String formatting is a basic concept in Python that allows you to modify and display text with ease. For this purpose, Python provides a string format() method which is a versatile and powerful tool for string formatting. In this tutorial, we will delve into this method, and its features, and learn how to use it with examples.
Python String Format() Method
Python’sstr.format()
method is a newer and more flexible way to format strings in Python. It has been available since Python 3.1 and is now the preferred method for string formatting.
To format a string using the str.format()
method, you pass it a format string and a set of keyword arguments. The format string contains placeholders for the values, which are replaced when the string formatting happens.
The placeholders in the format string are marked with curly braces ({ }), Inside the curly braces, you can specify the name of the keyword argument that contains the value to replace the placeholder.
Str.Format() Syntax
The basic syntax of the method str.format()
is as follows:
formatted_string = "Place any text here: {}".format(value)
In this syntax:
"Place any text here: {}"
is the string template where{}
serves as a placeholder for values.format()
is the method that formats the string.value
is the actual value that replaces the placeholder.
You can have multiple placeholders within a single string, and you have flexibility in how you provide values to replace them.
Parameters of str.format()
Let’s find out the important parameters used in the str.format()
method:
Parameter | Description |
---|---|
{} (Curly Braces) | Placeholders within the string that specify where values should be inserted. Multiple placeholders can be used within a single string. |
format() method | The method used to format the string. It should be called on the string containing placeholders. |
value | The value that replaces a placeholder. It can be a variable, literal, expression, or any valid Python object. |
Positional Arguments | Values provided to the format() method in the order they should replace placeholders. For example: "{} {}".format(value1, value2) . |
Named Arguments | Values passed in the format() method using names for placeholders. For example: "Name: {name}, Age: {age}".format(name="Alex", age=30) . |
Format Specifiers | Optional formatting instructions that follow the placeholders and define how the values should be displayed. For example: "{:.2f}".format(3.14159265359) specifies formatting a floating-point number with two decimal places. |
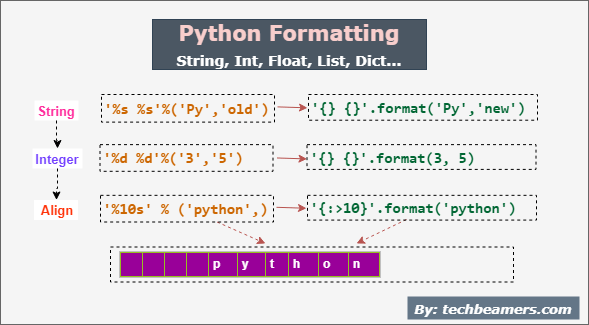
Using Python String Format() for Strings
You can invoke the format function in many of the following ways.
Basic String Formatting
Here’s an example to illustrate how these parameters work together:
name = "Alex" age = 30 height = 5.8 outstr = "Name: {}, Age: {}, Height: {:.2f}".format(name, age, height) print(outstr)
In this example:
{}
are placeholders within the string.format()
will format the string.name
,age
, andheight
are values that replace the placeholders."{:.2f}"
is a format specifier that formats theheight
variable as a floating-point number with two decimal places.
The output will be: Name: Alex, Age: 30, Height: 5.80
Padding and Align Strings
Padding and aligning strings is a common requirement when formatting text, and the str.format()
method provides easy ways to achieve this. Here’s a detailed example of how to pad and align strings using str.format()
:
# Define some example data item1 = "Apple" item2 = "Banana" item3 = "Cherry" # Create a template with placeholders and alignment formatted_string = "{:<10} | {:^10} | {:>10}".format(item1, item2, item3) # Print the formatted string print(formatted_string)
In this example:
item1
,item2
, anditem3
are three strings representing items.- The template string
"{:<10} | {:^10} | {:>10}"
defines the format of the output. It contains three placeholders separated by vertical bars (|
), each with a different alignment and width:"{:<10}"
aligns the text to the left (<
) and specifies a width of 10 characters. This meansitem1
(Apple) will be left-aligned within a 10-character-wide field."{:^10}"
aligns the text to the center (^
) and specifies a width of 10 characters. This centersitem2
(Banana) within a 10-character-wide field."{:>10}"
aligns the text to the right (>
) and specifies a width of 10 characters. This right-alignsitem3
(Cherry) within a 10-character-wide field.
When you run this code, the output will be:
Apple | Banana | Cherry
Also Read: Different Python Methods for String Formatting
Justify a variable string expansion
In the below example, we demonstrate the variable string expansion and justify it.
# Define a list of items with varying lengths items = ["Apple", "Banana", "Cherry", "Grapes", "Strawberry"] # Create a template with a placeholder for variable content and right alignment template = "{:>12}" # Print a header print("Fruit Names:") print("-" * 20) # Iterate through the items and format each one with right alignment for item in items: formatted_item = template.format(item) print(formatted_item)
In this code:
- We have a list of items which have different lengths.
- The template string
"{:>12}"
defines the format for each item. It contains a single placeholder that specifies the right alignment (>
) with a width of 12 characters. The width ensures equal spacing, even for shorter strings. - We print a header and a line below it for clarity.
When you run this code, the output will be:
Fruit Names: -------------------- Apple Banana Cherry Grapes Strawberry
Using Python String Format for Integers
There are many ways to format an Integer, let’s see the basic usage first.
print("I've <{}> years of experience and my salary is <{}> USD per annum.".format(10, 75000)) #I've <10> years of experience and my salary is <75000> USD per annum.
Separator Number Formatting
It is a standard convention to display the salary with commas. Python string format function supports this representation and requires a pair of ‘:’ and ‘,’ inside the parenthesis.
print("I've <{}> years of experience and my salary is <{:,}> USD per annum.".format(10, 75000)) #I've <10> years of experience and my salary is <75,000> USD per annum.
Specify Field Width for Numbers
The same as we did for strings is applicable for integers. For integers, we can’t use precision.
print("I've <{:5}> years of experience and my salary is <{:15,}> USD per annum.".format(10, 75000)) #I've < 10> years of experience and my salary is < 75,000> USD per annum.
Padding for Numbers
While specifying the width for integers, you can use a character for padding the space left vacant after printing the subject.
print("I've <{:#>8}> years of experience and my salary is <{:z>20,}> USD per annum.".format(10, 75000)) #I've <######10> years of experience and my salary is <zzzzzzzzzzzzzz75,000> USD per annum.
Format a Number as Binary
Python format function allows printing a number in binary style. The symbol ‘b’ after the colon inside the parenthesis notifies to display a number in binary format.
print('{0:b}'.format(10)) #1010
Format a Number in Octal Style
Python format function allows printing an integer in the octal style. The symbol ‘o’ after the colon inside the parenthesis notifies to display a number in octal format.
print('{0:o}'.format(10)) #12
Format a Number as Hex
Python format function allows printing an integer in the hex style. The symbol ‘x’ or ‘X’ after the colon inside the parenthesis notifies to display a number in hex format.
print('{0:x}'.format(10)) #a >>> print('{0:X}'.format(10)) #A
Using Python String Format for Floats
A float data type also has a couple of variations which you can style using the Python format function.
Simple Example
Let’s print a full floating point number.
print("{0:f}".format(1.123456)) #1.123456
Now, let’s print a floating point number truncating after three decimal points.
print("{0:.3f}".format(1.123456)) #1.123
Finally, let’s print a floating point number that truncates after three decimal places but does round the final value.
print("{0:.3f}".format(1.123556)) #1.124
Fixed Point
print('Fixed-point example: <{0:f}>'.format(2.2183)) #Fixed-point example: <2.218300> print('Fixed-point with right alignment example: <{0:25f}>'.format(2.2183)) #Fixed-point with right alignment example: < 2.218300> print('Fixed-point with precision and right alignment example: <{0:<25.10f}>'.format(2.2183)) #Fixed-point with precision and right alignment example: <2.2183000000 >
General
print('General format example: <{0:g}>'.format(2.2183)) #General format example: <2.2183> print('General format with right alignment example: <{0:25g}>'.format(2.2183)) #General format with right alignment example: < 2.2183> print('General format with precision and center alignment example: <{0:^25.10g}>'.format(2.2183)) #General format with precision and center alignment example: < 2.2183 >
Scientific
print('Scientific format example: <{0:e}>'.format(2.2183)) #Scientific format example: <2.218300e+00> print('Scientific format with left alignment example: <{0:<25e}>'.format(2.2183)) #Scientific format with left alignment example: <2.218300e+00 > print('General format with precision and right alignment example: <{0:>25.5e}>'.format(2.2183)) #General format with precision and right alignment example: < 2.21830e+00>
Format Lists and Dictionaries
Formatting List in Python
The Python string format method accepts a sequence of positional parameters. If we pass an array or a List, then let’s find out the result.
langs = ['C', 'C++', 'CSharp'] print('Skillset: {}'.format(langs)) #Skillset: ['C', 'C++', 'CSharp']
The whole list is displayed. You can also decide to print one item from the sequence by providing its index.
print('Skillset: {0[1]}'.format(langs)) #Skillset: C++
You can even send the list item as the positional parameters. To achieve this, unpack them using the * operator.
print('Skillset: {}'.format(*langs)) #Skillset: C
Formatting Dict in Python
Format function allows using a dictionary as a parameter. See the below example.
print(" Jake's salary is {0[jake]} \n Anand's salary is {0[anand]}".format({'jake': '$100K', 'anand': '$120K'})) #Jake's salary is $100K #Anand's salary is $120K
You can format dictionary data using the keyworded arguments.
print(" Jake's salary is {sal[jake]} \n Anand's salary is {sal[anand]}".format(sal={'jake': '$100K', 'anand': '$120K'})) #Jake's salary is $100K #Anand's salary is $120K
There can be more complex usages of the Python format function which you can face in your work environments. Please do let us know if you like to add one of them here.
To Learn Python from Scratch, Read the Python tutorial.