This tutorial will guide you on what is Inheritance in Java. You will know how to use Inheritance for a class and reuse its properties.
Basics of Inheritance in Java
You can go through the following sections to learn about Java Inheritance.
Inheritance concept in Java
Inheritance is one of the most-used features of Object-Oriented Programming. It denotes a methodology through which a newly built class extracts features (methods and variables/fields) from an already existing class.
Description:
The Inheritance represents a special relationship between classes. And, every relationship requires a minimum of two parties.
1. One is the primary class, a.k.a., base class or superclass which has a set of properties and methods.
2. Another is the child class which links up to the base class and inherits almost every feature the parent class offers.
With the implementation of inheritance, information is available in a hierarchical order.
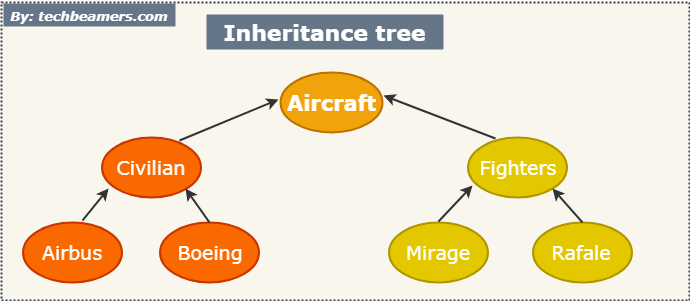
In Java, you can use the extend
keyword to implement the concept of inheritance. The use of inheritance comes into play when the programmer does not wish to define the same set of methods or fields for the new class if the parent already has them.
Syntax:
Here, we have captured the general syntax that you can use to define inheritance in a Java program. Please note that you will need a minimum of two classes to use the extend keyword:
class Parent { // methods // fields // …… } class Child extends Parent { // already supports the methods and fields in Parent class // additional features }
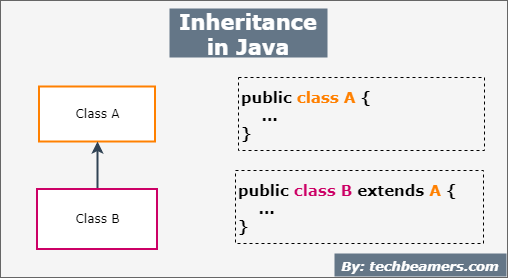
Inheritance example:
The example below will help you visualize the implementation of inheritance.
class Calculator { int add(int a , int b) { return a + b; } int sub(int a , int b) { return a - b; } } public class AdvancedCalculator extends Calculator { int mult(int a , int b) { return a * b; } int div(int a , int b) { return a / b; } public static void main(String args[]) { AdvancedCalculator cal = new AdvancedCalculator(); System.out.println( cal.add(1, 2) ); System.out.println( cal.sub(1, 2) ); System.out.println( cal.mult(1, 2) ); System.out.println( cal.div(1, 2) ); } }
The superclass AdvancedCalculator uses already defined add and sub-methods and adds more functionality (product and division). When the object cal comes to life, it gets a copy of the contents of the Calculator class by default.
Java facilitates all this with the help of constructors. It is important to note constructors are not inherited by subclass as they are neither methods nor fields. However, the child class can invoke the constructor of its parent.
Therefore, the output is:
3 -1 2 0
Super keyword
Description:
The super keyword provides a mechanism by which a subclass can invoke the constructor of the superclass. In case members and fields of both, classes have identical names, the super keyword helps differentiate between the superclass members from the subclass members.
The super keyword is very much like this keyword but only used for the superclass, within the subclass.
If the superclass has a predefined parametrized constructor, the subclass needs to invoke the superclass constructor using the super keyword.
Super keyword example:
The following example shows the use of the super keyword:
class Parent { int num = 1; int a; Parent(int a) { this.a = a; } void disp( ) { System.out.println("Number from Parent class " + num); } void getA( ) { System.out.println("Value of a " + a); } } public class Child extends Parent { int num = 2; Child(int a) { super( a ); } void disp( ) { System.out.println("Number from Child class " + num); } void methodToCallSuperKeyword( ) { System.out.println(super.num); super.disp(); } public static void main(String args[]) { Child child = new Child(100); child.methodToCallSuperKeyword(); System.out.println(child.num); child.disp(); child.getA(); } }
The output for the above snippet is:
1 Number from Parent class 1 2 Number from Child class 2 Value of a 100
The Java instanceof
operator can be used to check if a particular instance belongs to a class. For an inherited class, the instance of the subclass is an instance of both subclass and superclass.
Thus in the example above:
System.out.println(child instanceof Child); System.out.println(child instanceof Parent);
Outputs:
true true
Based on the example above, the Parent is the superclass of the Child class. In terms of the IS-A relationship, we can justify the Child’s IS-A Parent. By using the extend keyword, a subclass claims all the properties of its superclass, except for the private members.
HAS-A relation defines a way to determine if a class has a certain thing. For example in the above example the Child class HAS-A methodToCallSuperKeyword().
It is important to realize that Java does not support Multiple Inheritance. This means one class cannot extend multiple classes. However, with the help of interfaces, it is possible to achieve multiple inheritance in Java.