This tutorial will guide you on what are interfaces in Java. You will know how to create interfaces and use them in Java programs.
Basics of Interfaces in Java
You can go through the following sections to learn about Java interfaces.
Interfaces concept in Java
We can often mislead Interfaces with classes in Java. However, the outlining difference between a class and an interface is that an interface contains abstract methods. A class can implement an interface to provide definition or body to abstract methods while the other way is not possible.
Description:
An interface is just like a reference type in Java. An interface along with the abstract methods contains pre-defined constants and static methods. In OOPS language, an interface defines the behavior a class implements. It is important to realize that the class implementing the interface must define all the methods declared as abstract.
Syntactically, one can find many similarities between an interface and a class. An interface can define as many methods as needed. Much like a class, the name of the Java file for the interface contains the name of the interface. Even, JVM generates its class file in the same pattern.
However, it is equally important to remember that an interface unlike a class does not contain any constructors. Hence, there is no possibility to instantiate it. On the positive side, the interface can let us implement multiple Inheritance which is not possible with classes.
The variables defined through interfaces are always static and final. The methods within an interface are implicitly public. An interface with no methods is called a tagging interface.
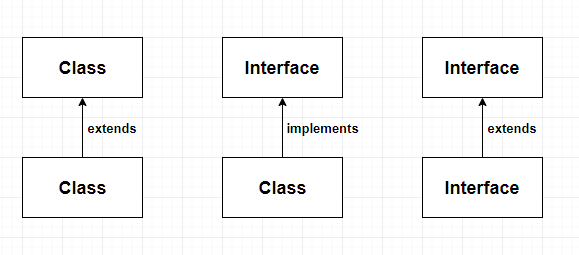
Syntax:
The general syntax to define an interface is much like that of a class. Interfaces and the methods within the interfaces are implicitly abstract, therefore, we do not need to use the abstract keyword.
The keyword used is “interface”:
public interface testInterface { // 1. *** abstract methods // 2. *** static methods // 3. *** static and final variables }
Example demonstrating Interfaces in Java:
In this example, we will explore the concept of interfaces in Java and demonstrate how to define and implement them to achieve better code organization and abstraction.
interface miInterface { public static void disp( ) { System.out.println("Static method in interface"); } public void testPrint( ); } public class jTest implements miInterface { public void testPrint( ) { System.out.println("Defining interface abstract method"); } public static void main(String args[]) { jTest o = new jTest(); o.testPrint(); miInterface.disp(); } }
Therefore, the output is:
Defining interface abstract method Static method in interface
Extend interfaces
Description:
While giving the body to the abstract methods in the class, the signature of the methods should not be changed. A class can implement multiple interfaces at the same time. An interface is extended to other interfaces much like A parent class inherits a child class.
The keyword used is “extends” to make sure the parent interface inherits the functionality of the child interface. The example below deals with multiple Inheritance through interfaces:
Example to demonstrate extending interfaces:
Here, we’ll provide a code example to illustrate how to extend interfaces in a Java program.
interface miInterface1 { static void miMethod1() { System.out.println("Static method 1"); } public void miMethod2(); } interface miInterface2 { static void miMethod2() { System.out.println("Static method 3"); } public void miMethod4(); } interface miInterface extends miInterface1, miInterface2 { static int num = 10; } public class ifTest implements miInterface { public void miMethod2() { System.out.println("Method from interface 1"); } public void miMethod4() { System.out.println("Method from interface 2"); } public static void main(String args[]) { ifTest o = new ifTest(); o.miMethod2(); o.miMethod4(); System.out.println(miInterface.num); } }
The output for the above snippet is:
Method from interface 1 Method from interface 2 10
The thing to keep in mind is that miInterface
does not have access to static methods of its subinterfaces (i.e., miMethod1 and miMethod3).
Related Posts
Class and Object in Java
Constructor in Java
Inheritance in Java
Types of Inheritance in Java