We’ve assembled 15 cool C Programming Tips and Tricks in this article.
If you are a “C” learning student or a “C” programmer, then these tips are for you and can come quite handy in your work assignments.
We discovered some of these ideas after lots of reading and some while working. All of our tips are based on real-time use cases that will help you solve real-time issues.
If you also have a few ideas in store, then don’t mind sharing them with us. Use the comment box section and let others know as well.
Also, you can participate in our knowledge drive by distributing this post to your social circle and friend groups.
Let’s now start looking into the tips one by one.
Check Out the Best 15 C Programming Tips and Tricks
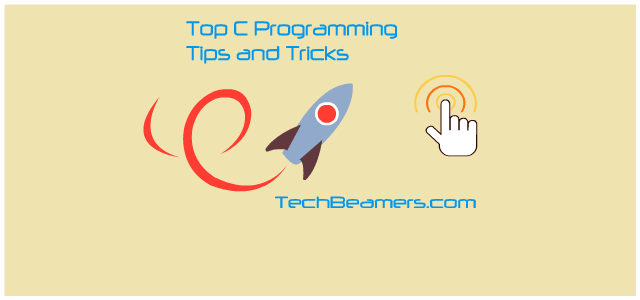
C Programming Tips#1) – Macro to Get Array Size of Any Data Type
The following macro will help you in getting the size of an array of any data type. It works by dividing the length of the array by the size of its field.
#define NUM_OF(x) (sizeof
(x) / sizeof
(*x))
#define num(x) (sizeof (x) / sizeof (*x)) int _tmain(){ int number[10] = {1,1,1,1,1,1}; char *teststr[20] = {"","","","","","","","",""}; printf("Size of number[10] is %d\n", num(number)); printf("Size of teststr[20] is %d\n", num(teststr)); }
Size of number[10] is 10 Size of teststr[20] is 20 Press any key to continue . . .
C Programming Tips#2) – Calculate Elapsed Time
Friends, have you ever found yourself in a situation where you needed to measure the time span between two occurrences? Or monitor a process that is unexpectedly consuming more time than anticipated?
Here is the code snippet implemented using a set of macros to help you figure out how long something will take to run.
#include "stdafx.h" #include <time.h> #include <windows.h> #include <stdlib.h> clock_t startm, stopm; #define BEGIN if ( (startm = clock()) == -1) \ { \ printf("clock returned error.");exit(1); \ } \ #define CLOSE if ( (stopm = clock()) == -1) \ {printf("clock returned error."); \ exit(1); \ } \ #define SHOWTIME printf( "%6.3f seconds elapsed.", ((double)stopm-startm)/CLOCKS_PER_SEC);
main() { BEGIN; // Specify set of instructions for you want to measure execution time Sleep(10); CLOSE; SHOWTIME; }
C Programming Tips#3) – Smart Random Number Generator
In C programming, the stdlib.h
header provides the rand() function for generating numbers. Did you use it and realize that every time you run your program, it returns the same result?
It’s because, by default, the standard (pseudo) random number generator gets seeded with the number 1. To have it start anywhere else in the series, call the function srand
(unsigned int seed).
For the seed, you can use the current time in seconds.
#include <time.h> // At the beginning of main, or at least before you use rand() srand(time(NULL));
Annexure:
For your note, the above code seeds the generator from the current second of time. This fact implies that if you expect your program to re-run more than once a second, the given code may not fulfill your requirement. A possible workaround is to store the seed in a file (that you will read later from your program), and you then increment it every time the program is run.
C Programming Tips#4) – Heard of “goes to-->
” Operator?
In C programming, the symbol (–>) doesn’t represent an operator.
Instead, it is a combination of two separate operators, i.e., --
and >
known as the “goes to.”
To understand how the “goes to” operator works, go through the below code snippet.
In the example, there is conditional code that decrements variable x, while returning x’s original (not decremented) value, and then compares it with 0 using the > operator.
int _tmain(){ int x = 10; while( x --> 0 ) // x goes to 0 { printf("%d ", x); } printf("\n"); }
9 8 7 6 5 4 3 2 1 0 Press any key to continue . . .
Tip#5) – Some Cool SCANF Tricks
Find out some of the unheard scanf()
tricks that you must know.
scanf(“%[^,]”, a); // This doesn’t scrap the comma scanf(“%[^,],”,a); // This one scraps the comma
scanf(“%[^\n]\n”, a); // It will read until you meet ‘\n’, then trashes the ‘\n’
scanf(“%*s %s”, last_name); // last_name is a variable
C Programming Tips#6) – Call Functions at Program Termination
Did you know about the atexit()
API? This C API is used to register functions that can get automatically called when the program finishes its execution.
For example –
#include <stdio.h> #include <stdlib.h> void foo(void) { printf("Goodbye Foo!\n"); } void bar(void) { printf("Goodbye Bar!\n"); } int main(int argc, wchar_t* argv[]) { atexit(bar); atexit(foo); return 0; }
Notice that foo and bar functions haven’t been called but are registered to get called when the program exits.
These should not return anything nor accept any arguments. You can register up to 32 such functions. They’ll get called in the LIFO order.
Tip#7) – Initialize a 2-D Array with a Long List of Values
It can be easily achieved by keeping the list values in a file and then storing the file content in the 2-D array with the following line of code.
double array[SIZE][SIZE] = { #include "float_values.txt" }
C Programming Tips#8) – Add Any Numbers without “+” Operator
Bitwise operators can be used to perform the addition (+) operation as shown in below C code:
int Add(int x, int y) { if (y == 0) return x; else return Add( x ^ y, (x & y) << 1); }
Tip#9) – Swapping Two Variables without Any Temp Variable
There are three ways to do this which I’ve mentioned below.
To swap two variables without using additional space or arithmetic operators, you can simply use the xor operator.
a = a ^ b; b = a ^ b; a = a ^ b; // OR a = a + b –(b=a); // OR a ^= b ^= a ^= b;
Tip#10) – Put the Constant As the First Term While Making Comparisons
Sometimes, we tend to confuse the “=” operator with the “==” operator. To avoid this, use the defensive programming approach. 0==x instead of x==0 so that 0=x can be caught by
It means you should write “1==x” instead of “x==1” so that the compiler will always flag an error for the miswritten “1=x”.
So whenever you mistakenly write the following.
if ( 1 = x )
The compiler will complain and refuse to compile the program.
While it’s not possible if you are comparing two variables. For example, the expression
if (x == y)
can be incorrectly written as
if(x = y)
Tip#11) – Quick Comment Your Code
Sometimes you may find yourself trying to comment out a block of code that already has some comments inside. Because C does not allow nested comments, you may find that the */ comment ends prematurely terminating your comment block.
You can utilize the C Preprocessor’s #if directive to circumvent this:
#if 0 /* This code here is the stuff we want commented */ if (a != 0) { b = 0; } #endif
Tip#12) – Use of Conditional Operator
The Conditional operator is also known as the Ternary operator. We mostly use it in the following form:
x = (y < 0) ? 10 : 20;
But in C++, you can also use it in the following manner:
(c < 0 ? a : b) = 1; // If c < 0 then a = 1 // If c > 0 then b = 1
Tip#13) – Arrays and Pointers not Entirely the Same
Many of us tend to misunderstand the concept that pointers and arrays are the same. They are not.
Pointers are merely variables holding the address of some location whereas an array is a contiguous sequence of memory locations.
Pointers can help to create heterogeneous data structures such as a link list or hash table. Whereas the arrays are homogenous and can hold only values of a similar type such as numbers and strings.
Pointers get allocated dynamically on the heap whereas the arrays are static allocations on the stack.
At compile time, an array is an array. Only during run-time, does an array devolve to a pointer.
To prove this fact, let me show you an example.
int a[10] = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 }; int *b = a; printf("%d\n%d\n", sizeof(a), sizeof(b)); And the output is (assuming size of int is 4 bytes and address size is 8 bytes) – 40 8
Tip#14) – Pointer to array and Array of Pointers.
Let’s check out an interesting comparison between the following three declarations.
int *ptr1[5]; int (*ptr2)[5]; int* (ptr3[5])
int *ptr1[5];
Here in int *ptr1[5], ptr1 is an array of 5 integer pointers (An array of int pointers).
int (*ptr2)[5];
And in int (*ptr2)[5], ptr2 is a pointer to an array of 5 integers (A pointer to an array of integers).
int* (ptr3[5]);
It’s the same as ptr1 (An array of int pointers).
Tip#15) – Log off the computer Using C.
#include <windows.h> int main(){ system("shutdown -l -f -t 00"); }
Summary – C Programming Tips and Tricks
While starting to write this article, we thought to present you with ten best of the C programming tips but ended up delivering 15 wonderful tips.
We tried to cover those tips that you can relate to and that are usable in your production environment. For your information, we’re deeply inspired by the father of C “Dennis Ritchie.”
Before we conclude, a humble request to you is to share this post with your friends. And also leave your valuable feedback in the comment box at the end of this post.
You are most welcome to ask questions about this post which we’ll be more than happy to answer.
However, below are some tutorials and posts that we recommend for you to read and build a better understanding of C/C++ programming.