Here is a quick guide on Python’s random number. You can always refer to it whenever you need to generate a random number in your programs. Python has a built-in random module for this purpose. It exposes several methods such as randrange(), randint(), random(), seed(), uniform(), etc. You can call any of these functions to generate a Python random number.
Multiple Ways to Generate Random Numbers in Python
Usually, a random number is an integer, but you can generate float random also. However, you first need to understand the context as a programmer and then pick the right function to use.
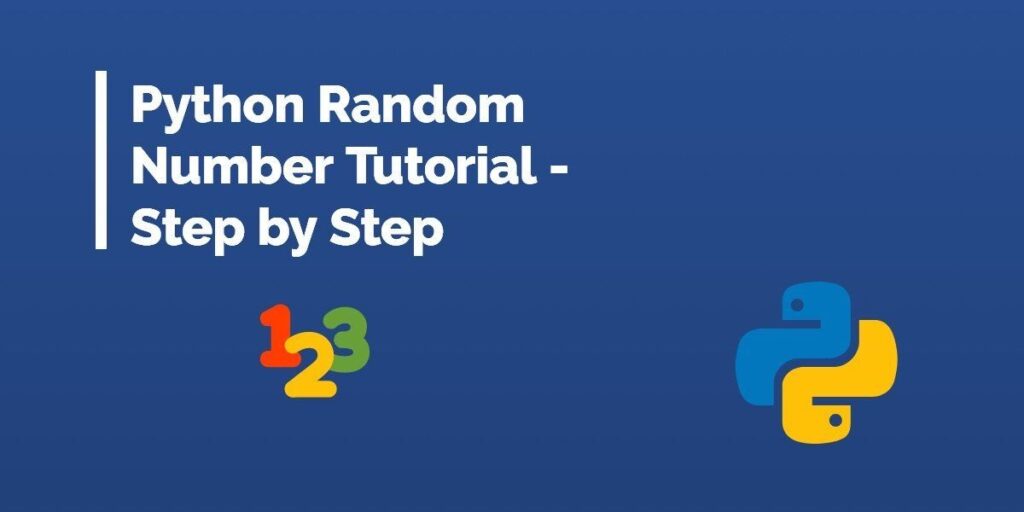
What is a Random Number Generator?
A random number generator is a deterministic system that produces pseudo-random numbers. It uses the Mersenne Twister algorithm that can generate a list of random numbers.
A deterministic algorithm always returns the same result for the same input.
It is by far the most successful PRNG technique implemented in various programming languages.
A pseudo-random number is statistically random, but has a defined starting point and may repeat itself.
What is Python Random Module?
Python has built-in support for generating numbers by using the random module. It has many functions to produce random numbers depending on your needs. These values are pseudo-random numbers. They are generated using algorithms that depend on an initial value called a seed.
Let’s now check out some real examples to generate random integer and float numbers.
Python randrange() Method
The randrange() function is available with the Python random module. It can help you produce a random number from a given range of values.
This function has the following three variations:
Syntax
# Type:1 randrange() with all three arguments
random.randrange(begin, end, step counter)
While using this form of randrange(), you have to pass the start, stop, and step values.
# Type:2 randrange() with first two arguments
random.randrange(begin, end)
With this form, you provide the start and stop, and the default (=1) is used as the step counter.
# Type:3 randrange() with a single argument
random.randrange(end)
You only need to pass the endpoint of the range. The value (=0) is considered for the starting point and the default (=1) for the step counter.
Also, please note the end/stop value is excluded while generating a random number. This function produces the ValueError exception in the following cases:
- If you have used a float range for the start/stop/step.
- Or if the start value is more than the stop value.
Let’s now check out a few examples.
Example-1
import random as rand
# Generate random number between 0 to 100
# Pass all three arguments in randrange()
print("First Random Number: ", rand.randrange(0, 100, 1))
# Pass first two arguments in randrange()
# Default step = 1
print("Second Random Number: ", rand.randrange(0, 100))
# Or, you can only pass the start argument
# Default start = 0, step = 1
print("Third Random Number: ", rand.randrange(100))
The output is:
First Random Number: 3
Second Random Number: 36
Third Random Number: 60
Example-2
import random as rand
# Generate random number between 1 to 99
# Pass all three arguments in randrange()
print("First Random Number: ", rand.randrange(1, 99, 1))
# Pass first two arguments in randrange()
# Default step = 1
print("Second Random Number: ", rand.randrange(1, 99))
The result is:
First Random Number: 18
Second Random Number: 77
Python randint() Method
The randint() function is somewhat similar to the randrange(). It, too, generates a random integer number from the range. However, it differs a bit:
- Randint() has two mandatory arguments: start and stop
- It has an inclusive range, i.e., can return both endpoints as the random output.
Syntax
# random module's randint() function
random.randint(start, stop)
This function considers both the beginning and end values while generating the random output.
Example
import random as rand
# Generate random number using randint()
# Simple randint() example
print("Generate First Random Number: ", rand.randint(10, 100))
# randint() along with seed()
rand.seed(10)
print("Generate Second Random Number: ", rand.randint(10, 100))
rand.seed(10)
print("Repeat Second Random Number: ", rand.randint(10, 100))
The following is the result:
Generate First Random Number: 14
Generate Second Random Number: 83
Repeat Second Random Number: 83
Python random() Method
It is one of the most basic functions of the Python random module. It computes a random float number between 0 and 1.
This function has the following syntax:
Syntax
# random() function to generate a float number
random.random()
Example
import random as rand
# Generate random number between 0 and 1
# Generate first random number
print("First Random Number: ", rand.random())
# Generate second random number
print("Second Random Number: ", rand.random())
The following is the output after execution:
First Random Number: 0.6448613829842063
Second Random Number: 0.9482605596764027
Python seed() Method
The seed() is a special method of the Python random module. It lets you control the state of the random() function. It means that once you set a seed value and call random(). Python then maps the given seed to the output of this operation.
So, whenever you call seed() with the same value, the subsequent random() returns the same random number.
Syntax
# random seed() function
random.seed([seed value])
The seed is an optional field. Its default value is None, which means to use the system time for random operation.
How to Use Seed() with random()
import random as rand
# Generate random number using seed()
# Using seed() w/o any argument
rand.seed()
print("Generate First Random Number: ", rand.random())
rand.seed()
print("Generate Second Random Number: ", rand.random())
# Using seed() with an argument
rand.seed(5)
print("Generate Third Random Number: ", rand.random())
rand.seed(5)
print("Repeat Third Random Number: ", rand.random())
Here is the execution result:
Generate First Random Number: 0.6534144429163206
Generate Second Random Number: 0.4590722400270483
Generate Third Random Number: 0.6229016948897019
Repeat Third Random Number: 0.6229016948897019
Please note that you can even pair up seed() with other Python random functions such as randint() or randrange().
Python uniform() Method
The uniform() function computes a random float number from the given range. It takes two arguments to specify the range.
The first parameter is inclusive of random processing.
Syntax
# random uniform() function
random.uniform(start, stop)
This function provides a random result (r) satisfying the start <= r < stop. Also, please note that both the start and stop parameters are mandatory.
Python Random Numbers Using uniform()
import random as rand
# Generate random float number using uniform()
# Simple randint() example
print("Generate First Random Float Number: ", rand.uniform(10, 100))
print("Generate Second Random Float Number: ", rand.uniform(10, 100))
The result after execution is:
Generate First Random Float Number: 20.316562022174068
Generate Second Random Float Number: 27.244409254588806
More Related Tutorials
Generate a Random Number List in Python
Generate Random Characters in Python
A Guide to Python Random Sampling
Generate Random Images in Python
Python Code to Generate Random Email
Python Code to Generate Random Integers
Now, we have come to the end of this Python random number generator tutorial. We hope that it will help you lead in the right direction. You may also like to read the other tutorials on our blog.
Happy coding!