This tutorial will guide you on how to use while loop in Java programs, perform repetitive tasks, and iterate through the elements of a collection or array.
The while loop is a core Java programming construct used to perform repetitive tasks. We’ll now see how seamlessly you can use it in programs.
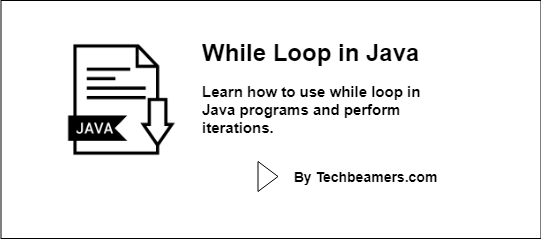
The tutorial has the following sections to help you learn quickly.
Basics of while loop in Java
A while loop is an entry-controlled loop statement that allows the code to execute if and only if it passes through the entry condition. You can assume it as a chain of repeating if expression.
Syntax
The while loop places the condition at the entry gate.
while (boolean expression) { statement(s); }
- The while loop in Java begins executing the code block only after the conditional expression returns true. That is why we call it a pre-condition loop.
- If the pre-condition evaluates to true, then the inside block gets executed. Usually, the conditional statement uses a dynamic counter which gets updated in every iteration.
- Whenever the test condition turns false, the loop ends the cycle which indicates the closing of its life cycle.
Also Read: Do-While Loop in Java
Example
Check this simple example of the while loop.
public class SimpleWhile { public static void main(String args[]){ int iter = 0; while (iter <= 10) { System.out.print(iter + " "); iter++; } } }
The above loop will run 11 times printing numbers from 0 – 10.
Flowchart
The Java while loop flow chart will help you visualize it:
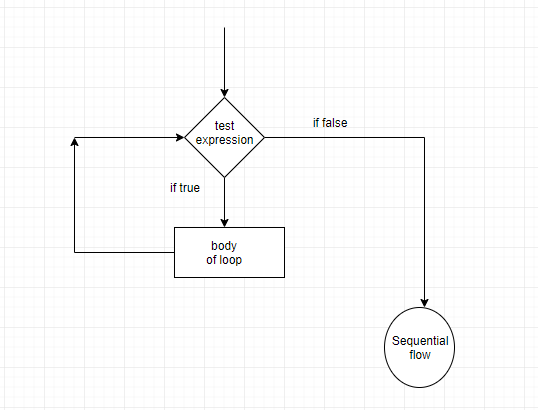
While loop examples
You can use a while loop in Java programs for many purposes. Below are some samples to demonstrate it.
Generate first N whole numbers
public class WhileLoop { public static void main(String args[]) { int n = 0; int N = 5; while ( n <= N ) { System.out.print(n + " "); n++; // Incrementing n by 1 in each iteration } } }
Instead of writing the print statement for N times, we made the while loop in this Java program resolve it. Here ‘n’ is the loop control variable and N has a fixed value.
The output is as follows:
0 1 2 3 4 5
Count backward from a given number
public class WhileLoop { public static void main(String args[]) { int N = 5; while ( N >= 0 ) { System.out.print(N + " "); N--; // Decrementing N by 1 in each iteration } } }
The result is as follows:
5 4 3 2 1 0
You can see that the while loop is letting us manipulate the test condition and update the counter to produce different outputs.
Iterate through an array/collection
public class WhileLoop { public static void main(String args[]) { char char_arr[] = {'a', 'b', 'c', 'd'}; // array index begins with 0 int iter = 0; while( iter < char_arr.length ){ System.out.print(char_arr[iter] + " "); iter++; } } }
Test run:
a b c d
Please note that if you replace the array type in the above example from integer to char, then while loop will print the ASCII values of each char.
97 98 99 100
Must Read – Java For Loop Statement