It is quite exciting to generate random images using the Python code. This tutorial will walk you through various methods for creating random images, including code examples and their comparisons. We will cover methods such as the Pillow library, NumPy, generative adversarial networks (GANs), and more. Once you are through with this tutorial, you’ll have a better grasp of the techniques for generating random images. You can then decide which method best suits your specific use case.
Also Read:
Python to Generate Random Email
Python to Generate Random IP Address
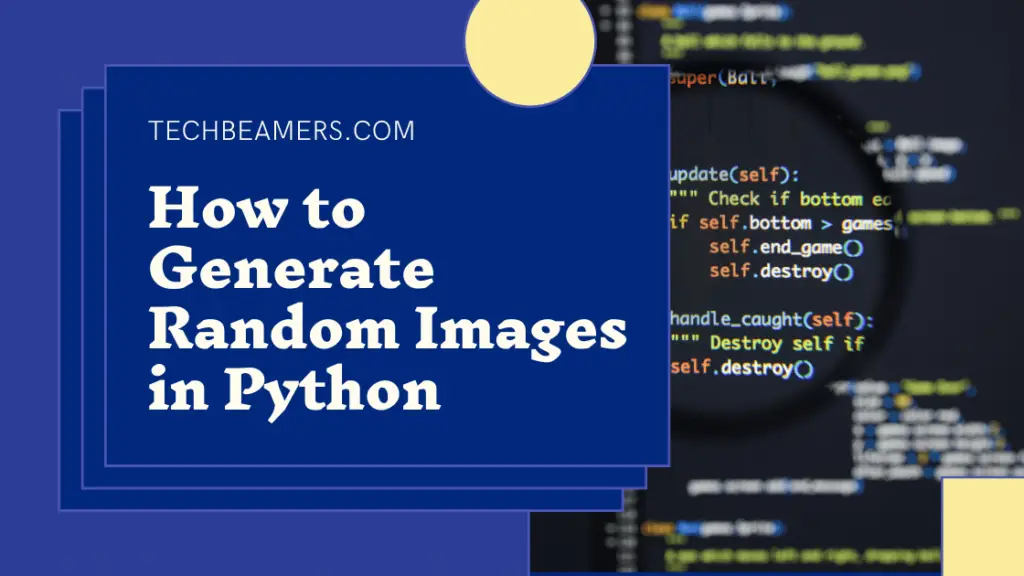
3 Ways to Generate Random Images in Python
In this tutorial, we’ll explore different methods to generate random images in Python. We will cover the following techniques:
- Pillow Library: Generate random pixel data using the Python Imaging Library (Pillow).
- NumPy and Matplotlib: Create random images with NumPy arrays and visualize them using Matplotlib.
- Generative Adversarial Networks (GANs): Explore more advanced techniques to generate images with GANs.
- Comparing different methods: Let’s compare the pros and cons of each method and advise on the most friendly approach.
Method 1: Pillow Library
Pillow is a powerful Python Imaging Library that provides various functions to generate random images in Python. You can install it using pip
:
pip install pillow
Here’s an example of how to generate a random image using Pillow:
from PIL import Image, ImageDraw
import random
# Create a blank image with a white bg
width, height = 800, 600
image = Image.new("RGB", (width, height), "white")
draw = ImageDraw.Draw(image)
# Generate and test random pixels
for _ in range(10000):
x = random.randint(0, width - 1)
y = random.randint(0, height - 1)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
draw.point((x, y), fill=color)
# Save the image
image.save("test_img_pillow.png")
Method 2: NumPy and matplotlib
NumPy is famous for large calculations and Matplotlib provides tools for presenting the data. We can combine these libraries to generate and display random images:
import numpy as np
import matplotlib.pyplot as plt
# Create a random image
width, height = 800, 600
random_image = np.random.randint(0, 256, (height, width, 3), dtype=np.uint8)
# Display the image
plt.imshow(random_image)
plt.axis('off')
plt.show()
# Save the image
plt.imsave("test_img_numpy.png", random_image)
Method 3: Generative Adversarial Networks (GANs)
GANs are an advanced way to generate real images. Building GANs is beyond the scope of this tutorial. Still, we’ll introduce you to the concept and provide some code as a starter.
GANs consist of two neural networks: a generator and a discriminator. The generator creates images, and the discriminator tries to filter real images from fake ones. With training, the generator becomes increasingly proficient at generating random images.
To get going with GANs, consider using libraries like TensorFlow and PyTorch. Here’s a basic example using PyTorch. Before using the below code, please make sure you have added these packages to your Python installation.
import torch
import torch.nn as nn
import torch.optim as optim
import matplotlib.pyplot as plt
# Let's write a simple GAN class
class TestImage(nn.Module):
def __init__(self):
super(TestImage, self).__init__()
self.fc1 = nn.Linear(100, 128)
self.fc2 = nn.Linear(128, 3)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.sigmoid(self.fc2(x))
return x
# Initialize the generator
test_gen = TestImage()
# Generate a random image
noise = torch.randn(1, 100)
random_image = test_gen(noise).detach().numpy().reshape(1, 3, 1)
# Display the image
plt.imshow(random_image)
plt.axis('off')
plt.show()
For a more in-depth GAN project, consider reading more stuff, and libraries specific to GAN development.
Comparing different ways to generate a random image
Let’s compare the methods based on various factors:
Method | Ease of Use | Customization | Realism | Speed |
---|---|---|---|---|
Pillow Library | Easy | Limited | Low | Fast |
NumPy and matplotlib | Moderate | High | Low | Fast |
Generative Adversarial Networks | Advanced | High | High | Slow |
Ease of Use: Pillow is the easiest to start with, while GANs are difficult. They require knowledge of neural networks and deep learning frameworks.
Customization: NumPy and Matplotlib provide greater control over image customization with pixel-level editing. GANs offer the highest level of customization, allowing you to generate highly realistic images tailored to your specific needs.
Realism: GANs are capable of generating highly realistic images, making them fit for tasks where visual fidelity is crucial. Pillow and NumPy approaches generate more abstract or random images.
Speed: Pillow and NumPy-based methods are somewhat faster than GANs, which can take hours or days to train and generate high-quality images.
Advice on Suitable Method
The choice of method depends on your specific requirements:
- Pillow Library: If you need quick, abstract, and simple random images for prototyping or basic visualizations, Pillow is a straightforward choice.
- NumPy and Matplotlib: This method works well if we want to make some customization and have control over the randomness. It is a good middle ground, setting a balance between ease of use and customizability.
- Generative Adversarial Networks (GANs): Use GANs when you need highly realistic images, such as for image synthesis, style transfer, or generative art. However, get ready for a steeper learning curve and the computational demands of training a GAN.
Your choice depends on your project’s specific goals and your expertise in the respective methods. Each method has its unique advantages and limitations. Also, remember that there are more ways to generate random images. So, keep exploring and learning. It’s not the end of the world.