We’ve curated this online Python quiz for beginners learning Python programming. It enlists 20 questions on Python classes and objects. It’s easy to define a class in Python using the <class> keyword. Check out a simple Python class definition below.
class TestClass: '''It is a doc string. I've implemented a new class.''' pass
In the above code, you can see the highlighted doc string specifying the description of the class. Let’s now see a little more about classes before moving on to the quiz.
Basics of Python Classes
In Python, every class has a local namespace to define all its members which could either be functions or variables. Also, every Python class holds a set of special attributes. Each of them starts and ends with a double underscore sign (__). If you consider the above example, then using the __doc__ will return the doc string of that class.
Once you have the class defined, it is easy to instantiate it anytime. You can use a class object to reference any of its attributes. Let’s see a simple example to define the class instance.
>>> myObj = TestClass()
Like constructors in C++, we’ve __init__() method in Python. This function gets called every time you instantiate the class. Please refer to the below example to view a complete Python class.
class EmployeeData: def __init__(self, sal=0, age=0): self.sal = sal self.age = age def getData(self): print("{0}+{1}j".format(self.sal,self.age))
Discover our complete Python tutorial that caters to all your programming requirements in Python.
Let’s now start the online Python quiz for beginners.
Online Python Quiz for Beginners – Classes and Objects.
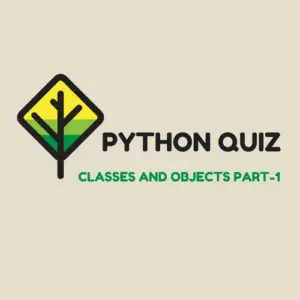
Q-1. Which of the following represents a distinctly identifiable entity in the real world?
A. A class
B. An object
C. A method
D. A data field
Q-2. Which of the following represents a template, blueprint, or contract that defines objects of the same type?
A. A class
B. An object
C. A method
D. A data field
Q-3. Which of the following keywords mark the beginning of the class definition?
A. def
B. return
C. class
D. All of the above.
Q-4. Which of the following is required to create a new instance of the class?
A. A constructor
B. A class
C. A value-returning method
D. A None method
Q-5. Which of the following statements is most accurate for the declaration x = Circle()?
A. x contains an int value.
B. x contains an object of the Circle type.
C. x contains a reference to a Circle object.
D. You can assign an int value to x.
Q-6. What will be the output of the following code snippet?
class Sales: def __init__(self, id): self.id = id id = 100 val = Sales(123) print (val.id)
A. SyntaxError, this program will not run
B. 100
C. 123
D. None of the above
Q-7. Which of the following statements is correct?
A. A reference variable is an object.
B. A reference variable refers to an object.
C. An object may contain other objects.
D. An object can contain references to other objects.
Q-8. What will be the output of the following?
s = "\t\tWelcome\n" print(s.strip())
A. \t\tWelcome\n
B. Welcome\n
C. \t\tWELCOME
D. Welcome
Q-9. What will be the output of the following code snippet?
class Person: def __init__(self, id): self.id = id sam = Person(100) sam.__dict__['age'] = 49 print (sam.age + len(sam.__dict__))
A. 1
B. 2
C. 49
D. 50
E. 51
Q-10. Which of the following can be used to invoke the __init__ method in B from A, where A is a subclass of B?
A. super().__init__()
B. super().__init__(self)
C. B.__init__()
D. B.__init__(self)
Q-11. Which of the following statements are correct about the given code snippet?
class A: def __init__(self, i = 0): self.i = i class B(A): def __init__(self, j = 0): self.j = j def main(): b = B() print(b.i) print(b.j) main()
A. Class B inherits A, but the data field “i” in A is not inherited.
B. Class B inherits A, thus automatically inherits all data fields in A.
C. When you create an object of B, you have to pass an argument such as B(5).
D. The data field “j” cannot be accessed by object b.
Q-12. Which of the following statements is true?
A. By default, the __new__() method invokes the __init__ method.
B. The __new__() method is defined in the object class.
C. The __init__() method is defined in the object class.
D. The __str__() method is defined in the object class.
E. The __eq__(other) method is defined in the object class.
Q-13. What will be the output of the following code snippet?
class A: def __init__(self): self.calcI(30) print("i from A is", self.i) def calcI(self, i): self.i = 2 * i; class B(A): def __init__(self): super().__init__() def calcI(self, i): self.i = 3 * i; b = B()
A. The __init__ method of only class B gets invoked.
B. The __init__ method of class A gets invoked and it displays “i from A is 0”.
C. The __init__ method of class A gets invoked and it displays “i from A is 60”.
D. The __init__ method of class A gets invoked and it displays “i from A is 90”.
Q-14. What will be the output of the following code snippet?
class A: def __init__(self): self.calcI(30) def calcI(self, i): self.i = 2 * i; class B(A): def __init__(self): super().__init__() print("i from B is", self.i) def calcI(self, i): self.i = 3 * i; b = B()
A. The __init__ method of only class B gets invoked.
B. The __init__ method of class A gets invoked and it displays “i from B is 0”.
C. The __init__ method of class A gets invoked and it displays “i from B is 60”.
D. The __init__ method of class A gets invoked and it displays “i from B is 90”.
Q-15. Which of the following statements can be used to check, whether an object “obj” is an instance of class A or not?
A. obj.isinstance(A)
B. A.isinstance(obj)
C. isinstance(obj, A)
D. isinstance(A, obj)
Q-16. What relationship correctly fits for University and Professor?
A. association
B. composition
C. inheritance
D. All of the above
Q-17. What relationship is suited for the Course and Faculty?
A. association
B. composition
C. inheritance
D. None of the above
Q-18. What relationship is best suited for Employee and Person?
A. association
B. composition
C. inheritance
D. None of the above
Q-19. What relationship is best suited for House and Door?
A. association
B. composition
C. inheritance
D. All of the above
Q-20. What relationship is appropriate for Fruit and Papaya?
A. association
B. composition
C. inheritance
D. All of the above
Summary – Online Python Quiz for Beginners.
The scope of Python classes is significantly large. That’s why we covered the basics in this online Python quiz and targeted it for beginners. We are hoping that it’ll help all freshers succeed in their Python programming interviews.
In the next post, we’ll showcase the advanced concepts of Python classes and related topics. So let’s end this quiz with an encouraging quote.
Disliking bugs in his own code makes a better programmer.
All the Best,
TechBeamers.