This tutorial will guide you on how to add conditions in Java programs. You will see different types of if, if-else statements to implement decision-making.
If you want your program to execute some code based on a condition or a different block otherwise, then you need to use control flow statements like if or if-else. Take an example; you have to print whether a year is a leap year or not.
If the days are 366, then you should display “A Leap Year” otherwise print “Not a Leap Year.” So, you need two print statements in the program, but only one of them will run at a time based on the “days” value.
Today, we’ll teach how can you add such types of conditions in your programs using if-else statements.
Basics of If-else in Java
There are four variations of if-else statements available in Java.
- if statement
- Nested if statement
- if-else statement
- if-else-if statement
Simple if statement
Description:
This if statement denotes a condition joined by one or more statements enclosed in curly braces.
If the block has one statement, then there is no need for the curly braces.
if ( test_condition or expr ) { statement(s); }
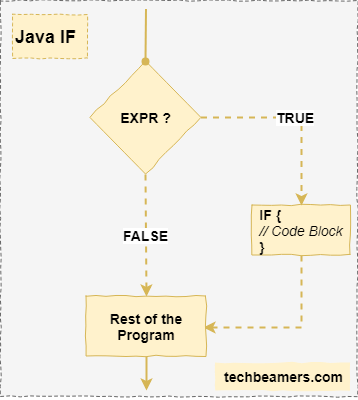
The block runs only when the condition evaluates to true. If the result is false, then the instructions inside if block ignore execution.
Please note that while forming a condition, you can join multiple expressions or boolean values using operators like AND (&&), OR (||), NOT (!), etc.
Example:
public class SimpleIfStatement { public static void main(String args[]) { int days = 366; if( days == 366 ) { System.out.println("A Leap Year!"); return; } System.out.println("Not a Leap Year."); } }
Output:
The above program would print the following:
A Leap Year
Nested if statement
Description:
It means you have a conditional block that has another if condition. This chain can go on and on. That’s why we call it a nested if statement.
In reality, a nested if would look like the following:
if(expr_1) { outer_statements; if(expr_2) { inner_statement(s); } }
If you review the example, you will understand that the outer statement would run first if the expr_1 returns true. The inner_statement will run if both the expressions ( expr_1/2) evaluate to true.
Example:
public class NestedIfProgram { public static void main(String args[]){ int days = 366; int month = 2; if( days == 366 ){ System.out.println("A Leap Year!"); if(month == 2){ System.out.println("Month is Feb with 29 days."); } } } }
Output:
A Leap Year! Month is Feb with 29 days.
If-else statement
Description:
This statement caters to both parts, one is the if block which executes upon matching the condition and the second is the else code block which runs when the condition doesn’t match.
if ( test condition ) { statement(s); } else { statement(s); }
The above statements inside “if” will run if the condition evaluates to true, and the code inside the “else” block executes if the condition fails.
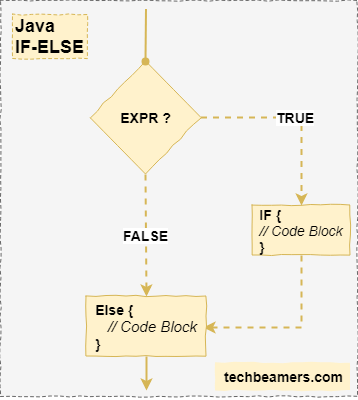
Example:
public class IfElseProgram { public static void main(String args[]){ int days = 365; if( days == 366 ){ System.out.println("A Leap Year!"); } else { System.out.println("Not a Leap Year."); } } }
Output:
Not a Leap Year.
If-else-if statement
Description:
When you have to handle a use case that has multiple conditions, you would need one starting if and the other following else-if statements.
The standard technical name for this construct is if else if ladder.
if ( test condition 1) { statement(s); } else if ( test condition 2) { other statement(s); else if ( test condition 3) { More statement(s); }
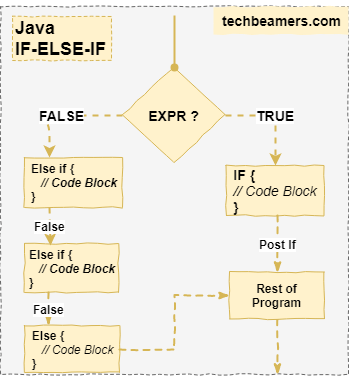
Please note that if a condition is found as true, then the corresponding set of statements executes leaving others as is. If no condition matches, then instructions inside the “else” part execute.
Example:
public class IfElseIfProgram { public static void main(String args[]){ int day = 1; if(day == 1) { System.out.println("Monday"); } else if(day == 2) { System.out.println("Tuesday"); } else if(day == 3) { System.out.println("Wednesday"); } else if(day == 4) { System.out.println("Thrusday"); } else if(day == 5) { System.out.println("Friday"); } else if(day == 6) { System.out.println("Saturday"); } else if(day == 7) { System.out.println("Sunday"); } else { System.out.println("Invalid day!"); } } }
Output:
Monday
Must Read – Switch Case Statement in Java