Python Time & Datetime are the primary Python modules to program date and time. In this tutorial, we’ll explore the Python time module, will see examples, and write code to perform time operations in Python.
In Python, time and date-time both are objects, not a plain string or a timestamp. So to handle them in a program, you need to import the respective module or the class from the module.
Also, while programming with time and datetime classes, you may need to set an appropriate time zone. Hence, we’ll cover the time zone-related functionality as well in this tutorial.
Let’s begin this tutorial and explore the essential Python time functions, structures, and their usage.
Get Started with the Python Time Module
Every computer system comes with a clock pre-programmed to a specific date, time, and time zone. Python time module provides the ability to read, represent, and reset the time information in many ways. We’ve covered all these essential ways and demonstrated them with examples. Below, we’ve captured the summary of this article so that you cannot even miss a part.
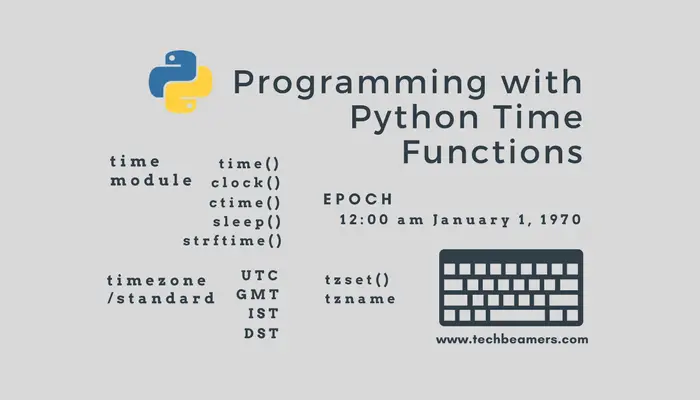
Here are some of the essential facts about the Time module.
- The time module wraps around the C runtime library to provide functions for time manipulation. It has a set of functions that you can call to deal with the system time.
- This module follows the EPOCH convention which refers to the point where the time starts. It supports the dates and times from the epoch to the year 2038.
- The Unix EPOCH is midnight on 12:00 am January 1, 1970. To determine the EPOCH value on your system, use the following code.
>>> import time >>> time.gmtime(0) time.struct_time(tm_year=1970, tm_mon=1, tm_mday=1, tm_hour=0, tm_min=0, tm_sec= 0, tm_wday=3, tm_yday=1, tm_isdst=0)
Note – The function time.gmtime()
returns a struct_time object.
- The term “Seconds since the Epoch” or the “No. of Ticks since epoch” represents the time (in seconds) that elapsed since the epoch.
A tick refers to a time interval which is a floating-point number measured as units of seconds.
- Some time functions return time in DST format. DST is the Daylight Saving Time. It is a mechanism that moves the clocks forward by 1 hour during the summertime, and back again in the fall.
Useful Functions in Python Time Module.
Following are the most used functions and the data structure of the time module in Python which you must know.
1. time.time()
function
#Syntax time.time()
The time() is a core function of the time module. It measures the number of seconds since the epoch as a floating point value. Let’s take a look:
import time print('The current time is:', time.time()) #Output - The current time is: 1504634843.1003768
The time() function as shown in the above example returns time in Decimal format. It could be useful for storing or comparing dates but not suitable for displaying in reports.
Another common application of the Python time function is to measure the elapsed Wall-clock time between two points.
Note – Wall clock time is the actual time lapsed in completing a job. It is the same as timing your job with a stopwatch.
Example – time() to measure the time elapsed (Wall clock time)
import time start = time.time() print("I'm doing a job.") time.sleep(0.1) end = time.time() print(end - start)
2. time.clock()
function
#Syntax time.clock()
Unlike the time.time() function which computes the wall clock time, the time.clock() returns the processor clock time.
We can use time.clock() for performance testing/benchmarking and time.time() to schedule something.
The output of the clock() function reflects the right time taken by the program. And it is more accurate than its counterpart.
However, its exact behavior may vary with the OS you are using. It could return process time ignoring the time to sleep. And in some cases, it could count the time elapsed even when the process was inactive.
Note – The processor clock does not tick if a program is in the sleep state.
Let’s see an example to demonstrate what happens when we use both time functions.
Example –
import time template = 'time()# {:0.2f}, clock()# {:0.2f}' print(template.format(time.time(), time.clock())) for i in range(3, 0, -1): print('>Sleeping for', i, 'sec.') time.sleep(i) print(template.format(time.time(), time.clock()) )
Output –
Python 2.7.10 (default, Jul 14 2015, 19:46:27) [GCC 4.8.2] on linux time()# 1504977071.40, clock()# 0.10 ('>Sleeping for', 3, 'sec.') time()# 1504977074.40, clock()# 0.10 ('>Sleeping for', 2, 'sec.') time()# 1504977076.40, clock()# 0.10 ('>Sleeping for', 1, 'sec.') time()# 1504977077.40, clock()# 0.10
Please note that Python 3.3 has deprecated the clock() function and introduced two functions i.e. time.perf_counter() and time.process_time().
time.perf_counter() – It uses a clock with the highest precision to measure a short duration and factors in the process of sleep time.
time.process_time() – Its output is the sum of the system and user CPU time of the current process. It ignores the time elapsed during sleep.
3. time.ctime()
function
#Syntax time.ctime(seconds)
It takes the time in “seconds since the epoch” as input and translates into a human-readable string value as per the local time. In case of no argument, it calls the time()
function and returns the current time.
We can even use it intuitively. Check out the below example.
Example –
import time print('The current time is :', time.ctime()) newtime = time.time() + 20 print('20 secs from now :', time.ctime(newtime))
Output –
The current time is : Sun Sep 10 00:02:41 2017 20 seconds from now : Sun Sep 10 00:03:01 2017
Please note that the length of the string returned by time.ctime()
is 24 characters.
4. time.sleep()
function
#Syntax time.sleep(seconds)
It halts the execution of the current thread for the specified number of seconds. You can pass a floating point value as the argument to register a more precise sleep time.
However, the program may not suspend for the exact time as any caught signal will end the sleep. Also, the sleep time may be extended due to the scheduling of other activities in the system.
With the sleep()
function, our program/script can pause for a given number of seconds. It is a useful function in cases like when I need to wait for a file to finish closing or let a database commit happen.
Let’s have a look at the below example. Open a new window in IDLE or your IDE and save the following code:
Example –
# importing "time" module for time operations import time # using ctime() to display present time print ("Begin Execution : ",end="") print (time.ctime()) # using sleep() to suspend execution print ('Waiting for 5 sec.') time.sleep(5) # using ctime() to show present time print ("End Execution : ",end="") print (time.ctime())
Output –
Begin Execution : Sun Sep 10 00:27:06 2017 Waiting for 5 sec. End Execution : Sun Sep 10 00:27:12 2017
Note – In Python 3.5, the sleep()
function continues irrespective of the interrupt caused by a signal. However, it breaks if the signal handler throws an exception.
5. time.struct_time
class
# Python time class time.struct_time
The time.struct_time is the only data structure present in the time module. It has a named tuple interface and is accessible via the index or the attribute name.
This class is useful when you need to access the specific fields of a date (year, month, etc.). There are a number of functions (like localtime()
, gmtime()
) that return the struct_time objects.
Example –
# importing "time" module for time operations import time print(' Current time:', time.ctime()) t = time.localtime() print(' Day of month:', t.tm_mday) print(' Day of week :', t.tm_wday) print(' Day of year :', t.tm_yday)
Output –
Current time: Sun Sep 10 01:19:25 2017 Day of month: 10 Day of week : 6 Day of year : 253
6. time.strftime()
function
#Syntax time.strftime(format[, t])
This function takes a tuple or struct_time in the second argument and converts it to a string as per the format specified in the first argument. If t is not present, then it calls the localtime()
to use the current date.
Here’s a simple example.
Example –
import time now = time.localtime(time.time()) print(time.asctime(now)) print(time.strftime("%y/%m/%d %H:%M", now)) print(time.strftime("%a %b %d", now)) print(time.strftime("%c", now)) print(time.strftime("%I %p", now)) print(time.strftime("%Y-%m-%d %H:%M:%S %Z", now))
Output –
Sun Sep 10 01:36:28 2017 17/09/10 01:36 Sun Sep 10 Sun Sep 10 01:36:28 2017 01 AM 2017-09-10 01:36:28 India Standard Time
In the first few lines, we are calling two time-related functions. The localtime()
function creates a struct_time object using the time argument. Whereas the asctime()
function converts a struct_time object into a time string.
Thereafter, you can see how the strftime()
function is slicing up the time and returns a formatted string.
Work with Time Zones in Python.
Time zone is a term commonly used with time. It represents a region where the same standard time is used for legal, commercial, and social purposes. There are 24 time zones in the world.
What exactly is UTC? Is it a time standard or a time zone?
UTC is an acronym for Coordinated Universal Time. And it is a time standard, not a time zone by which the world regulates clocks and time.
What are GMT and IST? What is the difference?
The GMT refers to Greenwich Mean Time and IST is a term used to represent Indian Standard Time. Both are time zones.
Note – GMT and IST contrast by +5.30 hours. Also, IST applies to India whereas GMT/UTC is applicable to the world.
Check Timezone in Python.
The time module has the following two properties that will give you the timezone info.
1. time.timezone
– It returns the offset of the local (non-DST) timezone in UTC format.
>>> time.timezone -19800
2. time.tzname
– It returns a tuple containing the local non-DST and DST time zones.
>>> time.tzname ('India Standard Time', 'India Daylight Time')
Set Timezone in Python.
time.tzset() function
– It resets the Timezone for all library functions and uses the environment variable TZ to set the new value.
The limitation is that it works only on Unix-based platforms.
Please see the below example for clarity on its usage.
TZSET Example.
# Set timezone on a unix system import os, time print('Current timezone is', time.tzname) os.environ['TZ'] = 'Europe/London' time.tzset() print('New timezone is', time.tzname)
#Output
Python 3.6.1 (default, Dec 2015, 13:05:11) [GCC 4.8.2] on linux Current timezone is ('UTC', 'UTC') New timezone is ('GMT', 'BST')
Quick Wrapup – Python Time and Its Usage.
After reading this tutorial, you might now have a fair idea of performing time operations using the Python time module. It has a lot of functions to work with time. However, we covered the most relevant of them so that you learn fast and still can use them efficiently.
In the next tutorial, we’ll take up the Python date-time module, its most useful functions, and usages with practical examples.
If you liked this post and are interested in seeing more such posts, then follow us on our social media accounts and be sure to receive all future updates.
Best,
TechBeamers.