This tutorial brings you the best 20 Python programs to print patterns like a square, triangle, diamond, alphabet, and Pascal triangle using stars, letters, and numbers. If you are a beginner to programming, then practicing these programs to print patterns is the fastest way to learn Python.
Python is popular because of its simplicity and versatility, making it an excellent choice for those taking their first steps in coding. To get you started, here’s a collection of beginner-friendly Python pattern programs. These star patterns are not only fun to create but also serve as a practical way to understand the basics of loops and logic in Python. From classic shapes like squares and triangles to more complex designs such as Pascal’s Triangle and letter patterns, this set has something for everyone. Let’s dive in and enjoy the art of coding through patterns!
20 Best Python Programs to Print Patterns with Full Code
In programming, a star pattern refers to a design or shape created by using asterisk (*) characters. Star patterns are a common exercise for beginners to practice control structures like loops and conditional statements like if-else in Python.
A star pattern typically consists of rows and columns of asterisks, with different arrangements to form various shapes or designs. Such a pattern can be symmetrical or asymmetrical. It can include patterns like right-angled triangles, squares, rectangles, diamonds, and more.
Star patterns are a fun way to understand the logic of loops and conditional statements while creating visual and artistic designs in the console or on paper.
Must Try: Practice with 40 Python Exercises for Beginners
Print Square and Rectangle Patterns
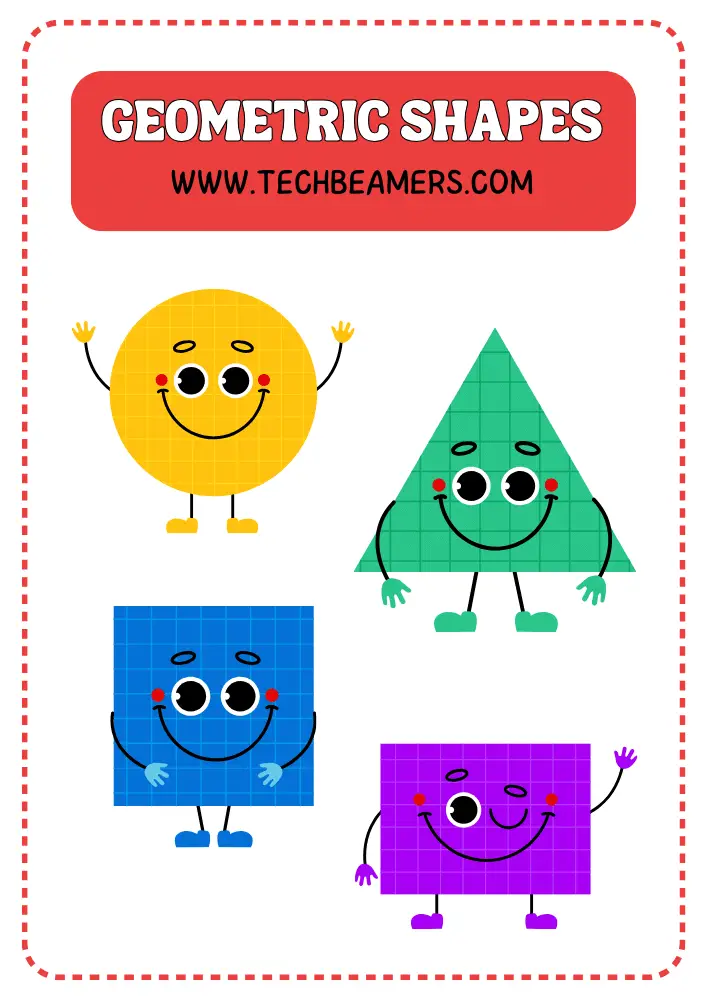
It is about creating designs that look like squares and rectangles using numbers or characters. These patterns help practice coding and create simple, geometric shapes.
- Square pattern
# Define the number of X and Y.
l = 6
# Create a nested for loop to iterate through the X and Y.
for x in range(l):
for y in range(l):
print('*', end='')
print()
- Hollow square pattern
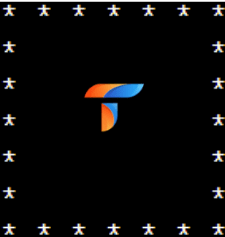
def make_holo_square(l):
if l < 3:
print("Too small size. Provide a larger value.")
return
for x in range(l):
for y in range(l):
if x == 0 or x == l - 1 or y == 0 or y == l - 1:
print("*", end=" ")
else:
print(" ", end=" ")
print()
l = 7 # Adjust the if you wish to.
make_holo_square(l)
- Solid rectangle pattern
def make_sol_rect(p, q):
if p < 1 or q < 1:
print("Both p & q must be +ve numbers.")
return
for x in range(p):
for y in range(q):
print("*", end=" ")
print()
p = 5 # Num Ps
q = 7 # Num Qs
make_sol_rect(p, q)
- Hollow rectangle pattern
p = 6
q = 6
for x in range(p):
if x == 0 or x == p - 1:
print('*' * q)
else:
print('*' + ' ' * (q - 2) + '*')
You can print the same shape using the below one line of code:
w = 6; h = 6
print('\n'.join(['*' * w if i in {0, h - 1} else '*' + ' ' * (w - 2) + '*' for i in range(h)]))
Python Programs to Print Triangle and Pyramid Patterns
Triangle patterns in programming are like stacking numbers or characters in a triangle shape. They are a helpful tool for learning coding. You can have different types of triangles, like ones that go down, ones that go up, and others that have spaces in them. In short, it’s a fun way to practice with triangle patterns. Here, we provide multiple Python programs using very minimal steps to print a triangle and pyramid pattern using for loop.
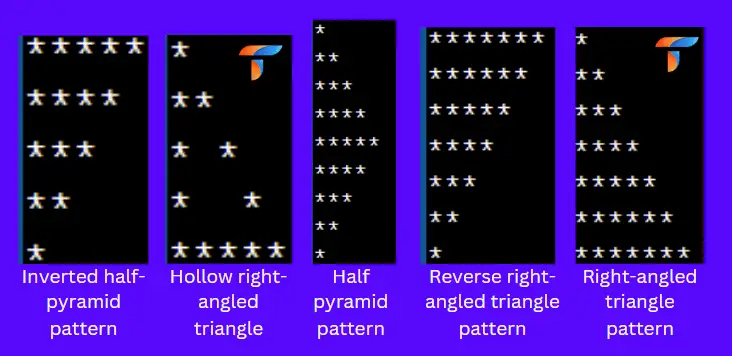
- Right-angled triangle pattern
l = 7
for x in range(1, l + 1):
print('*' * x)
More so, you can even create the same formation with a single line of code using the Python join method.
l = 7
print('\n'.join(['*' * (i + 1) for i in range(l)]))
- Reverse right-angled triangle pattern
l = 7
for x in range(l, 0, -1):
print('*' * x)
Another piece of code to do this is:
l = 7
print('\n'.join(['*' * (l - i) for i in range(l)]))
- Full and Half pyramid patterns
This code will draw a half-pyramid shape.
l = 5
for x in range(1, l + 1):
print('*' * x)
for x in range(l - 1, 0, -1):
print('*' * x)
Here is a one-liner Python instruction to print the full pyramid shape.
l = 7
print('\n'.join([' ' * (l - ix - 1) + '*' * (2 * ix + 1) for ix in range(l)]))
- Inverted half-pyramid pattern
l = 5
for x in range(l, 0, -1):
print('*' * x)
- Hollow right-angled triangle pattern
l = 5
for x in range(l):
if x == l - 1 or x == 0:
print('*' * (x + 1))
else:
print('*' + ' ' * (x - 1) + '*')
We tried to add multiple programs in each category. Here are additional patterns for the next set of categories:
Print Diamond Patterns in Python Using For Loop
Not to mention that diamond patterns in programming are shapes that look like diamonds. They are made by arranging numbers or characters in a specific way. Diamond patterns are often used for learning programming and can be a fun way to practice coding. Here are two examples of printing a diamond pattern in both solid and hollow forms using Python for loop.
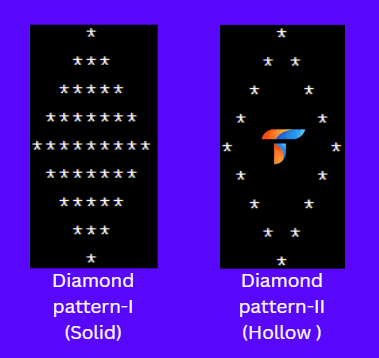
- Diamond pattern-I (Solid)
l = 5
for x in range(1, l + 1):
print(' ' * (l - x) + '*' * (2 * x - 1))
for y in range(l - 1, 0, -1):
print(' ' * (l - y) + '*' * (2 * y - 1))
You can print the same solid diamond shape with the following code:
l = 5
print('\n'.join([' ' * (l - ix - 1) + '*' * (2 * ix + 1) for ix in range(l)] + [' ' * (l - ix - 1) + '*' * (2 * ix + 1) for ix in range(l - 2, -1, -1)]))
- Diamond pattern-II (Hollow )
l = 5
for x in range(1, l + 1):
for y in range(1, l - x + 1):
print(" ", end="")
for y in range(1, 2 * x):
if y == 1 or y == 2 * x - 1:
print("*", end="")
else:
print(" ", end="")
print()
for x in range(l - 1, 0, -1):
for y in range(1, l - x + 1):
print(" ", end="")
for y in range(1, 2 * x):
if y == 1 or y == 2 * x - 1:
print("*", end="")
else:
print(" ", end="")
print()
Number Pattern Programs
In programming, a number pattern refers to a specific arrangement of numbers, often in a geometric or mathematical shape. These patterns make use of loops and conditional statements for dynamic formation.
Number patterns can range from simple to complex and can include various shapes, such as triangles, squares, rectangles, diamonds, and more. It is very common to use them for teaching coding concepts, learning loop structures, and exercising mathematical principles. They can be a fun way to explore and test your Python programming skills.
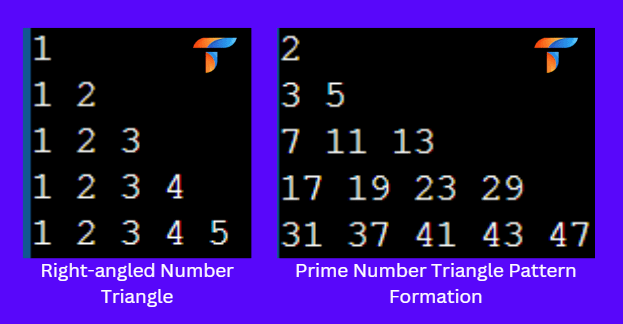
- Right-angled number triangle
l = 5
for x in range(1, l + 1):
for y in range(1, x + 1):
print(y, end=" ")
print()
- Prime Number Pattern
lns = 5 cur_no = 2 # Take 2 as the first num for ln in range(1, lns + 1): for position in range(1, ln + 1): while True: prime_no = True for ix in range(2, int(cur_no ** 0.5) + 1): if cur_no % ix == 0: prime_no = False break if prime_no: break cur_no += 1 print(cur_no, end=" ") cur_no += 1 print()
- Fibonacci Number Pattern
l = 5 a, b = 0, 1 for x in range(l): for y in range(x + 1): print(a, end=" ") a, b = b, a + b print()
Print Pascal’s Triangle in Python Using For Loop
Pascal’s Triangle patterns in programming create a special triangular arrangement of numbers. Nonetheless, creating this pattern is a great way to exercise your mathematical and logical thinking. In this Python program, we made a function using a for loop to print the Pascal triangle.
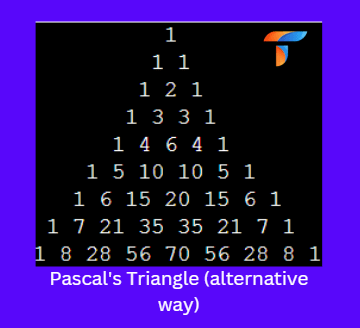
- Pascal’s Triangle (plus an alternative way)
def gene_pasc_tri(l):
tri = []
for ln in range(l):
r = []
for x in range(ln + 1):
if x == 0 or x == ln:
r.append(1)
else:
pr = tri[ln - 1]
r.append(pr[x - 1] + pr[x])
tri.append(r)
max_width = len(" ".join(map(str, tri[-1])))
for r in tri:
print(" ".join(map(str, r)).center(max_width))
gene_pasc_tri(9)
Here is one more code to print this shape using the comb method from math lib. It ensures the shape shows perfectly by double-checking the padding.
from math import comb
l = 7
def show_pascal(l):
w = len(str(comb(l - 1, l // 2))) # Calculate max width for padding
for ix in range(l):
row = ' '.join(str(comb(ix, j)).center(w) for j in range(ix + 1))
print(row.center(l * w + (l - 1)))
show_pascal(l)
Python Programs to Print Arrow Patterns
Arrow pattern programs in programming create shapes that look like arrows. They require arranging characters in a specific way and a nice way to learn coding.
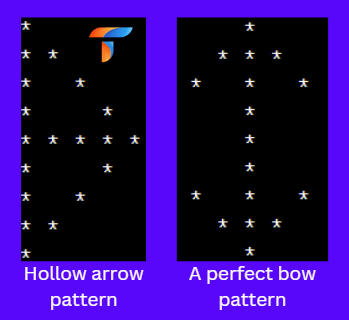
- Hollow arrow pattern
l = 5
for x in range(l):
for y in range(x + 1):
if y == 0 or y == x or x == l - 1:
print('*', end=" ")
else:
print(' ', end=" ")
print()
for x in range(l - 2, -1, -1):
for y in range(x + 1):
if y == 0 or y == x or x == l - 1:
print('*', end=" ")
else:
print(' ', end=" ")
print()
- A perfect bow pattern
l = 7 # Top edge of the bow for x in range(l // 2): for y in range(l): if y == l // 2 or (x + y) == l // 2 or (y - x) == l // 2: print('*', end=" ") else: print(' ', end=" ") print() # Middle of the bow for x in range(l // 2): for y in range(l): if y == l // 2: print('*', end=" ") else: print(' ', end=" ") print() # Bottom side of the bow for x in range(l // 2 - 1, -1, -1): for y in range(l): if y == l // 2 or (x + y) == l // 2 or (y - x) == l // 2: print('*', end=" ") else: print(' ', end=" ") print()
Printing Letter Patterns in Python
Letter pattern programs in programming are designs that use letters of the alphabet. They help learn coding and create artistic shapes with letters.
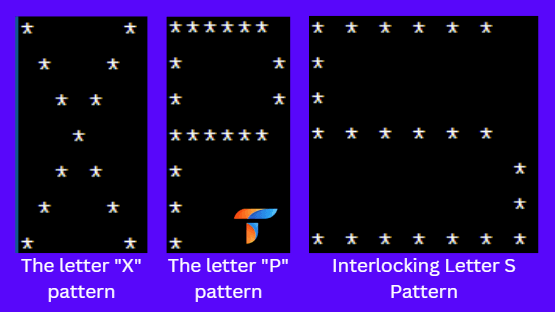
- The letter “X” pattern
l = 7
for x in range(l):
for y in range(l):
if y == x or y == l - x - 1:
print('*', end='')
else:
print(' ', end='')
print()
- The letter “P” pattern
def print_p_pattern(l):
"""Prints a P pattern of length l."""
# Use a nested for loop and traverse the rows as well as columns.
for x in range(l):
for y in range(l):
# Print the P pattern.
if (y == 0 or (x == 0 or x == l // 2) and y > 0 and y < l - 1) or (y == l - 1 and x > 0 and x < l // 2):
print('*', end='')
else:
print(' ', end='')
print()
# Printing a P pattern of length 7.
print_p_pattern(7)
- Interlocking Letter S Pattern
def gen_interlock_s(r):
if r % 2 == 0:
r += 1 # Make sure odd no. of rows for the symm. pattern
for x in range(r):
for y in range(r):
if (x == 0 or x == r - 1 or x == r // 2) and y < r - 1:
print("*", end=" ")
elif (x < r // 2 and y == 0) or (x > r // 2 and y == r - 1):
print("*", end=" ")
else:
print(" ", end=" ")
print()
r = 7 # Alter the no. of rows as you need
gen_interlock_s(r)
I hope these additional patterns are useful.
3 Most Popular Design Pattern Programs to Print Shapes
Since you’ve come this far, it itself shows you have grown up coding skills now. So, this is the right time, we introduce you to the world of design patterns that aim to solve common use cases. For the purpose and scope of this tutorial, we picked the top 3 of them illustrating the printing of a few simple shapes. However, you are free to modify the below codes at will.
In order to write the below programs, we’ve used classes, refer to our post on “Python classes: Everything you need to know” if you are new to using OOPs.
- Design Pattern: Factory Method (for pattern creation)
Description: The Factory Method is a pattern that acts as a blueprint for creating objects. However, it lets subclasses create the kind of objects they need.
Python code:
class PatternFactory:
@staticmethod
def create_pattern(pat_type, l):
if pat_type == 'rt_triangle':
return '\n'.join(['*' * (ix + 1) for ix in range(l)])
# Add more pattern types here
pat = PatternFactory.create_pattern('rt_triangle', 5)
print(pat)
- Design Pattern: Singleton (for pattern printing utility)
Description: The Singleton design pattern guarantees that a class has a sole instance and offers a universal entry point to it.
Python code:
def singleton(cls):
instances = {}
def get_instance(*args, **kwargs):
if cls not in instances:
instances[cls] = cls(*args, **kwargs)
return instances[cls]
return get_instance
@singleton
class PatternPrint:
def print_pat(self, pat):
print(pat)
pr = PatternPrint()
pr.print_pat('\n'.join(['*' * (ix + 1) for ix in range(5)]))
- Design Pattern: Adapter (for adapting different pattern interfaces)
Description: An adapter is a design pattern that allows one class’s interface to work seamlessly with another class’s interface, enabling them to collaborate without any alterations to the original code.
Python code:
class NewPattern:
def create(self, l):
return '\n'.join(['*' * (ix + 1) for ix in range(l)])
class OldPatternAdaper:
def __init__(self, old_pat):
self.old_pat = old_pat
def create(self, n):
return self.old_pat(n)
new_pat = NewPattern()
old_pat = OldPatternAdaper(new_pat.create)
print(old_pat.create(5))
These tips cover a variety of common pattern printing scenarios and demonstrate the use of design patterns in pattern generation and printing where applicable.
Also Check: The Best 30 Python Coding Tips and Tricks
10 One-Liner Tips to Get Better at Printing Patterns
Here are 10 tips that help in coding that a programmer can refer to write better code while printing patterns:
- Use nested loops to control the number of rows and columns in the pattern.
- Use list comprehensions to generate lists of characters to print.
- Call
str.join()
method to concatenate strings to form a pattern. - Try
str.center()
method to center a string within a specified width. - Use the
str.ljust()
andstr.rjust()
methods to justify a string to the left or right, respectively. - Apply the
f-strings
format to embed expressions within strings. - Call the
zip()
function to iterate over multiple lists in parallel. - Use the
enumerate()
function to get the index and value of each element in a list. - Follow the Factory pattern to create different types of patterns based on a given input.
- Use the Decorator pattern to add additional functionality to existing patterns.
A few logical ones
In addition to these general tips, here are some specific tips for printing patterns in Python:
- Call the
print()
function to print each row of the pattern on a new line. - Use whitespace to format the pattern and make it more readable.
- Use variables to store the number of rows and columns in the pattern. This will make your code more amicable and reusable.
- Define functions to encapsulate the logic for printing different types of patterns. This will make your code more modular and easier to maintain.
- Test your code thoroughly to make sure that it is printing the correct patterns.
Conclusion
Congratulations on exploring these Python star patterns for beginners! These fun shapes and designs are like building blocks for your coding journey. As you’ve worked through squares, triangles, and more, you’ve learned the basics of loops and logic. The key to getting better at coding is practice, so keep tinkering with these patterns, create your unique ones, and watch your skills grow. With time, patience, and your creative touch, you’ll continue to unlock the exciting world of Python and coding. Keep coding, keep learning, and enjoy your adventures in the world of programming!