This post explains how to create a random email generator in Python. It lays down different ways to generate random emails and provides code samples that you can quickly use.
Also Read:
Python Generate Random Images
Python Generate Random IP Address
But firstly, a random email is an auto-generated email address using a computer program. It does not have to be a real email address that can be used to send or receive emails. Random emails are often used for testing purposes.
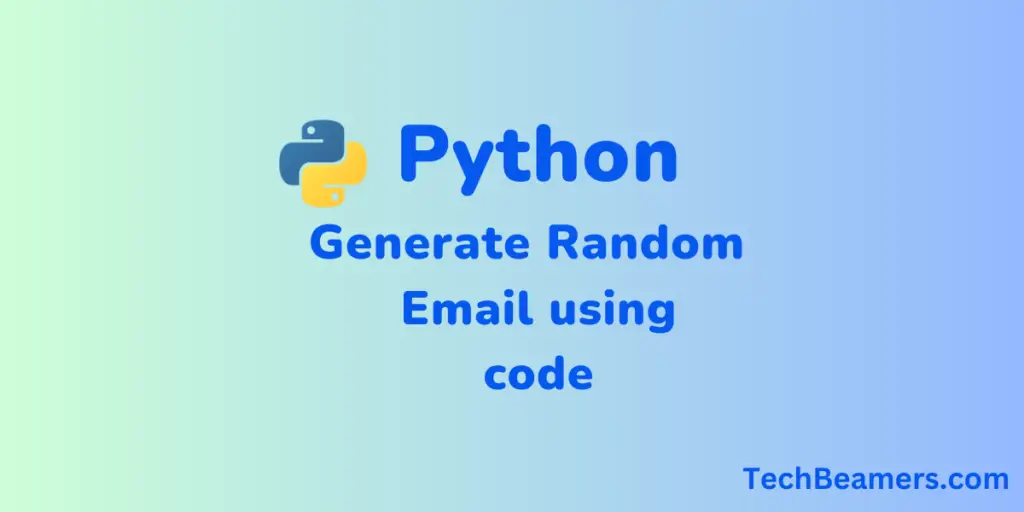
What is a random email generator?
A random email generator is a tool that creates email IDs that don’t belong to real people. They have quite a use in testing. For example, developers use it for sending or receiving test emails.
To generate a random email address, selecting a user and a domain name is necessary. The username is usually a combination of letters and numbers. While a domain name represents an email service provider like Gmail or Yahoo.
Here is an example of a random email address: randomemail@gmail.com
.This email address is generated by randomly selecting the username “randomemail
” and the domain name “gmail.com”.
Random email generators can be used for a variety of purposes. Some of the most common purposes include:
- Testing email sending and receiving code
- Creating temporary email IDs for online registrations
- New product promotion emails
Different ways to generate random email
Certainly, there are many ways to generate random emails in Python. Here are a few of the most common methods:
Using random strings
In Python, we can generate random strings and combine them into email-like addresses.
Approach: We’ll include Python’s random module to craft random characters into a string similar to an email address. To sum up, check the below code.
import random
import string
def generate_random_email():
domain = "@example.com"
username_length = random.randint(5, 10)
username = ''.join(random.choice(string.ascii_lowercase) for _ in range(username_length))
return username + domain
# Example usage
random_email = generate_random_email()
print(random_email)
Explanation: In this code, a random username with a length between 5 and 10 characters is created using lowercase letters. Subsequently, the domain “@example.com” is appended to produce a completely random email address.
By the way, check out if you are keen to know more about random numbers in Python.
Using the Faker library
The Faker library is a 3rd party library that provides functions for creating dummy data, such as names, addresses, and email IDs. We can use the faker.Faker()
function to create a Faker object, which we can then use to generate random email addresses.
Approach: We’ll install and employ the Faker library to enable random email generation. The below code helps to illustrate the same.
from faker import Faker
def generate_random_email():
dummy = Faker()
return dummy.email()
# Example usage
random_email = generate_random_email()
print(random_email)
Explanation: By utilizing the Faker library, the above code produces random email IDs that are more real, complete with appropriate domains.
You may even like this tutorial on how to generate a list of random integers in Python.
Using UUID and domain
Forming unique email addresses by combining a UUID (Universally Unique Identifier) with a domain.
Approach: We’ll use the uuid
module to generate a UUID and then combine it with a fixed domain. For instance, check the following code.
import uuid
def generate_random_email_uuid():
domain = "@example.com"
unique_id = str(uuid.uuid4()).replace("-", "")[:10]
return unique_id + domain
# Example usage
random_email = generate_random_email_uuid()
print(random_email)
Explanation: This code employs the uuid
module to create a unique identifier, shortening it to the first 10 characters, and finally appending the domain to craft a random email address.
Using Markov chains
In contrast to previous approaches, Markov chains can also generate email addresses for a more human-like touch.
Approach: We’ll build a Markov chain model based on existing email IDs to create new ones.
import random
from collections import defaultdict
def build_markov_chain(emails):
chain = defaultdict(list)
for email in emails:
for i in range(len(email) - 1):
chain[email[i]].append(email[i + 1])
return chain
def generate_random_email_markov(chain, length=10):
start = random.choice(list(chain.keys()))
email = start
for _ in range(length - 1):
next_char = random.choice(chain[email[-1]])
email += next_char
return email + "@example.com"
# Example usage
sample_emails = ["john@example.com", "mary@example.com", "alex@example.com"]
markov_chain = build_markov_chain(sample_emails)
random_email = generate_random_email_markov(markov_chain)
print(random_email)
Explanation: This code creates a Markov chain model from a list of sample emails. It then creates random email addresses by predicting the next character based on the previous one. The result is more human-like email ids.
Other ways to generate random email
In addition to the methods you have seen, there are some more ways to do it. Here are a few examples:
- Using a random number generator to produce the user name and domain name.
- Create a dictionary of real email IDs and randomly select one from it.
- Using a combination of the above methods.
- Also, many web services are commonly available that can help you with this task.
Finally, the best way to generate random emails depends on your specific needs. If you need a large number of random email IDs, look for a web service that can scale. Instead for shorter tasks, using the random module or the Faker library is easier.
Don’t leave without checking out this simple program to generate random integer numbers.
Conclusion: Python to Generate Random Email
At this point, you must know how useful can generating random emails be. And more importantly, you now know more than one way to do it. However, the method you choose depends on your specific needs.
Before we close, let us remind you to use this information wisely. It is important to be aware of the negative impact that can occur if it is not used with the correct intentions. If you have any questions, please feel free to ask.
Happy Learning!