Welcome, developers! Today, we have something special for you – 50 mind-bending C# coding interview questions that will test your knowledge and skills.
And the best part? Each question includes a unique coding snippet, so you can dive straight into the code and showcase your expertise. Try to attempt each question carefully and test your C# programming skills.
Solving these coding questions should be fun for you. However, we have listed a few quick C# coding tips, go through them first. These could be quite handy in attempting the questionnaire.
C# Coding Test – 50 Questions
Tips to write efficient code in C#.
- Write header comments and place them at the top of files and functions to explain the objective, author, and change history.
- Use single-line comments to define specific statements or codes and indent them accordingly.
- You can also use trailing comments on the same line as code and put a space or a tab to separate them.
- While naming a class, use a noun or a noun phrase. Also, follow the Pascal case – write the first letter of the class name and each subsequent concatenated word in Capital case.
- Don’t use keywords or reserved names while defining namespaces to avoid clashes.
- Instead of using ToUpper(), call the Equals() method for comparing a case-sensitive string.
- Note – ToUpper() will create a second temporary string object.
- Create a String as a verbatim string. Any verbatim string starts with the @ symbol.
- Note – The @ symbol notifies the string constructor to ignore escape characters and line breaks.
You can also read the following posts on C# and excel your programming skills.
- C# Questions – For, While Loops, and If Else Statements
- C# Programming Test with 15 Questions on OOPs and Classes
50 Insanely Awesome Coding Interview Questions for Dev Geniuses!
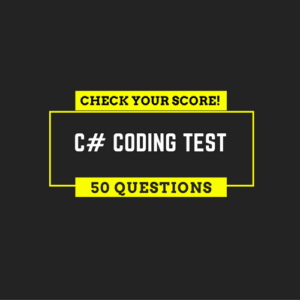
Q-1. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { Console.WriteLine(Math.Round(6.5)); Console.WriteLine(Math.Round(11.5)); } }
a) 6 12
b) 6 11
c) 7 12
d) 7 11
Q-2. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { int[] i = new int[0]; Console.WriteLine(i[0]); } }
a) 0
b) IndexOutOfRangeException
c) Nothing is printed as the array is empty
d) 1
Q-3. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { byte num = 100; dynamic val = num; Console.WriteLine(val.GetType()); val += 100; Console.WriteLine(val.GetType()); } }
a) Error
b) System.Byte
System.Byte
c) System.Byte
System.Int32
d) System.Int32
System.Int32
Q-4. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { #if (!pi) Console.WriteLine("i"); #else Console.WriteLine("PI undefined"); #endif Console.WriteLine("ok"); Console.ReadLine(); } }
a) ok
b) i
ok
c) PI undefined
ok
d) Error
Q-5. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { int[] arr = new int[2]; arr[1] = 10; Object o = arr; int[] arr1 = (int[])o; arr1[1] = 100; Console.WriteLine(arr[1]); ((int[])o)[1] = 1000; Console.WriteLine(arr[1]); } }
a) 10
10
b) 10
100
c) 10
1000
d) 100
1000
Q-6. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { String a = "TechBeamers"; String b = "TECHBEAMERS"; int c; c = a.CompareTo(b); Console.WriteLine(c); } }
a) -1
b) 1
c) 0
d) Error
Q-7. What will be the output of the following code snippet:
using System; public class Program { static void arrayMethod(int[] a) { int[] b = new int[5]; a = b; } public static void Main(string[] args) { int[] arr = new int[10]; arrayMethod(arr); Console.WriteLine(arr.Length); } }
a) 5
b) 10
c) 15
d) Error
Q-8. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { Program p = new Program(); p.print(2, 3, 8); int[] arr = { 2, 11, 15, 20 }; p.print(arr); Console.ReadLine(); } public void print(params int[] b) { foreach (int i in b) { Console.WriteLine(i); } } }
a) 2 3 8
2 11 15 20
b) 2 3 8 11 15 20
c) 2 11 15 20
d) Error
Q-9. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { char x = 'A'; int i = 0; Console.WriteLine (true ? x : 0); Console.WriteLine(false ? i : x); } }
a) 65
65
b) true
false
c) 1
0
d) Error
Q-10. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { int num1 = 20; int num2 = 30; num1 ^= num2 ^= num1 ^= num2; Console.WriteLine(num1 + ","+ num2); } }
a) 20,30
b) 0,20
c) 20,10
d) 10,50
Q-11. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { char[] num = { '1', '2', '3' }; Console.WriteLine(" char array: " + num); } }
a) char array: {123}
b) char array: [123]
c) char array: System.Char[]
d) char array: 123
Q-12. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { Program obj = null; Console.WriteLine(Program.print()); } private static String print() { return "Hi, I am Tech-savvy!!"; } }
a) Hi, I am Tech-savvy!!
b) Error
c) The program compiled successfully and nothing is printed
d) None of the above
Q-13. What will be the output of the following code snippet:
using System; using System.Collections.Generic; public class Program { public static void Main(string[] args) { string[] strings = { "abc", "def", "ghi" }; var actions = new List(); foreach (string str in strings) actions.Add(() => { Console.WriteLine(str); }); foreach (var action in actions) action(); } }
a) abc def ghi
b) ghi ghi ghi
c) abc abc abc
d) Error
Q-14. What will be the output of the following code snippet:
using System; using System.Collections.Generic; public class Program { public static void Main(string[] args) { var actions = new List(); for (int i = 0; i < 4; i++) actions.Add(() => Console.WriteLine(i)); foreach (var action in actions) action(); } }
a) 0 1 2 3
b) 1 2 3 4
c) 4 4 4 4
d) Error
Q-15. What will be the output of the following code snippet:
using System; using System.Collections.Generic; namespace TechBeamers { delegate string del(string str); class sample { public static string DelegateSample(string a) { return a.Replace(',', '*'); } } public class InterviewProgram { public static void Main(string[] args) { del str1 = new del(sample.DelegateSample); string str = str1("Welcome,,friends,,to,,TechBeamers"); Console.WriteLine(str); } } }
a) Welcome, friends, to, TechBeamers
b) Welcome**friends**to**TechBeamers
c) Welcome*friends*to*TechBeamers
d) Welcome friends to TechBeamers
Q-16. What will be the output of the following code snippet:
using System; using System.Collections.Generic; namespace TechBeamers { public delegate void sampleDelegate(int num); public class testDelegate { public void checkEven(int num) { if(num%2 ==0) Console.WriteLine("This number is an even number"); else Console.WriteLine("This number is an odd number"); } public void squareNumber(int num) { Console.WriteLine("The square of this number is: {0}", num*num); } } class sample { public static void Main( ) { testDelegate obj = new testDelegate(); sampleDelegate delegateObj = new sampleDelegate(obj.checkEven); delegateObj += new sampleDelegate(obj.squareNumber); delegateObj(25); } } }
a) Error
b) This number is an odd number
c) The square of this number is: 625
d) This number is an odd number
The square of this number is: 625
Q-17. What will be the output of the following code snippet:
using System; using System.Collections.ObjectModel; using System.Collections.Generic; public class Program { public static void Main() { var arr = new List { 20, 40, 35, 85, 70 }; var collection = new Collection(arr); arr.Add(60); arr.Sort(); Console.WriteLine(String.Join(",", collection)); } }
a) 20, 40, 35, 85, 70
b) 20, 40, 35, 85, 70, 60
c) 20, 35, 40, 60, 70, 85
d) 20, 35, 40, 60, 70
Q-18. What will be the output of the following code snippet:
using System; public class Program { public static void Main() { Nullable number = 0; int num = 1; Console.WriteLine(number.GetType() == num.GetType()); } }
a) True
b) False
c) Null
d) Error
Q-19. What will be the output of the following code snippet:
using System; using System.Collections.ObjectModel; using System.Collections.Generic; namespace TechBeamers { delegate void A(ref string str); public class sample { public static void StringMarker(ref string a) { a = a.Substring(0, a.Length - 6); } } public class Program { public static void Main(string[] args) { A str1; string str = "Let's Learn CSharp"; str1 = sample.StringMarker; str1(ref str); Console.WriteLine(str); } } }
a) Learn CSharp
b) Let’s Learn
c) Let’s Learn CSharp
d) Null
Q-20. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { bool a = true; bool b = false; a ^= b; Console.WriteLine(a); Console.ReadLine(); } }
a) True
b) False
c) Null
d) Error
Q-21. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { bool a = true; bool b = false; a |= b; Console.WriteLine(a); Console.ReadLine(); } }
a) True
b) False
c) Null
d) Error
Q-22. What will be the output of the following code snippet:
using System; public class Program { public static void Main() { classA a = new classC(); Console.WriteLine(a.Print()); } public class classA { public virtual string Print() { return "classA"; } } public class classB : classA { public override string Print() { return "classB"; } } public class classC : classB { public new string Print() { return "ClassC"; } } }
a) ClassA
b) ClassB
c) ClassC
d) Error
Q-23. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { { try { throw new NullReferenceException("C"); Console.WriteLine("A"); } catch (ArithmeticException e) { Console.WriteLine("B"); } Console.ReadLine(); } } }
a) C A
b) C A B
c) B
d) NullReferenceException: C
Q-24. What will be the output of the following code snippet:
using System; namespace TechBeamers { class sample { public int x; private int y; public void assign(int a, int b) { x = a + 1; y = b; } } public class Program { public static void Main(string[] args) { sample s = new sample(); s.assign(1, 1); Console.WriteLine(s.x + " " + s.y); } } }
a) 2 1
b) 1 1
c) Compilation error (y is inaccessible due to its protection level)
d) The program compiled successfully but prints nothing.
Q-25. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { int n = 5; int x = 4; int z, c, k; z = 3 * x * x + 2 * x + 4 / x + 8; for (c = 1; c <= n; c++) { for (k = 1; k <= c; k++) { Console.Write(Convert.ToString(Convert.ToChar(z))); z++; } Console.WriteLine("\n"); } Console.ReadLine(); } }
a) A
BC
DEF
GHIJ
KLMNO
b) A
AA
AAA
AAAA
AAAAA
c) A
AB
ABC
ABCD
ABCDE
d) A
AB
BC
BCD
BCDE
Q-26. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { int i, j = 1, k; for (i = 0; i < 5; i++) { k = j++ + ++j; Console.Write(k + " "); } } }
a) 8 4 16 12 20
b) 4 8 12 16 20
c) 2 4 6 8 10
d) 4 8 16 32 64
Q-27. What will be the output of the following code snippet:
using System; namespace TechBeamers { class Sample { public int num; public int[] arr = new int[10]; public void assign(int i, int val) { arr[i] = val; } } class Program { static void Main(string[] args) { Sample s = new Sample(); s.num = 100; Sample.assign(0, 10); s.assign(0, 9); Console.WriteLine(s.arr[0]); } } }
a) 10
b) 9
c) Compilation Error: an object reference required to access a non-static member
d) 100
Q-28. What will be the output of the following code snippet:
using System; class Program { static void Main(string[] args) { String s1 = "TechBeamers"; String s2 = "Welcomes its readers"; String s3 = s2; Console.WriteLine((s3 == s2) + " " + s2.Equals(s3)); Console.ReadLine(); } }
a) True True
b) True False
c) False True
d) False False
Q-29. What will be the output of the following code snippet:
using System; public class Program { static void Main(string[] args) { string str = "100p"; int i = 0; if(int.TryParse(str,out i)) {Console.WriteLine("Yes string contains Integer and it is " + i); } else { Console.WriteLine("string does not contain an Integer"); } } }
a) Yes string contains Integer and it is 100
b) string does not contain an Integer
c) Error
d) Null
Q-30. What will be the output of the following code snippet:
using System; public class Program { public static void Main() { int[] arr = { 1, 2, 3 }; int i = 1; arr[i++] = arr[i] + 10; Console.WriteLine(String.Join(",", arr)); } }
a) 1,13,3
b) 1,2,3
c) 11,12,13
d) 10,20,30
Q-31. What will be the output of the following code snippet:
using System; class Program { public static int i = 0; public static void Main() { (i++).Assign(); } } static class Extensions { public static void Assign(this int i) { Console.WriteLine(Program.i); Console.WriteLine(i); } }
a) 1 0
b) 1 1
c) 0 1
d) 0 0
Q-32. What will be the output of the following code snippet:
using System; class Program { enum Color: int { red, green, blue = 5, cyan, magenta = 10, yellow } public static void Main() { Console.WriteLine( (int) Color.green + ", " ); Console.WriteLine( (int) Color.yellow ); } }
a) 4,11
b) 1,11
c) 4,7
d) 1,7
Q-33. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { int i = 3; int j = 2; func1(ref i); func2(out j); Console.WriteLine(i + " " + j); } static void func1(ref int num) { num = num + num; } static void func2(out int num) { num = num * num; } }
a) 6 4
b) Compilation error: usage of unassigned out parameter
c) 3 2
d) Compilation Error: function call without creating an object
Q-34. What will be the output of the following code snippet:
using System; class Program { public static void Main() { var test = SingletonB.Test; } } class SingletonB { static readonly SingletonB _instance = new SingletonB(); public static SingletonB Test { get { return _instance; } } private SingletonB() { Console.WriteLine("Default Constructor"); } static SingletonB() { Console.WriteLine("Static Constructor"); } }
a) Static Constructor
b) The program compiles successfully and nothing is printed
c) Default Constructor
Static Constructor
d) Default Constructor
Q-35. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { try { Console.WriteLine("TechBeamers Welcomes Its Readers"); Environment.Exit(0); } finally { Console.WriteLine("To the World of C# !!"); } } }
a) TechBeamers Welcomes Its Readers
To the World of C# !!
b) TechBeamers Welcomes Its Readers
c) Error: unexpected system exit
d) The program compiles successfully but prints nothing.
Q-36. What will be the output of the following code snippet:
using System; public class Calculation { int sum = 0; int count = 0; float average; public void CalAverage() { if ( count == 0) throw ( new CountIsZeroException ("Zero count in DoAverage")); { average = sum / count; Console.WriteLine( "Program executed successfully" ); } } } public class CountIsZeroException : ApplicationException { public CountIsZeroException ( string message ) : base ( message ) { } } class Program { static void Main ( string[ ] args ) { Calculation c = new Calculation(); try { c.CalAverage(); } catch ( CountIsZeroException e ) { Console.WriteLine( "CountIsZeroException : {0}",e ); } Console.ReadLine(); } }
a) CountIsZeroException: Zero count in DoAverage
b) Compilation error: exception not handled properly
c) CountIsZeroException : CountIsZeroException: Zero count in DoAverage
d) Program executed successfully
Q-37. What will be the output of the following code snippet:
using System; public class Program { static void Main(string[] args) { Derived d = new Derived(); int i = 10; d.Func(i); Console.ReadKey(); } } public class Base { public virtual void Func(int x) { Console.WriteLine("Base.Func(int)"); } } public class Derived : Base { public override void Func(int x) { Console.WriteLine("Derived.Func(int)"); } public void Func(object o) { Console.WriteLine("Derived.Func(object)"); } }
a) Derived.Func(object)
b) Derived.Func(int)
c) Derived.Func(int)
Base.Func(int)
d) Base.Func(int)
Q-38. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { string str1 = "TechBeamers"; string str2 = "Techbeamers"; if (str1 == str2) Console.WriteLine("Both Strings are Equal"); else Console.WriteLine("Both Strings are Unequal"); if (str1.Equals(str2)) Console.WriteLine("Both Strings are Equal"); else Console.WriteLine("Both Strings are Unequal"); Console.ReadLine(); } }
a) Both Strings are Equal
Both Strings are Unequal
b) Both Strings are Equal
Both Strings are Equal
c) Both Strings are Unequal
Both Strings are Unequal
d) Both Strings are Unequal
Both Strings are Equal
Q-39. What will be the output of the following code snippet:
using System; class Program { public static void Main() { Sample s = new Sample(); s.Print(); ISample i = s; i.Print(); } public interface ISample { void Print(string val = "Interface Executed"); } public class Sample : ISample { public void Print(string val = "Class Executed") { Console.WriteLine(val); } } }
a) Class Executed
Interface Executed
b) Class Executed
c) Interface Executed
d) Error
Q-40. What will be the output of the following code snippet:
using System; class Program { public static void Main() { int num = 0; (num++); Console.WriteLine(num); } }
a) 0
b) 1
c) Error: wrong use as a statement
d) Nothing gets printed.
Q-41. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { int val = (byte)+(char)-(int)+(long)-2; Console.WriteLine(val); } }
a) Error
b) -2
c) 2
d) 0
Q-42. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { Boolean b1 = true, b2 = false; if ((b2 = true) | (b1 ^ b2)) { Console.WriteLine("execution success"); } else { Console.WriteLine("execution failure"); } } }
a) execution failure
b) execution success
c) Error
d) Null
Q-43. What will be the output of the following code snippet:
using System; class Program { public static void Main() { string str1 = "\U0010FADE"; string str2 = "\U0000FADE"; Console.WriteLine(str1.Length); Console.WriteLine(str2.Length); } }
a) 9
9
b) 10
10
c) 2
1
d) 1
0
Q-44. What will be the output of the following code snippet:
using System; class Program { public static void Main() { int[] singleDimArray = { 1, 2, 3, 4 }; int[,] multiDimArray = { { 1, 2 }, { 3, 4 } }; int[][] jaggedArray = { new int[] { 1, 2 }, new int[] { 3, 4 } }; Console.WriteLine(singleDimArray.Length); Console.WriteLine(multiDimArray.Length); Console.WriteLine(jaggedArray.Length); } }
a) 4
4
2
b) 4
2
2
c) 4
4
4
d) 4
2
4
Q-45. What will be the output of the following code snippet:
using System; class Program { public static void Main() { float num = 56, div = 0; try { Console.WriteLine(num / div); } catch (DivideByZeroException) { Console.WriteLine("Division By Zero"); } } }
a) Runtime Error
b) Compile time Error
c) Division By Zero
d) Infinity
Q-46. What will be the output of the following code snippet:
using System; class Program { public static void Main() { for (int x = 0; x < 10; x++) { Console.Write(x + ' '); } } }
a) 0 1 2 3 4 5 6 7 8 9
b) 0
1
2
3
4
5
6
7
8
9
c) 32333435363738394041
d) Compile time Error
Q-47. What will be the output of the following code snippet:
using System; class Program { static void Main(string[] args) { double num1 = 1.000001; double num2 = 0.000001; Console.WriteLine((num1 - num2) == 1.0); } }
a) True
b) False
c) Null
d) Error
Q-48. What will be the output of the following code snippet:
using System; public class Program { public Program(Object o) { Console.WriteLine("Object argument"); } public Program(double[] arr) { Console.WriteLine("double array argument"); } public static void Main(string[] args) { new Program(null); } }
a) Object argument
b) double array argument
c) Object argument
double array argument
d) The Program compiles successfully but nothing gets printed.
Q-49. What will be the output of the following code snippet:
using System; public class Program { public static void Main(string[] args) { Console.WriteLine("H" + 'I'); Console.WriteLine('h' + 'i'); } }
a) HI
hi
b) 145
209
c) HI
209
d) 145
hi
Q-50. What will be the output of the following code snippet:
using System; using System.Text; public class Program { public static void Main(string[] args) { String str = ""; StringBuilder sb1 = new StringBuilder("TechBeamers"); StringBuilder sb2 = new StringBuilder("TechBeamers"); StringBuilder sb3 = new StringBuilder("Welcome"); StringBuilder sb4 = sb3; if (sb1.Equals(sb2)) str += "1"; if (sb2.Equals(sb3)) str += "2"; if (sb3.Equals(sb4)) str += "3"; String str1 = "TechBeamers"; String str2 = "Welcome"; String str3 = str2; if (str1.Equals(str2)) str += "4"; if (str2.Equals(str3)) str += "5"; Console.WriteLine(str); } }
a) 12345
b) 135
c) 1345
d) Nothing gets printed
Wrapping Up – 50 C# Coding Interview Questions.
If the 50 C# coding interview questions aren’t enough, search for more C# questions on our site. You’ll find many other questions on loops, strings, classes, and OOP concepts.
Next, if you would to share a topic of your interest, shoot us an email for that. We hope, our posts will keep helping you transform into a great programmer.
Lastly, please don’t forget to like or follow us on our social media accounts (Facebook/Twitter). And, check out more CSharp Q&A on various programming topics from our blog.
Keep Learning,
TechBeamers