This Python tutorial explains how to create multiline strings and use them more cleanly. This can be handy when managing a long string in your program. It is cumbersome to keep such a lengthy text in a single line. It kills the readability of your code.
Simple steps to use multi-line text in Python
In Python, you have different ways to create a multiline text or string. You can split the string across multiple lines by enclosing it in triple quotes. Alternatively, you can use brackets to let the string span onto different lines.
By the way, backslash works as a line continuation character in Python. You can even employ it to join text on separate lines and create a multiline string. Python also provides the string join() function which can also be utilized to define a string containing newlines.
How to create multiline strings in Python
Let’s now discuss each of these options in detail. We have also provided examples with descriptions of every method.
Use triple quotes to create a multiline string
It is the most generic method in Python that lets a long string spill over multiple lines. You just need to enclose the text with a pair of Triple quotes, one at the start and the second at the end. Its usage is shown in the picture and also in the code block next.
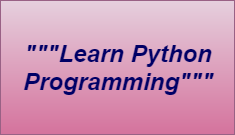
"""Learn Python Programming"""
Anything inside the enclosing Triple quotes will become part of one multiline string. Let’s have an example to illustrate this behavior.
# String containing newline characters line_str = "I'm learning Python.\nI refer to TechBeamers.com tutorials.\nIt is the most popular site for Python programmers."
Next, we’ll try to slice it into multiple lines using triple quotes.
# String containing newline characters line_str = "I'm learning Python.\nI refer to TechBeamers.com tutorials.\nIt is the most popular site for Python programmers." print("Long string with newlines: \n" + line_str) # Creating a multiline string multiline_str = """I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.""" print("Multiline string: \n" + multiline_str)
After running the above, the output is:
Long string with newlines: I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers. Multiline string: I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.
This method retains the newline ‘\n’ in the generated string. If you want to remove the ‘\n’, use the strip()/replace() function.
Use brackets to define a multiline string
Another simple technique is to enclose the slices of a string over multiple lines using brackets. Check the usage from the image below and in the code example following next.
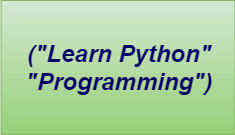
See the below example to know how to use it:
# Python multiline string example using brackets multiline_str = ("I'm learning Python. " "I refer to TechBeamers.com tutorials. " "It is the most popular site for Python programmers.") print(multiline_str)
After you run the above code, it prints the multiline line Python string as shown below.
I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.
You can see there is no newline character in the output. However, if you want it, add it while creating the string.
# Python multiline string with newlines example using brackets multiline_str = ("I'm learning Python.\n" "I refer to TechBeamers.com tutorials.\n" "It is the most popular site for Python programmers.") print(multiline_str)
Here is the output after execution:
I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.
Please note that the PEP 8 guide recommends using brackets to create a Python multiline string.
Learning Python strings is simple and interesting but hard to contain in just one tutorial. So, search and expand your reach to other related topics.
Backslash to join string on multiple lines
It is a less preferred way to use a backslash for line continuation. However, it certainly works and can join strings on various lines. The image below shows how to create the string going over multiple lines.
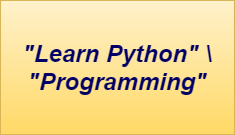
Check out the below code:
# Python multiline string example using backslash multiline_str = "I'm learning Python. " \ "I refer to TechBeamers.com tutorials. " \ "It is the most popular site for Python programmers." print(multiline_str)
The above Python code prints the multiline string in the following manner:
I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.
You can observe that the output isn’t showing any newlines. However, you may like to add some by yourself.
# Python multiline string example using backslash and newlines multiline_str = "I'm learning Python.\n" \ "I refer to TechBeamers.com tutorials.\n" \ "It is the most popular site for Python programmers." print(multiline_str)
The output:
I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.
Python Join() method to create a string with newlines
The final approach is applying the string join() function to convert a string into a multiline Python string. It handles the space characters by itself while contaminating the strings.
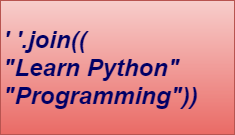
See the below sample code using the Python join() and without the newline separator.
# Python multiline string example using string join() multiline_str = ' '.join(("I'm learning Python.", "I refer to TechBeamers.com tutorials.", "It is the most popular site for Python programmers.")) print(multiline_str)
It outputs the following result:
I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.
Check one more piece of Python, in this, we have a newline character appended to each line to make it a multiline string.
# Python multiline string with newlines example using string join() multiline_str = ''.join(("I'm learning Python.\n", "I refer to TechBeamers.com tutorials.\n", "It is the most popular site for Python programmers.")) print(multiline_str)
And, when you run the above code, it prints each sentence in a separate line.
I'm learning Python. I refer to TechBeamers.com tutorials. It is the most popular site for Python programmers.
Bookmark our tutorial on string formatting methods in Python, if you want to master string manipulation.
How to pass arguments to multiline strings
In Python, you can create multiline strings with arguments by using triple-quoted strings and incorporating placeholders for the arguments. Placeholders are typically filled using string formatting techniques like f-strings, string.format()
or %
formatting. Here’s how to declare a multiline string with arguments:
Using f-strings (Python 3.6 and later)
F-strings are a new and concise way to format strings in Python. You can read about it in detail from our blog. It makes it very easy to supply arguments in a multiline string.
For example, the following code declares a multiline string with two arguments:
variable1 = "World" variable2 = 42 multiline_string = f""" Hello, {variable1}! I am {variable2} years old. """ print(multiline_string)
Using string.format()
Another way to dynamically fill variables in multiline strings is by using the Python string format() method. In this, we pass the arguments to the format() function which fills in the placeholders at runtime. To know how this works, check out the below example.
variable1 = "World" variable2 = 42 multiline_string = """ Hello, {}! I am {} years old. """.format(variable1, variable2) print(multiline_string)
Using %
to supply arguments in a string
Last but not least, check the old way of filling variables in a multiline string which is the percent (%) operator. For example, the below Python code passes two arguments, one is a string (%s) and another is a number (%d).
variable1 = "World" variable2 = 42 multiline_string = """ Hello, %s! I am %d years old. """ % (variable1, variable2) print(multiline_string)
How to search a multiline string in a text file
Sometimes, you might have to find a multiline string in a text file and the line number at which the string exists. So, to understand this, let’s first define our problem statement.
Problem statement and test data
Search a text file for a multiline string and return the line number in Python.
Sample data:
sample_file.txt:
This is the first line of the text file.
This is the second line.
apple.
mango.
grapse.
banana.
And here's the last line.
Mutltiline string to find:
mango.
grapse.
Program to search a multi-line string
The following Python code can be used to search a text file for a multiline string and return the line number. Please note that it not only searches the text but also creates the text file. So, it is fully working code that you can straightway use and run.
def search_in_txt(txt_file, multiline_str): """Searches a text file for a multiline string and returns the line number. Args: txt_file: The path to the text file. multiline_str: The multiline string to search for. Returns: The line number of the first line of the multiline string, or None if the multiline string is not found in the text file. """ with open(txt_file, "r") as txt: lines = txt.readlines() multiline_str_lines = multiline_str.strip().split('\n') for line_num, line in enumerate(lines): if multiline_str_lines[0] in line: found = True for j in range(1, len(multiline_str_lines)): if line_num + j >= len(lines) or multiline_str_lines[j] not in lines[line_num + j]: found = False break if found: return line_num + 1 return None # Example usage: txt_file = "sample_file.txt" file_content = """This is the first line of the text file. This is the second line. apple. mango. grapse. banana. And here's the last line. """ # Create and write the content to the text file with open(txt_file, "w") as file: file.write(file_content) with open(txt_file, "r") as file: lines = file.readlines() # Print the content of the text file with line numbers print("++++++++++++++++++++") for line_num, line in enumerate(lines, start=1): print(f"Line {line_num}: {line.strip()}") print("++++++++++++++++++++\n") multiline_str = """ mango. grapse. """ line_num = search_in_txt(txt_file, multiline_str) if line_num is not None: print("The multiline string was found on line {}".format(line_num)) else: print("The multiline string was not found in the text file.")
Once you run the above code, it automatically creates a text file with the sample data. Before searching, it also prints the file content so that you can verify the file content. After that, it searches for the Python multiline string in the text file and prints its line number.
++++++++++++++++++++ Line 1: This is the first line of the text file. Line 2: This is the second line. Line 3: apple. Line 4: mango. Line 5: grapse. Line 6: banana. Line 7: And here's the last line. ++++++++++++++++++++ The multiline string was found on line 4
We suggest you not only run the above code but also make more changes to it and sample data. Running it with different data sets will help you sustain the learning.
Before You Leave
Today, you learned how to create and use multiline strings in Python programs. However, it is important to practice with the examples we provided, then only you can become comfortable in using them.
To get an extra dose of practice, you can always refer to our 40 Python exercises for beginners. With these exercises, you can span your learning to different areas of Python.
Lastly, our site needs your support to remain free. So, share this post on social media (Linkedin/Twitter) if you gained some knowledge from this tutorial.
To learn Python from scratch to depth, read our step-by-step Python tutorial.
Enjoy coding,
TechBeamers.