From this tutorial, you will learn about different inheritance types available in Java. It will also teach you how to use them in Java programs with the help of code and examples.
Types of Inheritance in Java – A Beginner’s Guide
Before delving into different types of Inheritance in Java, first, let’s briefly understand what inheritance means.
The first thing to remember is that Inheritance is an important OOP concept in Object-Oriented programming. It is the process through which an already existing class extends its features to a new class. Java supports various kinds of inheritance.
Single Inheritance
As the name would suggest, single inheritance is simply one subclass extending one superclass. It is the simplest of all. You can have a look at the general syntax to implement single inheritance below. Here class Parent is the superclass and the Child is the subclass.
class Parent { // methods // fields // …… } class Child extends Parent { // already supports the methods and fields in Parent class // additional features }
The below diagram helps you understand the logical flow of this OOP concept.
Fig – 1
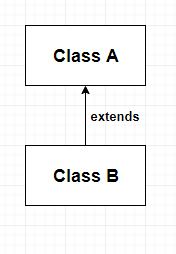
Now, go through the following code. It implements a Calculator class and its subclass AdvancedCalculator.
class Calculator{ int add(int a , int b) { return a+b; } int sub(int a , int b) { return a-b; } } public class AdvancedCalculator extends Calculator { int mult(int a , int b) { return a*b; } int div(int a , int b) { return a/b; } public static void main(String args[]) { AdvancedCalculator cal= new AdvancedCalculator(); System.out.println(cal.add(1,2)); System.out.println(cal.sub(1,2)); System.out.println(cal.mult(1,2)); System.out.println(cal.div(1,2)); } }
The superclass AdvancedCalculator uses already defined add and sub-methods and adds more functionality (product and division). Therefore, the output is:
3 -1 2 0
Multiple Inheritance
Multiple Inheritance is one class extending multiple classes. As discussed before, Java does not support multiple Inheritance. However, with the help of interfaces, we can visualize multiple Inheritance at work.
Conceptually, a visualization for multiple Inheritance would be something like this:
Fig – 2
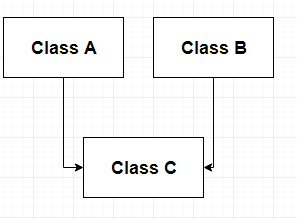
The example below shows multiple Inheritance through interfaces:
interface A { public void dis(int num); } interface B { public void disp(int num); } class Mult implements A, B { public void dis(int num) { System.out.println("From Interface A number is " + num); } public void disp(int num) { System.out.println("From Interface B number is " + num); } } public class Test extends Mult { public static void main(String args[]) { Mult ml = new Mult(); ml.dis(10); ml.disp(20); } }
The output is:
From Interface A number is 10 From Interface B number is 20
Multilevel Inheritance
We call a relationship of Java classes multilevel inheritance in which a superclass extends its features to a subclass. And, the subclass itself acts as a superclass to another class. This means, that once a subclass, will be a future superclass.
Evidently, you can see it from the diagram below. In the picture, a multilevel inheritance relationship is forming like a hierarchy of classes. See the picture below.
Fig – 3
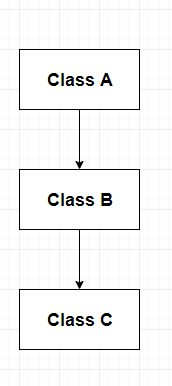
The example below is an example of multilevel inheritance:
class A { public void disA() { System.out.println("ClassA"); } } class B extends A { public void disB() { System.out.println("ClassB"); } } class C extends B { public void disC() { System.out.println("ClassC"); } } public class Test extends C { public static void main(String args[]) { C c = new C(); c.disA(); c.disB(); c.disC(); } }
The output is:
ClassA ClassB ClassC
Hierarchical Inheritance
In hierarchical inheritance, one class acts as a parent class for multiple subclasses. The visual diagram for hierarchical inheritance is given below:
Fig – 4
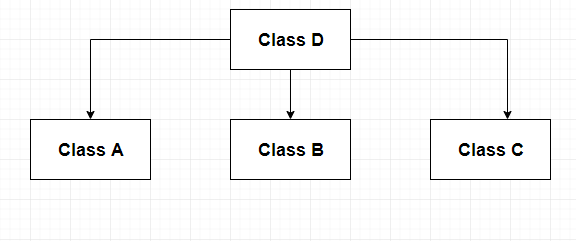
class A { public void disA() { System.out.println("ClassA"); } } class B extends A { public void disB() { System.out.println("ClassB"); } } class C extends A { public void disC() { System.out.println("ClassC"); } } public class Test extends C { public static void main(String args[]) { B b = new B(); b.disB(); b.disA(); C c = new C(); c.disC(); c.disA(); } }
In the example above class A acts as the parent class for all the sub-classes.
The output is :
ClassB ClassA ClassC ClassA
Hybrid Inheritance
Hybrid inheritance is formed as a result of the combination of both single and multiple Inheritance. As multiple Inheritance is not viable with classes, we achieve hybrid inheritance through interfaces.
The visualization for hybrid inheritance is given below:
Fig – 5
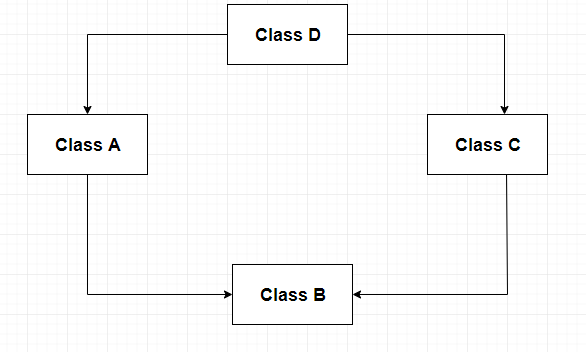
interface B { public void print(); } interface C { public void print(); } class A { public void disA() { System.out.println("ClassA"); } } class D extends A implements B, C { public void print() { System.out.println("method print()"); } public void disD() { System.out.println("ClassD"); } } public class Car extends D{ public static void main(String args[]) { D d = new D(); d.disD(); d.print(); } }
In the example above Class D singly inherits the features of Class A (single inheritance) and at the same time extends both interfaces B and C (Multiple Inheritance)
The output is:
ClassD method print()
Related Posts
Class and Object in Java
Constructor in Java
Interfaces in Java
Inheritance in Java