TestNG is a time-tested Swiss knife for automation engineers around the world. It is still the most useful testing tool to augment the functionality of your Selenium project. Hence, we have rewired this post with the 35 most trustworthy TestNG interview questions that will not only evaluate your hands-on skills but also put them to the test. Our goal is not only to help you master the subject but to see you do well during the interviews. That’s why we have written answers with absolute simplicity to make learning more effective.
TestNG was developed mainly as a unit testing framework for Java developers. However, it has helped the test engineers immensely in creating revolutionary Selenium automation test suites. They have been using it for both functional and regression testing.
35 TestNG Interview Questions to Get Results
If you are already using TestNG in your projects, then please proceed directly to the TestNG interview questions. For those who are just beginning to learn TestNG, we highly recommend the following two tutorials.
Let’s now dive into the latest set of TestNG interview questions and try to learn from their answers.
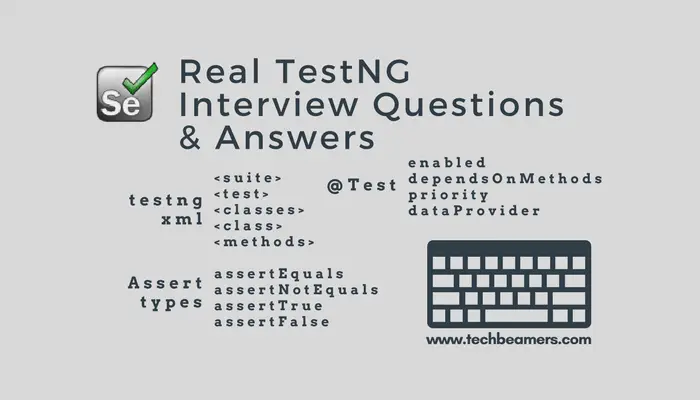
TestNG Interview Questions – Basic Concepts
Firstly, we’ll focus on the basic concepts of TestNG, including its definition, core features, standard annotations, assertions, and differences between hard and soft assertions.
Q-1: What is TestNG, and why should you use it?
Answer: TestNG is an innovative test framework built on top of the JUnit framework. Its primary consumers are Java developers who use it for unit testing purposes.
It comes with many useful features like TestNG annotations and TestNG assertions that give a lot of flexibility to testers. Also, there are a couple of solid reasons to use TestNG for automation testing.
- The reporting in the TestNG framework is one of its cornerstone features. It can generate reports both in HTML and XML format. The report provides many indicators for predicting the health of the project. It includes the count of passed/failed cases, the age of a defect, and a lot more.
- Since Selenium doesn’t have a built-in report generator, the TestNG report is an ideal candidate to be used for Selenium report generation.
- TestNG provides an excellent exception-handling mechanism to ensure the seamless execution of your test automation project.
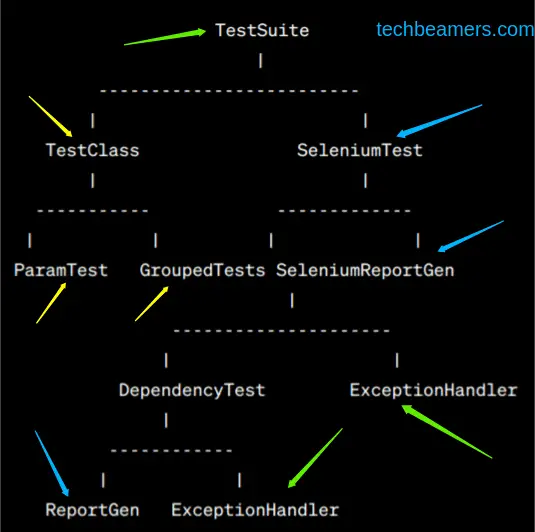
Q-2: What are the core features of the TestNG framework?
Answer:
Feature | Description |
---|---|
Concurrency | Supports running multiple tests in parallel. |
Dependency | Allows adding test method dependencies. |
Prioritization | Specifies test execution order using the “priority” parameter. |
Grouping | Enables efficient distribution of tests into groups. |
Parameterization | Supports passing values to test methods. |
Data Sourcing | Introduces data provider annotation for external data sources. |
Q-3: What are the standard annotations available in TestNG?
Answer: TestNG annotations control the way the test method below them will get executed. They begin with the “@” symbol.
//TestNG annotation syntax
@<annotation-name>(<attribute-name>=<value>)
public void SampleTest() {
...
}
In the following table, we’ve listed the most common TestNG annotations.
Annotation | Description |
---|---|
Test | Denotes a test method. |
BeforeMethod | Runs before each test method. |
AfterMethod | Runs after each test method. |
BeforeClass | Runs before the first test method in a class. |
AfterClass | Runs after the last test method in a class. |
BeforeTest | Runs before any test method belonging to the classes inside the <test> tag in the XML file. |
AfterTest | Runs after all the test methods belonging to the classes inside the <test> tag in the XML file. |
BeforeSuite | Runs before all tests in a suite. |
AfterSuite | Runs after all tests in a suite. |
BeforeGroups | Runs after the execution of a group of test methods. |
AfterGroups | Runs after the execution of a group of test methods. |
DataProvider | Supplies data to test methods. |
Factory | Marks a method as a factory that returns objects that will be used as test class instances. |
Listeners | Represents a set of annotations to define listeners for test classes. |
Parameters | Represents parameters that can be passed to test methods. |
Q-4: What are the standard assertions available in TestNG?
Answer: Assertions are the essential tools for testers to debug if a test case fails. It not only lets us add validation to the tests but also helps decide their state (Passed/Failed).
Assertion | Description |
---|---|
assertEquals | Passes if the arguments match or fail otherwise. |
assertNotEquals | Fails if the actual and expected values match. |
assertTrue | Passes if the input condition is true, otherwise throws AssertionError. |
assertFalse | Passes if the input condition is false, otherwise throws AssertionError. |
assertNull | Performs the null test on an object. In case of null, the test aborts with an exception. |
assertNotNull | The opposite of “assertNull.” The test method breaks with an exception upon discovering a non-null object. |
Q-5: What are the key differences between TestNG’s hard and soft assertions?
Answer:
Hard assertions break a test immediately with an AssertException after the assert method reports failure. Soft assertions, on the other hand, collect all errors until test completion, allowing the execution of all steps even if one fails.
TestNG Interview Questions – Advanced Features
In this section, you’ll find questions related to advanced features of TestNG, such as the purpose of the “TestNG.XML” file, running tests without the XML file, passing parameters using XML, and running tests in parallel.
Q-6: What is the purpose of the “TestNG.XML” File?
Answer:
The “TestNG.XML” file is a configuration file containing TestNG project settings and representing a TestNG suite. It allows customizations such as setting up multiple tests, including/excluding test cases, selecting specific groups, supplying parameters, introducing group-level dependencies, setting up test parallelization, and configuring listeners.
Q-7: Can you run a TestNG test without the “TestNG.XML” file? If Yes, then how?
Answer:
Yes, a TestNG test can be run from the command line using Ant or Maven by passing the required class, method, or group name in the command. However, using TestNG features without the “TestNG.XML” file is limited.
Q-8: How to pass a string value to a test using the “TestNG.XML” file?
Answer:
To pass a string value using the “TestNG.XML” file, use the parameter tag inside the suite and access the string value using the parameter annotation in the Java code.
<suite name="TestNG Param Suite" verbose="1">
<parameter name="my-string-param" value="my string value" />
<!-- other suite configurations -->
</suite>
Q-9: How to pass an optional parameter to a test using the “TestNG.XML” file?
Answer:
To pass an optional parameter, use the “@Optional” annotation in the Java code and define the parameter in the “TestNG.XML” file.
<suite name="Optional Param Suite" verbose="1">
<test name="Optional Param">
<parameter name="opt-value" value="optional text" />
<!-- other test configurations -->
</test>
</suite>
Q-10: How to run multiple TestNG tests in parallel?
Answer:
TestNG supports concurrent executions of tests, and parallelization can be achieved by setting the “parallel” attribute in the TestNG XML file at different levels such as Tests, Classes, Methods, or Instances.
TestNG Interview Questions – Execution and Integration
In this section, we focused on questions related to execution-related aspects and integration with tools like Maven. It covers several real situations such as blocking test methods, test dependency, controlling test execution order, and running tests using Maven.
Q-11: How to block a test method from execution in TestNG?
Answer:
To block a test method, set the “enabled” attribute to “false” in the @Test annotation.
Q-12: How to block a TestNG test depending on a condition?
Answer:
Utilize TestNG’s annotation transformer to initialize the disabled property of a @Test annotation based on a condition.
Q-13: How to make a test dependent on another in TestNG?
Answer:
Use the “dependsOnMethods” attribute in the @Test annotation to declare dependencies between test methods.
Q-14: How to control the order of test execution in TestNG?
Answer:
Control the order of test execution by setting the “preserve-order” attribute to “true” in the “TestNG.XML” file or specifying priorities with the “priority” attribute.
Q-15: What if the priority of a test case in TestNG is zero?
Answer:
A test case with a lower priority value takes precedence, and by default, every test case gets a zero priority.
Q-16: What is DataProvider annotation in TestNG and why do you use it?
Answer:
The “@DataProvider” annotation in TestNG allows writing data-driven tests by accepting input from external sources like CSV or Excel, enhancing flexibility and supporting large datasets.
Q-17: What is Factory annotation in TestNG and why do you use it?
Answer:
The “@Factory” annotation dynamically produces tests at runtime, solving the problem of
executing tests with different datasets without explicit creation.
Q-18: How is the @Factory annotation different from the @DataProvider?
Answer:
@Factory creates instances of a test class at runtime, executing all test methods, while @DataProvider is only applicable to the test methods immediately following it, running specific methods multiple times.
Q-19: What are Listeners in TestNG and why do you use them?
Answer:
Listeners in TestNG monitor different states of tests, extending the "org.testng.ITestListener"
interface. They improve test behavior and include various types such as IExecutionListener, ISuiteListener, ITestListener, and more.
Q-20: What are the methods available in TestNG’s ITestListener interface?
Answer:
ITestListener interface includes methods like onStart, onTestSuccess, onTestFailure, onTestSkipped, onTestFailedButWithinSuccessPercentage, and onFinish, capturing events during different test states.
Q-21: How do you group tests at the method level in TestNG?
Answer:
Group tests at the method level by specifying group names in the “groups” attribute of the @Test annotation before test methods.
Q-22: How do you group tests at the class level in TestNG?
Answer:
Group tests at the class level by annotating the class with @Test and using the “groups” attribute, which applies to all public methods of the class.
Q-23: How will you execute tests from a TestNG group?
Answer:
Execute tests from a TestNG group via the TestNG XML file by including/excluding groups or directly from Eclipse IDE by configuring Run Configurations.
Q-24: How to run a single TestNG test using Maven?
Answer:
Run a single TestNG test using Maven with the command:
mvn -Dtest=<Test Class>#<Test Method> test
Q-25: How to run a set of TestNG groups using Maven?
Answer:
Run a set of TestNG groups using Maven with the command:
mvn test -Dgroups=story1,story2,story3
These questions cover various aspects of TestNG, from its basic concepts to advanced features and integration with Maven and other tools.
Testing Your Practical Coding Knowledge
In this section, we aim for those TestNG interview questions that could easily assess your practical coding knowledge. It includes questions about blocking tests based on conditions, grouping tests at different levels, executing tests from specific groups, and running TestNG tests using Maven.
Q-26: How do you implement parallel test execution for methods within a test class?
Parallel test execution is crucial for optimizing the performance of our test suite. There are two primary ways to achieve this in TestNG – at the method level and through XML configuration.
Firstly, at the method level, we can use the parallel attribute within the @Test annotation. For example, set the parallel = “methods” to run the test methods in parallel. Here’s a simple example:
@Test(priority = 1, parallel = "methods")
public void test1() {
// Test logic here
}
Secondly, at the XML configuration level, we can set the “parallel” attribute in the TestNG XML file. For instance, to run entire tests in parallel set the parallel=”tests” in the <suite>
tag. Here’s a snippet:
<suite name="suite" parallel="tests">
<test name="Test1">
<!-- Test1 config -->
</test>
<test name="Test2">
<!-- Test2 config -->
</test>
</suite>
Now, when it comes to ensuring thread safety, it’s crucial to address any potential conflicts between concurrently executing test methods. This involves strategies such as using thread-local variables, synchronization mechanisms, or designing tests to be state-independent.
Q-27: Can you demonstrate the use of TestNG’s data provider with an external data source, such as a CSV file?
Certainly! Below is an example of using TestNG’s data provider with an external CSV file:
@DataProvider(name = "csvData")
public Object[][] provideDataFromCSV() {
// Logic to read data from a CSV file and return a 2D array
}
@Test(dataProvider = "csvData")
public void testWithCSVData(String param1, int param2) {
// Test logic using parameters from the CSV file
}
In the below screenshot, we’ve presented the code to read the CSV file using the TestNG data provider.
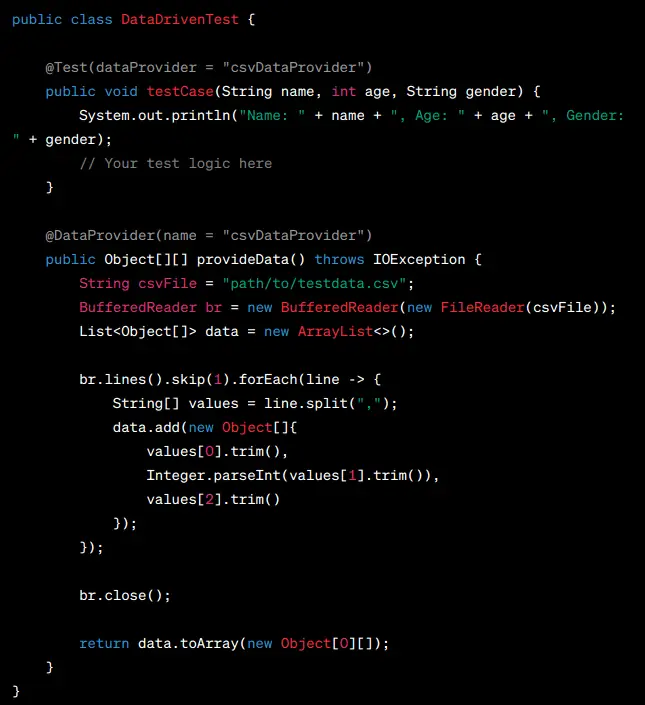
Q-28: How would you perform cross-browser testing using TestNG?
To perform cross-browser testing using TestNG, we need to define multiple test methods, each targeting a specific browser, and use the parallel execution feature in the TestNG XML file. It is also important to ensure the correct config and handling for each browser.
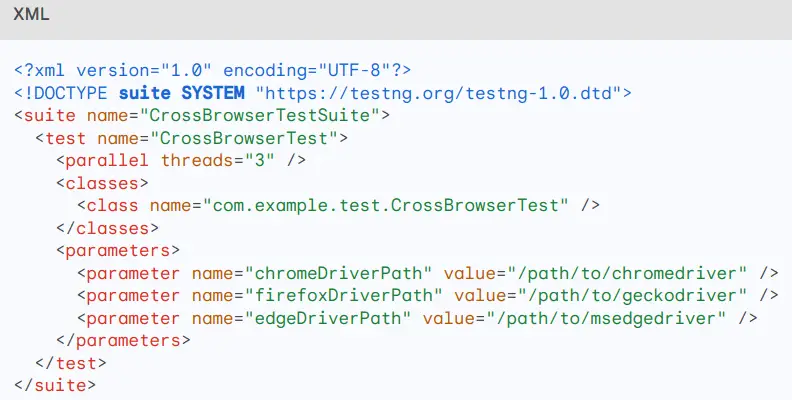
Q-29: Explain the use of TestNG listeners in handling test failures and successes.
TestNG listeners, such as ITestListener, provide methods like onTestSuccess and onTestFailure. We can implement these methods to customize actions upon test success or failure, such as taking screenshots of failure or logging additional information.
To implement a TestNG listener, firstly, we create a class that implements one of the TestNG listener interfaces. After that, we register the listener with TestNG. We can do this by adding the @Listeners
annotation to our test class or test suite.
Here is a demo screenshot to illustrate the use of listeners.
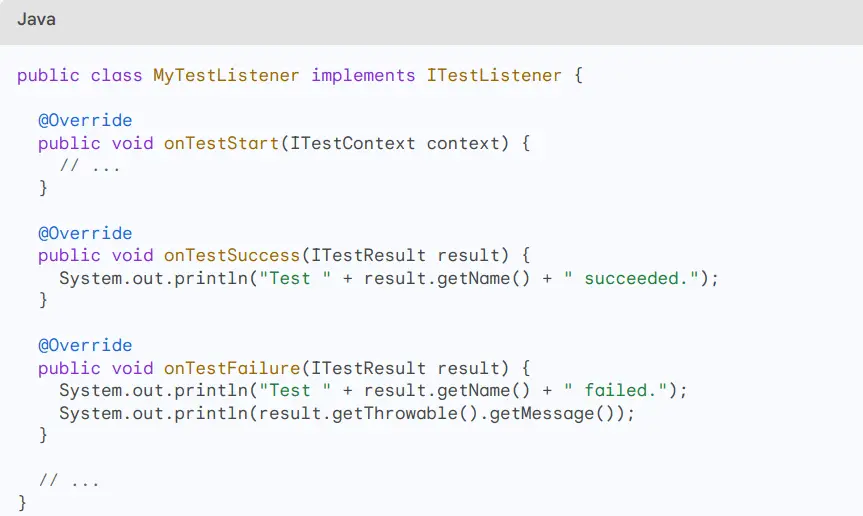
Q-30: Share an example of using TestNG’s grouping feature to run specific sets of tests.
@Test(groups = "smoke")
public void smokeTest() {
// Smoke test logic
}
@Test(groups = "regression")
public void regressionTest() {
// Regression test logic
}
In the TestNG XML file:
<suite name="TestSuite">
<test name="SmokeTests">
<groups>
<run>
<include name="smoke" />
</run>
</groups>
<classes>
<!-- Include relevant test classes -->
</classes>
</test>
</suite>
Q-31: How can you rerun failed tests automatically in TestNG?
Use the “rerun-failed” attribute in the TestNG XML file to automatically rerun failed tests. Specify the maximum number of retry attempts and other relevant configurations.
Q-32: Share a scenario where you would use TestNG’s parameterization feature.
@Parameters({"username", "password"})
@Test
public void loginTest(String username, String password) {
// Login test logic using parameters
}
In the TestNG XML file:
<suite name="ParameterizedSuite">
<test name="LoginTest">
<parameter name="username" value="testuser" />
<parameter name="password" value="password123" />
<classes>
<!-- Include relevant test classes -->
</classes>
</test>
</suite>
Q-33: Explain the use of TestNG factories in managing test instances.
TestNG factories, marked with the “@Factory” annotation, enable the dynamic creation of test instances with varying parameters. This is useful when you want to execute the same test logic with different inputs.
Q-34: How can you skip a test conditionally in TestNG?
Use the “skip” attribute in the @Test annotation along with a condition. For example:
@Test
public void conditionalTest() {
if (conditionIsMet) {
// Test logic
} else {
throw new SkipException("Test skipped due to a condition.");
}
}
Q-35: Demonstrate the use of TestNG listeners to log test execution details.
Implement a custom listener by extending ITestListener, override the onTestStart, onTestSuccess, and onTestFailure methods, and include logging statements to capture test execution details.
These practical coding knowledge questions assess the candidate’s ability to apply TestNG concepts in real-world scenarios.
Conclusion
The above TestNG interview questions would undoubtedly help you during a real-time faceoff with the interviewer. We recommend reading them carefully and please do that 2-3 times at least.
You can follow us on our social media accounts to see more technical and practical stuff.
Best,
TechBeamers