This tutorial explains how to use switch-case in Java programs. It is a multi-branch statement that allows the execution of different pieces of code based on the result of an expression.
The switch case block can accept a value of int, byte, char, or short types. From JDK7, it even started allowing Enums, Strings, and objects of Wrapper classes as well.
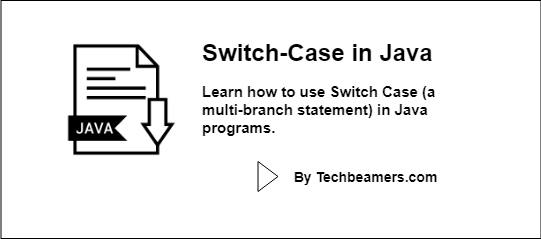
Basics of switch-case in Java
The tutorial has the following sections to help you learn quickly.
The flow of a Java program
Whenever you write a piece of code, the compiler has to convert it into bytecode and further give you the desired output. The flow of a program represents the execution order of underlying statements.
There can be three types of flows in a program:
Sequential
The sequential flow of a program is the normal flow. It means line 1 executes first, then line 2, line 3, and so on until the control reaches the end of your code.
Conditional:
The conditional flow of a program occurs when a specific part of the code executes leaving aside another piece. It means which part to run depends upon the result of conditional statements.
Java supports two conditional statements: if-else and switch-case.
Iterative
An iterative flow encounters when a block in a program runs repeatedly. Iterative flow is made sure by a structure called loops in Java. The user decides how many times the block runs in the program.
Switch-case statement in Java
Description
The switch statement is used when the deciding expression can take more than two values. It means to test the expression against a list of values.
We can draw similarities between Java if else statements and switch-case.
Each else-if block can be compared with the case block, and the variable is checked at every case. However, one big difference between them is visible through the concept of fall through.
The fall-through can occur with a switch case.
Syntax
The expression mentioned within the switch can take values of integer or other integer primitive data types(byte, short, long) or Strings. A switch block can include any number of case blocks.
The syntax of a switch case block in Java is to write the keyword ‘case’ along with the value with which you want to check, followed by a colon.
It is important to remember the values in case blocks must be of the same data type which is mentioned within the switch expression.
Switch-case in Java does not allow any other relational operation except equality. A general syntax for switch statements looks like:
switch (expression) { case val1: { statement(s); break; // optional } case val2: { statement(s); break; // optional } default: { statement(s); } }
An example of the same would be:
e.g.
int value = 10; switch(value) { case 10: { System.out.println("Value is 10"); break; } case 20: { System.out.println("Value is 20"); break; } default: { System.out.println("Default value is 0"); } }
Output:
Value is 10.
Flowchart
Let’s try to understand the Java switch case statement with the help of a flowchart.
When the variable matches with a certain case’s value, the statements within the case block run until an optional break statement occurs.
“break
” is a keyword in Java that makes sure when reached, the conditional flow terminates, and the sequential control of flow is attained.
This means the flow of the program reaches the next line after the switch block. The flow diagram will help you visualize the role of the break statement:
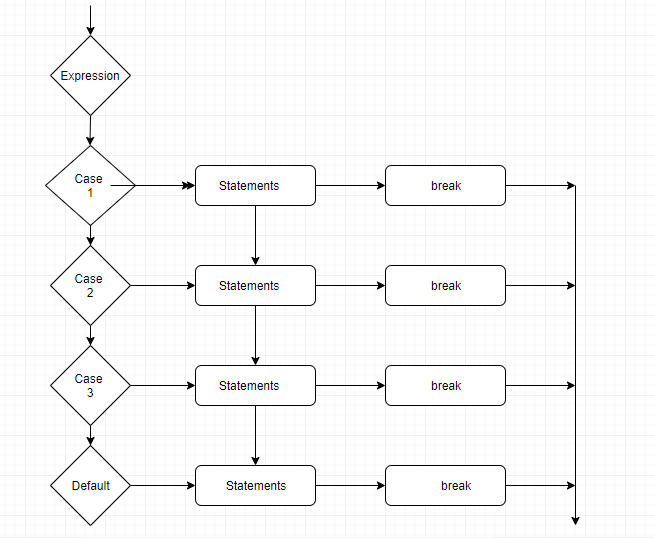
Fall through
It is not necessary to include break statements for all cases. However, it would not make any sense not to.
If the break skips for a matching case, then the compiler is still in the conditional flow, and irrespective of the value, matched or not, it keeps on executing the subsequent case blocks until it finds a break statement.
In Java, we call this scenario a fall-through. Refer to the below example illustrating this condition:
int value = 10; switch(value) { case 10: { System.out.println("Value is 10"); } case 20: { System.out.println("Value is 20"); } case 30: { System.out.println("Value is 30"); } default: { System.out.println("Default value is 0"); } }
Output:
Value is 10 Value is 20 Value is 30 Value is 0