Have you got an Angular interview lined up? Don’t worry. We’ve got the latest and most relevant AngularJS interview questions and answers compiled in this post. It covers all elementary, intermediate, and advanced-level Angular topics that you might get asked by the interview panel.
Don’t forget that success not only depends on your technical skills but your behavior matters too. Hence, if you want to be a cut above the rest, then prepare in advance, practice more, and perform well. Let’s now move to the AngularJS interview questions section.
100+ AngularJS Interview Questions and Answers
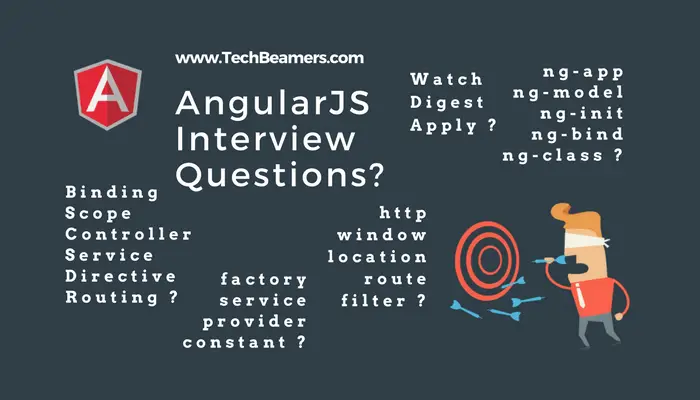
What is AngularJS technology?
AngularJS is a JavaScript-based Web Development Framework for creating dynamic web pages. It incorporates HTML as the template language and enables the developer to extend the HTML tags to represent the application components more clearly.
What type of architecture does AngularJS support?
Angular proposes to create applications based on the MVC architecture. It helps to develop a maintainable solution that is easy to test at the client’s end.
It requires the following three core components.
- Template – the view part
- Scope – the model
- Controller – the control part
AngularJS customizes the HTML attributes with directives and data binding using expressions.
What does Template mean in AngularJS?
The templates are unique HTML components in AngularJS applications. They are more like a static version of a web page with some additional properties to inject and render data at runtime.
What does Scope mean in AngularJS?
A scope is an object that defines the model for an AngularJS application. It provisions data fields to pass in templates for presenting to the user.
It can even bind functions for responding to actions that a user could perform.
What does Controller mean in AngularJS?
The controller takes the form of a function that accepts an empty scope object as the argument and delegates it to the fields and functions for exposure to the user.
What does Directive mean in AngularJS?
AngularJS directives are an extension of the HTML attributes. The following are three standard directives in Angular JS:
- ng-app: It marks the HTML element that Angular intends to be the root element of our application. The custom attributes use spinal-case whereas the corresponding directives follow the camelCase.
- ng-model: It binds values of HTML elements (such as input, select, and textarea) to the application data.
- ng-bind: It binds application modal data to the HTML template view.
What are the main features of AngularJS?
Here is the list of AngularJS features that make it the hottest tech for web dev.
- Data-binding – Handles synchronization of data across models, controllers, and views.
- Scope – The object representing the model, acts as a glue layer between the controller and the view.
- Controllers – These are JS functions bound to the scope object.
- Services – Substitutable objects that are wired together using dependency injection. e.g. $location service.
- Filters – Format the value of an expression for displaying to the user. e.g., uppercase, lowercase.
- Directives – These are extended HTML attributes that start with the “ng-” prefix. e.g., the ng-app directive used to initialize the angular app.
- Templates – HTML code including AngularJS-specific elements and attributes.
- Routing – It’s an approach to switch views.
- MVC pattern – A design pattern made up of three parts.
- Model – Represents data, which could be static data from a JSON file or dynamic data from a database.
- View – Renders data for the user.
- Controller – Gives control over the model and view for collating information to the user.
- Deep linking – Enables the encoding of the application state in the URL and vice versa.
- Dependency injection – A design pattern to let the components injected into each other as dependencies.
Why would you as a developer choose AngularJS?
Following are some of the key reasons to choose AngularJS as your web development framework:
- It follows the MVC design pattern which allows segregating an application into different components (called Model, View, and Controller) thus making it easy to maintain.
- It allows the HTML to extend by adding directives to the HTML markup. This feature helps in defining dynamic templates which can include new attributes, tags, and expressions.
- It allows the creation of user-defined directives and reusable components. These directives help the developer to concentrate on creating the logic, thus enabling them to work efficiently.
- It supports two-way data binding, i.e., enables automatic synchronization of data between model and view components. Thus, any update in the model gets reflected in the view automatically. And there is no need to add any Javascript code or event listeners to notify the data changes.
- It encapsulates the behavior of your application in controllers which gets instantiated with the help of dependency injection.
- It supports built-in services to perform routine tasks for web applications. For example, it provides a $http service to communicate with the REST service.
- It makes the development and testing of the application’s JavaScript code easy.
- Also, AngularJS has a mature community to help developers. It has broad support over the Internet.
List down the popular AngularJS IDE Plugins/Extensions for web development.
Here is a list of IDE Plugins and Extensions that can enhance the way you code with AngularJS:
- Sublime Text
- WebStorm
- Eclipse
- Netbeans
- Visual Studio 2012/2013 Express or higher
- TextMate
- Brackets
- ATOM
What are the steps involved in the boot process for AngularJS?
Whenever a web page loads in the browser, the following steps get executed in the background.
- First, the HTML file containing the code gets loaded into the browser. After that, the JavaScript file mentioned in the HTML code gets loaded. It then creates a global object for angular. Now, the JavaScript that displays the controller functions gets executed.
- In this step, AngularJS browses the complete HTML code to locate the views. If the same is available, then Angular links it to the corresponding controller function.
- In this step, AngularJS initiates the execution of required controller functions. Next, it populates the views with data from the model identified by the controller. With this the page is ready.
Which browsers are compatible with AngularJS?
AngularJS works fine with the latest versions of Safari, Chrome, Firefox, Opera 15+, and IE9+ (Internet Explorer). However, below is the recent compatibility chart.
Browser | Compatibility |
Google Chrome | Latest |
Mozilla Firefox | Laest |
Edge | Two most recent major versions IE. |
IE | v11, v10, v9 |
IE Mobile | v11 |
Safari | Two most recent major versions |
iOS | Two most recent major versions |
Android | Nougat (v7.0) Marshmallow (v6.0) Lollipop (v5.0, v5.1) KitKat (v4.4) |
What are Polyfills?
Polyfills are compatibility scripts for Angular support in browsers that don’t have all modern HTML5 or above features.
How do you enable Polyfills?
We can get it enabled from the Angular CLI via the “src/polyfills.ts” file.
This file has got all the mandatory and optional polyfills required to run an AngularJS application.
What are the security features provided by AngularJS?
AngularJS provides built-in protection from the following security flaws.
- It prevents cross-site scripting attacks: Cross-site scripting is a technique where anyone can send a request from the client side and can get confidential information easily.
- It prevents HTML injection attacks.
- It prevents XSRF protection for server-side communication: the “Auth token” mechanism can handle it. When the user logs in for the first time a user ID and password are sent to the server, and it will, in turn, return an auth token. Now, this token does the authentication in future transactions.
What are the security risks that a web developer should manage while developing an AngularJS App?
The following are the most critical web application development flaws that a developer should take care of:
- Injection attack
- Broken authentication and Session management
- Cross-Site Scripting (XSS)
- Insecure direct object references
- Security misconfiguration
- Sensitive data exposure
- Missing function level access control
- Cross-Site request forgery (CSRF)
- Using components posing vulnerabilities
- In-validated redirects and forwards
What are the most common directives used in AngularJS applications?
AngularJS extends the behavior of HTML and DOM elements with new attributes called directives.
The following is the list of AngularJS built-in directives.
- ng-bind – The ng-bind directive tells AngularJS to replace the content of an HTML element with the value of a given variable, or expression.
If there is any change in the value of the given variable or expression, then the content of the specified HTML element will also be updated accordingly. It supports one-way binding only. - ng-model – This directive is used to bind the value of HTML controls (input, select, text area) to application data. It is responsible for linking the view to the model. Directives such as ‘input,’ ‘text area,’ and ‘select’ require it. It supports two-way data binding.
- ng-class – This directive dynamically binds one or more CSS classes to an HTML element. The value of the ng-class directive can be a string, an object, or an array.
- ng-app – Just like the “Main()” function of Java language, this directive marks the beginning of the application to AngularJS’s HTML compiler ($compile). If we do not use this directive first, an error gets generated.
- ng-init – This is used to initialize the application data so that we can use it in the block where it is declared. If an application requires local data like a single value or an array of values, this can be achieved using the ng-init directive.
- ng-repeat – This repeats a set of HTML statements for a defined number of times. The set of HTML statements will be repeated once per item in a collection. This collection must be an array or an object.
We can even create custom directives and use them in our AngularJS Application.
Why are expressions used in AngularJS?
AngularJS binds data to HTML using Expressions. It can be written inside double braces: {{ expression}} or inside a directive as ng-bind=”expression”. AngularJS evaluates the expression and substitutes the result in place of the expression.
AngularJS expressions are much like JavaScript expressions. They can include literals, operators, and variables.
For example –
{{ 2 + 2 }} (numbers) {{Name + " " + email}} (string) {{ Country.Name }} (object) {{ fact[4] }} (array)
What does a Filter do in AngularJS?
A Filter in Angular changes or transforms the data before passing it to the view. These Filters work in combination with AngularJS expressions or directives.
AngularJS uses a pipe character (“|”) to add filters to the expressions or directives.
For example:
<p> {{ bid | currency }} </p>
The above example is an expression enclosed in the curly braces using the currency filter.
It is important to note that filters are case-sensitive.
Which are the filters AngularJS supports?
AngularJS provides the following filters to transform data.
- Currency – It is used to format a number to a currency format.
- Date – It allows date formatting to a specified format.
- Filter – It chooses a subset of items from an array.
- JSON – It formats an object to a JSON string.
- LimitTo – Its purpose is to create an array or string containing a specified number of elements/characters either from the beginning or the end of the source. It depends on the value and sign (positive or negative) of the limit.
- Lowercase – This filter converts a string to lowercase.
- Number – It formats a number as text.
- OrderBy – It enables sorting an array. By default, the sorting of strings happens alphabetically. And the sorting of numbers happens numerically. It also supports a comparator function where we can define what will be counted as a match or not.
- Uppercase – This filter converts a string to uppercase.
What are AngularJS prefixes $ and $$?
To prevent accidental name collisions within the code, AngularJS prefixes the names of public objects with $ and the private ones with $$.
However, we should not use these literals ($ or $$) for any other purposes or reasons.
What are the different ways to invoke a directive?
There are four different ways to invoke a directive in an AngularJS application. They are as follows.
1) As an attribute:
<span my-directive></span>
2) As a class:
<span class="my-directive: expression;"></span>
3) As an element:
<my-directive></my-directive>
4) As a comment:
<!-- directive: my-directive expression -->
What is a Singleton pattern? How does AngularJS use it?
Generally, the singleton pattern is a design approach that allows us to limit the instantiation of a class to have only one object.
In AngularJs, we can use the dependency injection and the services to enable the singleton pattern.
Technically, if we call the “new Class()” two times without following the singleton pattern, the outcome will be two objects of the same class.
Whenever the singleton pattern is enabled, then the class will create the object the first time and return its reference in the next call.
What are the essential characteristics of the AngularJS scope object?
A scope is an application object that behaves as the owner of the app’s variables and functions. It has access to both the View and controller. Thus it works as a medium of communication between both of them. This object contains both data and functions. We can use it to access the model data of the controller.
The following are the essential characteristics of the scope object.
- It allows observers to watch for all the model changes.
- Provides the ability to propagate model changes through the application as well as outside the system to other associated components.
- Scopes allow nesting in such a way that they can isolate functionality and model properties.
- Provides an execution environment in which expressions get evaluated.
What is “$rootScope” in AngularJS?
Every AngularJS application has a “$rootScope” that is the top-most scope created on the DOM element.
An app can have only one $rootScope which shares among all its components. It contains the ng-app directive. Every other scope is its child scope. It can watch expressions and propagate events.
Using the root scope, we can set the value in one controller and read it from the other controller.
What is scope hierarchy in AngularJS? How many scopes can an application have?
Every AngularJS application consists of one root scope but may have several child scopes.
As child controllers and directives create new child scopes, they get attached to the application. These new scopes get added as children of their parent scope.
Similar to DOM, they also create a hierarchical structure.
What are Single page applications (SPA) in AngularJS?
Single-page applications (SPAs) are web applications that fit on a single HTML page. It dynamically updates the web page as the user performs actions on the app.
SPAs use AJAX and HTML to create quick and responsive web apps. A single page load extracts all the web app code (JS, HTML, CSS).
Thus the user navigates to different parts of the application quickly as it happens without refreshing the whole page.
What are the benefits SPA provides?
The main characteristics of Single-Page Applications are as follows.
- Its UI is fast and responsive. Also, the Back/Forward buttons present in the UI work properly.
- It contains more JavaScript code than actual HTML as compared to other applications.
- Dynamic data loading occurs from the server side. The API uses a restful web service with JSON format.
- It allows you to pre-load and cache all the app pages. Thus fewer data download requests are made to the server.
- Applications written in AngularJS are cross-browser compliant. It automatically handles the JavaScript code suitable for each browser.
- Even if the user has lost the internet connection, then also the SPA can work as all the pages load in the starting itself.
What is the difference between $scope and scope?
It is mandatory to use “$scope” while defining a controller. However, the “scope” will be used to create a link function for the custom directive. Both of them refer to the “scope” object in AngularJS. The difference between them is that “$scope” uses dependency injection whereas “scope” does not.
Factory methods like controller, filter, service, etc. receive their arguments via dependency injection (DI). In DI, the order of passing the arguments does not matter.
For example, a controller may have the following definition.
(Let’s set $scope as the first parameter in this case)
myApp.controller('MyController', ['$scope', function($scope, $http) { //rest of the code goes here }
OR ( if the $scope is the second parameter)
myApp.controller('MyController', ['$scope', function($http, $scope) { //rest of the code goes here }
Thus, AngularJS does not care for the position of “$scope” in both cases. It uses the argument name to retrieve an object out of the dependency injection container.
But, in the case of the directive linker function, the position of scope matters, as it does not use DI. The reason is that the supplied arguments get to their caller. In this case, the very first parameter has to be the scope as per AngularJS syntax.
app.directive("myDirective", function() { return { scope: {}; link: function(scope, element, attrs) { // code goes here. } }; });
In the case of non-dependency injected arguments, we can also give them a name as seen in the below example:
app.directive("myDirective", function() { return { scope: {}; link: function(foo, bar, biz) { // code goes here. } }; });
To summarize, in the DI case, we pass the <scope object> as <$scope> whereas, in non-DI cases, the <scope object> is returned either as a scope or with any name.
How does the compilation process happen in AngularJS?
Angular’s HTML compiler allows you to teach the browser, new HTML syntax. It enables the developer to attach new behaviors or attributes to any HTML element called directives. The AngularJS compilation process takes place in the web browser itself. It does not involve any server-side or pre-compilation step.
AngularJS uses the “$compiler” service to compile the angular HTML page. Its compilation begins after the HTML page (static DOM) is fully loaded.
The compilation occurs in two phases.
- Compile – The service first traverses the entire DOM, retrieves all the directives, and generates a linking function.
- Link – It supplements the directives with a scope and generates a live view. Changing either the “Scope” or “View” will have a reciprocal effect.
The concept of compile and link has come from the C language. Here the code is compiled first and then linked.
How is AngularJS compilation different from other JavaScript frameworks?
Popular Javascript frameworks like Backbone and jQuery parse the HTML template as a stream and return the result as a string.
They dump the resulting string into the DOM where we can retrieve it using the innerHTML() method.
On the contrary, AngularJS processes the template differently. It directly works on HTML DOM rather than strings and manipulates it as required. It uses two-way data binding between the model and views to sync the data.
What is the use of ng-view in AngularJS?
The ng-view tag creates a placeholder where an HTML or ng-template view can be placed based on the configuration.
Give an example of ng-view in AngularJS.
Let’s take an example of the ng-view.
<div ng-app = "testApp"> <div ng-view> <!-- Target Html Template here --> </div> </div>
In other words, ng-view is the directive that works as a container for angularJS to switch between views.
What is the purpose of ng-template in AngularJS?
The ng-template directive allows the creation of an HTML page using the script tag. It contains an “id” attribute which is used by $routeProvider to map a view with a controller.
While defining ng-template, it is mandatory to specify the type of the <script> element as the text/ng-template. Also, assign a cache name to the template using the element’s id. Later on, this name gets used as the directive’s templateUrl
.
Provide an example of ng-template in AngularJS.
Following is the syntax of using an ng-template directive in the AngularJS application.
Example-
<div ng-app = "mainApp"> <scrip t type = "text/ng-template" id = "addEmployee.htm"> <h2> Add Employee </h2> {{message}} </scrip t> </div>
$routeProvider part.
var mainApp = angular.module("mainApp", ['ngRoute']); mainApp.config(['$routeProvider', function($routeProvider) { $routeProvider. when('/addEmployee', { templateUrl: 'addEmployee.htm', controller: 'AddEmployeeController' }). otherwise({ redirectTo: '/addEmployee' }); }]);
What is $routeProvider in AngularJS?
The $routeProvider is a primary AngularJS service that sets the configuration of URLs, maps them to the corresponding HTML page or ng-template, and attaches a controller with the same.
Provide an example of the $routeProvider in AngularJS.
Let’s see the following example:
var mainApp = angular.module("mainApp", ['ngRoute']); mainApp.config(['$routeProvider', function($routeProvider) { $routeProvider. when('/addEmployee', { templateUrl: 'addEmployee.htm', controller: 'AddEmployeeController' }). otherwise({ redirectTo: '/addEmployee' }); }]);
The following are some important points to consider for the above example.
- The routeProvider acts as a function under the config of the main app module using a key as ‘$routeProvider.’
- $routeProvider.when defines a URL “/addEmployee” which is then mapped to “addEmployee.htm”. The “addEmployee.htm” should be present on the same path as the main HTML page.
- The “otherwise” clause sets the default view.
- The “controller” clause sets the corresponding controller for the view.
What does data binding mean in AngularJS?
Data binding is the connection bridge between the view and business logic (view model) of the application. It does the automatic synchronization between the model and the view.
When the model changes, the view reflects it automatically and vice versa. AngularJS supports the following two types of bindings:
- One-way and,
- Two-way
What data-binding directives does AngularJS support?
AngularJS provides the following data-binding directives:
- ng-model
- ng-bind
- ng-bind-HTML
- ng-bind-template
- ng-non-bindable
What is the role of the ng-bind directive in AngularJS?
It updates the text content of the specified HTML element with the value of the given expression.
The text content gets updated when there is any change in the expression value. It is very similar to the double curly markup ( {{expr }}) but is less verbose.
It has the following syntax.
<ANY ELEMENT ng-bind="expression"> </ANY ELEMENT>
What does the ng-bind-HTML directive do in AngularJS?
It evaluates the expression and inserts the HTML content into the element in a secure way. To use this functionality, it has to use the $sanitize service. For this, it is mandatory that $sanitize is available.
It has the following Syntax.
<ANY ELEMENT ng-bind-html=" expression "> </ANY ELEMENT>
Why is the ng-bind-template directive used in AngularJS?
The ng-bind-template Directive replaces the element text content with the interpolation of the template. It can contain multiple double curly markups.
The following is the syntax.
<ANY ELEMENT ng-bind-template=" {{expression1}} {{expression2}} … {{expressionn}} "> </ANY ELEMENT>
Why is the ng-non-bindable directive used in AngularJS?
This directive informs AngularJS, not to compile or bind the contents of the current DOM element. It is useful in the case when the user wants to display the expression only and does not want to execute it.
It has the following syntax.
<ANY ELEMENT ng-non-bindable > </ANY ELEMENT>
What is the ng-model directive in AngularJS?
This directive is capable of binding with the input, select, text area, or any custom form control. It provides two-way data binding.
The ng-model directive also provides validation behavior. It also retains the state of the HTML elements (like valid/invalid, touched/untouched, and so on).
It has the following syntax.
<input ng-model="name"/>
What directives are used to show and hide HTML elements in AngularJS?
The directives used to show and hide HTML elements in AngularJS are <ng-show> and <ng-hide>. They do this based on the result of an expression.
Its syntax is as follows.
<element ng-show="expression"></element>
When the expression for <ng-show> evaluates to true, then HTML element(s) are shown on the page, otherwise, the HTML element is hidden. Similarly, the <ng-hide> directive hides the HTML element if the expression evaluates to true.
Let’s take the following example.
<div ng-controller="MyCtrl"> <div ng-show="data.isShow">ng-show Visible</div> <div ng-hide="data.isHide">ng-hide Invisible</div> </div> <script> var app = angular.module("app", []); app.controller("MyCtrl", function ($scope) { $scope.data = {}; $scope.data.isShow = true; $scope.data.isHide = true; }); </script>
Why is the ng-if directive used in AngularJS?
This directive can add/remove HTML elements from the DOM based on the input expression.
If the expression evaluates to true, it adds a copy of HTML elements to the DOM. If the expression evaluates to false, this directive removes the HTML element from the DOM.
<div ng-controller="MyCtrl"> <div ng-if="data.isVisible">ng-if Visible</div> </div> <script> var app = angular.module("app", []); app.controller("MyCtrl", function ($scope) { $scope.data = {}; $scope.data.isVisible = true; }); </script>
What does the ng-switch directive do in AngularJS?
This directive can add/remove HTML elements from the DOM conditionally based on scope expression.
Child elements with the “ng-switch-when” directive will be displayed if it gets a match. Otherwise, the element and its children get removed.
The ng-switch directive also allows defining a default section, by using the “ng-switch-default” directive. It falls back to the default when no other section matches.
Let’s see the following example that displays the syntax for “ng-switch.“
<div ng-controller="MyCtrl"> <div ng-switch on="data.case"> <div ng-switch-when="1">Shown when case is 1</div> <div ng-switch-when="2">Shown when case is 2</div> <div ng-switch-default>Shown when case is anything else than 1 and 2</div> </div> </div> <script> var app = angular.module("app", []); app.controller("MyCtrl", function ($scope) { $scope.data = {}; $scope.data.case = true; }); </script>
What does the ng-repeat directive do in AngularJS?
This directive is used to iterate over a collection of items and generate HTML from it.
<div ng-controller="MyCtrl"> <ul> <li ng-repeat="name in names"> {{ name }} </li> </ul> </div> <script> var app = angular.module("app", []); app.controller("MyCtrl", function ($scope) { $scope.names = [ 'Mahesh', 'Raj', 'Diksha' ]; }); </script>
What are the different variables used with the ng-repeat directive?
The “ng-repeat” directive has a set of unique variables that are useful while iterating the collection.
These variables are as follows.
- $index
- $first
- $middle
- $last
The “$index” contains the index of the element used for the traversal. The variables $first, $middle, and $last return a boolean value depending on whether the current item is the first, middle, or last element in the collection.
Can you demonstrate the use of ng-repeat variables?
Below is the Angular code to show the usage of ng-repeat variables.
<html> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <head> <script> var app = angular.module("app", []); app.controller("ctrl", function ($scope) { $scope.employees = [ { name: 'A', gender: 'alphabet' }, { name: 'B', gender: 'number' }, { name: 'C', gender: 'alphanumeric' }, { name: 'D', gender: 'special character' }]; }); </script> </head> <body ng-app="app"> <div ng-controller="ctrl"> <ul> <li ng-repeat="employee in employees"> <div> {{employee.name}} is a {{employee.gender}}. <span ng-if="$first"> <strong>(first element found)</strong> </span> <span ng-if="$middle"> <strong>(middle element found)</strong> </span> <span ng-if="$last"> <strong>(last element found)</strong> </span> </div> </li> </ul> </div> </body> </html>
The output is as follows.
A is a alphabet. (first element found) B is a number. (middle element found) C is a alphanumeric. (middle element found) D is a special character. (last element found)
What do you know about the Factory method in AngularJS?
A factory is a simple function that allows you to add some logic before creating the object.
In the end, it returns the packed object. The factory method has the following signature.
Syntax
app.factory('serviceName',function(){ return serviceObj;})
How do you create a service in Angular JS using the factory method?
See the below Angular code to create a service.
<script> //creating module var app = angular.module('app', []); //define a factory using factory() function app.factory('MyFactory', function () { var serviceObj = {}; serviceObj.function1 = function () { //TO DO: }; serviceObj.function2 = function () { //TO DO: }; return serviceObj; }); </script>
Where can you use the Factory method in Angular JS?
It is just a collection of functions, like a class. Hence, it can be instantiated in different controllers when you are using it with a constructor function.
How does string interpolation take place in AngularJS?
During the compilation process, the AngularJS compiler matches text and attributes using an interpolate service to see if it contains embedded expressions.
During the normal, digest life cycle, these expressions are updated and registered as watches.
Define AngularJS Application Lifecycle.
Understanding the life cycle of an AngularJS application makes it easier to learn about the way to design and implement the code.
The Apps life cycle consists of the following three phases-
- Bootstrap,
- Compilation, and
- Run-time
Describe the different stages of the AngularJS Application Lifecycle.
These three phases of the life cycle occur each time a web page of an AngularJS application gets loaded into the browser. Let’s learn about each of the three stages in detail:
- The Bootstrap Phase – In this phase, the browser downloads the AngularJS javascript library. After this, AngularJS initializes its necessary components and the modules to which the ng-app directive points. Now that the module has loaded, required dependencies are injected into it and become available to the code within that module.
- The Compilation Phase – The second phase of the AngularJS life cycle is the HTML compilation stage. Initially, when a web page loads in the browser, a static form of the DOM gets loaded. During the compilation phase, this static DOM gets replaced with a dynamic DOM which represents the app view. There are two main steps – first, is traversing the static DOM and collecting all the directives. These directives map to the appropriate JavaScript functionality which lies either in the AngularJS built-in library or custom directive code. After adding the scope, it generates a live view.
- The Runtime Data Binding Phase – This is the final phase of the AngularJS application. It remains until the user reloads or navigates to a different web page. At this point, any changes in the scope get reflected in the view, and vice-versa. It makes Scope the single source of data for the View.
The above points indicate that AngularJS behaves differently from traditional methods of binding data. The conventional approaches combine a template with data, received from the engine and then manipulate the DOM each time there is any change in the data.
However, AngularJS compiles the DOM only once and then links the compiled template as necessary, making it much more efficient than the traditional methods.
Define AngularJS Scope Lifecycle.
After the angular app gets loaded into the browser, scope data passes through different stages called its life cycle. Learning about this cycle helps us to understand the interaction between scope and other AngularJS components.
What are the different phases of the AngularJS Scope Lifecycle?
The scope data traverses through the following phases.
- Creation – This phase initializes the scope. During the bootstrap process, the $injector creates the root scope of the application. And, during template linking, some directives create new child scopes. A digest loop also gets created to interact with the event loop. It is responsible for updating DOM elements as well as executing any registered watcher functions.
- Watcher registration – This phase registers watchers (by using the $watch() function) for the scope created in the above point. These watches propagate the model changes to the DOM elements, automatically.
- Model mutation – This phase occurs when we do any modification in the angular app code. The scope function <$apply()> updates the model and then calls the <$digest()> function to update the DOM elements and the registered watches. However, when we change the scope of the angular code like within controllers or services, angular internal calls <$apply()> function for us. But, when we make changes to the scope outside the Angular code, we have to call the <$apply()> function explicitly, to force the model and DOM to be updated correctly.
- Mutation observation – This phase occurs, when the digest loop executes the $digest() function at the end of the $apply() call. When the $digest() function executes, it evaluates all watches for model changes. If there is a change in the value, $digest() calls the $watch listener and updates the DOM elements.
- Scope destruction – This phase occurs when the child scopes that are no longer needed, are removed from the browser’s memory by using the $destroy() function. It is the responsibility of the child scope creator to destroy them via scope.$destroy() API. It stops the propagation of $digest calls into the child scopes and enables the garbage collector to reclaim unused memory.
What is an auto bootstrap process in AngularJS?
AngularJS initializes automatically upon the “DOMContentLoaded” event or when the browser downloads the angular.js script and at the same time document.readyState is set to ‘complete.’ At this point, AngularJS looks for the ng-app directive which is the root of the Angular app compilation process.
After locating the ng-app directive, AngularJS does the following tasks.
- Load the module associated with the directive.
- Create the application injector.
- Compile the DOM starting from the ng-app root element.
We term this process Auto-bootstrapping.
Give an example of the auto bootstrap process in AngularJS.
Following is the sample code that helps to understand it more clearly:
Example-
<html> <body ng-app="myApp"> <div ng-controller="Ctrl">Hello {{msg}}!</div> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.5/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('Ctrl', function($scope) { $scope.msg = 'Welcome'; }); </script> </body> </html>
What is the manual bootstrap process in AngularJS?
Sometimes we may need to manually initialize the Angular app to have more control over the initialization process. We can do that by using angular.bootstrap() function within angular.element(document).ready() function. AngularJS fires this function when the DOM is ready for manipulation.
The <angular.bootstrap()> function takes two parameters, the document, and the module name injector.
Give an example of the manual bootstrap process in AngularJS.
Following is the sample code that helps to understand the concept more clearly.
<html> <body> <div ng-controller="Ctrl">Hello {{msg}}!</div> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.5/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('Ctrl', function($scope) { $scope.msg = 'Welcome'; }); //manual bootstrap process angular.element(document).ready(function () { angular.bootstrap(document, ['myApp']); }); </script> </body> </html>
How does the automatic bootstrap of multiple modules happen in Angular?
AngularJS gets initialized for the first module by default. If there are many modules, then we combine them into one, and the angular app automatically initializes it. Other modules act as dependencies for this newly created module.
Let’s take an example, suppose we have two modules: module1 and model2. To initialize the app automatically, based on these two modules the following code is used:
<html> <head> <title>Multiple modules bootstrap</title> <script src="lib/angular.js"></script> <script> //module1 var app1 = angular.module("module1", []); app1.controller("Controller1", function ($scope) { $scope.name = "Welcome"; }); //module2 var app2 = angular.module("module2", []); app2.controller("Controller2", function ($scope) { $scope.name = "World"; }); //module3 dependent on module1 & module2 angular.module("app", ["module1", "module2"]); </script> </head> <body> <!--angularjs autobootstap process--> <div ng-app="app"> <h1>Multiple modules bootstrap</h1> <div ng-controller="Controller2"> </div> <div ng-controller="Controller1"> </div> </div> </body> </html>
How does the manual bootstrap of multiple modules happen in AngularJS?
We can manually bootstrap the app by using angular.bootstrap() function for multiple modules.
Let’s modify the example in the previous question for a manual bootstrap process.
<html> <head> <title>Multiple modules bootstrap</title> <script src="lib/angular.js"></script> <script> //module1 var app1 = angular.module("module1", []); app1.controller("Controller1", function ($scope) { $scope.name = "Welcome"; }); //module2 var app2 = angular.module("module2", []); app2.controller("Controller2", function ($scope) { $scope.name = "World"; }); //manual bootstrap process angular.element(document).ready(function () { var div1 = document.getElementById('div1'); var div2 = document.getElementById('div2'); //bootstrap div1 for module1 and module2 angular.bootstrap(div1, ['module1', 'module2']); //bootstrap div2 only for module1 angular.bootstrap(div2, ['module1']); });
What is compile-time linking in AngularJS?
It collects an HTML string or DOM into a template and produces a template function.
It can then be used to link the scope and the template together.
AngularJS uses the compile function to change the original DOM before creating its instance and before the creation of scope.
Let’s see the Link function in detail.
It has the duty of linking the model to the available templates. AngularJS does the data binding to the compiled templates using Link.
Following is the Link syntax.
link: function LinkFn(scope, element, attr, ctrl){}
Where each of the four parameters is as follows-
- Scope – It is the scope of the directive.
- Element – It is the DOM element to go for processing.
- Attr- It is the collection of attributes of the DOM element.
- Ctrl – It is the array of controllers required by the directive.
AngularJS allows setting the link property to an object also. The advantage of having an object is that we can split the link function into two separate methods called, pre-link and post-link.
When does the pre/post-linking happen in AngularJS?
Execution of the post-link function starts after the linking of child elements. It is safer to do DOM transformation during the link time. The post-link function is suitable to execute the logic.
The post-linking gets executed before the child elements are linked. It is not safe to do DOM transformation as the compiler linking function will fail to locate the correct fields.
It is good to use the pre-link function to implement the logic that runs when AngularJS has already compiled the child elements. Also, before any of the child element’s post-link functions have been called.
Give an example of the Compile, Pre-Link, and Post-Link functions.
Let’s see an example that talks about Compile, Pre-Link, and Post-Link functions.
<html> <head> <title>Compile vs Link</title> <script src="lib/angular.js"></script> <script type="text/javascript"> var app = angular.module('app', []); function createDirective(name){ return function(){ return { restrict: 'E', compile: function(tElem, tAttrs){ console.log(name + ': compile'); return { pre: function(scope, iElem, iAttrs){ console.log(name + ': pre link'); }, post: function(scope, iElem, iAttrs){ console.log(name + ': post link'); } } } } } } app.directive('levelOne', createDirective('levelOne')); app.directive('levelTwo', createDirective('levelTwo')); app.directive('levelThree', createDirective('levelThree')); </script> </head> <body ng-app="app"> <level-one> <level-two> <level-three> Hello {{name}} </level-three> </level-two> </level-one> </body> </html>
Output: Hello
What rules does a Controller enforce in AngularJS?
A Controller is a set of JavaScript functions that are bound to a specified scope, the ng-controller directive.
Angular creates a new instance of the Controller object to inject the new scope as a dependency. The role of the Controller is to expose data to our view via $scope and add functions to it, which contains business logic to enhance view behavior.
Controller Rules
- A Controller helps in setting up the initial state of the scope object and defining its behavior.
- The Controller should not be used to manipulate the DOM as it contains only business logic. Instead, for managing the DOM, we should use data binding and directives.
- Do not use Controllers to format input. Instead, using angular form controls is recommended for that.
- Controllers should not be used to share code or states. Instead, use angular services for it.
What are the steps to create a Controller in AngularJS?
- It needs <ng-controller> directive.
- The next step is to add Controller code to a module.
- Name your Controller based on functionality. Its name should follow the camel case format (i.e., SampleController).
- Set up the initial state of the scope object.
Example-
Declaring a Controller using ng-Controller directive-
<div ng-app="mainApp" ng-controller="SampleController"> </div>
The following code displays the definition of SampleController.
<script> function SampleController($scope) { $scope.sample = { firstSample: "INITIAL", lastSample: "Initial", fullName: function() { var sampleObject; sampleObject = $scope.sample; return sampleObject.firstSample + " " + sampleObject.lastSample; } }; } </script>
What does service mean in AngularJS?
Answer.
Services are functions that are bound to perform specific tasks in an application.
- It gives us a method that helps in maintaining the angular app data for its lifetime.
- It gives us methods that facilitate to transfer of data across the controllers in a consistent way.
- It is a singleton object, and its instance is created only once per application.
- It is used to organize and share, data and function across the application.
Two main execution characteristics of angular services are that they are Singleton and lazy instantiated.
What does lazy instantiation mean in Angular JS?
It means that AngularJS instantiates a service only when a component of an application needs it. The dependency injection method is used here which makes the Angular codes, robust and less error-prone.
What are singletons in Angular JS?
Each application component dependent on the service, works with the single instance of the service created by the AngularJS.
Let us take an example of a straightforward service that calculates the square of a given number:
var CalculationService = angular.module('CalculationService', []) .service('Calculation', function () { this.square = function (a) { return a*a}; });
What are the built-in services provided by Angular JS?
AngularJS provides many built-in services. Each of them is responsible for a specific task. They always have prefixed with the $ symbol.
Some of the commonly used services in any AngularJS application are as follows:
- $http – used to make an Ajax call to get the server data.
- $window – Provides a reference to a DOM object.
- $Location – Provides a reference to the browser location.
- $timeout – Provides a reference to the window.set timeout function.
- $Log – used for logging.
- $sanitize – Used to avoid script injections and display raw HTML on the page.
- $Rootscope – Used for scope hierarchy manipulation.
- $Route – Used to display browser-based path in browser URL.
- $Filter – Used for providing filter access.
- $resource – Used to work with Restful API.
- $document – Used to access the window. Document object.
- $exceptionHandler – Used for handling exceptions.
- $q – Provides a promise object.
- $cookies – Use this service for reading, writing, and deleting browser cookies.
- $parse – This service intends to convert an AngularJS expression into a function.
- $cacheFactory – This service evaluates the specified expression when the user changes the input.
What are the different ways to create a service in AngularJS?
There are five different ways to create services in AngularJS.
- Value
- Factory
- Service
- Provider
- Constant
How to create a service using AngularJS value?
It is simple to create a value service type in AngularJS. Let’s take an example and define a service that displays the username:
var app=angular.module("app",[]); app.value("username","Madhav");
Code to use “Value”:
We can use this service anywhere by using dependency injection. The following example injects the service into a controller:
app.controller("MainController",function($scope, username){ $scope.username=username; });
In the above example, we have created a Value service “username” and used it in MainController.
How to create a service using Angular Factory?
Value Service is simple to write, but it may lack many essential features. So, the next service type we will look at is the “Factory” service. After its creation, we can even inject other services into it. Unlike Value service, we cannot add any dependency on it.
Let’s take an example to create the Factory service.
app.factory("username",function(){ var name="John"; return { name:name } });
The above code shows that the Factory service takes “function” as an argument. We can inject any number of dependencies or methods in this “function” as required by this service. This function must return some object. In our example, it returns an object with the property name. Now, let us look at how can we use this service:
Code to use “Factory”:
The function returns an object from a service that has a property name so we can access it and use it anywhere. Let’s see how we can use it in the controller:
app.controller("MainController",function($scope, username){ $scope.username=username.name; });
We are assigning the username from the factory service to our scope username.
How to create a service from the Angular JS service?
It works the same as the “Factory” service. But, instead of a function, it receives a Javascript class or a constructor function as an argument. Let’s take an example. Suppose we have a function:
function MyExample(num){ this.variable="value"; }
Now, we want to convert the function into a service. Let’s take a look at how we can do this with the “Factory” method:
app.factory("MyExampleService",["num" ,function(num){ return new MyExample(num); }]);
Thus in this way, we will create a new instance and return it. Also, we have injected <num> as a dependency on Factory service. Now, let’s see how we can do this using the Service type:
app.service("MyExampleService",["num", MyExample]);
Thus, we have called the service method on the module and provided its name, dependency, and the name of the function in an array.
How to create a service using an Angular JS provider?
It is the parent of all the service types supported by AngularJS, except the “Constant” that we will discuss in the next section. It is the core of all the service types. Thus we can say that other services work on top of it. It allows us to create a configurable service that must implement the <$get> method.
We use this service to expose the API that is responsible for doing the application-wide configuration. The configuration should be completed before starting the application.
Let’s take an example.
Example-
app.provider('authentication', function() { var username = "John"; return { set: function(newUserName) { username = newUserName; }, $get: function() { function getUserName() { return username; } return { getUserName: getUserName }; } }; });
This example initializes a provider with its name as “authentication.” It also implements a <$get> function, which returns a method <getUsername> which in turn returns the private variable called username. It also has a setter, using it we can set the username on the application startup as follows:
app.config(["authenticationProvider", function(authenticationProvider) { authenticationProvider.set("Mihir"); }]);
How to create a service using Angular JS Constant?
As the name suggests, this service helps us to declare constants in our application. We can then use them wherever needed, just by adding them as a dependency. There are many places, where we use constants like some base URLs, application names, etc.
We define them once and use them anywhere as per our needs. Thus, this technique allows us to write the definition in one place. If there is any change in the value later, we have to do the modifications at one location only.
Here is an example of how we can create constants:
app.constant('applicationName', 'Service Tutorials');
What is the use of $watch() in AngularJS?
The use of this function is to observe changes in a variable on the $scope. It triggers a function call when the value of that variable changes. It accepts three parameters: expression, listener, and equality object. Here, listener and equality objects are optional parameters.
$watch(watchExpression, listener, [objectEquality]).
Following is an example of using the $watch() function in AngularJS applications.
Example-
<html> <head> <title>AngularJS Watch</title> <script src="lib/angular.js"></script> <script> var myapp = angular.module("myapp", []); var myController = myapp.controller("myController", function ($scope) { $scope.name = 'dotnet-tricks.com'; $scope.counter = 0; //watching change in name value $scope.$watch('name', function (newValue, oldValue) { $scope.counter = $scope.counter + 1; }); }); </script> </head> <body ng-app="myapp" ng-controller="myController"> <input type="text" ng-model="name" /> <br /><br /> Counter: {{counter}} </body> </html>
What is the use of $digest() in AngularJS?
This function iterates through all the watch list items in the $scope object, and its child objects (if it has any). When $digest() iterates over the watches, it checks if the value of the expression has changed or not. If the value has changed, AngularJS calls the listener with the new value and the old value.
The $digest() function gets called whenever AngularJS thinks it is necessary. For example, after a button click, or after an AJAX call. You may have some cases where AngularJS does not call the $digest() function for you. In that case, you have to call it yourself.
Following is the example of using the $digest() function in AngularJS applications:
Example-
<html> <head> <title>AngularJS Digest Example</title> <script src="lib/jquery-1.11.1.js"></script> <script src="lib/angular.js"></script> </head> <body ng-app="app"> <div ng-controller="Ctrl"> <button class="digest">Digest my scope!</button> <br /> <h2>obj value : {{obj.value}}</h2> </div> <script> var app = angular.module('app', []); app.controller('Ctrl', function ($scope) { $scope.obj = { value: 1 }; $('.digest').click(function () { console.log("digest clicked!"); console.log($scope.obj.value++); //update value $scope.$digest(); }); }); </script> </body> </html>
What is the use of $apply() in AngularJS?
AngularJS automatically updates the model changes which are inside the AngularJS context. When you apply changes to any model, that lies outside of the Angular context (like browser DOM events, setTimeout, XHR, or third-party libraries), then you need to inform Angular about the changes by calling $apply() manually. When the $apply() function call finishes, AngularJS calls $digest() internally, to update all data bindings.
Following is the example of using the $apply() function in AngularJS applications.
Example-
<html> <head> <title>AngularJS Apply Example</title> <script src="lib/angular.js"></script> <script> var myapp = angular.module("myapp", []); var myController = myapp.controller("myController", function ($scope) { $scope.datetime = new Date(); $scope.updateTime = function () { $scope.datetime = new Date(); } //outside angular context document.getElementById("updateTimeButton").addEventListener('click', function () { //update the value $scope.$apply(function () { console.log("update time clicked"); $scope.datetime = new Date(); console.log($scope.datetime); }); }); }); </script> </head> <body ng-app="myapp" ng-controller="myController"> <button ng-click="updateTime()">Update time - ng-click</button> <button id="updateTimeButton">Update time</button> <br /> {{datetime | date:'yyyy-MM-dd HH:mm:ss'}} </body> </html>
What is the main difference between $apply() and digest()?
Following is the key difference between $apply() and $digest().
- The $apply() method performs an update to the model properties forcibly.
- The $digest() method evaluates the watchers for the current scope. However, the $apply() method does the same for the root scope.
Which one handles exceptions automatically, $digest or $apply?
When an error occurs in one of the watchers, $digest() cannot handle it via the $exceptionHandler service. In that case, you have to manage the exception yourself. However, $apply() uses a try-catch block internally to handle errors. But, if an error occurs in one of the watchers, then it transfers the errors to the $exceptionHandler service.
Code for $apply() function.
$apply(expr) { try { return $eval(expr); } catch (e) { $exceptionHandler(e); } finally { $root.$digest(); } }
What is the use of $watchgroup() in AngularJS?
This function $watchgroup() first came in AngularJS 1.3. It works in the same way as the $watch() function except that the first parameter is an array of expressions.
$watchGroup(watchExpression, listener)
The listener is also an array containing the new and old values of the variables. The listener gets called whenever any expression contained in the <watchExpressions> array changes.
$scope.teamScore = 0; $scope.time = 0; $scope.$watchGroup(['teamScore', 'time'], function(newVal, oldVal) { if(newVal[0] > 20){ $scope.matchStatus = 'win'; } else if (newVal[1] > 60){ $scope.matchStatus = 'times up'; });
What is the use of $watchCollection() in AngularJS?
The use of this function is to watch the properties of an object. It gets fired when there is any change in their values.
It takes an object as the first parameter and monitors the properties of the object.
$watchCollection(object, listener)
The listener gets called whenever there is any change in the object.
$scope.names = ['shailendra', 'deepak', 'mohit', 'kapil']; $scope.dataCount = 4; $scope.$watchCollection('names', function (newVal, oldVal) { $scope.dataCount = newVal.length; });
How to perform mandatory input field validation in AngularJS?
AngularJS allows form validation on the client side in a simplistic way. First of all, it monitors the state of the form and its input fields. Secondly, it observes any change in the values and notifies the same to the user.
By using “Required Field” validation we can prevent, form submission with a null value. It’s mandatory for the user to fill in the form fields.
The syntax for required field validation is as follows.
<input type="text" required />
Example Code.
<form name="myForm"> <input name="myInput" ng-model="myInput" required> </form> <p>The input's valid state is:</p> <h1>{{myForm.myInput.$valid}}</h1>
How to perform minimum & maximum field length validations in AngularJS?
To prevent the user from providing a lesser or an excess number of characters in the input field, we use Minimum and maximum length validation. The AngularJS directives used for Minimum and maximum length validations are <ng-minlength> and <ng-maxlength>. Both of these attributes take integer values. The <ng-minlength> attribute is used to set the number of characters a user is limited to, whereas the <ng-maxlength> attribute sets the maximum number of characters that a user is allowed to enter.
This type of validation requires the following syntax.
<input type="text" ng-minlength=5 /> <input type="text" ng-maxlength=10 />
Example code:
<label>User Message:</label> <textarea type="text" name="userMessage" ng-model="message" ng-minlength="100" ng-maxlength="1000" required> </textarea> <div ng-messages="exampleForm.userMessage.$error"> <div ng-message="required">This field is required</div> <div ng-message="minlength">Message must be over 100 characters</div> <div ng-message="maxlength">Message must not exceed 1000 characters</div> </div>
How to perform pattern validation in AngularJS?
AngularJS provides a <ng-pattern> directive to ensure that input fields match the regular expressions in the attributes.
To handle pattern validation, we can use the following syntax.
<input type="text" ng-pattern="[a-zA-Z]" />
To activate the error message in <ng-pattern>, we pass the value of the pattern into ng-message.
Example code.
<label>Phone Number:</label> <input type="email" name="userPhoneNumber" ng-model="phoneNumber" ng-pattern="/^[\+]?[(]?[0-9]{3}[)]?[-\s\.]?[0-9]{3}[-\s\.]?[0-9]{4,6}$/" required/> <div ng-messages="exampleForm.userPhoneNumber.$error"> <div ng-message="required">This field is required</div> <div ng-message="pattern">Must be a valid 10 digit phone number</div> </div>
How to perform email validation in AngularJS?
To validate an email address, AngularJS provides the ng-model directive. Using the following syntax, we can verify the email from an input field.
<input type="email" name="email" ng-model="user.email" />
Example code.
<label>Email Address:</label> <input type="email" name="userEmail" ng-model="email" required /> <div ng-messages="exampleForm.userEmail.$error"> <div ng-message="required">This field is required</div> <div ng-message="email">Your email address is invalid</div> </div>
How to perform number validation in AngularJS?
To validate input against Number we can use <ng-model> directive from AngularJS.
Its syntax is as follows.
<input type="number" name="personage" ng-model="user.age" />
How to perform URL validation in AngularJS?
To validate an input field for the URL, we can use the following signature.
<input type="url" name="weblink" ng-model="user.facebook_url" />
Example Code.
<div ng-app="urlInputExample"> <form name="myForm" ng-controller="UrlController"> <label for="exampleInput">Enter Email</label> <input type="url" name="input" ng-model="example.url" required /> <p style="font-family:Arial;color:red;background:steelblue;padding:3px;width:350px;" ng-if='!myForm.input.$valid'>Enter Valid URL</p> </form> </div>
How do you exchange data among different modules of your Angular JS application?
There are a number of ways in Angular to share data among modules. A few of them are as follows.
- The most common method is to create an Angular service to hold the data and dispatch it to the modules.
- Angular has a matured event system that provides $broadcast(), $emit(), and $on() methods to raise events and pass data among the controllers.
- We can also use $parent, $nextSibling, and $controllerAs to directly access the controllers.
- Variables defined at the root scope level ($rootScope) are available to the controller scope via prototypical inheritance. But they behave like globals and are hard to maintain.
How would you use an AngularJS service to pass data between controllers? Explain with examples.
Using services is the best practice in Angular to share data between controllers. Here is a step-by-step example to demonstrate data transfer.
We can prepare the data service provider in the following manner.
Example-
app.service('dataService', function() { var dataSet = []; var addData = function(newData) { dataSet.push(newData); }; var getData = function(){ return dataSet; }; return { addData: addData, getData: getData }; });
Now, we’ll inject the service dependency into the controllers.
Say, we have two controllers – pushController and popController.
The first one will add data by using the data service provider’s addData method. And, the latter will fetch this data using the service provider’s getData method.
Example-
app.controller('pushController', function($scope, dataService) { $scope.callToAddToProductList = function(currObj){ dataService.addData(currObj); }; }); app.controller('popController', function($scope, dataService) { $scope.dataSet = dataService.getData(); });
How will you send and receive data using the Angular event system?
We can call the $broadcast method using the $rootScope object and send any data we want.
$scope.sendData = function() { $rootScope.$broadcast('send-data-event', data); }
To receive data, we can use the $scope object inside a controller.
$scope.$on('send-data-event', function(event, data) { // process the data. });
How do you switch to different views from a Controller function?
With the help of <ui-sref> directive or using the $state.go() function, we can switch between different views from the controllers.
Provide a working example of switching views in AngularJS.
Here is a working example to bring you more clarity on switching views in Angular.
AngularJS Code
var myapp = angular.module('myapp', ['ui.router']); //Setup a basic AngularJS app. myapp.config(['$stateProvider', function ($stateProvider) { $stateProvider.state('python', { url: '/python', template: '<div style="background: blue">{{pythonCtrl.title}}</div>', controller: 'TestFirstCtrl', controllerAs: 'pythonCtrl' }); $stateProvider.state('java', { url: '/java', template: '<div style="background: green">{{javaCtrl.title}}</div>', controller: 'TestSecondCtrl', controllerAs: 'javaCtrl' }); }]); //These two controllers will control the same view with different routes. myapp.controller('TestFirstCtrl', ['$scope', function($scope) { this.title = 'Learn Python'; }]); myapp.controller('TestSecondCtrl', ['$scope', function($scope) { this.title = 'Learn Java'; }]);
HTML Page
It is the HTML code to test the Angular functionality given above.
<html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script src="//angular-ui.github.io/ui-router/release/angular-ui-router.js"></script> </head> <body ng-app="myapp"> <input type="button" ui-sref="python">Go to Learn Python</button> <input type="button" ui-sref="java">Go to Learn Java</button> <div ui-view></div> </body> </html>
What would you do to limit a scope variable to have one-time binding?
We can prefix the “::” operator to the scope variable.
It’ll make sure the candidate is aware of the available variable bindings in AngularJS.
What is the difference between one-way binding and two-way binding?
The main difference between one-way binding and two-way binding is as follows.
- In one-way binding, the scope variable in the HTML gets initialized with the first value its model specifies.
- In two-way binding, the scope variable will change its value whenever the model gets a different value.
Which AngularJS directive would you use to hide an element from the DOM without modifying its style?
It is the conditional ngIf Directive that we can apply to an element. Whenever the condition becomes false, the ngIf Directive removes it from the DOM.
More Interview Questions on Web Development
Top Web Development Tips and Tricks
Summary – AngularJS Interview Questions for Full Stack Developers
After going through the AngularJS interview questions given above, we believe that you’ll undoubtedly do well in a job interview. By the way, you may need to keep practicing until you get confirmed.
We wish you success in your job hunt and appreciate you for being the part of TechBeamers family. Don’t miss to read the last line of this post.
The difference between getting a job offer or not getting a job offer is that the successful person does some little thing, that no one else does.
If you find this post useful and like to see more such updates, then follow us on our social media accounts.
Best,
TechBeamers