In Python, decision-making is an essential aspect of programming. It allows us to create logic that adapts and responds based on specific conditions. One of the fundamental constructs for decision-making in Python is the If Else statement. In this tutorial, we will explore the If Else statement, along with its variations: If-Elif-Else and Nested If. By understanding these concepts and their syntax, you will gain the ability to write more dynamic and intelligent programs.
Write Python Programs Using If Else and If Elif Else
Before we start, it’s important to know about Python indentation. Indentation helps group statements and shows the flow of control in your code. In Python, we indent the code using either four spaces or a tab. Keeping consistent and proper indentation is vital for readability and functionality. It makes it easier for others to understand your code and ensures that the Python interpreter can interpret it correctly. Let’s now dive in and discover the power of Python If Else statements!
What is a basic if statement in Python?
A basic if statement in Python allows you to run a block of code when a certain condition evaluates to true. It serves as a fundamental construct for controlling the flow of your program based on specific criteria. Here’s the syntax:
if Logical_Expression : Indented Block
Check out the below flowchart that represents the basic if statement in Python.
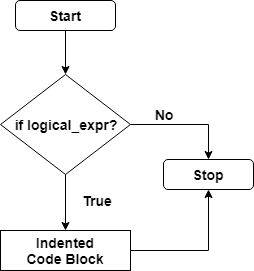
Also Read: Python Switch Case Statement with Examples
Here is an example.
# Simple program to learn the use of Python if statement days = int(input("How many days in a leap year? ")) if days == 366: print("You have cleared the test.") print("Congrats!")
The output of the above code is –
How many days in a leap year? 366 You have cleared the test. Congrats!
How to use Python if else in programs
A Python if else statement allows you to execute different blocks of code based on a condition. If it is True, then the code block following the expression will run. Otherwise, the code indented under the else clause would execute. Check the syntax of Python if Else statement.
if Logical_Expression : Indented Block 1 else : Indented Block 2
This flowchart defines how the basic Python if else statement works.
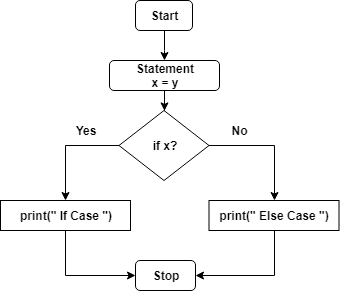
Here is an example showing how to use the if else condition in Python.
# Simple program to practice Python if else condition answer = input("Is Python an interpreted language? Yes or No >> ").lower() if answer == "yes" : print("You have cleared the test.") else : print("You have failed the test.") print("Thanks!")
When you run the above code, it asks for your input. It converts the entered value into lowercase and runs the if else condition. If you enter a ‘yes,’ then the output of the above program would be –
Is Python an interpreted language? Yes or No >> yes You have cleared the test. Thanks!
If you enter a ‘no,’ then the result would be –
Is Python an interpreted language? Yes or No >> no You have failed the test. Thanks!
Python Test: 30 Python Questions on List, Tuple, and Dictionary
What is shorthand Python if else?
Python provides a way to shorten the if/else statement to one line. Let’s see how can you do this.
The shorthand If else has the following syntax:
# If Else in one line - Syntax action_when_true if condition else action_when_false
See the below example of Python If Else in one line.
# Program to check if a number is either even or odd (using shorthand if) num = 21 result = "even" if num % 2 == 0 else "odd" print("The number is", result + ".") # Output: The number is odd.
The above code evaluates the condition num % 2 == 0
: If it is true, we assign the value “even” to the variable result. If the condition is false, we assign the value “odd”. Finally, the program prints “The number is odd.”
Python if-elif-else explained with a detailed example
The if-elif-else statement tests multiple conditions and executes the code block associated with the condition that evaluates to true. Here’s the syntax:
if Logical_Expression_1 : Indented Block 1 elif Logical_Expression_2 : Indented Block 2 elif Logical_Expression_3 : Indented Block 3 ... else : Indented Block N
See the below Python if-elif-else flowchart. It helps you remember the code flow.
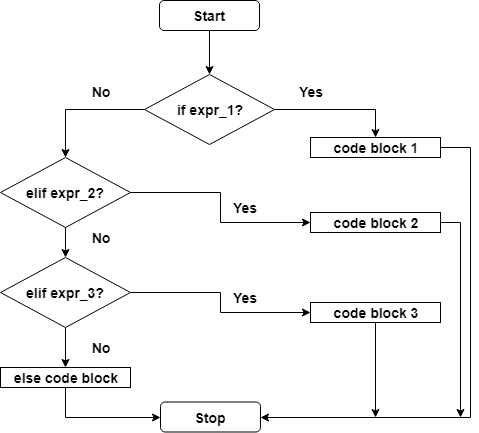
Here we give you a sample program that you can run, modify, and learn by using it.
# Sample program to demonstrate Python if elif else while True: response = input("Which Python data type is an ordered sequence? ").lower() print("You entered:", response) if response == "list" : print("You have cleared the test.") break elif response == "tuple" : print("You have cleared the test.") break else : print("Your input is wrong. Please try again.")
This program has a while loop and asks you to enter the correct Python data type. If you provide a wrong value, it will again prompt you for the input.
Only by entering the correct value, the loop could break. However, you can also press the CTRL+C to exit the program. Had you entered a wrong answer, then the output would be :
Which Python data type is an ordered sequence? dictionary You entered: dictionary Your input is wrong. Please try again. Which Python data type is an ordered sequence?
Once you provide the correct answer, the program will end with the following output.
Which Python data type is an ordered sequence? tuple You entered: tuple You have cleared the test.
What is a nested if-else in Python?
A nested if-else is a Python statement that includes an if-else block within another if-else block. This structure proves useful for handling intricate decision-making scenarios. Check the syntax below:
if Logical_Expression_1 : if Logical_Expression_1.1 : if Logical_Expression_1.1.1 : Indented Block 1.1.1 else : Indented Block else : Indented Block
Go through the below Python nested if example. Execute it, modify, practice, and learn.
# Nested If Else Demo: Check the eligibility of a person for a driver's license based on his age and passing the test age = 17 written_test_passed = True if age >= 18: if written_test_passed: print("Congratulations! You can proceed for a driver's license.") else: print("Sorry, clear the written test first.") else: print("Sorry, your age is less than 18 years.")
In this example, we check the eligibility for a driver’s license based on the person’s age and whether he passed the written test. If the age is 18 or above, and the written test is passed, the person is eligible for a driver’s license. Otherwise, he needs to pass the written test first. If the age is below 18, he must be at least 18 years old to be eligible for a driver’s license.
This example demonstrates how nested if statements help evaluate multiple conditions and provide specific eligibility messages based on those conditions.
How to use the “Not” operator in conditions
The not
is a negation logical operator in Python. It reverses the result of its operand and converts it to a boolean outcome, i.e., True or False. The operand could be a variable or an expression evaluating a numeric type.
Example-1
a = 10 b = 20 if not a > b : print("The number %d is less than %d" %(a, b)) # Output # The number 10 is less than 20
Example-2
X = 0 if not X : print("X is not %d" %(X)) else : print("X is %d" %(X)) # Output # X is not 0
Using the “And” operator in conditions
By using the ‘and’ operator, you can join multiple expressions in a Python if condition. It is also a logical operator that evaluates as True if both/all the operands (x and y and z) are True.
We have created a comprehensive flowchart to help you understand the logic. The code for this flowchart can be found in the accompanying example.
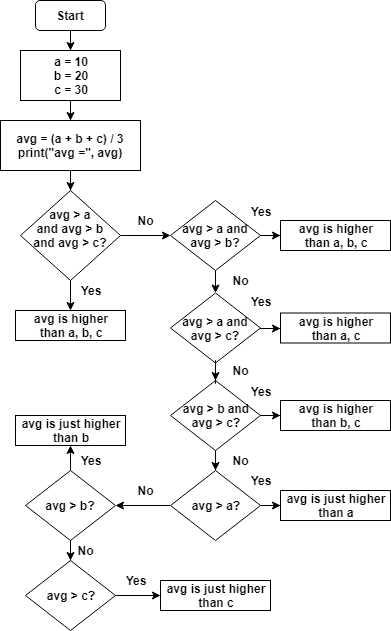
Check out the following example to see the ‘and’ operator in action.
# Program to demonstrate "and" operator with Python If Else a = 10 b = 20 c = 30 avg = (a + b + c) / 3 print("avg =", avg) if avg > a and avg > b and avg > c: print("%d is higher than %d, %d, %d" %(avg, a, b, c)) elif avg > a and avg > b: print("%d is higher than %d, %d" %(avg, a, b)) elif avg > a and avg > c: print("%d is higher than %d, %d" %(avg, a, c)) elif avg > b and avg > c: print("%d is higher than %d, %d" %(avg, b, c)) elif avg > a: print("%d is just higher than %d" %(avg, a)) elif avg > b: print("%d is just higher than %d" %(avg, b)) elif avg > c: print("%d is just higher than %d" %(avg, c))
The output of the above code is –
avg = 20.0 20 is just higher than 10
Using the “in” operator with Python if else
With Python <in>
operator, you can compare a variable against multiple values in a single line. It makes decision-making more comfortable by reducing the use of many if-elif statements.
In Python, we often refer to it as the membership operator. It can let you check values from objects of different types. They could be a list, tuple, string, or dictionary type. Let’s demonstrate it with an example.
In this example, we first create a list of six numbers. After that, there is a for loop which we are using to traverse the list and print values.
The loop has an if statement which prints specific numbers from the list that are not in the tuple used in the condition. Hence, we’ve also used the “not” along with the “in” operator.
#Example of "in" operator with Python If statement num_list = [1, 10, 2, 20, 3, 30] for num in num_list: if not num in (2, 3): print ("Allowed Item:", num)
The output of the above code is as follows.
Allowed Item: 1 Allowed Item: 10 Allowed Item: 20 Allowed Item: 30
Summary
Yes, the software programs can make decisions at runtime. But their correctness depends on how effectively have you added the conditions.
In this tutorial, we covered Python’s If Else, If-Elif-Else, and a couple of its variations using various Python operators.
If you found this tutorial useful, then do share it with your colleagues. Also, connect to our social media accounts to receive timely updates.
Best,
TechBeamers