This tutorial will guide you on how to use do while loop in Java programs, perform repetitive tasks, and iterate through the elements of a collection or array.
It is a core Java programming construct used to perform repetitive tasks. The tutorial has the following sections to help you learn quickly.
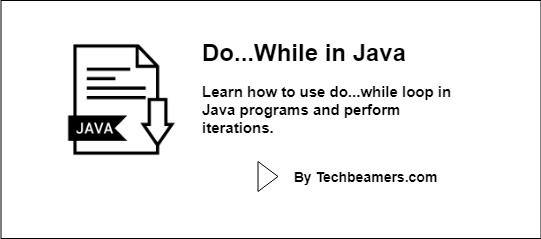
Basics of do while loop in Java
Unlike the while loop in Java which checks the condition at the entry, do…while allows the code block to execute at least once. After the first iteration, it evaluates the test condition and decides whether to continue or not.
Similar to other loops, it too accepts only those expressions which produce a boolean result. Please note that a non-zero integer value also evaluates to true.
Syntax
The do-while loop in Java places the condition at the entry gate.
do { statement(s); } while (boolean expression);
- The do…while loop executes a block of code continuously until the given expression returns true.
- It is more like a Java while loop except that the do-while guarantees the execution at least once.
- It terminates the cycle immediately when the condition turns false.
e.g.
public class SimpleDoWhile { public static void main(String args[]){ int iter = 0; do { System.out.print(iter + " "); } while (iter++ < 10); } }
The above loop will run 11 times producing numbers from 0 – 10.
Output:
0 1 2 3 4 5 6 7 8 9 10
Flowchart
The flow chart will help you visualize the do-while loop in Java:
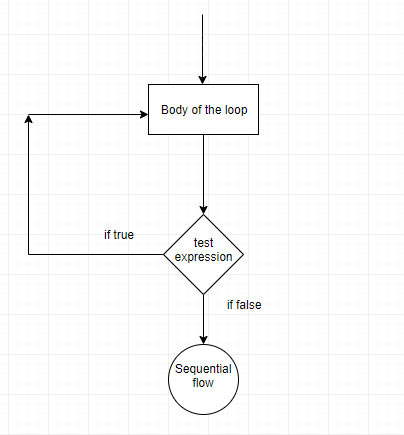
Do while examples
You can use the do-while loop for many purposes. Below are some samples to demonstrate it.
Generate first N whole numbers
public class doWhileLoop { public static void main(String args[]) { int n = 0; int N = 5; do { System.out.print(n + " "); n++; // Incrementing n by 1 in each iteration } while ( n <= N ); } }
In the above Java program, instead of writing the print statement for N times, we made the do-while loop resolve it. Here ‘n’ is the loop control variable and N has a fixed value.
The output is as follows:
0 1 2 3 4 5
Count backward from a given number
public class doWhileLoop { public static void main(String args[]) { int N = 5; do { System.out.print(N + " "); N--; // Decrementing N by 1 in each iteration } while ( N >= 0 ); } }
The result is as follows:
5 4 3 2 1 0
You can see that do…while loop is letting us manipulate the test condition and update the counter to produce different outputs.
Iterate through an array/collection
public class doWhileLoop { public static void main(String args[]) { char char_arr[] = {'a', 'b', 'c', 'd'}; // array index begins with 0 int iter = 0; do { System.out.print(char_arr[iter] + " "); iter++; } while( iter < char_arr.length ); } }
Test run:
a b c d
Please note that if you replace the array type in the above example from integer to char, then do…while loop will print the ASCII values of each char.
97 98 99 100
Must Read – For Loop in Java