This tutorial provides you with the details of various Java data types. It is an essential topic for you to know and develop real Java programs.
Learn to Use Data Types in Java
The tutorial has the following sections to help you learn quickly.
Data types in Java
Every programming language provides a set of data types for the programs to pass data and tell its compiler/interpreter to process it accordingly.
Java too defines the following two major categories for data types:
- Primitive
- Non-primitive
Primitive types:
Primitive data types consist of the following eight variations:
· Integer (int):
This data type is used to hold integer data values. The size of an int is 4 bytes. And the range of values it can store is – 2,147,483,648 (-2^31) to 2,147,483,647 (2^31 -1) (inclusive).
int a = 10;
· byte:
This data type can also hold integer values. The size of a single byte is 1 byte. Therefore, the range of values it can store is -128 to 127 (inclusive).
byte b = 10;
· short:
It holds integer values, and its size is 2 bytes. The range of values is -32,768 to 32,767 (inclusive).
short c = 120;
· long:
The long data type is the biggest of all data types as it consumes memory of 8 bytes and holds integer type values. Range of long is -9,223,372,036,854,775,808(-2^63) to 9,223,372,036,854,775,807(2^63 -1)(inclusive).
long d = 10000;
By default, integer literals will have an int data type. Literal is the value stored in the variable.
int a = 20; // 20 is the literal and its type is int byte b = 20; // here also, 20 is the literal but its type is byte
We rely on the concept of typecasting (both internal and external) to convert the int literal to any other format.
· float:
A float is a data type used to store real numbers in Java. The size of a float is 4 bytes, and the range of values it can allow is approximately ±3.40282347E+38F up to 6-7 significant decimal digits as per the IEEE 754 standard.
float f= 23.4f;
· double:
A double is another data type that allows real numbers with double precision. The size of the double is 8 bytes, and it can store approximately ±1.79769313486231570E+308 up to 15 significant decimal digits.
double d = 1.2;
By default, all decimal numbers end up as double literals. Therefore, we typecast the value for float by adding an addition “f” along with the value.
float f = 1.2f;
· boolean:
This data type stores only boolean values. It means that either it can assume a true or false value.
boolean val = true;
· char:
The char data type facilitates to storage of characters. Its size is 2 bytes, and the values map according to the Unicode characters. The range of values lies between ‘\u0000’ (or 0) to ‘\uffff’.
Learn to Write Your First Java Program.
Non-primitive types:
Non-primitive are user-defined data types. Their purpose is not to store a value. Instead, they refer to a memory location. In programming terms, we call it a Heap, which further allocates space for the primitive data types (involved in the non-primitive operations).
For non-primitive data types, Java keeps the reference, additionally called location, not merely a value.
Alternatively, we refer to them as reference data types. They provide a way to grant space to members of a class or interface. An array is the simplest example of a non-primitive data type.
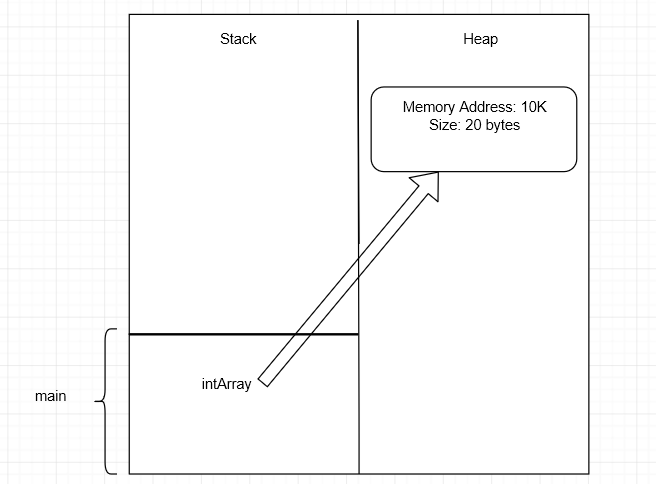