In this tutorial, you’ll learn how to use TestNG assertions and know the different methods to assert conditions. While using Selenium for automated testing of web applications, we need to add validations in our tests to report them as pass or fail. Assertions can let us do that within a single line of code.
Assertions give you a way (other than If-Else blocks) to test conditions. They are not only easy to use but also eliminate the chances of making errors in test conditions. Hence, it’s always beneficial to use them in Selenium Webdriver projects.
Moreover, in this tutorial, we’ll demonstrate TestNG assertions with the help of a POM framework. We’ll add simple page object tests and call different TestNG methods to assert them.
If you are starting afresh with Selenium TestNG, then read the below tutorial.
READ – How to Create a Simple TestNG Project in Eclipse
TestNG Assertions in Selenium
Table of Index
- Types of Assertions
- Assert Methods Available in TestNG
- Setup a Demo POM Framework for Testing Assertions
- Add Page Object Tests and Use Assertions
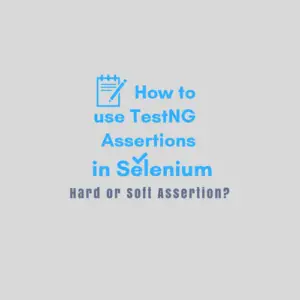
1- Types of Assertions
First of all, let us understand what the different types of assertions available in TestNG are and when to use them.
1.1- Hard Assertion
It is the default assert mechanism built into TestNG’s “org.testng.Assert” package. We use it when a test has to stop immediately after the assertion fails.
Here are two scenarios to understand the concept.
Hard Assertion – Scenario(1)
Follow the below code, which includes multiple assert calls, all of which get passed, and so the test case.
package com.techbeamers.hardassertion; import org.testng.Assert; import org.testng.annotations.Test; public class HardAssertion { String className = "HardAssertion"; @Test public void test_UsingHardAssertion() { Assert.assertTrue(true == true); Assert.assertEquals("HardAssertion", "HardAssertion"); Assert.assertEquals(className, "HardAssertion"); System.out.println("Successfully passed!"); } }
You can see the message “Successfully passed!” appearing in the execution output.
... Successfully passed! [Utils] Attempting to create C:\workspace\work\selenium\TestNGAssertions\test-output\Default suite\Default test.xml [Utils] Directory C:\workspace\work\selenium\TestNGAssertions\test-output\Default suite exists: true PASSED: test_UsingHardAssertion =============================================== Default test Tests run: 1, Failures: 0, Skips: 0 ===============================================
Hard Assertion – Scenario(2)
In this scenario, the second assert call fails, which leads to the end of the test case.
package com.techbeamers.hardassertion; import org.testng.Assert; import org.testng.annotations.Test; public class HardAssertion { String className = "HardAssertion"; @Test public void test_UsingHardAssertion() { Assert.assertTrue(true == true); Assert.assertEquals("HardAssert", "HardAssertion"); Assert.assertEquals(className, "HardAssertion"); System.out.println("Successfully passed!"); } }
After the expected string didn’t match the original, the execution of the test case stopped immediately.
... FAILED: test_UsingHardAssertion java.lang.AssertionError: expected [HardAssertion] but found [HardAssert] ...
1.2- Soft Assertion
It is a custom assert mechanism supported by TestNG’s org.testng.asserts.Softassert package. We use it when a test has to continue execution even after an assertion fails in the sequence.
Here, we again have two scenarios to explain Soft assertion.
First scenario
Follow the below code, which creates a Soft assertion object. Then, it includes multiple assert methods followed by an assertAll() call. The second assertion fails, but that doesn’t interrupt the execution. It is because of the assertAll() method that lets the other assert calls to complete.
package com.techbeamers.softassertion; import org.testng.annotations.Test; import org.testng.asserts.SoftAssert; public class SoftAssertion { SoftAssert softAssert = new SoftAssert(); String className = "SoftAssertion"; @Test public void test_UsingSoftAssertion() { softAssert.assertTrue(true == true); softAssert.assertEquals("SoftAssert", "SoftAssertion"); softAssert.assertEquals(className, "SoftAssertion"); System.out.println("Last statement gets executed!"); softAssert.assertAll(); } }
You can cross-check from the output below that the message “The Last statement gets executed!” appeared there even after one of the asserts calls failed.
... Last statement gets executed! [Utils] Attempting to create C:\workspace\work\selenium\TestNGAssertions\test-output\Default suite\Default test.xml [Utils] Directory C:\workspace\work\selenium\TestNGAssertions\test-output\Default suite exists: true FAILED: test_UsingSoftAssertion java.lang.AssertionError: The following asserts failed: expected [SoftAssertion] but found [SoftAssert] ...
Second scenario – An issue in using the Soft assertion
In this example, you can see that there are multiple test cases. They are using the same Soft assertion object. We added it to highlight the issue that occurs when one test failure makes other tests fail. It happens due to the use of the same assert object that evaluates all occurrences of assert methods despite being in different cases.
package com.techbeamers.softassertion; import org.testng.annotations.Test; import org.testng.asserts.SoftAssert; public class SoftAssertIssue { SoftAssert softAssert = new SoftAssert(); String className = "SoftAssertion"; @Test public void test1_UsingSoftAssertion() { softAssert.assertTrue(true == true); softAssert.assertEquals("SoftAssert", "SoftAssertion"); softAssert.assertEquals(className, "SoftAssertion"); softAssert.assertAll(); } @Test public void test2_UsingSoftAssertion() { softAssert.assertTrue(true == true); softAssert.assertEquals("SoftAssertion", "SoftAssertion"); softAssert.assertEquals(className, "SoftAssertion"); softAssert.assertAll(); } }
You can verify from the output below that both cases failed while only the first one had the error.
FAILED: test1_UsingSoftAssertion java.lang.AssertionError: The following asserts failed: expected [SoftAssertion] but found [SoftAssert] ... FAILED: test2_UsingSoftAssertion java.lang.AssertionError: The following asserts failed: expected [SoftAssertion] but found [SoftAssert] ... =============================================== Default test Tests run: 2, Failures: 2, Skips: 0 ===============================================
Third scenario – The right way to use the Soft assertion
The solution to the previous issue is to create a separate SoftAssert object for each test case. You can even use a Factory design pattern to instantiate it on the fly.
Please see the updated code below.
package com.techbeamers.softassertion; import org.testng.annotations.Test; import org.testng.asserts.SoftAssert; public class SoftAssertSolution { SoftAssert softAssert1 = new SoftAssert(); SoftAssert softAssert2 = new SoftAssert(); String className = "SoftAssertion"; @Test public void test1_UsingSoftAssertion() { softAssert1.assertTrue(true == true); softAssert1.assertEquals("SoftAssert", "SoftAssertion"); softAssert1.assertEquals(className, "SoftAssertion"); softAssert1.assertAll(); } @Test public void test2_UsingSoftAssertion() { softAssert2.assertTrue(true == true); softAssert2.assertEquals("SoftAssertion", "SoftAssertion"); softAssert2.assertEquals(className, "SoftAssertion"); softAssert2.assertAll(); } }
From the output below, you can see now both tests are now running without conflicting with each other.
... PASSED: test2_UsingSoftAssertion FAILED: test1_UsingSoftAssertion java.lang.AssertionError: The following asserts failed: expected [SoftAssertion] but found [SoftAssert] ... =============================================== Default test Tests run: 2, Failures: 1, Skips: 0 ===============================================
2- Get to Know the Assert Methods Available in TestNG
Let’s now quickly outline the methods that you can call from Selenium code for using TestNG assertions.
Methods | Description |
---|---|
1- assertEquals( | 1- It accepts two string arguments. 2- It’ll check whether both are equal. If not, it’ll fail the test. |
2- assertEquals( | 1- It accepts three string arguments. 2- It’ll test whether both are the same. If not, it’ll fail the test. 3- Also, it’ll throw a message that we provide. |
3- assertEquals( | 1- It assumes two boolean arguments. 2- It tests them for equality. If not, it’ll fail the test. |
4- assertEquals( | 1- It accepts two collection-type objects. 2- It checks whether they hold the same elements and in the same order. 3- It’ll fail the test if the above condition doesn’t meet. 4- Also, you’ll see the message appear in the report. |
5- assertTrue( | 1- It accepts one boolean argument. 2- It tests that the given condition is true. 3- If it fails, then an AssertionError would occur. |
6- assertTrue( | 1- It assumes one boolean argument and a message. 2- It asserts that the given condition is true. 3- If it fails, then an AssertionError would occur with the message you passed. |
7- assertFalse( | 1- It assumes one boolean argument and a message. 2- It asserts that the given condition is true. 3- If it fails, then an AssertionError will occur with the message you passed. |
8- assertFalse( | 1- It assumes one boolean argument and a message. 2- It asserts that the given condition is false. 3- If it fails, then an AssertionError would occur with the message you passed. |
3- Setup a Demo POM Framework for Using TestNG Assertions
You’ll need to prepare a simple POM project first to see how to use TestNG assertions. Our demo project will include the following Java files.
- These Java files will store the element locators and methods.
- HomePage.java
- ChapterFirstPage.java
- ChapterSecondPage.java
- This Java file defines the base test case class to provide standard methods.
- TestBase.java
- In the below Java file, we write the page object tests to execute.
- MyTest.java
You can get the source code of the above files from the below post. Open this tutorial and copy/paste the code to your project.
READ – How to Implement Page Object Model (POM) in Selenium
It’s important to mention that we’ll modify these files in the next section. And then, we’ll demonstrate how to use the TestNG assertions.
4- Add Page Object Tests and Use TestNG Assertions
Here, we have given the code for each page object test. You’ll need to add it to the appropriate files in your project.
4.1- Adding Test Methods in ChapterFirstPage.java
- From the below code, you can see the last method, String getDropDownText().
- We’ve added it only for the sake of this post.
- Later, we’ll call it from the page tests and verify the results using TestNG assertions.
Code Sample
package com.techbeamers.page; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.support.FindBy; import org.openqa.selenium.support.PageFactory; import org.openqa.selenium.support.ui.Select; public class ChapterFirstPage { private WebDriver driver; @FindBy(id = "secondajaxbutton") WebElement secondajax; @FindBy(xpath = "//select[@id='selecttype']") WebElement dropdown; @FindBy(id = "verifybutton") WebElement verifybutton; public ChapterFirstPage(WebDriver driver) { this.driver = driver; } // Method-1. public ChapterFirstPage clickSecondAjaxButton() { secondajax.click(); return PageFactory.initElements(driver, ChapterFirstPage.class); } // Method-2. public ChapterFirstPage clickSecondAjaxButton1(String data1) { System.out.println(data1); return PageFactory.initElements(driver, ChapterFirstPage.class); } // Method-3. public ChapterFirstPage selectDropDown(String value) { new Select(dropdown).selectByVisibleText(value); return PageFactory.initElements(driver, ChapterFirstPage.class); } // Method-4. public ChapterFirstPage verifyButton() { verifybutton.click(); return PageFactory.initElements(driver, ChapterFirstPage.class); } // Method-5. public String getDropDownText() { return new Select(dropdown).getFirstSelectedOption().getText(); } }
4.2- Adding Page Object Tests in MyTest.java
- In this module, you can see that we’ve added four new test cases. e.g. Test-1, Test-2, Test-3, and Test-4.
- To verify the test results, we’ll use the TestNG assertions.
- In each of the cases, there is a different assertion method called to test the condition.
Code Sample
package com.techbeamers.scripts; import org.openqa.selenium.support.PageFactory; import org.testng.Assert; import org.testng.annotations.Test; import com.techbeamers.page.HomePage; import com.techbeamers.util.TestBase; public class MyTest extends TestBase { // Test-0. (disabled) @Test(enabled = false) public void testPageObject() throws Exception { homePage = PageFactory.initElements(driver, HomePage.class); driver.get(baseUrl); chapterSecond = homePage.clickChapterSecond(); chapterSecond.clickbut2(); chapterSecond.clickRandom(); String data = chapterSecond.getTest(); homePage = chapterSecond.clickIndex(); chapterFirstPage = homePage.clickChapterFirst(); chapterFirstPage.clickSecondAjaxButton(); chapterFirstPage.clickSecondAjaxButton1(data); chapterFirstPage.selectDropDown("Selenium Core"); chapterFirstPage.verifyButton(); } // Test-1. @Test public void testAssertSuccess() throws Exception { homePage = PageFactory.initElements(driver, HomePage.class); driver.get(baseUrl); Thread.sleep(1000); // Intentional pause. chapterFirstPage = homePage.clickChapterFirst(); Thread.sleep(500); // Intentional pause. chapterFirstPage.selectDropDown("Selenium Core"); Thread.sleep(1000); // Intentional pause. Assert.assertEquals("Selenium Core", chapterFirstPage.getDropDownText()); } // Test-2. @Test public void testAssertTrue() throws Exception { homePage = PageFactory.initElements(driver, HomePage.class); driver.get(baseUrl); Thread.sleep(1000); // Intentional pause. chapterFirstPage = homePage.clickChapterFirst(); Thread.sleep(500); // Intentional pause. chapterFirstPage.selectDropDown("Selenium Core"); Thread.sleep(1000); // Intentional pause. Assert.assertTrue(chapterFirstPage.getDropDownText().equalsIgnoreCase("Selenium Core")); } // Test-3. @Test public void testAssertFailure() throws Exception { homePage = PageFactory.initElements(driver, HomePage.class); driver.get(baseUrl); Thread.sleep(1000); // Intentional pause. chapterFirstPage = homePage.clickChapterFirst(); Thread.sleep(500); // Intentional pause. chapterFirstPage.selectDropDown("Selenium Core"); Thread.sleep(1000); // Intentional pause. Assert.assertEquals("Selenium RC", chapterFirstPage.getDropDownText()); } // Test-4. @Test public void testAssertFailureWithMessage() throws Exception { homePage = PageFactory.initElements(driver, HomePage.class); driver.get(baseUrl); Thread.sleep(1000); // Intentional pause. chapterFirstPage = homePage.clickChapterFirst(); Thread.sleep(500); // Intentional pause. chapterFirstPage.selectDropDown("Selenium Core"); Thread.sleep(1000); // Intentional pause. Assert.assertEquals("Selenium RC", chapterFirstPage.getDropDownText(), "Invalid Component Selected!!!\n\t"); } }
4.3- Execute POM Tests
Now it’s time to see the project running. We hope you have merged the above code with the POM framework source code. And we are all set to run it. Though, we indeed are, and here is a screenshot of the execution that we ran at our end. You should also see a similar report on your side.
Summary – How to Use TestNG Assertions in Selenium
Since this post was one of our readers’ choices, we tried to bring all the required ingredients into it. Hopefully, it’ll carry its benefits for the other readers as well.
If you have any questions about using TestNG assertions in your projects, then please speak about it. And we’ll try to address it here.
Best,
TechBeamers