In this short tutorial, we’ll find out different ways to find the length of a list in Python. Go through each of these methods and practice with the code provided. Out of these, Python len() is the most convenient method to determine the list length.
As programmers, we often deal with various tasks such as storing a number of items or printing them one by one. The program may also require us to print all the items from the list or find the sum of the values in the list. In order to do all of this, we must know how to find the length of a list in Python.
Different Ways to Find the List Length in Python
We have brought 10 different ways that you can reuse in your code to determine the length of a list in Python. Explore each and every method given below. Also, expand your knowledge with the techniques used in the examples.
However, start with this simple algorithm that determines the list length in a step-by-step manner. It is a good starting point before delving into the 10 methods.
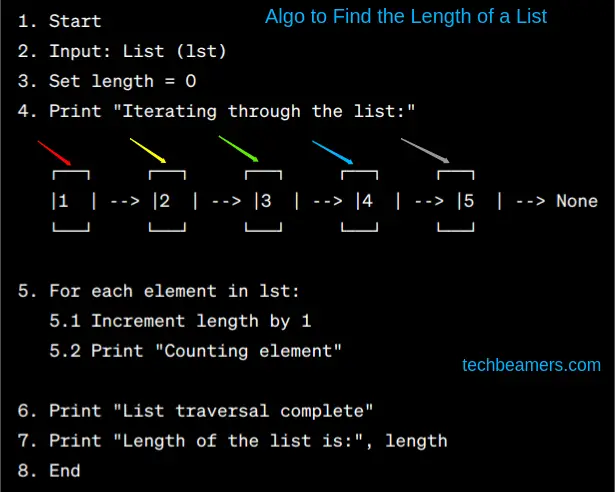
Great, now, check out the 10 unique methods to calculate the length of a list in Python with fully working code examples:
1. Using the len() function to get the length of the list in Python
The len() function is the most straightforward and efficient way to determine the length of a list in Python. It takes a single argument, which is the list whose length you want to find. The function returns an integer representing the number of elements in the list.
numbers = [1, 2, 3, 4, 5]
list_length = len(numbers)
print(list_length)
Output:
5
2. Using a for loop to determine the list length in Python
You can also calculate the length of a list using a for loop. The loop iterates through each element in the list, and a counter is incremented for each iteration. The final value of the counter represents the list length.
numbers = [1, 2, 3, 4, 5]
list_length = 0
for _ in numbers:
list_length += 1
print(list_length)
Output:
5
3. Using enumerate() to find the length of a list in Python
The enumerate() function returns two values for each element in a list: the index of the element and the element itself. You can use this function to iterate through the list and increment a counter to determine the list length.
numbers = [1, 2, 3, 4, 5]
list_length = 0
for index, _ in enumerate(numbers):
list_length = index + 1
print(list_length)
Output:
5
4. Using sum() to return the list length
The sum()
function can be used to calculate the length of a list by summing the boolean values of each element. Since the boolean value of an element is 1 if it exists and 0 if it doesn’t, the sum of these values will be equal to the list length.
numbers = [1, 2, 3, 4, 5]
list_length = sum(bool(x) for x in numbers)
print(list_length)
Output:
5
5. Using a list comprehension
List comprehensions provide a concise way to create lists based on a specific condition. You can use a list comprehension to create a list of boolean values, each corresponding to an element in the original list. The length of the original list can then be determined as the length of the boolean list.
numbers = [1, 2, 3, 4, 5]
list_length = len([bool(x) for x in numbers])
print(list_length)
Output:
5
6. Using the reduce() function
The reduce()
function applies a binary function repeatedly to a list, accumulating the results into a single value. You can use the reduce()
function to determine the length of a list by combining the boolean values of each element using the or
operator.
# Example 8: Using reduce() from the functools module
from functools import reduce
my_list = [1, 2, 3, 4, 5]
# Use reduce() with a lambda function to accumulate the count of elements
length = reduce(lambda acc, _: acc + 1, my_list, 0)
# Print the result
print(f"The length of the list is: {length}")
Output:
5
7. Using pandas to calculate the length of a list in Python
The pandas
module provides a collection of functions for efficient iteration over data structures. You can use the series()
function from the pandas
module and then get the size
attribute to determine the total length of the list.
# Example 7: Using pandas library
import pandas as pd
my_list = [1, 2, 3, 4, 5]
# Create a pandas Series and use .size to get the length of the list
length = pd.Series(my_list).size
# Print the result
print(f"The length of the list is: {length}")
Output:
6
8. Using the map() function
The map()
function applies a function to each element in a list, producing a new list of transformed values. You can use the map()
function to convert each element in the list to a boolean value and then use the len()
function to determine the length of the resulting list of boolean values.
numbers = [1, 2, 3, 4, 5]
list_length = len(list(map(bool, numbers)))
print(list_length)
Output:
5
Sure, here are the remaining methods for calculating the length of a list in Python with fully working code examples:
9. Using the filter() function to find the length of a list in Python
The filter() function takes a function and an iterable as arguments and returns a new iterator that contains elements from the original iterable for which the function evaluates to True. You can use the filter()
function to filter the list to only contain boolean values, and then use the len()
function to determine the length of the filtered list.
numbers = [1, 2, 3, 4, 5]
list_length = len(list(filter(bool, numbers)))
print(list_length)
Output:
5
10. Using a generator expression
Generator expressions offer an alternative approach to creating a list from an iterable. You can use a generator expression to create a list of boolean values, each corresponding to an element in the original list. The length of the original list can then be determined as the length of the generator expression.
numbers = [1, 2, 3, 4, 5]
list_length = len([bool(x) for x in numbers])
print(list_length)
Output:
5
These are 10 different methods for calculating the length of a list in Python, each with its own nuances and applications. The choice of method depends on personal preference, specific context, and the desired level of efficiency. For most common scenarios, the len()
function is the most straightforward and efficient option.