JavaScript is at the forefront of web development and remains a highly sought-after skill. As a web developer, it’s essential to have a solid understanding of JavaScript concepts. To help you prepare for your next interview, we’ve compiled a list of the top 30 JavaScript interview questions and answers.
Our team has carefully analyzed common JavaScript interview questions and answers both online and offline. Whether you’re a beginner or a seasoned developer, these questions will test your knowledge and help you sharpen your skills.
30 JavaScript Interview Questions and Answers
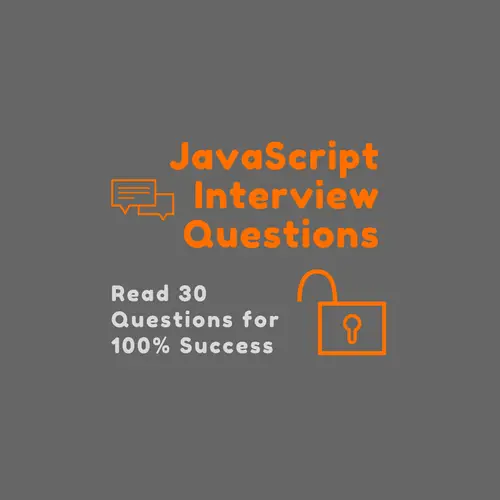
We have three sections here for 30 JavaScript interview Questions and Answers. Each section has 10 questions focussing on basic, intermediate, and a bit advanced areas. We have compiled these after thorough research both online and offline and you are most likely to face them in interviews.
Set#1 Let’s Get the Basics Right.
Firstly, you should check the basic JavaScript interview questions and answers. And see how well you be able to answer if asked in an interview.
Q.1- What is a JavaScript object? What are the different ways to create it?
A JavaScript object is a data structure that stores key-value pairs. You can create objects in several ways:
- Object Literal: The simplest way to create an object is by using curly braces.
const person = {
name: "John",
age: 30,
};
- Object Constructor: You can use the
Object
constructor to create an empty object and add properties later.
const car = new Object();
car.make = "Toyota";
car.model = "Camry";
- Object.create(): This method allows you to create an object with a specified prototype object.
const animal = Object.create(null);
animal.type = "Dog";
- Class Constructor: With ES6 classes, you can create objects using constructor functions.
class Fruit {
constructor(name, color) {
this.name = name;
this.color = color;
}
}
const apple = new Fruit("Apple", "Red");
All these methods result in objects that store data in a key-value format, making them versatile data structures in JavaScript.
It is one of the most obvious and important JavaScript interview questions and answers. So, you must have clarity on what kind of OOP support JavaScript has.
Q-2. How do you figure out the scope of variables in JavaScript?
The scope of a variable in JavaScript refers to the regions of the code from where the variable is accessible. There are two types of scope in JavaScript: global scope and local scope.
Global scope: It refers to using the global variables that exist outside of any function or block of code. This means that you can access them from anywhere in the code.
Local scope: It refers to using local variables that have scope inside of a function or block of code. This means that you can only access them from within the function or block of code in which they exist.
Here is an example of global and local scope in JavaScript:
// Global variable
var name = "User name";
function testFunc() {
// Local variable
var age = 30;
console.log(name); // User name
console.log(age); // 27
}
testFunc();
console.log(name); // User name
console.log(age); // ReferenceError: age is not defined
In this example, the variable name
is a global variable because it is declared outside of any function or block of code. This means you can access it from anywhere in the code.
The variable age
is a local variable because it is declared inside of the myFunction()
function. This means that it can only be accessed from within the myFunction()
function.
When we call the myFunction()
function, the local variable age
is created. The value of the local variable age
is then logged to the console.
Once the myFunction()
function returns, the local variable age
is destroyed. This means you no longer have access to it.
When we try to log the value of the local variable age
outside of the myFunction()
function, we get a ReferenceError
because the variable does not exist.
Q-3. What is <this> keyword in JavaScript?
The this
keyword in JavaScript refers to the current context or object, which depends on how and where it is used.
For example:
const person = {
name: "John",
greet: function() {
console.log(`Hello, my name is ${this.name}`);
},
};
person.greet(); // "Hello, my name is John"
In this example, this
inside the greet
function refers to the person
object, allowing access to its properties. The actual value of this
depends on the context in which it’s used, such as in functions or event handlers.
Q-4. What does the “prototype” property do in JavaScript?
The prototype property in JavaScript enables objects to inherit methods and properties. You use it to add functions or attributes that other objects can share.
For example:
function Animal(name) {
this.name = name;
}
Animal.prototype.sayName = function() {
console.log(`My name is ${this.name}`);
};
const cat = new Animal("Whiskers");
cat.sayName(); // Outputs: "My name is Whiskers"
Here, the sayName
function is added to the Animal
constructor’s prototype and any objects created from it inherit this function, promoting code reusability.
Q-5. Describe Closures and why JavaScript needs them.
Closures in JavaScript are functions that have access to the outer (enclosing) function’s variables, even after the outer function has finished executing. Moreover, we often use them to create private variables, maintain state, and encapsulate data.
Example:
function testCounter() {
let count = 0;
return function() {
return count++;
};
}
const test = testCounter();
console.log(test()); // Outputs 0
console.log(test()); // Outputs 1
In this example, the inner function maintains access to the count
variable due to the closure, allowing it to preserve and update the state between function calls. Hence, without any doubt, we can say Closures are a fundamental concept in JavaScript, enabling data encapsulation and more.
Q-6. When would you opt for “self” rather than “this” in JavaScript, and why?
You would choose “self” over “this” in JavaScript when you need to maintain a consistent reference to an outer scope, particularly in callback functions or closures.
For example:
const person = {
name: "John",
greet: function() {
const self = this;
setTimeout(function() {
console.log(`Hello, my name is ${self.name}`);
}, 1000);
},
};
person.greet(); // Outputs: "Hello, my name is John"
Using “self” ensures the inner function correctly accesses the “name” property from the outer scope, even inside the setTimeout callback, providing more predictable behavior.
Q-7. What’s an unnamed function, and when is it useful in JavaScript?
An unnamed function, also known as an “anonymous function,” is a function without a nem. It is useful in JavaScript when you need a function for a specific task but do not want a formal declaration.
For instance, it’s valuable in cases like event handling or when passing them as arguments in another function. Here’s a simple example:
const numbers = [1, 2, 3, 4, 5];
const squared = numbers.map(function(x) {
return x * x;
});
console.log(squared); // Outputs: [1, 4, 9, 16, 25]
In this case, the anonymous function squares each number in the array, demonstrating its utility in special use cases.
Q-8. What distinguishes “==” from “===” when used in JavaScript for value comparison?
The main difference between “==” (loose equality) and “===” (strict equality) in JavaScript is how they compare values:
- “==” checks for equality after performing type coercion, which means it converts operands to a common type before making the comparison.
- “===” checks for equality without type coercion, so both value and type must be the same for it to return true.
For example:
"5" == 5; // true (loose equality, type coercion)
"5" === 5; // false (strict equality, type matters)
In the first comparison, “==” converts the string to a number and finds them equal. In the second comparison, “===” considers the string and number to be different due to their distinct types.
Q-9. Could you provide an overview of the data types available in JavaScript?
A data type in JavaScript defines the type of a variable, and JavaScript supports several data types, including:
Data Type | Purpose |
---|---|
Number | For numeric values. |
String | For text data. |
Boolean | For true or false values. |
Object | For complex data structures. |
Array | For ordered lists of values. |
Function | For executable code. |
Undefined | For uninitialized variables. |
Null | For reflecting the absence of a value. |
Symbol | For unique and unchangeable values. |
BigInt | For handling large integers. |
This table provides a quick reference for JavaScript data types.
Q-10. What is JavaScript’s prototypal inheritance?
Prototypal inheritance in JavaScript is a way of sharing properties and behaviors between objects. In this system, each object has a prototype (parent) object, and it inherits properties and methods from that prototype. It promotes code reusability and hierarchy creation.
function Fruit(type) {
this.type = type;
}
Fruit.prototype.describe = function() {
return `This is a ${this.type} fruit.`;
};
const apple = new Fruit("red apple");
console.log(apple.describe()); // Outputs: "This is a red apple fruit.
In this example, we have a Fruit
constructor with a prototype method describe
. The apple
object inherits the describe
method through prototypal inheritance, allowing it to describe itself as a “red apple fruit.”
Set#2 of JavaScript Interview Questions and Answers
Here comes the second section of JavaScript interview questions and answers. Brace yourself to face a little bit of difficult questions. We tried to cover a mix of conceptual and practical aspects here. An interviewer would ask these to assess the depth of your knowledge.
Q-11: What is asynchronous programming in JavaScript, and why is it important?
Asynchronous programming is a programming technique that allows your program to start a long-running task without blocking other tasks from running. This is important in JavaScript because JavaScript is a single-threaded language, meaning that only one task can run at a time. If a single task blocks the main thread, then the entire program will become unresponsive.
It allows JavaScript programs to remain responsive even when they are performing long-running tasks. This is because asynchronous tasks are run in the background, without blocking the main thread.
Here are some examples of asynchronous tasks in JavaScript:
- Fetching data from a server
- Uploading files to a server
- Performing complex calculations
- Animating elements on a web page
To be sure, check the example of asynchronous programming in JavaScript:
// Fetch data from a server
fetch("https://example.com/api/users")
.then(response => response.json())
.then(users => {
// Do something with the users data
});
// Continue running other tasks while the data is being fetched
In this example, the fetch()
function is used to fetch data from a server. The then()
method is used to handle the response from the server. The first then()
method will be called when the response is received, and the second then()
method will be called when the response is parsed as JSON.
The fetch()
function is an asynchronous function, meaning that it does not block the main thread while the data is being fetched. This allows other tasks to continue running while the data is being fetched.
Q-12: Why is JavaScript called a loosely typed language? What does loosely mean here?
In the context of programming languages, loosely typed means that the compiler or interpreter does not strictly enforce the types of variables and expressions. This means that you can assign any type of value to a variable, and you can perform operations on values of different types.
For example, in a loosely typed language, you could assign a string value to a variable that was holding a number. The language would automatically convert the string to a number before performing the operation.
This flexibility can be useful in some cases, but it can also lead to errors. For example, if you accidentally assign a string value to a number variable, you might get an unexpected result.
JavaScript has very few restrictions on the types of values that we can set for variables and expressions. This can make it easy to write code quickly, but it can also make it difficult to debug errors.
Without delay, go through this example using loosely typed code in JavaScript:
// Declare a variable of type number
let number = 10;
// Assign a string value to the variable
number = "Hello, world!";
// Perform a mathematical operation on the variable
console.log(number + 1); // Outputs "Hello, world!1"
In this example, the JavaScript interpreter automatically converts the string “Hello, world!” to a number before performing the addition operation.
Q-13. What are the primary methods for creating arrays in JavaScript?
It is important to realize that two main methods for creating arrays in JavaScript are using array literals (square brackets) and the Array constructor.
Firstly, use Array literal syntax: This is the most common way to create an array in JavaScript. It involves enclosing a list of elements in square brackets ([]). For example:
const myArray = [1, 2, 3, 4, 5];
Secondly, use the Array() constructor: This constructor takes a single argument, which is the number of elements that the array will have. For example:
const myArray = new Array(5);
Now, it is up to us to go for the method we want. Moreover, it depends on our personal choice and the specific needs of the code. However, the array literal syntax is more concise and readable.
Q-14. What are cookies and how do they work in JavaScript?
The first thing to remember is JavaScript cookies are small pieces of data that websites store on a user’s device. They work by sending the data back and forth between the user’s browser and the web server. That’s how the same session is able to work across different pages.
In order to understand it more, take the following example:
// Setting a cookie
document.cookie = "username=John Doe; expires=Thu, 01 Jan 2099 00:00:00 UTC; path=/";
// Retrieving a cookie
const username = document.cookie.split('; ').find(cookie => cookie.startsWith("username="));
const usernameValue = username ? username.split('=')[1] : undefined;
In this example, “username” is a cookie that has a value. We are accessing it later to display the stored information. The use of cookies is common for tasks like remembering user preferences or login sessions.
Next are some more JavaScript questions and answers, but with a few more lines of explanation in each answer:
Q-15. Which JavaScript function extends an array by adding elements to the end?
Firstly, the push() method is a built-in method in JavaScript that adds elements to the rear end of the array. And, secondly, it also calculates and provides the updated length. Here is an example so that you can understand more clearly.
const myArray = [1, 2, 3, 4, 5];
// Add the element 6 to the end of the array
myArray.push(6);
// The array now contains the elements [1, 2, 3, 4, 5, 6]
// The new length of the array is 6
const newLength = myArray.length;
console.log(newLength); // 6
Q-16. Does JavaScript use any scheme for naming variables?
Yes, in JavaScript, it is a best practice to use camelCase for variable names. This scheme makes the code more readable and maintainable.
By the way, variable names in JavaScript should be descriptive and start with a lowercase letter. It is also important to avoid using reserved keywords and global variables.
Simultaneously, you can check the rules for naming variables in JavaScript.
- Variable names must begin with a letter, underscore (_), or a dollar sign ($).
- Variable names can consist of letters, numbers, underscores (_), and dollar signs ($).
- They must not contain spaces or other special characters.
- Don’t use reserved keywords for naming. They are special words in JavaScript with predefined meanings, such as “var,” “function,” and “if.” Using these words as variable names can cause errors.
Subsequently, here are some examples of good variable names:
const name = "John Doe";
const age = 30;
const isLoggedIn = true;
Q-17. How do you set up a cookie in JavaScript?
In order to set up a cookie in JavaScript, you can use the document.cookie
property. Here’s a concise example:
document.cookie = "myCookieName=myCookieValue; expires=Thu, 01 Jan 2099 00:00:00 UTC; path=/";
This line of code creates a cookie named “myCookieName” with the value “myCookieValue” that expires on January 1, 2099, and is accessible from the root path (“/”).
Q-18. What mechanism does JavaScript use to read a cookie?
JavaScript uses the document.cookie
property to read cookies. It returns a string containing all the cookies for the current document. You can parse this string to retrieve specific cookie values. For example:
const myCookie = document.cookie.split('; ').find(cookie => cookie.startsWith("myCookieName="));
const myCookieValue = myCookie ? myCookie.split('=')[1] : undefined;
In this code, we split the document.cookie
string to find and extract the value of a specific cookie named “myCookieName.”
Q-19. How do you clear a cookie in JavaScript?
To clear a cookie in JavaScript, you can set the cookie’s expiration date to a past time, effectively making it immediately expire. Here’s a concise example:
document.cookie = "myCookieName=; expires=Thu, 01 Jan 1970 00:00:00 UTC; path=/";
This code sets the “myCookieName” cookie’s expiration date to a past time (January 1, 1970), rendering it invalid and effectively destroying it.
Q-20. What is the process of submitting a form in JavaScript?
To submit a form in JavaScript, you can use the submit()
method on the form element. Here’s a brief example:
document.getElementById("myForm").submit();
In this code, we use the getElementById
method to access the form element with the ID “myForm” and then call the submit()
method on it to trigger the form submission.
Q-21. Why does JavaScript discourage to use of global variables?
Global variables are variables that exist outside of any function or block of code. This means that you can access them from anywhere in your JavaScript code.
They are dangerous to use because they can lead to errors and conflicts. For example, if two different parts of your code try to access the same global variable at the same time, it can be difficult to predict what will happen.
To prevent using global variables, you should use local variables and functions to scope your code. Local variables are variables that have declarations inside a function or block of code.
Here is an example of how to use local variables and functions to scope your code:
function testCode() { // Declare a local variable const name = "Test User"; // Use the local variable console.log(name); // Test User } // Call the function testCode();
Q-22. What are the main objects JavaScript uses?
JavaScript has various key object types that are as follows:
- Window: It is global and contains all global variables and functions.
- Built-in: These are essential objects like String, Array, Date, and Math, providing common functionality.
- User-Defined: Custom objects created by developers as per application needs.
- Document Object Model (DOM): It is the object form of all elements of a web page.
- Events: They are like a trigger that occurs when a user clicks a mouse or presses any keys.
- Function: Functions are also objects in JavaScript as they allow dynamic code execution.
These objects enable JavaScript to manage and interact with data, the web page, and user input.
Q-23. What is the purpose of deferring JavaScript loading?
Deferring JavaScript improves the page load speed. It delays non-essential script execution, ensuring critical content loads first. For example, instead of this code:
<script src="testscr.js"></script>
The below code is way better to use:
<script src="testscr.js" defer></script>
This loads and runs the script after the page content, improving user experience.
Q-24: What is JavaScript strict mode, and why is it important?
A: JavaScript strict mode is a way to write more robust and reliable code. It makes the JavaScript engine parse and execute your code more rigorously, and it helps you to catch errors early on.
The strict mode had its way in ECMAScript 5, and now all major browsers support it. To activate the strict mode, you simply add the"use strict";
at the beginning of your code.
Why is strict mode important?
Strict mode is important because it can help you to write better and error-free JavaScript code. Here are some specific examples of how strict mode can help.
- Prevent accidental errors: In strict mode, mistyping a variable name will throw an error, instead of silently creating a new global variable. This can help you to catch errors early.
- Make your code more efficient: In strict mode, if you try to use a variable that hasn’t been declared yet, you will get an error.
- Make your code more readable and maintainable: Strict mode discourages bad programming practices. For example, in strict mode, you cannot delete variables that are declared with the
const
keyword. - Make your code more secure: Strict mode prevents certain types of code that could be exploited by attackers. For example, in strict mode, you cannot use the
with
statement.
How eval plays a role in strict mode
The eval()
function is a dangerous function that can be used to execute arbitrary code. In strict mode, the behavior of the eval()
function is changed to make it more secure.
Q-25. What is the use of JavaScript handlers and how do you use them?
The use case of JavaScript event handlers is supporting user interactions or events on a web page. We attach them to HTML elements and define the actions to take when an event occurs.
For example, to use an "onclick"
event handler to trigger a function when the user clicks a button.
<button id="testBtn">Click me</button>
<script>
document.getElementById("testBtn").onclick = function() {
alert("Button clicked!");
};
</script>
Q-26. What is the behavior of a JavaScript function returning nothing?
A JavaScript function that returns nothing or lacks a return statement implicitly returns undefined
. For example:
function testme() {
// This function returns undefined.
}
const test= testme();
console.log(test); // Outputs 'undefined'
In this example, testme()
returns undefined
, which is the default return value for functions without an explicit return statement.
Q-27. What does the JavaScript functionencodeURI()
do?
The encodeURI()
function in JavaScript works to encode a URI (Uniform Resource Identifier) by replacing certain characters with their UTF-8 encoding. This ensures that the URI is valid and safe for sending over the web.
For example:
const test = "some test url";
const postTest = encodeURI(originalURI);
console.log(postTest);
In this example, encodeURI()
converts the space in the URL to “%20,” making it a valid and safe URI for web usage.
Q-28. What is different between JavaScript Array() and []?
Array()
and []
both create arrays in JavaScript. However, []
is more common to use because it’s simpler and more concise.
For example:
Using Array()
:
const testArr = new Array(1, 2, 3);
Using []
:
const testArr = [1, 2, 3];
Both create arrays with the same elements but []
is easier to read and write. Additionally, Array()
can lead to unexpected results when not used as intended, so it’s generally best to stick with []
.
Q-29. How does variable hoisting take place in JavaScript?
In JavaScript, variable declarations are the first piece of code that executes irrespective of their location. Hence, it doesn’t matter whether we declare a variable at the top or anywhere else. This functionality which moves the declaration to the top either inside a function or in the global code is known as hoisting.
out = 2; var out; function myfunc() { var in = "inside" // some code } // ...
JavaScript will interpret the above in the following manner.
var out; out = 2; function myfunc() { var in; // some code in = "inside"; }
Q-30. How will you replace all occurrences of a string in JavaScript?
We can use String’s <replace()> method to substitute any string. There are the following two ways to use this method.
Pass the input string as a regular expression.
str = "ghktestlllltest-sdds" str = str.replace(/test/g, ''); alert(str)
Use the RegExp class to create a regular expression and pass it.
String.prototype.replaceAll = function(find, replace) { var target = this; return target.replace(new RegExp(find, 'g'), replace); }; str = "ghktestlllltest-sdds" str = str.replaceAll('test', ''); alert(str)
Great! You have successfully finished reading the 30 most essential JavaScript interview questions and answers.
Conclusion
The web development market has been growing from last many years. And with the inception of new technologies like AngularJS/Node.js, it shall expand further. And we are sure that JavaScript will keep its place as the leading skill for web developers. We suggest you save this list of JavaScript interview questions and answers as you can then refresh your knowledge at any time.
Here are more popular resources from our website to follow:
- 20 Proven JavaScript Tips and Coding Practices
- JavaScript Quiz- Web Developers
- Top 10 JavaScript IDE for Web Development
- 6 Web Application Testing Techniques Every Tester Should Know
Also, we hope you will use the above JavaScript interview questions as a quick reference to brush up on your skills.
Best,
TechBeamers