Are you tired of reading lengthy Python tutorials? Perhaps you are. Then, you must go through the collection of the top ten Python coding tips explained in this post. It’s the result of thorough research and qualitative filtering.
Such quality tips and tricks not only help those who need them for quick interview preparation but solve problems of the ones working on live projects. The reason these tips are so resourceful is that we keep them archiving as keynotes since we started learning Python.
For your information, we’ve thoroughly verified each of the Python coding tips before adding them to this post. And it’s just the first set of tips and tricks that we are sharing today. There will be more such posts for developers, and QA engineers to help with tips.
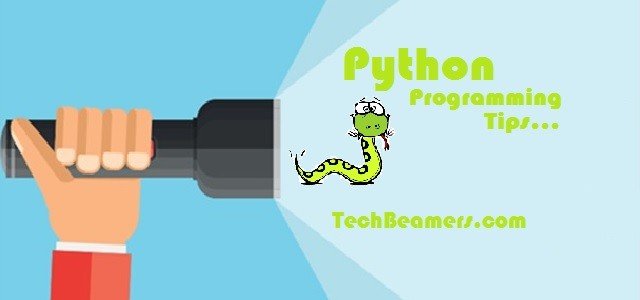
All of our coding tips work on both Python 2.x
and 3.x
versions.
For our readers who are planning for a Python interview, we suggest they read our recently published posts on Python programming which are as follows.
Let’s now dig into the ten essential Python coding tips, especially for testers and Python programming beginners. Even experienced users can find these tips useful.
Python Coding Tips – Essential for Beginners and Experienced
1. Running Python scripts.
On most UNIX systems, you can run Python scripts from the command line in the following manner.
# run python script $ python MyFirstPythonScript.py
2. Running Python programs from a Python interpreter.
The Python interactive interpreter is very easy to use. You can try your first steps in programming and use any Python command. You type the command at the Python console, one by one, and the answer is immediate.
Python console can get started by issuing the command:
# start python console $ python >>> <type commands here>
In this article, all the code starting at the >>> symbol is meant to be given at the Python prompt. It is also important to remember that Python takes tabs very seriously – so if you are receiving any error that mentions tabs, correct the tab spacing.
3. Using enumerate() function.
The enumerate() function adds a counter to an iterable object.
An iterable is an object that has an __iter__ method that returns an iterator. It can accept sequential indexes starting from zero and raises IndexError
when the indexes are no longer valid.
A typical example of the enumerate() function is to loop over a list and keep track of the index. For this, we could use a count variable. But Python gives us a nicer syntax for this using the enumerate() function.
# First prepare a list of strings subjects = ('Python', 'Coding', 'Tips') for i, subject in enumerate(subjects): print(i, subject)
# Output: 0 Python 1 Coding 2 Tips
4. The data type SET.
The data type “set” is a kind of collection. It has been part of Python since version 2.4.
A set contains an unordered collection of unique and immutable objects. It is one of the Python data types which is an implementation of the <sets> from the world of Mathematics.
This fact explains, why the sets unlike lists or tuples can’t have multiple occurrences of the same element.
If you want to create a set, use the built-in set() function with a sequence or another iterable object.
# *** Create a set with strings and perform search in set objects = {"python", "coding", "tips", "for", "beginners"} # Print set. print(objects) print(len(objects)) # Use of "in" keyword. if "tips" in objects: print("These are the best Python coding tips.") # Use of "not in" keyword. if "Java tips" not in objects: print("These are the best Python coding tips not Java tips.")
# ** Output {'python', 'coding', 'tips', 'for', 'beginners'} 5 These are the best Python coding tips. These are the best Python coding tips not Java tips.
# *** Lets initialize an empty set items = set() # Add three strings. items.add("Python") items.add("coding") items.add("tips") print(items)
# ** Output {'Python', 'coding', 'tips'}
5. Dynamic typing.
In Java, C++, and other statically typed languages, you have to specify the data type of the function return value as well as the kind of each function argument. On the other hand, Python is a dynamically typed language. In Python, you don’t explicitly provide the data types. Based on the value you’ve assigned, Python keeps track of the datatype internally. Another good definition of dynamic typing is as follows.
“Names are bound to objects at run-time with the help of assignment statements. And it is possible to attach a name to the objects of different types during the execution of the program.”
The following example demonstrates how a function can examine its arguments. And do different things depending on their types.
# Test for dynamic typing. from types import * def CheckIt (x): if type(x) == IntType: print("You have entered an integer.") else: print("Unable to recognize the input data type.") # Perform dynamic typing test CheckIt(999) # Output: # You have entered an integer. CheckIt("999") # Output: # Unable to recognize the input data type.
6. == and = operators.
Python uses ‘==’ for comparison and ‘=’ for assignment. Python does not support inline assignment. So there’s no chance of accidentally assigning the value when you want to compare it.
7. Conditional Expressions.
Python allows for conditional expressions. Here is an intuitive way of writing conditional statements in Python. Please follow the below example.
# make number always be odd number = count if count % 2 else count - 1 # Call a function if the object is not None. data = data.load() if data is not None else 'Dummy' print("Data collected is ", data)
8. Concatenating strings.
You can use ‘+’ to concatenate strings in the following manner.
# See how to use '+' to concatenate strings. >>> print('Python' + ' Coding' + ' Tips') # Output: Python Coding Tips
9. The __init__ method.
The __init__ method is invoked soon after the object of a class is instantiated. The method is useful to perform any initialization you plan. The __init__ method is analogous to a constructor in C++, C#, or Java.
# Implementing a Python class as InitEmployee.py class Employee(object): def __init__(self, role, salary): self.role = role self.salary = salary def is_contract_emp(self): return self.salary <= 1250 def is_regular_emp(self): return self.salary > 1250 emp = Employee('Tester', 2000) if emp.is_contract_emp(): print("I'm a contract employee.") elif emp.is_regular_emp(): print("I'm a regular employee.") print("Happy reading Python coding tips!")
The output of the above code would look as given below.
[~/src/python $:] python InitEmployee.py I'm a regular employee. Happy reading Python coding tips!
10. Modules.
To keep your programs manageable as they grow, you may want to break them up into several files. Python allows you to put multiple function definitions into a file and use them as a module. You can import these modules into other scripts and programs. These files must have a .py extension.
# 1- Module definition => save file as my_function.py def minmax(a,b): if a <= b: min, max = a, b else: min, max = b, a return min, max
# 2- Module Usage import my_function x,y = my_function.minmax(25, 6.3) print(x) print(y)
Summary: Python Coding Tips – Essential for Beginners and Experienced
We hope all of you would have enjoyed reading the Python coding tips. We at TechBeamers.com always aspire to deliver the best stuff that we can serve to our readers. Your satisfaction is our topmost priority.
In the end, we request you to share this post on all social media platforms of your choice. And please float it into your friend circle as well.
Keep Visiting and Enjoy Reading!!!
TechBeamers