In this tutorial, you’ll learn about Python operator precedence and associativity. This topic is crucial for programmers to understand the semantics of Python operators. After reading it, you should be able to know how Python evaluates the order of its operators.
Python Operator Precedence and Associativity
Some operators in Python have higher precedence than others such as the multiplication operator has higher priority than the addition operator, so do multiplication before addition.
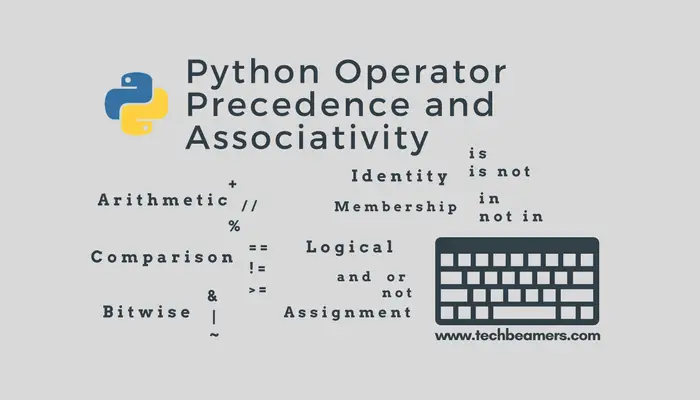
Python operator precedence
In an expression, the Python interpreter evaluates operators with higher precedence first. And, except the exponent operator (**) all other operators get evaluated from left to right.
How does the operator precedence work in Python?
When we group a set of values, variables, operators or function calls that turn out as an expression. And once you execute that expression, the Python interpreter evaluates it as a valid expression. See a simple example given below.
>>> 3 + 4 7
Here, the ‘3 +4’ is a Python statement. It contains one operator and two operands. However, a more complex statement can include multiple operators.
To evaluate complex expressions, Python lays out the rule of precedence. It governs the order in which the operations take place.
Examples of operator precedence in Python
See the below example which combines multiple operators to form a compound expression.
# Multiplication get evaluated before # the addition operation # Result: 17 5 + 4 * 3
However, it is possible to alter the evaluation order with the help of parentheses (). It can override the precedence of the arithmetic operators.
# Parentheses () overriding the precedence of the arithmetic operators # Output: 27 (5 + 4) * 3
Operator precedence table in Python
Refer to the below table which lists the operators with the highest precedence at the top and lowest at the bottom.
Operators | Usage |
---|---|
{ } | Parentheses (grouping) |
f(args…) | Function call |
x[index:index] | Slicing |
x[index] | Subscription |
x.attribute | Attribute reference |
** | Exponent |
~x | Bitwise not |
+x, -x | Positive, negative |
*, /, % | Product, division, remainder |
+, – | Addition, subtraction |
<<, >> | Shifts left/right |
& | Bitwise AND |
^ | Bitwise XOR |
| | Bitwise OR |
in, not in, is, is not, <, <=, >, >=, <>, !=, == | Comparisons, membership, identity |
not x | Boolean NOT |
and | Boolean AND |
or | Boolean OR |
lambda | Lambda expression |
Python operator associativity
In the above table, you can confirm that some of the groups have many operators. It means that all operators in a group are at the same precedence level.
And, whenever two or more operators have the same precedence, then associativity defines the order of operations.
What does associativity mean in Python?
The associativity is the order in which Python evaluates an expression containing multiple operators of the same precedence. Almost all operators except the exponent (**) support the left-to-right associativity.
Examples of associativity in Python
For example, the product (*) and the modulus (%) have the same precedence. So, if both appear in an expression, then the left one will get evaluated first.
# Testing Left-right associativity # Result: 1 print(4 * 7 % 3) # Testing left-right associativity # Result: 0 print(2 * (10 % 5))
As said earlier, the only operator that has right-to-left associativity in Python is the exponent (**) operator. See the examples below.
# Checking right-left associativity of ** exponent operator # Output: 256 print(4 ** 2 ** 2) # Checking the right-left associativity # of ** # Output: 256 print((4 ** 2) ** 2)
You might have observed that the ‘print(4 ** 2 ** 2)’ is similar to ‘(4 ** 2 ** 2).
Nonassociative operators in Python
Python does have some operators such as assignment operators and comparison operators which don’t support associativity. Instead, there are special rules for the ordering of this type of operator which can’t be managed via associativity.
Examples of nonassociative operators
For example, the expression 5 < 7 < 9 does not mean (5 < 7) < 9 or 5 < (7 < 9). Also, the statement 5 < 7 < 9 is the same as 5 < 7 and 7 < 9 and gets evaluated from left to right.
Moreover, chaining of assignment operators like a = b = c is perfectly alright whereas the ‘a = b += c’ will result in an error.
# Set the values of a, b, c x = 11, y = 12, z = 13 # Expression is incorrect # Non-associative operators # Error -> SyntaxError: invalid syntax x = y += 12
Now, you might also like to check out the most versatile XOR operator in Python. It can come in handy in many of your programming tasks.
Conclusion – Python operator precedence
This tutorial did cover a very important topic – Python operator precedence and associativity. So, it should now be easier for you to create compound/complex expressions in Python.
If you find something new to learn today, then do share it with others. And, follow us on our social media accounts to get the latest tutorials and updates.
Best,
TechBeamers