In this tutorial, you’ll learn everything about AngularJS expressions. These are one of the core building blocks in Angular which bind model data to HTML DOM elements.
We’ve added a number of AngularJS examples with each type of expression to bring more clarity. Mainly, there are four types of AngularJS expressions which symbolize types like Numbers, Strings, Arrays, and Objects.
We’ll start this tutorial with two AngularJS examples using the expressions and cover the details in the later sections of this tutorial.
If you like to quickly brush up on Angular concepts, then do go through the below post once.
Learn AngularJS Expressions
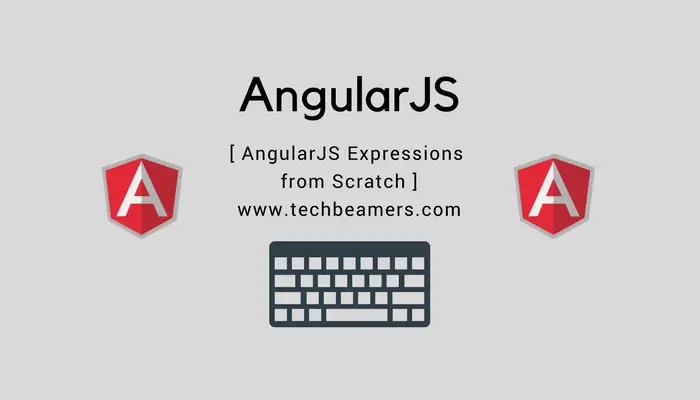
AngularJS expressions can be written inside double braces: {{ expression }}. It can also be written inside a directive as <ng-bind=”expression”>. After evaluating the Expression, AngularJS displays its value in the HTML, in place of Expression.
Expressions used in AngularJS applications are similar to Javascript Expressions. They can contain literals, operators, and variables.
For Example {{ 3 + 4 }} or {{ firstName + " " + lastName }}
Syntax-
<script>
<p> {{ expression }} </p>
</script>
Example-1
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Expression Example</title>
<script
src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.js">
</script>
</head>
<body>
<h1>AngularJS Expression Demo:</h1>
<div ng-app="">
<h3> Value of the Expression (10*10) is: {{ 10 * 10 }} </h3>
</div>
</body>
</html>
It will give the following output.
AngularJS Expression Demo:
The value of the Expression (10*10) is: 100
The above example defines the <ng-app> directive as blank. It means that in this particular example, the module is not declared to assign controllers, directives, and services attached to the code.
If we remove the <ng-app> directive from the above code, HTML displays the Expression as it is, without evaluating it.
Example-2
<!DOCTYPE html>
<html>
<head>
<title>AngularJS Expression Example</title>
<script
src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.js">
</script>
</head>
<body>
<div>
<h1>AngularJS Expression printed as it is on removing the "ng-app" directive:</h1>
<h3> Value of the Expression (10*10) is: {{ 10 * 10 }} </h3>
</div>
</body>
</html>
Output.
AngularJS Expression printed as it is on removing the “ng-app” directive:
Value of the Expression (10*10) is: {{ 10 * 10 }}
An expression either assigns a value to a variable or will output its value in its place.
Types of AngularJS Expressions.
In AngularJS, Expressions are categorized, into the following types.
1. Number Expressions
2. String Expressions
3. Object Expressions
4. Array Expressions
Let’s follow up on each of these one by one.
Number Expressions.
An Expression that uses numbers and operators < -, +, *, and % > are number Expression. Let’s see an example to multiply the number of apples <num> with the cost of a single apple <cost>, to get the amount required to purchase them.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.min.js"></script>
</head>
<body>
<h1> AngularJS Number Expression Example</h1>
<div ng-app='' ng-init="num=10;cost=50">
<p>Total purchase price of apples is: {{ num * cost }}</p>
</div>
</body>
</html>
In the above code,
- AngularJS defines variables using the <ng-init> directive. We can also assign values to them. It is similar to defining local variables, in other programming languages. In this example, we are defining two variables <num> and <cost>.
- We use these variables by multiplying them.
If the above code, executes successfully(when we run the code in the browser), the following output gets displayed.
AngularJS Number Expression Example
The total purchase price of apples is: 500
String Expressions.
It performs operations on String values.
Let’s take an example, where we will define two Strings and print them.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.min.js"></script>
</head>
<body>
<h1> AngularJS String Expression Example</h1>
<div ng-app="" ng-init="messageOne='Welcome, Viewers to';messageTwo='TechBeamers !!'">
<p>{{ messageOne + " " + messageTwo }}</p>
</div>
</body>
</html>
Here, we have defined two variables, <messageOne> and <messageTwo>. We concatenate the Strings and print their values using the expression, {{ messageOne + ” ” + messageTwo }}. The following output is displayed.
AngularJS String Expression Example
Welcome, Viewers to TechBeamers !!
Object Expressions.
It holds object properties and evaluates at the view, where we use it. It is similar to JavaScript objects that consist of name-value pairs.
Let’s take an example where we will define an object <student> which will have 4 key-value pairs, “studentName”, “studentClass”, “studentRoll”, and “studentPercent”.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.min.js"></script>
</head>
<body>
<h1> AngularJS Object Expression Example</h1>
<div ng-app="" ng-init="student={studentName:'John',studentClass:'Fifth',studentRoll:'15',studentPercent:'94%'}">
<p> Student Name: {{ student.studentName }}</p>
<p> Percentage: {{ student.studentPercent }}</p>
</div>
</body>
</html>
Here, we use the <ng-init> directive to define the object <student>. It has the following key-value pairs, <studentName> with the value <John>, <studentClass> with the value <Fifth>, <studentRoll> with the value <15>, and <studentPercent> with the value <94%>.
We then use expressions {{student.studentName}} and {{ student.studentPercent }} to access the value of these variables and display them in the view accordingly. Since the member variables are part of the object <student>, we use the dot (.) notation to access its actual value.
The following output is displayed.
AngularJS Object Expression Example
Student Name: John
Percentage: 94%
Array Expressions.
The Array Expression refers to an array of object values. Let’s take an example, where we will define an array, that stores the marks of a student in five subjects. We will then print these values using the Array Expression.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.4/angular.min.js"></script>
</head>
<body>
<h1> AngularJS Array Expression Example</h1>
<div ng-app="" ng-init="marks=[95,92,85,89,90]">
Marksheet John:<br>
Marks in Mathematics: {{ marks[0] }}<br>
Marks in Science: {{ marks[1] }}<br>
Marks in Hindi: {{ marks[2] }}<br>
Marks in English: {{ marks[3] }}<br>
Marks in Computers: {{ marks[4] }}<br>
</div>
</body>
</html>
Here we use Array Expression, <marks[index]> to access each element of the array. The following output gets printed.
AngularJS Array Expression Example
Marksheet John:
Marks in Mathematics: 95
Marks in Science: 92
Marks in Hindi: 85
Marks in English: 89
Marks in Computers: 90
AngularJS Expression Capabilities and Limitations.
Capabilities.
- AngularJS Expressions are similar to JavaScript Expressions. Hence, both have the same capabilities.
- AngularJS Expressions can contain literals, operators, and variables.
- AngularJS Expression supports the use of filters. Using filters, we can format data before it gets displayed.
- HTML code is not allowed.
Limitations.
- AngularJS Expressions cannot contain conditions, loops, exceptions, and regular expressions.
- We cannot declare functions in an AngularJS Expression, even inside the <ng-init> directive.
- They cannot contain commas or voids.
- They cannot contain the return keyword.
Quick Summary
We’ve tried to provide all the relevant details about the AngularJS expressions in this tutorial. Hope, you could have learned it easily.
If you liked this post and are interested in seeing more such posts, then follow us on our social media accounts.
Best,
TechBeamers.