If you want to test your comfort level with Python functions, check out this quiz. Functions, along with classes, exceptions, and file handling, are essential ingredients of the Python programming ecosystem. Our quiz covers 20 basic questions on Python functions and is an excellent tool for beginners to practice and reinforce concepts.
Test Your Python Function Knowledge with Beginner Level Quiz – Part I
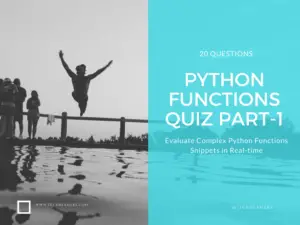
In Python, every entity that holds or references data or metadata is an object, including functions.
This unique ability enables programmers to fully leverage the potential of functions.
To excel in Python programming, it’s essential to know how to optimize your code.
Take the Python functions quiz below and see how you score on this test.
Q-1. What type of value does a Python function return by default?
- A. None
- B. int
- C. double
- D. public
- E. null
Q-2. Which of the following does a Python function include in its header?
- A. function name
- B. function name and parameter list
- C. parameter list
- D. return value
Q-3. Which type of scheme does a Python function use to enclose its arguments?
- A. brackets
- B. parentheses
- C. curly braces
- D. quotation marks
Q-4. Which of the below keywords is used to begin a function in Python?
- A. fun
- B. define
- C. def
- D. function
Q-5. Where does a Python function keep its parameters and local variables?
- A. a heap
- B. storage area
- C. a stack
- D. an array
Q-6. Which of the given scenario functions won’t return any value?
- A. a function that prints integers from 1 to 100.
- B. a function that returns a random integer from 1 to 100.
- C. a function that checks whether the current second is an integer from 1 to 100.
- D. a function that converts an uppercase letter to a lowercase.
Q-7. Which of the given options fit correctly in the function body?
def f(number): # Missing function body print(f(5))
- A. return “number”
- B. print(number)
- C. print(“number”)
- D. return number
Q-8. What is the output of the following Python code?
def func(message, num = 1): print(message * num) func('Welcome') func('Viewers', 3)
- A. Welcome
Viewers - B. Welcome
ViewersViewersViewers - C. Welcome
Viewers, Viewers, Viewers - D. Welcome
Q-9. What will the Python function return in the below code?
def myfunc(text, num): while num > 0: print(text) num = num - 1 myfunc('Hello', 4)
- A. HelloHelloHelloHelloHello
- B. HelloHelloHelloHello
- C. invalid call
- D. infinite loop
Q-10. Which of the following is a valid statement in Python?
- A. function invocation
- B. pass-by-value
- C. pass by reference
- D. pass by name
Q-11. What is the output of the following Python code?
def func(x = 1, y = 2): x = x + y y += 1 print(x, y) func(y = 2, x = 1)
- A. 1 3
- B. 2 3
- C. The program has a runtime error because x and y are not defined.
- D. 3 2
- E. 3 3
Q-12. What does the function in the below Python code return?
num = 1 def func(): num = 3 print(num) func() print(num)
- A. 1 3
- B. 3 1
- C. 1 1
- D. 3 3
Q-13. What is the output of the following Python coding snippet? It is modifying a static variable.
num = 1 def func(): num = num + 3 print(num) func() print(num)
- A. 1 4
- B. 4 1
- C. The program has a runtime error because the local variable ‘num’ is referenced before the assignment.
- D. 1 1
- E. 4 4
Q-14. What is the output of the following Python coding snippet? It is modifying a global variable.
num = 1 def func(): global num num = num + 3 print(num) func() print(num)
- A. 1 4
- B. 4 1
- C. 1 1
- D. 4 4
Q-15. What will the below Python function return? It has default values set for its parameters.
def test(x = 1, y = 2): x = x + y y += 1 print(x, y) test()
- A. 1 3
- B. 3 1
- C. 1 1
- D. 3 3
Q-16. What is the output of the following Python code? Will it behave differently from the previous question?
def test(x = 1, y = 2): x = x + y y += 1 print(x, y) test(2, 1)
- A. 1 3
- B. 2 3
- C. 3 2
- D. 3 3
Q-17. This Python script is playing around with the parameters. Try to understand how and answer.
def test(x = 1, y = 2): x = x + y y += 1 print(x, y) test(y = 2, x = 1)
- A. 1 3
- B. 2 3
- C. 3 2
- D. 3 3
Q-18. Which of the following is a correct Python function header?
- A. def f(a = 1, b):
- B. def f(a = 1, b, c = 2):
- C. def f(a = 1, b = 1, c = 2):
- D. def f(a = 1, b = 1, c = 2, d):
Q-19. What does the following Python code print?
exp = lambda x: x ** 3 print(exp(2))
- A. 6
- B. 222
- C. 8
- D. None of the above
Q-20. What does the following Python script using the lambda operator return?
myList = [lambda x: x ** 2, lambda x: x ** 3, lambda x: x ** 4] for f in myList: print(f(3))
- A. 27
81
343 - B. 6
9
12 - C. 9
27
81 - D. 8
27
64
Recap: Python Functions Quiz Part 1 – Beginner Level
We hope you enjoyed taking the Python Functions Quiz – Part 1. If you’re interested in exploring more programming topics in Python, check out our collection of quizzes in the section below.
More Food for Thoughts
- Entry-Level Java Developer Quiz
- Java Quiz on Exception Handling with 20 Questions
- Entry-Level Python Developer Quiz
- Python File Handling Quiz for Beginners
- Python File Handling Quiz for Experienced
- 30 Python List, Tuple, Dictionary Questions
- Python Functions Quiz Part II
- Automation Testing Quiz for Software Engineers
- Linux Basic Question and Answers for Starters
Stay tuned for the upcoming Python tutorials and quizzes. Keep learning and expanding your knowledge.
Thank you,
TechBeamers.