In this post, we have covered the five most asked Selenium questions and their answers in great detail. Many of our readers had requested us to write a dedicated post to address these important concepts.
Since these Selenium questions are quite important from the interview perspective, we adopted a practical approach while answering each of them. You can read these five questions by clicking on the hyperlinks given below.
Must Read: 100+ Selenium Interview Questions
Do You Know the 5 Most Asked Selenium Questions?
You must be wondering as you might have read a lot about Selenium, but what is that still missing? Don’t worry we have compiled this short yet important list of 5 such questions. Check out and go through each and every one of these below.
- What types of data have you handled in Selenium for automating web applications?
- What are various build and deploy tools apart from Maven used in the industry?
- What is WebdriverIO? Which technology does it use? Why should QA use it for UI automation?
- What are the different network protocols that Selenium supports? Also, how will you handle them in Selenium?
- What is Continuous Integration (CI), and what are its benefits? What are the different CI tools available in the Industry?
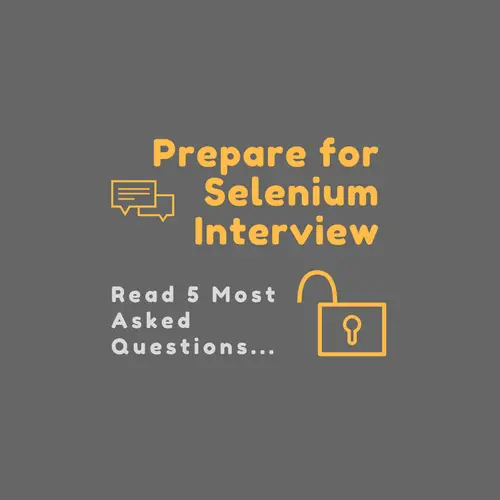
List of 5 Most Asked Selenium Questions to Know
Also, if you like to revise your core Selenium knowledge and want to prepare thoroughly, then you should consider reading the below tutorial.
1) What types of data have you handled in Selenium for automating web applications?
Data is probably a very critical and important piece of information in ensuring that Automation can run successfully. It can be in a variety of form factors to serve different purposes. For example, in automation, we use input data that includes test cases.
This is one of the most asked interview questions for writing a Selenium test suite. Here are some of the commonly used mechanisms to handle data in automation.
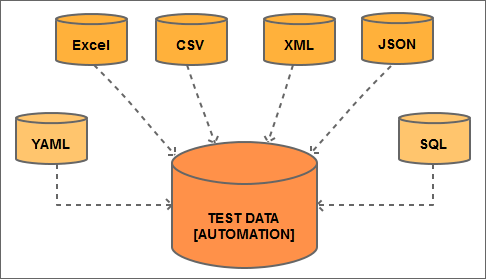
1. Excel data.
It is the most popular data format used widely by automation testers. We can not only use it to store test cases but to keep test data as well.
This format is very flexible and supports CRUD (Create /Delete /Update /Delete) operations. Almost all languages that integrate with Selenium like Java, Python, and Ruby have ready-made libraries available to access Excel data.
2. CSV data.
CSV stands for comma-separated values. It is the most simplistic way of organizing test data in tabular format. It represents a sequence of rows and columns (delimited by commas) stored in a text file with CSV as the file extension. Each row corresponds to a record whereas every column maps to a field.
3. XML data.
XML is an acronym for Extensible Markup Language. It generates documents in a format that is readable by both humans and machines. XML is a web standard and tends to be faster than Excel when it comes to reading or writing data.
<testsuite name="TestSuite"> <test id="1" desc="Create a new document" > <step value="New" onclick="CreateNew()" /> <step value="Open" onclick="Open()" /> <step value="Close" onclick="Close()" /> </test> </testsuite>
4. JSON data.
JSON is an acronym for JavaScript Object Notation. It supports a lightweight data interchange format. Since JSON data is compact and fast, it has become a preferred choice for supplying input in web services.
We can also use it to represent the test data replacing Excel and XML formats. If the size of data is invariably large, then JSON would surely deliver better performance.
{ "testsuite": { "test": { "step": [ {"_value": "New", "_onclick": "CreateNew()"}, {"_value": "Open", "_onclick": "Open()"}, {"_value": "Close", "_onclick": "Close()"} ], "_id": "1", "_desc": "Create a new document" }, "_name": "TestSuite" } }
5. YAML data.
YAML is another human-readable language for data serialization. It stands for YAML Ain’t Markup Language. This type of data format is ideal for holding configurations. However, it could be useful for various other purposes.
Unlike other data formats, it allows referencing other elements inside YAML using anchors.
testsuite: test: step: - _value: New _onclick: "CreateNew()" - _value: Open _onclick: "Open()" - _value: Close _onclick: "Close()" _id: 1 _desc: "Create a new document" _name: TestSuite
6. SQL data.
Another type of data that doesn’t get used often is SQL data. It is highly customizable and enables fast data access. It can store both the test cases and any configuration or settings as required.
However, there is a separate database software like MySQL/MongoDB/SQL/Oracle needed to make it work.
2) What are various build and deploy tools apart from Maven used in the industry?
The use of Maven is widespread not just because it builds code or manages dependencies on the fly but also due to its ability to support deployments. So it eases up a lot of post-development tasks. However, we can’t ignore the challenges that show up while working with complex commands and XML configuration. It is the second most asked selenium question.
Also Read: Create Selenium Project using Maven
Hence, here are a bunch of other tools that are worth trying.
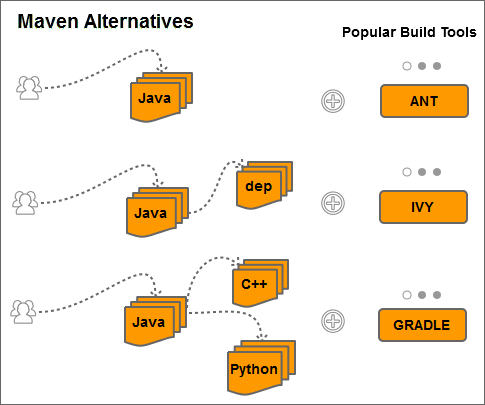
Ant.
ANT is an acronym for Another Neat Tool. It is a command-line tool developed by James Duncan Davidson and written in Java. It makes use of XML configuration to build modules, define targets, and specify dependencies.
<?xml version="1.0"?> <project name="Demo" default="compile"> <target name="cleanup" depends="clean" description="perform cleanup"> <delete file="hello.jar"/> </target> <target name="compile" description="build a java module"> <mkdir dir="classes"/> <javac srcdir="." destdir="classes"/> </target> </project>
IVY.
Ivy is another build tool from Apache and brought as an extension to Ant. It helps in managing the external dependencies during the development and provides a way of adding them to the classpath and packaging them with the application.
It is a perfect lightweight alternative to Maven.
Gradle.
Gradle is an open-source build tool. It automates the build process taking over Ant and Maven tools. Unlike its predecessors, Gradle makes use of a domain-specific language (DSL) instead of XML templates for defining the project configuration.
Must Read: The Best 30 Node.js Interview Questions and Answers
3) What is WebdriverIO? Which technology does it use? Why should QA use it for UI automation?
WebdriverIO is a powerful framework for testing mobile and web applications. It uses JavaScript to implement the Selenium Webdriver API and the wire protocol as per the W3C standards. It is available for installation as an NPM package and runs on Node.js. Hence, it is also famous for the name “Selenium 2.0 bindings for NodeJS”.
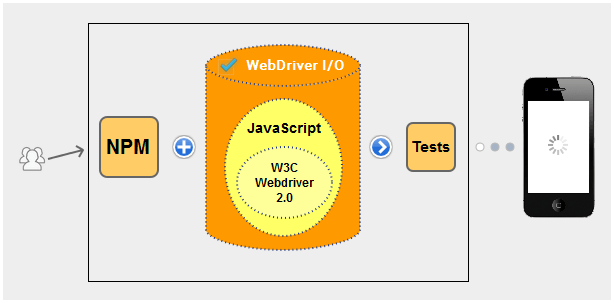
It is the third most asked selenium question. Here, we are listing down a few of the silent features of WebdriverIO.
WebdriverIO Features.
- It is a multipurpose automation tool that can fit in to test both the web applications and the native mobile Apps.
- Command execution takes place asynchronously in WebdriverIO. However, we can write them in a synchronous way to avoid any race conditions that may occur while handling a promise.
- WebdriverIO provides a set of simple JavaScript functions to write tests that are lean, fast, and easy to understand.
- It comes with an intelligent Test Runner that optimizes test execution to achieve maximum concurrency.
- Test runner also adds a feature to register hooks for handling errors and altering test flow based on previous test results.
- It integrates seamlessly with CI tools like Jenkins and utilizes JUnit reporter to debug issues and track test execution.
The primary objective of WebdriverIO is to automate end-to-end testing on a large scale. Its test runner helps in building a reliable Test Suite that is easy to understand and manage.
4) What are the different network protocols that Selenium supports? Also, how will you handle them in Selenium?
HTTP and HTTPS are the two most commonly used network protocols. And in Selenium, there are multiple ways to handle both of these protocols.
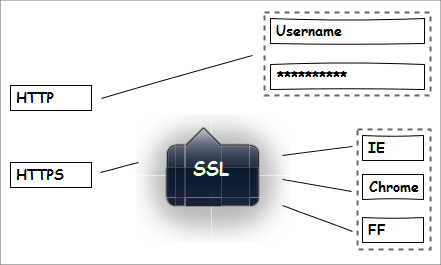
Handle HTTP protocol.
A website not using authentication.
If a website is only using HTTP and does not require any authentication, then it’s very easy to handle in Selenium.
WebDriver driver = new ChromeDriver(); driver.get("http://destination-url/");
Web site using basic authentication.
There are many websites that apply a basic authentication scheme before allowing access to their home page. Here are the three most prominent ways to do it in Selenium.
The below code uses WebDriverWait and Alert classes to implement basic authentication for HTTP.
WebDriverWait testwait = new WebDriverWait(driver, 10); Alert testalert = testwait.until(ExpectedConditions.alertIsPresent()); testalert.authenticateUsing(new UserAndPassword(**user**, **pass**));
Alternatively, we can also pass the user/pass pair within the HTTP URL as a parameter to the Webdriver’s Get method.
String target = “http://user:pass@host”;
public void login(String username, String password){ WebDriver driver = new ChromeDriver(); String URL = "http:// + username + ":" + password + "@" + "website link"; driver.get(URL); driver.manage().window().maximize(); }
We can also perform basic authentication by setting up browser preferences using Selenium’s profile classes.
FirefoxProfile FF = new FirefoxProfile(); FF.setPreference("network.http.phishy-userpass-length", 255); driver = new FirefoxDriver(FF); driver.get("http://user:pass@www.targetsite.com/");
Handle HTTPS protocol.
HTTPS is the secure version of HTTP. And it encrypts all the communication exchanged between the web server and the browser. So, we need to handle the SSL certificates via Selenium.
Handle SSL in Firefox.
In Firefox, we can enable SSL via Selenium Profile APIs.
First of all, we load the profile of the running browser instance.
ProfilesIni pf = new ProfilesIni(); FirefoxProfile ff = pf.getProfile ("myProfile");
Consequently, we have to set the following two properties to avoid any security exception that might occur while opening the HTTPS site.
ff.setAcceptUntrustedCertificates(true); ff.setAssumeUntrustedCertificateIssuer(false);
Finally, we can create the Firefox driver instance by using the profile object.
WebDriver driver = new FirefoxDriver (ffProfile);
Handle SSL in Chrome.
Unlike Firefox, Chrome requires setting up the SSL options via the desired capabilities of Selenium Webdriver. Here is the code to suppress all SSL errors and accept all the SSL certificates in Chrome.
First of all, we’ll prepare the DesiredCapabilities instance in our code.
DesiredCapabilities set_ssl = DesiredCapabilities.chrome(); set_ssl.setCapability (CapabilityType.ACCEPT_SSL_CERTS, true); WebDriver driver = new ChromeDriver (set_ssl);
5) What is Continuous Integration (CI), and what are its benefits? What are the different CI tools available in the Industry?
It is one of the most important and frequently asked selenium questions.
Continuous Integration is a software engineering technique that regulates the development process by automating the build and testing of code for every commit made to the version control. It greatly helps in making sure that new changes don’t have any adverse effect on the build and that existing functionality is intact.
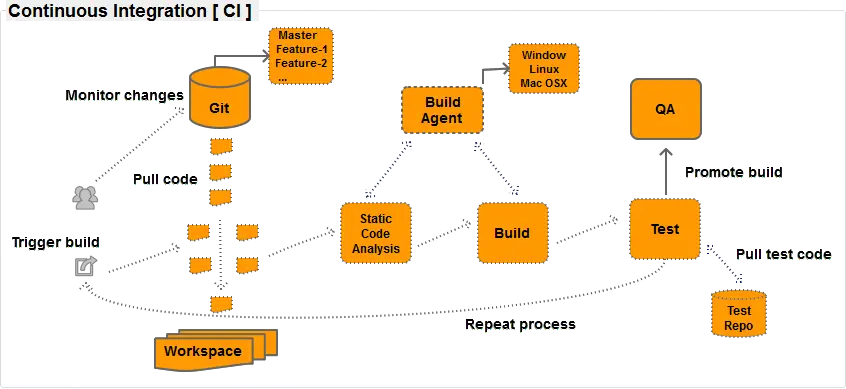
It promotes best practices amongst the developers by making them merge their changes with others’ code before checking into a shared repository.
There are many CI tools available that can assist in implementing the continuous integration process. Here we are listing a few of the popular ones.
1. Jenkins.
Jenkins is undoubtedly the most popular CI tool used across the IT industry. It is open source, written in Java, and created by Kohsuke Kawaguchi.
You can easily manage CI tasks via commands as well as from its UI. It supports a rich set of plugins to customize both build and automation jobs.
2. Bamboo.
Bamboo is another great CI tool but a paid one. It’s from Atlassian, the company that provides helping tools like JIRA, and BitBucket for software development. Unlike Jenkins which uses Build/Automation jobs terminology, Bamboo has introduced the concepts of projects and plans. It targets to bring more user-friendly features so that the user can implement CI with ease.
Some other CI tools are TeamCity, Travis CI, and Circle CI.
Conclusion
However, this post has only five Selenium questions. But all of them are quite critical for the interview purpose. We did our best to simplify the answers so that it is easy for you to understand them quickly.
Also, we picked these questions at the request of our readers. If you have any query or question to ask, then please do write to us.
Best,
TechBeamers.