number(n): temp = n num_digits = len(str(n)) sum = 0 while temp > 0: digit = temp % 10 sum += digit ** num_digits temp //= 10 return sum == n number = 15 if is_armstrong_number(number): print("The number is an Armstrong number.") else: print("The number is not an Armstrong number.")
Fibonacci Series
Description: Write a function that generates the Fibonacci series up to a specified number of terms. This exercise focuses on the Fibonacci sequence, where each number is the sum of the two preceding ones. You will practice using loops and variables to calculate and display the series.
Flowchart: The Fibonacci sequence, named after the Italian mathematician Leonardo of Pisa, also known as Fibonacci, is like a mathematical dance of numbers. Let’s first sketch it out using a flowchart.
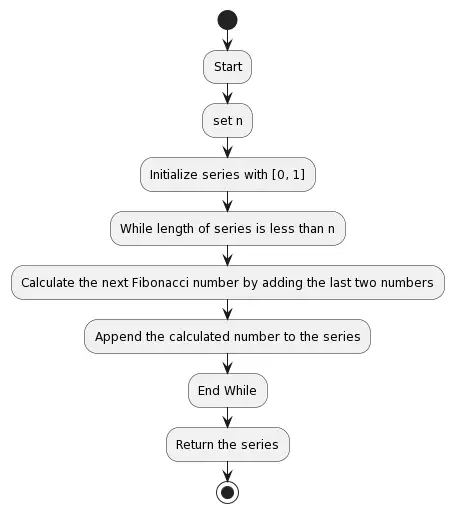
Solution:
def fibonacci_series(n): series = [0, 1] while len(series) < n: series.append(series[-1] + series[-2]) return series num_terms = 10 print("Fibonacci series:", fibonacci_series(num_terms))
Pascal’s Triangle
Description: Write a function that generates Pascal’s triangle up to a specified number of rows. This exercise involves constructing Pascal’s triangle, where each number is the sum of the two numbers directly above it. You will practice using nested loops, lists, and indexing to create and display the triangle.
Flowchart: Pascal’s Triangle is a special arrangement of numbers where each number is the sum of the two above it. It helps in probability, counting combinations, and solving problems in a structured way. It’s like a math tool that makes certain calculations easier. Would you mind if we first solve it using a flowchart?
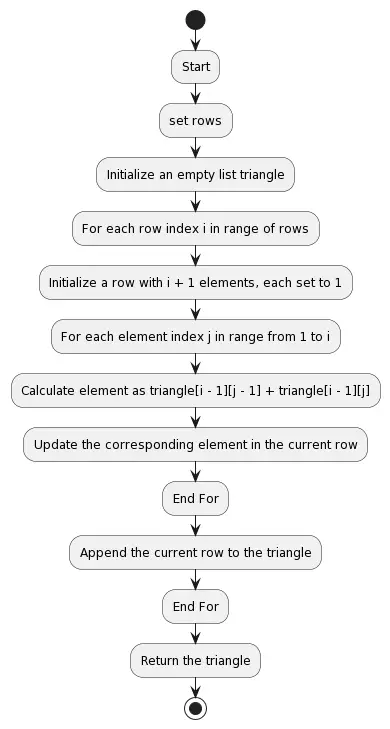
Solution:
def pascals_triangle(rows): triangle = [] for i in range(rows): row = [1] * (i + 1) for j in range(1, i): row[j] = triangle[i - 1][j - 1] + triangle[i - 1][j] triangle.append(row) return triangle num_rows = 5 triangle = pascals_triangle(num_rows) for row in triangle: print(row)
Merge Sort
Description: Write a function that implements the Merge Sort algorithm to sort a list of numbers. This exercise introduces the concept of merge sort, a recursive sorting algorithm that divides the list into smaller sublists, sorts them, and merges them back together. You will learn about recursion, list slicing, and merging sorted lists.
Flowchart: Merge Sort sorts a list by dividing it into tiny parts, sorting each part, and then getting them back together. Take it like, you are sorting toys by breaking them into groups, sorting each group, and then neatly combining them. Below is a flowchart depicting the flow code in the solution part.
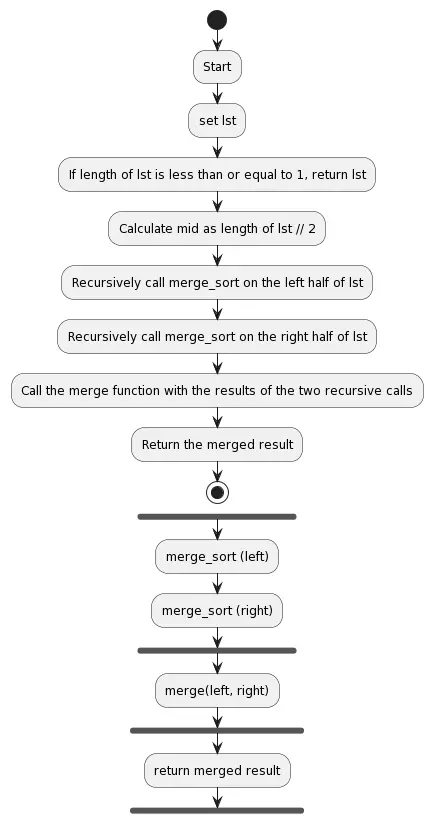
Solution:
def merge_sort(lst): if len(lst) <= 1: return lst mid = len(lst) // 2 left = merge_sort(lst[:mid]) right = merge_sort(lst[mid:]) return merge(left, right) def merge(left, right): merged = [] i = j = 0 while i < len(left) and j < len(right): if left[i] <= right[j]: merged.append(left[i]) i += 1 else: merged.append(right[j]) j += 1 merged.extend(left[i:]) merged.extend(right[j:]) return merged numbers = [5, 3, 8, 4, 2, 1, 6, 9, 7] sorted_numbers = merge_sort(numbers) print("Sorted numbers:", sorted_numbers)
Find the Missing Number
Description: Write a function that takes a list of numbers from 1 to n (with one number missing) and returns the missing number. This exercise involves finding the missing number in a list by comparing the expected and actual sums. You will practice list iteration, arithmetic operations, and conditional statements.
Flowchart: Here”Find the Missing Number” is like solving a number puzzle. You have a list with one number missing. To find it, you add up all the numbers in a special way and compare it with the sum of the given numbers. The difference is the missing number – like figuring out the secret code! Check out its flowchart.
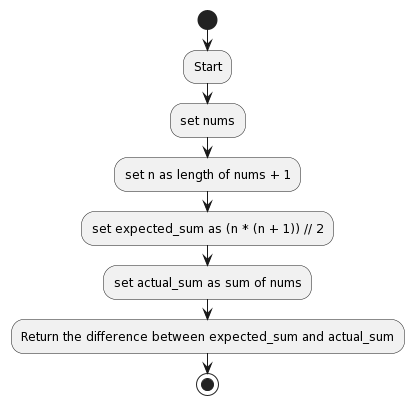
Solution:
def find_missing_number(nums): n = len(nums) + 1 expected_sum = (n * (n + 1)) // 2 actual_sum = sum(nums) return expected_sum - actual_sum numbers = [1, 2, 3, 5, 6, 7, 8, 9, 10] missing_number = find_missing_number(numbers) print("Missing number:", missing_number)
Level-3 Python Practice Problems
Take up the following advanced-level Python exercises and put your knowledge to the test.
Finally, we are in the final lap of 40 Python exercises for beginners. So, brace for an ultimate test of your programming excellence. And, don’t forget to enter the last level of Python programming problems, hit the next button now.
Python Basic Level-4 Exercises
Hey there, beginners! Are you ready to take on the challenge of 5 expert-level Python exercises? Push your coding expertise to the limit and conquer complex problems with advanced algorithms and data structures. Get set to level up your Python skills and become a coding maestro!
Count Words in a Sentence
Description: Write a function that takes a sentence as input and returns the count of each word in the sentence. This exercise focuses on word frequency analysis, where you will split a sentence into words, create a frequency dictionary, and count the occurrences of each word. You will practice string manipulation, loop iteration, and Python dictionary operations.
Flowchart: “Count Words in a Sentence” is like keeping track of people in a group. Think of your sentence as the group. Your task is to figure out how many people (words) are there. You go through each word, and whenever you come across a new one, you mark it down. The final count tells you how many people, or words, are in the group. Let’s create the flowchart.
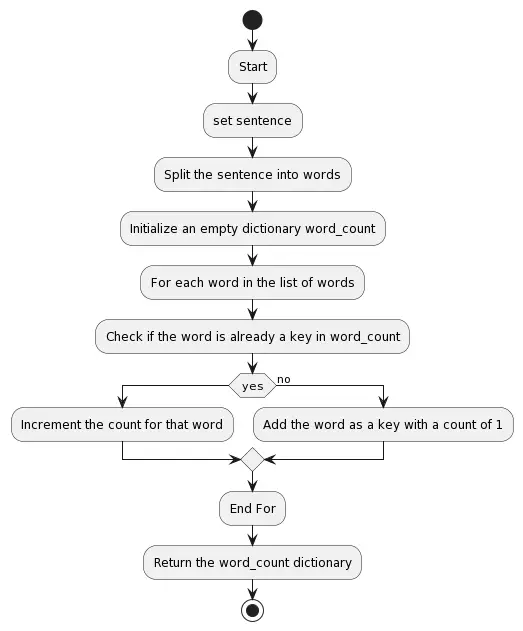
Solution:
def count_words(sentence): words = sentence.split() word_count = {} for word in words: if word in word_count: word_count[word] += 1 else: word_count[word] = 1 return word_count sentence = "I love Python programming. Python is fun!" word_counts = count_words(sentence) for word, count in word_counts.items(): print(word + ":", count)
Remove Duplicates from a List
Description: Write a function that takes a list and returns a new list with duplicate elements removed while preserving the order in the result This exercise focuses on removing duplicates from a list by iterating over it, checking for duplicates, and creating a new list without duplicates. You will practice list manipulation, element comparisons, and list comprehension.
Solution:
def remove_duplicates(lst): unique_lst = [] for num in lst: if num not in unique_lst: unique_lst.append(num) return unique_lst numbers = [1, 2, 3, 2, 4, 1, 5, 6, 3] unique_numbers = remove_duplicates(numbers) print("Unique numbers:", unique_numbers)
Binary to Decimal Conversion
Description: Write a function that takes a binary number as input and returns its decimal equivalent. This exercise involves converting a binary number to its decimal representation using positional notation. You will practice string manipulation, arithmetic operations, and exponentiation.
Solution:
def binary_to_decimal(binary): decimal = 0 power = 0 for digit in reversed(str(binary)): decimal += int(digit) * (2 ** power) power += 1 return decimal binary_number = 101011 decimal_number = binary_to_decimal(binary_number) print("Decimal number:", decimal_number)
Check if Linked List is Palindrome
Description: Write a function that takes the head of a linked list as input and returns True if the linked list is a palindrome (reads the same forward and backward), False otherwise. This exercise focuses on checking if a linked list is a palindrome by comparing elements from both ends. You will practice linked list traversal, stack data structure usage, and element comparisons.
Solution:
class Node: def __init__(self, data=None): self.data = data self.next = None def is_palindrome(head): slow = fast = head stack = [] while fast and fast.next: stack.append(slow.data) slow = slow.next fast = fast.next.next if fast: slow = slow.next while slow: if slow.data != stack.pop(): return False slow = slow.next return True # Create a linked list: 1 -> 2 -> 3 -> 2 -> 1 head = Node(1) head.next = Node(2) head.next.next = Node(3) head.next.next.next = Node(2) head.next.next.next.next = Node(1) if is_palindrome(head): print("The linked list is a palindrome.") else: print("The linked list is not a palindrome.")
These exercises cover a range of concepts and can help beginners improve their problem-solving skills in Python.
Reverse a Linked List
Description: Write a function that takes the head of a linked list and reverses the order of the elements in the list. Return the new head of the reversed list. This exercise tests your understanding of linked lists and requires you to manipulate pointers to reverse the order of the list efficiently.
Solution:
class Node: def __init__(self, data=None): self.data = data self.next = None def reverse_linked_list(head): prev = None current = head while current: next_node = current.next current.next = prev prev = current current = next_node return prev # Create a linked list: 1 -> 2 -> 3 -> 4 -> 5 head = Node(1) head.next = Node(2) head.next.next = Node(3) head.next.next.next = Node(4) head.next.next.next.next = Node(5) print("Original linked list:") current = head while current: print(current.data, end=" ") current = current.next head = reverse_linked_list(head) print("\nReversed linked list:") current = head while current: print(current.data, end=" ") current = current.next
In this exercise, you needed to implement the function reverse_linked_list
that takes the head of a linked list as input. The function reverses the order of list elements by manipulating the pointers of each node. The new head of the reversed list is then returned. The code also includes an example to demonstrate the reversal of a linked list.
Level-4 Python Practice Problems
Finally, check out the last 5 Python programming exercises you should practice to claim proficiency in your programming skills.
Some More Python Programming Problems
Learn Python by Practice: A Quick Summary
We have tried to cover a wide range of fundamental concepts and problem-solving techniques through these 40 Python exercises for beginners. These exercises included several topics such as string manipulation, sorting algorithms, anagram checks, and linked list manipulation.
Such programming tasks are useful for beginners to practice and strengthen their Python skills before interviews. By actively working through these exercises, beginners can gain confidence in their coding abilities.
Let us know, if you want us to create more such exercises. Also, share this tutorial on your Facebook / Twitter accounts to let others get a chance to practice these exercises.
Happy learning,
Team TechBeamers