This tutorial describes the Java ArrayList class and its operations like add, remove, search, and sort elements. It is available under the java.util
package.
A Simple Introduction to ArrayList in Java
Java ArrayList is a dynamic array that adjusts its size accordingly as elements get added or removed. The native array type has a fixed length and doesn’t allow resizing. You can’t add/remove values from it. To do so, one has to create a new object. On the contrary, the ArrayList grows or shrinks its size automatically as the elements pop in or out.
ArrayList is defined under Java’s collection framework and implements the List interface. Let’s check it out in detail and with the help of examples.
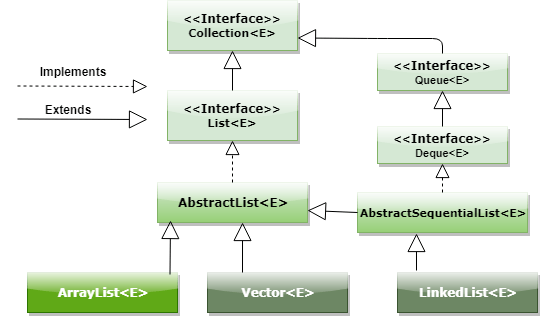
The following are the points that we are discussing in this Java ArrayList tutorial. Let’s check them out one by one.
Define ArrayList in Java
Java provides a wide variety of abstract data structures for better control over data and its features. The ArrayList in Java is one such data structure that is extended by AbstarctList and further implements the List interface.
Also Read: Interfaces in Java – OOP Concept
Java ArrayList and the arrays have a common property as both store data in a linear format. It means you can access any element from the base address of the first entry in the ArrayList or array.
Standard Java arrays have a predefined fixed length. They can’t be made to expand or shrink once they are defined. It means the programmer must be sure of how many entries the array needs to store. Hence, they are static by nature.
If you need to work with a dynamic data structure with similar properties as arrays, then use ArrayLists. They have a minimum initial size during their creation. Eventually, the list expands as more entries get added. Similarly, it shrinks when data entries get removed from the list.
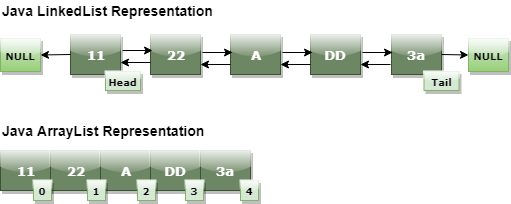
Syntax to Create Java ArrayList
Before starting to use the ArrayList, you must import the ArrayList library first. The imported library works for Generic Class E. It means you can use the ArrayList to hold data of any type. E can refer to Integer, String, or Boolean.
ArrayList<E> al = new ArrayList<>();
If you wish to use a predefined size for the ArrayList, then specify that too in the definition.
ArrayList<E> al = new ArrayList<>(n);
Where n is the capacity of the ArrayList, and it can grow as the user keeps on adding more elements to the list.
Some Keypoints
Here are some handful of facts about ArrayList in Java.
- Java ArrayList is a dynamic array, also called a resizable array. It adjusts (meaning expands/shrinks) itself automatically upon element addition/removal.
- It is a wrapper over arrays that it uses to store the elements. Therefore, it allows access using index values.
- The ArrayList doesn’t check for duplicate or null values.
- It is an ordered collection and preserves the insertion order by default.
- You can’t create an ArrayList from native types such as int, char, etc. Instead, use boxed types such as Integer, Double, Character, Boolean, etc.
- Java ArrayList is not threadsafe. The programmer should take care of synchronization while accessing ArrayList from multiple threads.
Also Read: How to Use Java String Format with Examples
Create an ArrayList and Add Elements
In this section, you’ll see how to create an ArrayList in a Java program. The very first step is to define an object of the ArrayList class and initialize it using the constructor method.
Below is an example that creates the ArrayList and adds elements using the add() method.
The add () method has this syntax:
# ArrayList add() method i. add(int index, Obejct obj) : Index where to add object, the object. ii. add(Obejct obj) : Object to be added to the end.
Now, check out the example.
import java.util.ArrayList; import java.util.List; public class TestArrayList { public static void main(String[] args) { // Define an ArrayList of Week days List weekDays = new ArrayList<>(); // Adding new elements to the ArrayList weekDays.add("Monday"); weekDays.add("Tuesday"); weekDays.add("Wednesday"); weekDays.add("Thursday"); weekDays.add("Friday"); weekDays.add("Saturday"); System.out.println(weekDays); // Adding an element at a zeroth index weekDays.add(0, "Sunday"); System.out.println(weekDays); } }
The result:
[Monday, Tuesday, Wednesday, Thursday, Friday, Saturday] [Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday]
Create ArrayList from ArrayList and Collection
If there is an existing ArrayList variable with some elements, then you can use it to initialize another object. Also, you can even add another collection to an existing ArrayList.
In the below example, we first created an ArrayList object and added a few elements to it. After that, the same variable is used as a seed to generate another ArrayList.
Moreover, the program also appends a collection to the ArrayList object using the addAll() method in the end.
import java.util.ArrayList; import java.util.List; public class CreateArrayListFromArrayList { public static void main(String[] args) { List seedArrayList = new ArrayList<>(); seedArrayList.add(1); seedArrayList.add(2); seedArrayList.add(3); System.out.println("Seed ArrayList: " + seedArrayList); // Let's create a new ArrayList from existing ArrayList List newArrayList = new ArrayList<>(seedArrayList); newArrayList.add(4); newArrayList.add(5); System.out.println("New ArrayList Seeded from an ArrayList: " + newArrayList); // Define a collection and add to our ArrayList List moreNumbers = new ArrayList<>(); moreNumbers.add(6); moreNumbers.add(7); moreNumbers.add(8); // Add the collection to our ArrayList newArrayList.addAll(moreNumbers); System.out.println("Updated ArrayList using Another Collection: " + newArrayList); } }
Here is the output:
Seed ArrayList: [1, 2, 3] New ArrayList Seeded from an ArrayList: [1, 2, 3, 4, 5] // 4 and 5 are new elements Updated ArrayList using Another Collection: [1, 2, 3, 4, 5, 6, 7, 8] // 6, 7, 8 get added from a collection
Access and Remove Elements from ArrayList
In this section, you’ll see how to use ArrayList in a Java program. First of all, you’ll create an ArrayList object and call the add()/remove()/get() method to insert/delete/access its elements.
Remove() method syntax is as follows:
# ArrayList remove() method i. remove(int index) : Index of the object to be removed. ii. remove(Obejct obj) : Object to be removed.
Get() method to access elements from an ArrayList:
# ArrayList get() method get(index)
The example below shows all the primary methods associated with ArrayList.
import java.util.ArrayList; import java.util.List; import java.util.Arrays; public class Test { public static void main(String args[]) { ArrayList al = new ArrayList<>(); al.add(0,1); // add element 1 at index 0 and so on al.add(2); al.add(2,3); // Difference between add(index, element) and add(element) // The first one throws an error - index is out of bound System.out.println(al); String name[]={"Hello","World","My","Name","Is","Java"}; List list = Arrays.asList(name); ArrayList Stringal = new ArrayList<>(); Stringal.addAll(list); // adds to the end of the list Stringal.addAll(5, list); // adds in the same order from the starting index // Will throw null pointer exception if Collection is null System.out.println(Stringal); System.out.println(Stringal.contains("H")); // Returns boolean value true if the element is present in the list System.out.println(al.get(1)); // Returns the element at specfied index System.out.println(Stringal.lastIndexOf("Is")); // Returns the last index of occurrence of the specified element al.remove(1); // Removes the element at the specified index Stringal.remove("3"); // Removes the specified element if present in the list System.out.println(al); System.out.println(Stringal); Stringal.set(0, "Hi"); // Replaces the element at specified index System.out.println(Stringal); System.out.println(Stringal.size()); // Returns the size of the list Stringal.clear(); // Removes all the element from the list System.out.println(Stringal); } }
The result is here:
[1, 2, 3] [Hello, World, My, Name, Is, Hello, World, My, Name, Is, Java, Java] false 2 9 [1, 3] [Hello, World, My, Name, Is, Hello, World, My, Name, Is, Java, Java] [Hi, World, My, Name, Is, Hello, World, My, Name, Is, Java, Java] 12 []
Traversing Java ArrayList
Java provides multiple ways to traverse an ArrayList. You can watch out for some of the approaches below, and later, the example shows how to use them to iterate the ArrayList.
- Standard Java for loop
- Standard While loop in Java
- Enhanced Java for loop (a.k.a. advanced for loop)
- Java ArrayList
forEach
method - Iterator approach
import java.util.ArrayList; import java.util.Iterator; public class TraverseArrayList { public static void main(String[] args) { ArrayList<String> books = new ArrayList<String>(); books.add("Python"); books.add("Java"); books.add("Go"); books.add("PHP"); System.out.println("\n*** Traverse ArrayList using Java for loop ***"); for(int i = 0; i < books.size(); i++) { System.out.println(books.get(i)); } System.out.println("\n*** Traverse ArrayList using While loop in Java ***"); int j = 0; while(j < books.size()) { System.out.println(books.get(j++)); } System.out.println("\n*** Iterate ArrayList using Enhanced Java for loop ***"); for(String book: books) { System.out.println(book); } System.out.println("\n*** Iterate Java ArrayList using its forEach method ***"); books.forEach((book) -> System.out.println(book)); System.out.println("\n*** Traverse Using the Standard Iterator() Approach ***"); Iterator bookIterator = books.iterator(); while (bookIterator.hasNext()) { System.out.println(bookIterator.next()); } } }
After execution, the output is:
*** Quick Traversal Using forEach and Lambda (Java 8) *** Python Java Go PHP *** Traverse Using the Standard Iterator() Approach*** Python Java Go PHP *** Using the Standard iterator() and forEachRemaining() (Java 8) *** Python Java Go PHP *** Using ListIterator to Traverse ArrayList (bidirectional) *** PHP Go Java Python *** Traverse via Regular for Loop *** Python Java Go PHP *** Traverse in a C-style for Loop with indices *** Python Java Go PHP
Find Elements in ArrayList
Java ArrayList class provides various methods to search for elements. These are as follows:
- Contains() – It returns true or false based on whether the ArrayList contains the element or not.
- IndexOf() – It returns the index of the first occurrence of an element.
- LastIndexOf() – Returns the index of the final occurrence of an element.
Check out the below Java ArrayList example:
import java.util.ArrayList; public class FindElementsInArrayList { public static void main(String[] args) { ArrayList<String> weekDays = new ArrayList<String>(); weekDays.add("Sunday"); weekDays.add("Monday"); weekDays.add("Tuesday"); weekDays.add("Wednesday"); weekDays.add("Thursday"); weekDays.add("Friday"); weekDays.add("Saturday"); weekDays.add("Sunday"); // Find if an ArrayList contains a specific element System.out.println("Does weekDays List Contain \"Monday\"? : " + weekDays.contains("Monday")); // Find the first position of an element in the ArrayList System.out.println("First Index Of \"Wednesday\": " + weekDays.indexOf("Wednesday")); System.out.println("First index Of \"Sunday\": " + weekDays.indexOf("Sunday")); // Find the last position of an element in the ArrayList System.out.println("Last Index Of \"Sunday\" : " + weekDays.lastIndexOf("Sunday")); } }
The output is:
Does weekDays List Contain "Monday"? : true First Index Of "Wednesday": 3 First index Of "Sunday": 0 Last Index Of "Sunday" : 7
Create an ArrayList of User-defined Type (UDT)
An ArrayList is a generic container. Therefore, it can hold all kinds of data such as all built-in types (Integer, Double, String, etc.), ArrayList, or any user-defined types.
In the below example, you’ll see how can we create an ArrayList of user-defined (UDT) type.
import java.util.ArrayList; class Book { private String author; private String subject; public Book(String author, String subject) { this.author = author; this.subject = subject; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public String getSubject() { return subject; } public void setSubject(String subject) { this.subject = subject; } } public class ArrayListBookDefinedObjectExample { public static void main(String[] args) { ArrayList<Book> Books = new ArrayList<Book>(); Books.add(new Book("Zed A. Shaw", "Python")); Books.add(new Book("Herbert Schildt", "Java")); Books.add(new Book("David Powers", "PHP")); Books.forEach(Book -> System.out.println("Author : " + Book.getAuthor() + ", Subject : " + Book.getSubject())); } }
The output is:
Author : Zed A. Shaw, Subject : Python Author : Herbert Schildt, Subject : Java Author : David Powers, Subject : PHP
Sort Elements in Java ArrayList
Sorting is a quite frequent activity that programmers need to do while programming. Java provides some innovative ways to sort the data in ArrayList.
- Collections.sort()
- ArrayList.sort()
- User-defined sorting
Let’s illustrate the sorting of Java ArrayList using an example.
Sort ArrayList using Java Collections’s sort() method
import java.util.ArrayList; import java.util.Collections; public class CollectionsSortClass { public static void main(String[] args) { ArrayList<Integer> numList = new ArrayList<Integer>(); numList.add(44); numList.add(22); numList.add(11); numList.add(33); numList.add(55); numList.add(66); numList.add(22); System.out.println("List prior to sorting : " + numList); // Sort the ArrayList using Collections.sort() Collections.sort(numList); System.out.println("List after sorting : " + numList); } }
The result:
List prior to sorting : [44, 22, 11, 33, 55, 66, 22] List after sorting : [11, 22, 22, 33, 44, 55, 66]
Sort using Java ArrayList’s sort() method
import java.util.ArrayList; import java.util.Comparator; public class BuiltInSortClass { public static void main(String[] args) { ArrayList<String> books = new ArrayList<String>(); books.add("Leran Python the Hard Way"); books.add("Java Primer"); books.add("PHP Objects"); books.add("JavaScript and JQuery"); System.out.println("books : " + books); // Sort using the sort() method. It needs a Comparator to specify as the argument books.sort(new Comparator<String>() { @Override public int compare(String source, String target) { return source.compareTo(target); } }); // Alternatively, you can use a lambda expression books.sort((source, target) -> source.compareTo(target)); // Here comes the most compact solution books.sort(Comparator.naturalOrder()); System.out.println("Sorted books : " + books); } }
The output:
books : [Leran Python the Hard Way, Java Primer, PHP Objects, JavaScript and JQuery] Sorted books : [Java Primer, JavaScript and JQuery, Leran Python the Hard Way, PHP Objects]
Sort ArrayList using a user-defined method in Java
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; class Employee { private String title; private Integer salary; public Employee(String title, Integer salary) { this.title = title; this.salary = salary; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public Integer getSalary() { return salary; } public void setSalary(Integer salary) { this.salary = salary; } @Override public String toString() { return "{" + "title='" + title + '\'' + ", salary=" + salary + '}'; } } public class SortUDTArrayList { public static void main(String[] args) { ArrayList<Employee> resource = new ArrayList<Employee>(); resource.add(new Employee("Manager", 2500000)); resource.add(new Employee("Team Lead", 1500000)); resource.add(new Employee("Engineer", 1000000)); resource.add(new Employee("Trainee", 500000)); System.out.println("Employee List before sorting: " + resource); // Sort by salary resource.sort((source, target) -> {return (source.getSalary() - target.getSalary());}); // A more compact approach resource.sort(Comparator.comparingInt(Employee::getSalary)); System.out.println("Sorted List by salary : " + resource); // Substitute method Collections.sort(resource, Comparator.comparing(Employee::getTitle)); System.out.println("Sorted List by title : " + resource); } }
The result:
Employee List before sorting: [{title='Manager', salary=2500000}, {title='Team Lead', salary=1500000}, {title='Engineer', salary=1000000}, {title='Trainee', salary=500000}] Sorted List by salary : [{title='Trainee', salary=500000}, {title='Engineer', salary=1000000}, {title='Team Lead', salary=1500000}, {title='Manager', salary=2500000}] Sorted List by title : [{title='Engineer', salary=1000000}, {title='Manager', salary=2500000}, {title='Team Lead', salary=1500000}, {title='Trainee', salary=500000}]
How to Protect ArrayList from Multiple Threads
The ArrayList class is not thread-protected by default. If multiple threads update an ArrayList simultaneously, then the result may become non-deterministic. It is because the changes by one thread may get overridden by another.
To observe this behavior, let’s first consider an example where multiple threads are trying to modify an ArrayList. If you need, check out our in-depth tutorial on multithreading in Java.
Accessing Un-synchronized ArrayList
This example intends to highlight the problem with ArrayList in a multi-threaded program.
import java.util.ArrayList; import java.util.concurrent.*; public class ArrayListInMultipleThreads { public static void main(String[] args) throws InterruptedException { ArrayList<Integer> sharedArrayList = new ArrayList<Integer>(); sharedArrayList.add(0); sharedArrayList.add(0); sharedArrayList.add(0); sharedArrayList.add(0); sharedArrayList.add(0); // Set up thread pool with 5 threads ExecutorService execService = Executors.newFixedThreadPool(5); // Prepare a Runnable task for decrementing ArrayList elements by one Runnable task = () -> {updateArrayList(sharedArrayList);}; // Execute the runnable task 999 times to update the ArrayList, all at once for(int i = 0; i < 999; i++) { execService.submit(task); } execService.shutdown(); execService.awaitTermination(60, TimeUnit.SECONDS); System.out.println(sharedArrayList); } // Update ArrayList by subtracting one from each element private static void updateArrayList(ArrayList<Integer> sharedArrayList) { for(int i = 0; i < sharedArrayList.size(); i++) { Integer value = sharedArrayList.get(i); sharedArrayList.set(i, value - 1); } } }
The output is:
[-985, -995, -981, -907, -996]
The expected result was [-999, -999, -999, -999, -999], but what we get is [-985, -995, -981, -907, -996].
Synchronizing ArrayList in Java
The below example demonstrates how to protect ArrayList from accessing multiple threads at the same time. Now, we’ll get the desired result.
import java.util.ArrayList; import java.util.concurrent.*; public class ArrayListInMultipleThreads { public static void main(String[] args) throws InterruptedException { ArrayList<Integer> sharedArrayList = new ArrayList<Integer>(); sharedArrayList.add(0); sharedArrayList.add(0); sharedArrayList.add(0); sharedArrayList.add(0); sharedArrayList.add(0); // Set up thread pool with 5 threads ExecutorService execService = Executors.newFixedThreadPool(5); // Prepare a Runnable task for decrementing ArrayList elements by one Runnable task = () -> {updateArrayList(sharedArrayList);}; // Execute the runnable task 999 times to update the ArrayList, all at once for(int i = 0; i < 999; i++) { execService.submit(task); } execService.shutdown(); execService.awaitTermination(60, TimeUnit.SECONDS); System.out.println(sharedArrayList); } // Update ArrayList by subtracting one from each element private static void updateArrayList(ArrayList<Integer> sharedArrayList) { synchronized(sharedArrayList) { for(int i = 0; i < sharedArrayList.size(); i++) { Integer value = sharedArrayList.get(i); sharedArrayList.set(i, value - 1); } } } }
We used Collections.synchronizedList() function to enable synchronization for the ArrayList. Also, we defined the updateArrayList()
method, which modifies the ArrayList in a synchronized manner.
The result of the above sample code is as follows:
[-999, -999, -999, -999, -999]
Java ArrayList Quick Q&A
Here are some quick questions and answers to refresh your knowledge of ArrayList.
Q. Why should you prefer ArrayList over Arrays?
Ans.
- Arrays don’t have built-in sorting or searching support.
- ArrayList can contain mixed objects, whereas Arrays can only hold similar data.
- An array is of pre-defined size and reserves memory in advance.
Q. How do you get an array from an ArrayList?
Ans.
ArrayList provides a toArray() method to return an array of elements.
String array[] = arrayList.toArray();
Q. How do you add new elements to an array list after it has been marked as final?
Ans.
Yes, we can add more elements to an ArrayList. Marking it as final only restricts that the ArrayList can’t contain any other ArrayList.
Q. How does insertion happen in the case of ArrayList and LinkedList?
Ans.
Since ArrayList uses arrays, when it needs to add an element in the middle, then it has to update all the subsequent indices. If the current container is full, then it requires to move the whole list to a new object.
On the contrary, the insertion is quite simple in the LinkedList. It is a doubly-linked list and only needs to adjust the pointers of the previous and next elements.
Q. How to find the duplicate elements in an ArrayList (Without Iteration/Loops)?
Ans.
The fastest to find duplicates is by copying the ArrayList elements to a Set. And then determine the difference between the length of ArrayList and Set.
It is the nature of the Set that it doesn’t accept duplicates. Hence, it’ll exclude all of them.
Q. What is the primary difference between List and ArrayList?
Ans.
The list is a Java interface, whereas ArrayList is an implementation of List.
Q. What are the differences between Arrays and ArrayList?
Ans.
- Arrays are of static size, but ArrayList is of variable length.
- An array can have both primitives and objects but of the same type at a time.
- ArrayList can only have object elements but can be of different types.
- Arrays can’t work with generics, but ArrayList is flexible to do so.
- The array has a length attribute, whereas ArrayList provides a size() method to return the number of elements.
- You can use the assignment operator to add elements to an array, whereas ArrayList provides add() method.
Q. What are the differences between ArrayList and LinkedList?
Ans.
- ArrayList uses arrays, whereas LinkedList gets a doubly-linked list to store elements.
- Updating an ArrayList is slower than a LinkedList because it consumes time in bit-shifting.
- The ArrayList can only act as a List, but the LinkedList can work both as a List and Queue.
- ArrayList is better when it comes to storing and searching. However, the LinkedList is good at manipulating data.
Q. What is the difference between ArrayList and Vector?
Ans.
The Vector implements synchronization for its methods, whereas ArrayList leaves it to the programmers.
Also, both the Vector and ArrayList make use of arrays internally. And, they enable dynamic resizing. However, the difference is in the way they implement it.
By default, Vector takes double the size of its array for expansion, whereas ArrayList expands only by half.
Q. Which of Vector or ArrayList is more useful in Java?
Ans.
Vector has built-in synchronization, which at times becomes an overhead. Hence, ArrayList is better as it allows programmers to turn protection on or off.
Before You Leave
Today, we covered an important concept in Java which is ArrayList. It is an essential topic to learn for serious programmers. It has O(n) performance and is faster than its counterparts, such as Vector, LinkedList, and HashMap.
Lastly, our site needs your support to remain free. Share this post on social media (Linkedin/Twitter) if you gained some knowledge from this tutorial.
Thanks,
TechBeamers