Hey there! Welcome to this in-depth tutorial on exception handling in Java. In programming, errors happen but you can handle them gracefully with the help of a cool feature like exception-handling. In this guide, we’ll break down the basics of exceptions, talk about different types, and walk through some hands-on examples to get you comfortable with handling those hiccups in your code.
Understanding Exceptions
An exception in Java is an event that disrupts the normal flow of a program. When an exceptional situation occurs, such as dividing by zero or accessing an array index out of bounds, Java creates an exception object. This object contains information about the error, allowing you to handle it gracefully.
We have added a general flowchart that depicts how a Java program can handle errors by using exception handling.
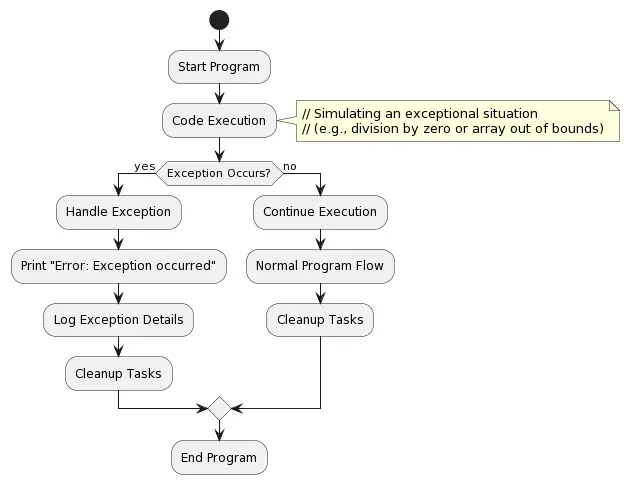
Types of Exceptions
Java classifies exceptions into two main types: checked and unchecked.
Checked Exceptions
Checked exceptions are those that the compiler forces you to handle explicitly. They include situations that may arise at runtime but are known during compilation. Examples include IOException
and SQLException
.
Here is a simple Java example to just give you a sense of what the checked exception is all about.
import java.io.*;
public class SimpleReader {
public static void main(String[] args) {
try {
BufferedReader r = new BufferedReader(new FileReader("file.txt"));
String c = r.readLine();
System.out.println("File content: " + c);
r.close();
} catch (IOException e) {
System.out.println("Error: Unable to read the file. Details: " + e.getMessage());
}
}
}
The above Java code deals with potential file reading issues. The try-catch block manages IOException, displaying an error message if it occurs. It ensures robust handling of file operation challenges.
Unchecked Exceptions
Unchecked exceptions, also known as runtime exceptions, do not require explicit handling. They typically occur due to programming errors at runtime and are subclasses of the RuntimeException
class. Examples include ArithmeticException
and NullPointerException
.
Let’s take a basic Java example to show how to handle unchecked exceptions in real-time.
public class SimpleDivision {
public static void main(String[] args) {
int num = 10, denom = 0;
try {
int result = num / denom;
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: Division by zero. " + e.getMessage());
}
}
}
In this program, we try to divide two numbers. If the second number is 0, an ArithmeticException occurs. The try-catch block catches this exception, and we print an error message. This demonstrates how we handle unchecked exceptions in a basic division operation.
Checked vs Unchecked
Here is a neck to the comparison between the two types of exceptions.
Checked Exceptions | Unchecked Exceptions |
---|---|
Must be handled or declared in the method signature. | Not required to be handled explicitly. |
IOException, SQLException | ArithmeticException, NullPointerException |
External conditions (I/O, database operations) | Programming errors or runtime issues |
In code, think of it this way:
Checked Exceptions: You have to deal with them or tell the program you’re aware they might happen.
Unchecked Exceptions: They might happen, and the program doesn’t insist you specifically plan for them. They often point to coding mistakes.
Basic Exception Handling in Java
Let’s delve into the basic syntax of handling exceptions in Java.
public class ExceptionHandlingExample {
public static void main(String[] args) {
try {
// Simulating a scenario that may cause an exception
int result = performOperation(8, 0);
System.out.println("Result of the operation: " + result);
} catch (ArithmeticException e) {
// Handling the specific exception
System.out.println("Oops! Cannot divide by zero.");
} finally {
// Code executed regardless of an exception
System.out.println("Finally block executed.");
}
}
// A method that may throw an exception
private static int performOperation(int num1, int num2) {
// Simulating a situation where dividing by zero occurs
return num1 / num2;
}
}
In this Java snippet, we’re trying to divide numbers, but things get tricky when dividing by zero. The performOperation
method deals with this by throwing an ArithmeticException, caught in a try-catch block. The finally
block guarantees that certain code runs, regardless of what happens during the operation.
Catching Multiple Exceptions
You can catch multiple exceptions in the same catch
block or have multiple catch
blocks for different exception types.
public class MultipleExceptionsExample {
public static void main(String[] args) {
try {
// Simulating situations that may cause different exceptions
int[] numbers = {1, 2, 3};
System.out.println("Value at index 4: " + numbers[4]); // ArrayIndexOutOfBoundsException
int result = performComputation(10, 0); // ArithmeticException
} catch (ArrayIndexOutOfBoundsException | ArithmeticException e) {
// Handling multiple exceptions with a single catch block
System.out.println("Ex Text: " + e.getMessage());
}
}
// A method that may throw an exception
private static int performComputation(int num1, int num2) {
// Simulating a situation where dividing by zero occurs
return num1 / num2;
}
}
In this Java example, we’re dealing with numbers. The performComputation
method tries to calculate, but it might run into trouble, like reaching beyond an array’s limits or attempting to divide by zero. The try-catch
block helps manage these issues neatly in a single go.
The finally
Block
The finally
block runs no matter if an exception happens or not. It’s often used for cleaning up tasks, such as closing files or releasing resources in your code.
public class CleanupTaskExample {
public static void main(String[] args) {
try {
// Simulating a situation that may cause an exception
int result = performTask(15, 3);
System.out.println("Task Result: " + result);
} catch (ArithmeticException e) {
// Handling the specific exception
System.out.println("Oops! Cannot perform the task.");
} finally {
// Code executed regardless of an exception
System.out.println("Cleanup: Task completed or not, we clean up here.");
}
}
// A method that may throw an exception
private static int performTask(int num1, int num2) {
// Simulating a situation where dividing by zero occurs
return num1 / num2;
}
}
In this Java code, check out our CleanupTaskExample
. We’re doing a task, but if things go south (like dividing by zero), the try-catch block handles it. The finally
block ensures cleanup happens, no matter what. It’s like tidying up after the task, making our code solid and dependable.
Handling Custom Exceptions in Java
In Java, you can extend the Exception
class to create your custom exception classes. This enables you to define and throw exceptions that suit your application’s specific needs. Here is a flowchart that is trying to demonstrate the custom exception handling flow in Java.
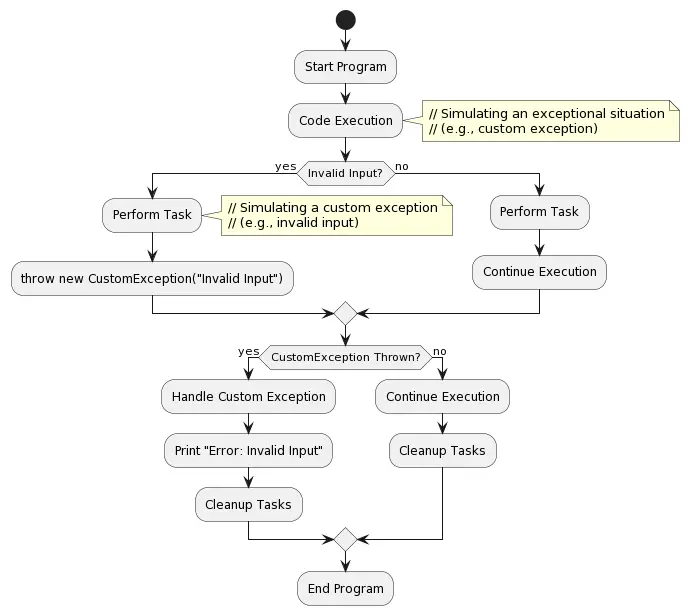
The following is the code snippet illustrating how to handle custom exceptions in Java code.
public class AgeValidationExample {
public static void main(String[] args) {
try {
// Simulating a scenario that may lead to a custom exception
verifyAge(15);
} catch (InvalidAgeException e) {
// Handling the custom exception
System.out.println("Oops! " + e.getMessage());
}
}
// A method that may throw a custom exception
private static void verifyAge(int age) throws InvalidAgeException {
if (age < 18) {
throw new InvalidAgeException("Age must be 18 or older.");
}
System.out.println("Age successfully verified.");
}
}
// Custom exception class
class InvalidAgeException extends Exception {
public InvalidAgeException(String message) {
super(message);
}
}
In this Java example, meet our AgeValidationExample. The verifyAge
method checks if the age is below 18, and if it is, it throws a custom exception (InvalidAgeException). The try-catch block takes care of this exception, giving us a fitting approach to handle age-related checks in our code.
Here’s an FAQ section to highlight some of the key concerns around exception handling in Java.
Frequently Asked Questions (FAQ)
Go through the below FAQs and clear any confusion you may have. You can ask any additional query via the comment box at the end of the tutorial.
Q1: What’s the primary purpose of exception handling in Java?
A1: Exception handling in Java helps manage unexpected situations, ensuring that programs can respond gracefully to errors and continue running without crashing.
Q2: When should I use a custom exception in my Java program?
A2: Use a custom exception when you want to represent and handle application-specific errors or situations not covered by standard Java exceptions. It adds clarity to your code.
Q3: What makes the finally
block important in dealing with exceptions?
A3: The finally
block guarantees the execution of specific code, irrespective of whether an exception occurs. It’s particularly handy for tasks like completing file operations or freeing resources.
Q4: Can I catch multiple exceptions in a single catch block?
A4: Yes, you can catch multiple exceptions using the pipe symbol (|
) in a single catch block. It provides a concise way to handle different exception types with similar actions.
Q5: What happens if an exception is thrown but not caught in Java?
A5: If an exception is not caught, it propagates up the call stack, potentially leading to program termination. It’s crucial to handle exceptions to prevent unexpected crashes.
Q6: How do I create a custom exception class in Java?
A6: To create a custom exception, extend the Exception
class. This allows you to define and throw exceptions tailored to your application’s specific needs.
Q7: Is it necessary to declare exceptions in the method signature?
A7: You can declare exceptions in the method signature using the throws
keyword, but it’s not mandatory for unchecked exceptions. Checked exceptions, however, must be declared.
Q8: Why is it important to catch exceptions at the appropriate level in the code?
A8: Catching exceptions at the right level allows you to handle errors where you have the most context, making your code more readable and responsive to unexpected situations.
Q9: Can I use the finally
block without a catch
block in Java?
A9: Yes, you can use the finally
block without a catch
block. It ensures that the specified code runs whether an exception occurs or not, providing a consistent cleanup mechanism.
Q10: How can I gracefully handle division by zero in Java?
A10: To handle division by zero, use a try-catch block around the division operation and catch the ArithmeticException
. Implement appropriate actions in the catch block to address the situation.
These FAQs aim to provide clarity and guidance on common questions related to Java Exception Handling. If you have more questions, feel free to dive into the specifics of your code and explore the nuances of exception handling in Java.
Exception Handling for Robust Java Code
Exception handling is key for robust Java programs. Understand try, catch, and finally block to create code that manages errors well, improving the user experience. Pick the right exception types, catch errors where needed, and use finally for cleanup.
- Catch errors where they happen.
- Log details for debugging help.
- Be specific with exception types.
- Keep error-fixing code short in Java.
So, in summary, wrap your code with care, catch issues where they arise, log wisely, use specific types, and keep it short for tidy Java programs.
Happy coding,
Team TechBeamers