This tutorial will guide you on how to use a for loop in Java programs, perform repetitive tasks, and iterate through the elements of a collection or array. It is a core Java programming construct used to perform repetitive tasks.
Basics of For Loop in Java
The tutorial has the following sections to help you learn quickly.
The flow of a program
The workflow of an application represents how the compiler executes the lines of your code. There are three basic types of flow in a Java program:
Sequential:
Sequential flow is the normal flow of execution. It means the very first instruction that will execute is line 1, then 2, and so on until the control reaches the end of your code.
Conditional:
Conditional flow occurs when the execution reaches a specific part in your code that has multiple branches. Here, the result of the condition decides the course of the program.
Java supports two conditional statements: if-else and Switch-Case.
Iterative:
Iterative flow comes into the light when the control enters a block which repeats itself for a specified number of cycles.
Java provides looping statements such as for, while, and do-while loops to achieve this effect. The user can decide how many times the block runs in the program.
Must Read – Variable in Java
For Loop
Description:
For loop provides the most straightforward way to create an iterative block. It has a three-instruction template where the first is to initialize the loop counter, the second is the condition to break, and the third increments the counter.
It is like a contract which makes all the terms and conditions pretty clear and visible. The for loop also gives the programmer the highest level of visibility over the number of iterations and the exit condition.
Syntax:
It has a cleaner and informative structure:
for (init counter; check condition ; move counter) { statement(s); }
As we said there are three statements in the for-loop. The first instruction tells when to start the loop; you initialize a variable here with some value.
The second statement is a condition which if evaluates to true; then the loop continues or else breaks.
In the next statement, you can move the counter both ways, i.e., increment or decrement its value.
e.g.
for (int iter = 0; iter <= 10 ; iter++) { System.out.println("iter: " + iter); }
The above loop will run 11 times printing numbers from 0 – 10.
Flowchart:
Check below is the for-loop flow diagram.
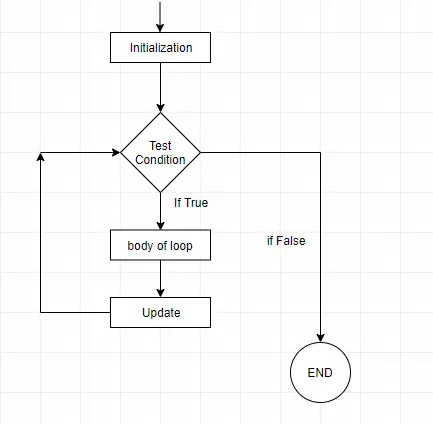
Also, Read – Data types in Java
Advanced looping technique
Java has one more style of “for” loop first included in Java 5. It lays down an easy way to traverse through the items of a collection or array. You should use it only for sequentially iterating an array without using indexes.
In this type, the object/variable doesn’t change, i.e., the array doesn’t change, so you can also call it a read-only loop.
Syntax:
for (T item:Collection obj/array) { instruction(s) }
Examples:
Print numbers in a single line:
public class MyClass { public static void main(String args[]) { int N = 5; for (int iter = 0; iter < N; ++iter) { System.out.print(iter + " "); } } }
Instead of writing the print statement for n times, we made the for loop resolve it. Here ‘iter’ is the loop control variable.
The output is as follows:
0 1 2 3 4
Count backward from a given number:
public class MyClass { public static void main(String args[]) { int N = 5; for ( int iter = N; iter > 0; iter-- ) { System.out.print(iter + " "); } } }
The result is as follows:
5 4 3 2 1
You can see that the “for” loop lets us manipulate the test condition and update the statement to yield different outputs.
Iterate through a collection:
public class MyClass { public static void main(String args[]) { String array[] = {"Python", "Java", "CSharp"}; // Advanced for loop for (String item:array) { System.out.print(item + " "); } System.out.println(" "); // Standard for loop for (int iter = 0; iter < array.length; iter++) { System.out.print(array[iter] + " "); } } }
After execution, the following values will print:
Python Java CSharp Python Java CSharp