Selenium Webdriver is a powerful automation tool for testing web applications. It’s an open-source library bundled with a rich set of features. Using it for testing a website is just like simulating the actions of a real user. All these abilities together make it a perfect tool for automation. But you need proper guidance to get the best out of it. In this blog post, we’ve assembled some of the best Selenium Webdriver coding tips for software testers.
With these tips, you’ll be able to optimize code, improve quality, and provide robust automation solutions. Selenium Webdriver supports a lot of programming languages such as Java, Python, C#, and Ruby. But in this article, we’ll focus only on Java-based tips for Selenium. Though, you can easily change them to the language of your choice.
To download the latest Selenium automation tool, please Download Selenium Webdriver. And follow the step-by-step Selenium Webdriver tutorial to create a quick Selenium demo from scratch.
20 Selenium Webdriver Coding Tips
With these Selenium Webdriver coding tips, we’ve tried to cover various use cases that you face in real time. We expect you to use these coding snippets directly in your current project assignments. BTW if you fall into any issues while using these tips, then do write to us. We’ll try to respond to every query of our readers.
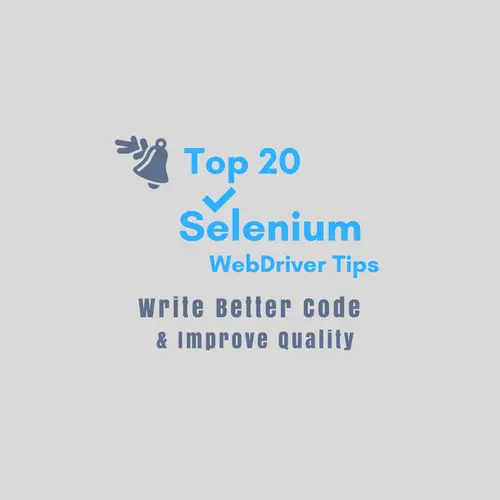
Tip-1: Best method to create a Webdriver instance.
It’s one of the most common Selenium Webdriver coding tips that you can’t avoid using.
1.1- Use the <Factory> design pattern to create objects based on browser type.
1.2- Extend the below code or use it as is in your projects.
public class DriverFactory { private WebDriver driver = null; public static WebDriver getBrowser(String browserType) { if (driver == null) { if (browserType.equals("Firefox")) { driver = new FirefoxDriver(); } else if (browserType.equals("Chrome")) { driver = new ChromeDriver(); } else if (browserType.equals("IE")) { driver = new InternetExplorerDriver(); } } return driver; } }
Tip-2: Simple method to check if an element exists.
2.1- You should use <findElements> instead of <findElement>.
2.2- <findElements> returns an empty list when it doesn’t find any matching elements.
2.3- You can use the code given below to check for the presence of an element.
Boolean isItemPresent = driver.findElements(By.testLocator).size() > 0
Tip-3: Avoid Exception while checking an element exists.
3.1- The code in the previous tip may lead to <NoSuchElementException>. Also, it could be a bit slower in some cases.
3.2- Webdriver has a Fluent wait feature. We can use it to check the element.
3.3- It gives the ability to ignore any exception and allows to testing of the <WebElement>. Let’s see how to achieve what’s said here.
/* 1- Test if an element exists on the page. 2- Ignore the no element found exception. */ By element = By.xpath(".//*[@id='demo']/p"); Wait < WebDriver > wait = new FluentWait < > (driver) .withTimeout(60, TimeUnit.SECONDS) .pollingEvery(5, TimeUnit.SECONDS) .ignoring(NoSuchElementException.class); wait.until(ExpectedConditions .presenceOfElementLocated(element));
Tip-4: Wait for a page with JavaScript(JS) to load.
4.1- It’s quite common to work with pages bloated with JavaScript. The challenge is how to make sure the page has finished loading.
4.2- Here, we are giving a solution that works. This code will help you set to wait for a page containing JavaScript.
wait.until(new Predicate < WebDriver > () { @Override public Boolean apply(WebDriver driver) { return ((JavascriptExecutor) driver).executeScript("return document.readyState").equals("complete"); } });
Tip-5: Take a screenshot using Webdriver.
5.1- It’s easy to capture a screenshot of any error using Webdriver.
5.2- Use the below Webdriver code. It saves the screenshot to the current project directory.
WebDriver driver = new FirefoxDriver(); driver.get("https://techBeamers.com/"); // Store the screenshot in current project dir. String screenShot = System.getProperty("user.dir") + "\\screenShot.png"; // Call Webdriver to click the screenshot. File scrFile = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE); // Save the screenshot. FileUtils.copyFile(scrFile, new File(screenShot));
Tip-6: Take a partial screenshot using Selenium Webdriver.
It’s one of the premium Selenium Webdriver coding tips.
6.1- Sometimes you only need to take a screenshot of the part of the screen. Like only one frame within a set of frames.
6.2- You can add the below code in your project to shoot the image of a particular frame.
6.3- Find the <WebElement> of the frame and pass it to the function given below.
public void takePartialScreenShot(WebElement element) throws IOException { String screenShot = System.getProperty("user.dir") + "\\screenShot.png"; File screen = ((TakesScreenshot) this.driver).getScreenshotAs(OutputType.FILE); Point p = element.getLocation(); int width = element.getSize().getWidth(); int height = element.getSize().getHeight(); BufferedImage img = ImageIO.read(screen); BufferedImage dest = img.getSubimage(p.getX(), p.getY(), width, height); ImageIO.write(dest, "png", screen); FileUtils.copyFile(screen, new File(screenShot)); }
6.4- You may require adding a few imports to get the above code working.
import org.openqa.selenium.Point; import org.openqa.selenium.TakesScreenshot; import java.awt.image.BufferedImage; import javax.imageio.ImageIO;
6.5- Alternatively, you can use the <CTRL+SHIFT+O> shortcut in Eclipse.
Tip-7: Get the HTML Source of WebElement in Selenium WebDriver.
7.1- Webdriver’s <WebElement> class provides <getAttribute()> method to get the HTML inside the element.
7.2- You need to pass the <innerHTML> as an attribute type in the above method. See the code below.
String html = element.getAttribute("innerHTML");
Tip-8: How to select/get the drop-down option in Selenium Webdriver.
You can achieve this in the following two steps.
8.1- Select an option based on the label.
Select dropdown = new Select(driver.findElement(By.xpath("//drop_down_x_path"))); dropdown.deselectAll(); dropdown.selectByVisibleText("selectLabel");
8.2- Get the first selected value from the drop-down.
WebElement option = dropdown.getFirstSelectedOption();
Tip-9: Refreshing web page by WebDriver during tests.
9.1- The below code should refresh the web page.
driver.navigate().refresh();
Tip-10: Run A JavaScript Code Using Selenium Webdriver.
10.1- When you are testing a website, you can’t skip using JavaScript.
10.2- Webdriver provides support to call JavaScript.
10.3- You just need to add the below lines to your project.
JavascriptExecutor JS = (JavascriptExecutor) driver; JS.executeScript("alert('hello world')");
Tip-11: Getting the result of Javascript code in Webdriver.
11.1- It’s a bit tricky but easy. Use the <return> keyword in JavaScript to send back the result.
11.2- Place the following code in your project.
((JavascriptExecutor) driver).executeScript("return 10");
Tip-12: Perform the mouseover function using WebDriver in Java.
12.1- Sometimes you need to use a mouseover function with a drop-down menu.
12.3- When you hover over the menu, it shows new options.
12.5- Webdriver provides a <Actions> class to handle mouse events.
12.4- We can achieve this by chaining all actions to simulate mouse hover.
Actions action = new Actions(driver); WebElement item = driver.findElement(By.xpath("html/body/div[5]/ul/li[5]/a")); action.moveToElement(item).moveToElement(driver.findElement(By.xpath("/write-expression-here"))).click().build().perform();
Tip-13: Make Selenium WebDriver click a checkbox that is not currently visible.
13.1- Sometimes you’ve to click checkboxes on a form. But you fall into problems when they get added dynamically.
13.2- If you use Webdriver calls, then the following error occurs.
"Element is not currently visible and so may not be interacted with."
13.3- In such cases, we advise using Webdriver’s JavaScript executor to do the job.
13.4- Check out the below lines of code.
((JavascriptExecutor)driver).executeScript("arguments[0].checked = true;", checkbox);
Tip-14: Switch to a new browser window in Webdriver.
14.1- You can easily switch between popup windows.
14.2- Copy the below code in your project to switch windows.
// Get the current window handle. String hBefore = driver.getWindowHandle(); // Click to open new windows. // Switch to new windows. for(String hNew: driver.getWindowHandles()){ driver.switchTo().window(hNew); } // Close all new windows. driver.close(); // Switch back to first window. driver.switchTo().window(hBefore); // Resume your work.
Tip-15: Setting the driver executable path in Webdriver.
15.1- For some browsers, Webdriver needs to know the locations of their driver files. e.g. Chrome, and IE.
15.2- We need to set the driver file path in the Webdriver configuration.
15.3- See the code snippet given below. We took IE as an example.
File ieDriver = new File("path/to/iexploredriver.exe"); System.setProperty("webdriver.ie.driver", ieDriver.getAbsolutePath()); WebDriver driver = new InternetExplorerDriver();
Tip-16: Find the element using CSS in Webdriver.
16.1- While working with list items, you may find it interesting to use CSS for locating elements. Let’s learn in-depth with the help of examples.
16.2- Use case: You’ve to find the third element from a list. The element is an anchor text and uses <css1> and <css2> classes for styling.
16.3- Implement with <By.CssSelector>.
IList<IWebElement> list = driver.FindElements(By.CssSelector(".css1.css2")); IWebElement target = list[3];
16.4- Implement with <By.XPath>.
driver.FindElement(By.XPath("(//a[contains(@class, 'css1 css2')])[3]"));
Tip-17: Open a new tab using Selenium WebDriver in Java.
17.1- At times you may need to open a new tab in the same browser window. It’s easy to do it, use the following code.
driver.findElement(By.cssSelector("body")).sendKeys(Keys.CONTROL +"t");
17.2- If you wish to run your tests in the new tab, then you’ve to switch to it first. Check out the given code fragment.
ArrayList<String> tablist = new ArrayList<String> (driver.getWindowHandles()); driver.switchTo().window(tablist.get(0));
Now let’s hear some of the premium Selenium Webdriver coding tips that we’ve already published on our blog.
Tip-18: Handle Ajax calls in Selenium Webdriver.
18.1- Read the below post to see 6 unique ways to handle Ajax calls.
Tip-19: Scheduling Selenium Webdriver tests in Windows 7.
19.1- Read the below post to set up Selenium Webdriver testing using the Windows task scheduler.
Tip-20: Scheduling Selenium Webdriver tests in Linux.
20.1- Read the below post to run Selenium Webdriver tests using crontab in Linux.
Footnote – Selenium Webdriver Coding Tips
We wish the above post would have been able to hit the right chord with you. We’ve tried hard to touch upon all basic, advanced, and premium Selenium Webdriver coding tips.
We believe these quickies would not only solve your problems but also help in upgrading your Selenium Webdriver coding skills. Our end goal is to see you outshining in your work. That’s why we keep researching new ways to use and innovate using Selenium Webdriver, Java, and Python.
So, help us reach this post further to benefit every other individual who’s striving to learn automation. Don’t just leave without a like, share, or comment. 🙂
Best,
TechBeamers.