This tutorial explains Python List Comprehension, the fastest way to create, iterate, and return new lists with elements filtered using conditions made of a boolean expression or if-else.
How to Use List Comprehension in Python
You may find it similar to the Python filter() method that filters values based on some condition. However, list comprehension has a much more powerful syntax which gives options to add expressions and use if statements.
First, let’s answer this question: Why use List Comprehension?
The main reason is that it makes you write less code. It prevents you from using a for loop to go through the list.
That’s correct, but there are more benefits. For instance, it’s faster because it optimizes operations that happen right within the List Comprehension itself.
This inlining of operations reduces function call overhead. And as you use it, you might discover even more advantages. It is a unique one-liner coding technique in Python. Let’s dig into it quickly.
Please go through each section so that you can understand it in depth. And it shouldn’t take more than two minutes to read. Also, there is a short quiz/exercise given at the end of the tutorial.
What is List Comprehension in Python?
Python List Comprehension is an inline way of writing logic to search the existing list, do some operations on it, and return a new list.
You can achieve the same effect by using a for loop or the filter function. But it is only list comprehension which can do it with one line of code.
Basic Python List Comprehension
Look at its signatures below:
# Basic Syntax - Python List Comprehension [new_list] = [expr1 FOR element IN in_list]
It is the most basic form of list comprehension in Python. The ideal use case to apply it is when you want to perform some operations on list elements.
See this example where we are converting each element of a list to upper case using list comprehension.
# Using Python List Comprehension langs = ['python', 'csharp', 'go', 'javascript', 'php', 'mysql'] langs = [item.upper() for item in langs] print(langs)
The output is:
['PYTHON', 'CSHARP', 'GO', 'JAVASCRIPT', 'PHP', 'MYSQL']
Advanced Python List Comprehension If
# Advanced Syntax - Python List Comprehension If out = [expr1 for element in in_list if <condition> OR/AND expr2]
Note:
With this format, you can add an if condition with Python list comprehension and join it with OR and AND clauses.
The FOR (for), IN (in), IF (if), and OR/AND (or/and) are reserved keywords in Python. Rest others like new_list, expr1, element, condition, in_list, and expr2 are either variable names or expressions.
The syntax itself is quite explanatory. It saves you from writing multiple lines of code (such as a loop requires) and improves readability.
Let’s take an example where we have a list of fruits. We have to form a new list that contains only fruit names starting with a vowel.
# Using Python List Comprehension If condition # Find fruits starting with a vowel fruits = ['Apples', 'Oranges', 'Guavas', 'Grapes', 'Mangoes', 'Apricots', 'Olives'] fruits = [fruit for fruit in fruits if fruit[0].lower() in ['a', 'e', 'i', 'o', 'u']] print(fruits)
The result is:
['Apples', 'Oranges', 'Apricots', 'Olives']
Since list comprehension is related to the list data structure, you must also know about them. Check out the below tutorial.
Python List Comprehension If Else
To use if else with Python list comprehension, the following is the syntax, you can try:
# Advanced Syntax - Python List Comprehension If Else out = [expression_if_true if condition else expression_if_false for item in iterable]
As an illustration, assume we have a list of integers and strings. With the help of Python list comprehension if else, for each value in the list, do the following:
- If the value is an integer, return it as is.
- If it is a string, return -1.
Here is the code to achieve this by using the list comprehension if else:
my_list = [1, 2, "python", 3, 4, 5, "comprehension"] print([x if isinstance(x, int) else -1 for x in my_list]) # [1, 2, -1, 3, 4, 5, -1]
Also Check: List in Python with Examples
Let’s now see some practical things with the help of examples.
List Comprehension Examples
While using Python list comprehension, you should be wise enough to use it. In some cases, it is better to write more code to maintain readability.
Let’s assume that you have a list of 1000 integers which has both odd and even numbers. Below is the test input list.
# list of integers for the test list_of_ints = range(1, 1000)
We used the Python range() function to generate 1K numbers.
You’ll now have multiple tasks at hand. And you should be using the list comprehension technique.
Example-1
Your first task is to create a list of even integers using the list comprehension method.
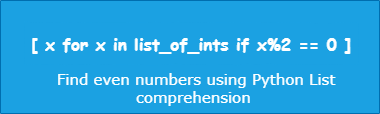
""" Desc: Program to filter even numbers from a list of 1000 integers Method: By using Python list comprehension """ # list of 1000 integers for the test list_of_ints = range(1, 1000) def test_ListComprehension(): # let's prepare the list comprehension list_of_even_nums = [x for x in list_of_ints if x%2 == 0] return list_of_even_nums if __name__ == '__main__': out = test_ListComprehension() # print list of even numbers and its size print("Found {} even numbers: {}".format(len(out), out))
Below is the output captured after the execution of the above code. We used the Python string format() function to print the output dynamically.
Found 499 even numbers: [2, 4, 6, 8, 10, ..., 998]
Note: We didn’t show the full output as it was a lot to display 499 odd numbers.
Example-2
Your second assignment is to compute the performance of list comprehension vs. for loop. So, you’ll be writing two functions and using the Python time module to measure their execution time.
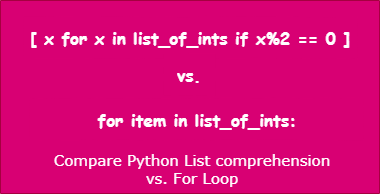
""" Desc: Program to measure performance of list comprehension vs. for loop Method: Using list comprehension and for loop """ import time as t # list of 1000 integers for the test list_of_ints = range(1, 1000) def test_ListComprehension(): # let's prepare the list comprehension list_of_even_nums = [x for x in list_of_ints if x%2 == 0] return list_of_even_nums def test_ForLoop(): # using for loop to find out even numbers even_nums = [] for item in list_of_ints: if item%2 == 0: even_nums.append(item) return even_nums if __name__ == '__main__': # measure performance of list comprehension start = t.time() out = test_ListComprehension() end = t.time() # print list of even numbers and its size print("Found {} even numbers in {}s: {}".format(len(out), round(end - start, 6), out)) # measure performance of for loop start = t.time() out = test_ForLoop() end = t.time() # print list of even numbers and its size print("Found {} even numbers in {}s: {}".format(len(out), round(end - start, 6), out))
Since we have to measure the performance of the two functions, let’s run the program several times.
The first iteration result is:
Found 499 even numbers in 0.000176s: [2, 4, 6, 8, 10, ..., 998] Found 499 even numbers in 0.000291s: [2, 4, 6, 8, 10, ..., 998]
The second iteration result is:
Found 499 even numbers in 0.000208s: [2, 4, 6, 8, 10, ..., 998] Found 499 even numbers in 0.000291s: [2, 4, 6, 8, 10, ..., 998]
And finally, the third iteration result is as follows:
Found 499 even numbers in 0.00021s: [2, 4, 6, 8, 10, ..., 998] Found 499 even numbers in 0.000279s: [2, 4, 6, 8, 10, ..., 998]
It is quite evident from the results that List Comprehension was quite faster than the for loop in creating a new list of integers.
In the next task or example, we’ll see the next level of Python List Comprehension.
Also Read: Python for loop
Example-4
Make a single list comprehension to return two lists, one for even and another for odd numbers.
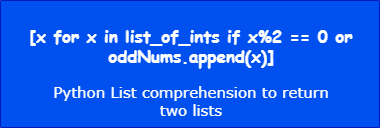
Yes. Don’t worry. It is 100% doable, and let’s see how to achieve it.
""" Desc: Program to find odd and even numbers from a list of integers Method: By using a single Python list comprehension """ # list of 1000 integers for the test list_of_ints = range(1, 1000) oddNums = [] evenNums = [] def test_ListComprehension(): # let's prepare the list comprehension return [x for x in list_of_ints if x%2 == 0 or oddNums.append(x)] if __name__ == '__main__': evenNums = test_ListComprehension() # print list of even numbers and its size print("Found {} even numbers: {}".format(len(evenNums), evenNums)) # print list of odd numbers and its size print("Found {} odd numbers: {}".format(len(oddNums), oddNums))
Here is the outcome of the above program:
Found 499 even numbers: [2, 4, 6, 8, 10,..., 998] Found 500 odd numbers: [1, 3, 5, 7, 9, 11, ..., 999]
We haven’t finished yet. You need to go over one more task to get through.
Example-5
Here, you need to find even numbers from a list of lists of integers. We are giving a clue which is to use nested list comprehension.
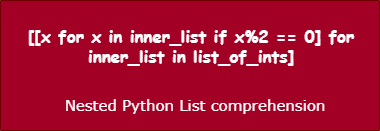
It is not at all tough to create a nested comprehension. First, write a regular LC and then wrap it with an outer LC.
""" Desc: Program to find even numbers from a list of list of integers Method: By using nested Python list comprehension """ # list of lists of integers for the test list_of_ints = [[1, 2, 3, 4], [2, 5, 6], [7, 8, 9, 10]] def nested_ListComprehension(): # let's prepare the nested list comprehension return [[x for x in inner_list if x%2 == 0] for inner_list in list_of_ints] if __name__ == '__main__': evenNums = nested_ListComprehension() # print list of even numbers and its size print("Found {} list of even numbers: {}".format(len(evenNums), evenNums))
The output is:
Found 3 list of even numbers: [[2, 4], [2, 6], [8, 10]]
Lastly, it is essential to discuss when not to use the Python list comprehension.
When should you not use the LC?
The list comprehension works like magic once you get used to it. However, there are scenarios where using it doesn’t make sense.
One such use case is when you have more than one condition to operate. The readability would go down as the number of if…else grows.
lucky_number = [x for x in range(1, 1000) if x%2 != 0 and x > 99 and x not in range(500, 1000)]
Another point is when you need to raise some exception or add a Python try-except block. For example, the Python list comprehension won’t work in the below case.
alphabets = ['P', 'Y', 'T', 'H', 'O', 'N', 3, 6] print(alphabets) lower_case = [x.lower() for x in alphabets] print(lower_case)
The code is valid, but when you run it, it fails with AttributeError. You can’t change the case of a number. So, rather handle it via for loop.
alphabets = ['P', 'Y', 'T', 'H', 'O', 'N', 3, 6] print("Pre:- ", alphabets) lower_case = [] for alphabet in alphabets: try: out = alphabet.lower() lower_case.append(out) except AttributeError: lower_case.append(alphabet) print("Post:- ", lower_case)
Here is the result:
Pre:- ['P', 'Y', 'T', 'H', 'O', 'N', 3, 6] Post:- ['p', 'y', 't', 'h', 'o', 'n', 3, 6]
Self-Assessment Test
Now, face this quick Python list comprehension quiz. It’ll help you remember the important points you learned from this tutorial.
1. What is the result of the code shown below?
import math [str(round(math.e)) for i in range (1, 5)]
A. [‘3’, ‘3’, ‘3’, ‘3’, ‘3’, ‘3’]
B. [‘2.7’, ‘2.71’, ‘2.718’, ‘2.7182’, ‘2.71828’, ‘2.718281’]
C. [‘3’, ‘3’, ‘3’, ‘3’, ‘3’]
D. [‘2.7’, ‘2.71’, ‘2.718’, ‘2.7182’, ‘2.71828’]
E. [‘2’, ‘2’, ‘2’, ‘2’, ‘2’]
2. What is the result of this list comprehension expression?
val=[11.0] [round((i-11)*3/6) for i in val]
A. [0]
B. 0
C. [0.0]
D. Error
3. What is the result of the code given below?
print([char.upper() for char in "python"])
A. [‘PYTHON’].
B. ‘PYTHON’
C. [‘P’, ‘Y’, ‘T’, H’, ‘O’, ‘N’].
D. PYTHON
4. What is the result of this simple Python list comprehension?
langs = ['Python', 'Csharp', 'JavaScript'] langs_i = [lang.lower() for lang in langs] print(langs_i[2][0])
A. None
B. p
C. c
D. j
5. What is the result of the code given below?
vec = [[11, 21, 31], [42, 52, 62], [73, 83, 93]] [vec[i][len(vec)-1-i] for i in range(len(vec))]
A. [11, 52, 93]
B. [31, 52, 73]
C. [42, 52, 62]
D. [21, 52, 83]
We hope that after wrapping up this tutorial, you are now comfortable with Python list comprehension. However, you may practice more with the given examples and gain more confidence.