String formatting is the process of constructing a string by inserting values into specific locations. With this purpose in mind, we’ll explore different methods in this quick tutorial. You’ll get to know which method from the League of format() function, percentage (%), f-strings, center(), or template class to use in Python to format strings.
Python String Formatting Different Methods
In this tutorial, we will explore five common string formatting methods: f-strings, the str.format()
method, old-style %
formatting, the string.Template
class, and the str.center()
method. We will also provide a comparison table at the end to help you choose the most suitable method for your specific needs.
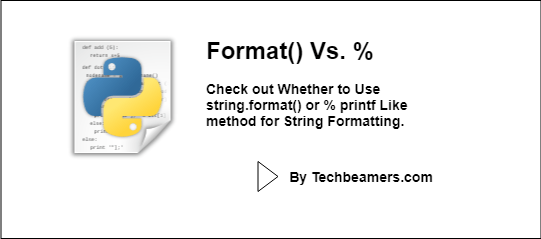
Let’s start with the %
method and then move on to the others.
1. Using Percentage (%) to Format Strings
The %
operator is a legacy Python method for string formatting. It works by replacing placeholders in a string with values from a tuple.
If you just have to do some basic string changes, then the operator ‘%’
is useful. It will evoke similarities with the C programming language. Here is a basic example to illustrate its usage.
name = "Duckworth-Lewis-Stern" age = 1997 formatted_string = "The name method %s was introduced in %d." % (name, age) print(formatted_string) # The Duckworth-Lewis-Stern method was introduced in 1997.
Please note that you need to postfix '%'
with a type-specific char to indicate the type of data to print. It signifies whether to substitute a number or a string in the formatted message.
The following table shows some of the most common conversion specifiers. You can use these commonly with the ‘%’
.
Conversion specifier | Description |
---|---|
s | String |
d | Integer |
f | Floating-point number |
In case you don’t specify the integer type value against the ‘%d’, Python will throw the TypeError. The limitation of such a method is that it restricts the type of parameter input.
Advantages of %
Formatting:
- Suitable for developers coming from a C or C++ background.
- Can be used in Python 2 and Python 3.
Note
Do you want to know how to use the XOR operator in Python? It is a powerful tool for crypto operations such as encryption, generating checksum, and hashing functions.
2. Using Format() Function
The str.format()
method provides a flexible way to format strings by substituting placeholders with values. This method is available in Python 2 and Python 3, making it a good choice for code that needs to be compatible with both versions.
How to use str.format()
In a string, you can define placeholders using curly braces {}
. Then, call the format()
method on the string and provide the values to be inserted as arguments to the format()
method.
city = "New York" population = 8_400_000 average_income = 72_000 formatted_info = "Welcome to {}, where {} people thrive with an average income of ${} per year.".format(city, population, average_income) print(formatted_info)
Once you run the above code, it prints the following:
Welcome to New York, where 8400000 people thrive with an average income of $72000 per year.
In this example, we’re formatting a string to display information about a city, its population, and the average income using the str.format()
method.
Advantages of str.format()
- Provides flexibility in specifying placeholders.
- Compatible with both Python 2 and Python 3.
3. Python F-string for String Formatting
f-strings are the newest method of string formatting in Python. They first surfaced in Python 3.6 and became the most concise and readable way to format strings.
f-strings work by embedding expressions in curly braces ({}), and the expressions are evaluated at runtime and inserted into the string. For example, the following code clarifies how simple it is to use f-string to format strings in Python.
name = "Steve" age = 21 profession = "Software Engineer" print(f"{name} is {age} years old, and he is a {profession}.")
Furthermore, f-strings can help you format multiple values, and even specify the order of insertion. For example, the following code will print the same string as the previous example:
name = "Steve" age = 21 profession = "Software Engineer" print(f"{0} is {1} years old, and he is a {2}.")
Advantages of F-Strings:
- Readable and concise syntax.
- Supports complex expressions within curly braces.
- Available in Python 3.6 and later.
4. string.Template
Class
The string.Template
is available in the Python template module. It provides a safe and extensible way to perform string substitutions. For instance, it can be useful for creating dynamic strings that reoccur frequently, such as generating HTML pages or emails.
In order to use the Template
class, you first need to create a template string. Simultaneously, you have to specify the placeholders using dollar signs ($). For example, the following template string contains two placeholders:
from string import Template name = "Ageless" age = 'constant' template = Template("I am $name and my immortality is a $age.") formatted_string = template.substitute(name=name, age=age) print(formatted_string)
After running the above code, you will get the following output.
I am Ageless and my immortality is a constant.
Advantages of string.Template
- Safe against common security issues, such as code injection.
- Simple and easy to use for basic string substitutions.
5. str.center()
Method
The method str.center()
is used for the purpose of center-aligning a string within a given width. While it’s not a traditional string formatting method, it’s helpful when you need to align text in a specific way.
How to use str.center()
Call the center()
method on a string, passing the desired width as an argument. The method will pad the string with spaces to center it within the specified width.
For example, the following code will center the string “Hello, world!” within a width of 20:
text = "Hello, world!" print(text.center(20))
The above code will print the input text in the center of the given width.
Hello, world!
Advantages of str.center()
- Useful for aligning text in specific formatting scenarios.
- Simplicity and ease of use.
Comparison of Python String Formatting Methods
Now, let’s compare these string formatting methods to help you choose the most suitable one for your needs:
Method | Description | Advantages | Disadvantages |
---|---|---|---|
% operator | The oldest method of string formatting in Python. | Simple and straightforward. | Not very powerful or flexible. |
.format() method | A newer method of string formatting in Python. | More powerful and flexible than the % operator. | Can be complex. |
f-strings | The newest method of string formatting in Python. | Most concise and readable way to format strings. | Not supported in older versions of Python. |
Template class | Allows you to create template strings with placeholders that can be substituted with actual values. | Useful for creating dynamic strings that are used repeatedly. | Can be complex. |
Center method | Centers a string within a given width. | Useful for formatting strings for display. | Only formats a single string. |
Which Method Should You Use?
The best method to use for string formatting depends on your specific needs. If you need to format a simple string, then the %
operator or format()
method is a good option. If you need to format a string that is used repeatedly, then the Template
class is a good option. However, in order to center a string within a given width, the center()
method is a good option.
In summary, if you are using Python 3.6 or higher, then f-strings are the best option for most cases. They are the most concise and readable way to format strings.