Whether we are working on a Webdriver project or building an online sample for learning, it’s the Firefox browser we mostly use to run the test cases. But it’s also a fact that it fails quite often due to an incompatible version of the Selenium Jar. So the next best alternative is to use Chrome for running Webdriver test cases.
You can use Firefox by merely adding the Selenium Jar, but you would need an additional driver module for Chrome to run test cases. Hence, in this tutorial, we thought to address this issue. So we came up with a practical example for running Webdriver test cases in Chrome.
However, we’d recently done a post on the Selenium TestNG interview questions where we gave tips to solve a similar type of issue. So you might also like to read it to know about these problems and their solution.
Firefox failing to run Webdriver is real. Also, you must believe that it’s not an imaginary issue with Firefox. We’d ourselves faced it with Firefox v47.0.0 and Selenium Jar 2.53.0. You may even check it on the Stackoverflow site where people make queries asking for a solution. Nonetheless, this issue now stands resolved in Firefox v47.0.1 and Selenium Jar 2.53.1. But this incident led us to choose Chrome for running Webdriver test cases.
How to Use Chrome for Running Webdriver Test Cases?
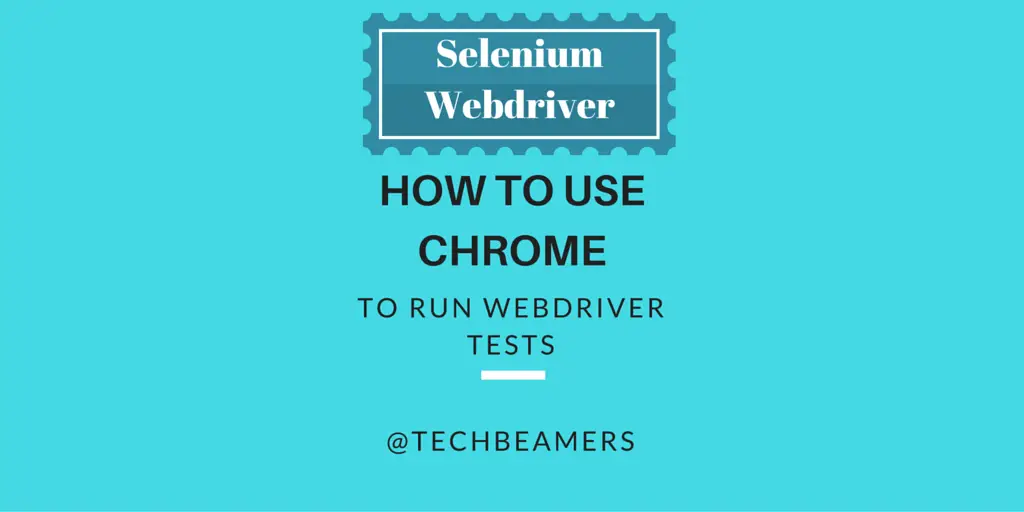
As we already mentioned above barring Firefox, Selenium requires other browsers to provide their driver modules for supporting Webdriver. These drivers are executable files that work as a server for the respective browser. Let’s now read the follow-up instructions to set up the Chrome driver for running web driver tests.
To run WebDriver in the Chrome browser, we require ChromeDriver which is a separate executable that Selenium WebDriver uses to control Chrome. ChromeDriver gets support from the Chromium team. It’s a standalone server that implements the WebDriver’s wire protocol for Chromium.
1- How to set up Chrome driver in Selenium?
1.1- First of all, download the latest version of the ChromeDriver server. You can download it by clicking the below link.
1.2- You have to select the Chrome driver based on your working environment. If you are working on Windows, then click on the <Chromedriver_win32.zip>. After that save the downloaded file to your local machine.
1.3- Next, you’ve to set the property for Chrome driver in the WebDriver code. Here, you need to provide the location of the ChromeDriver executable. See the code given below.
System.setProperty("webdriver.chrome.driver", "pathofChromeDriveronlocalsystem\\chromedriver.exe");
Let’s see an example program written in Java. It’ll help you in running Webdriver test cases.
2- Example: Using Chrome to run Webdriver Tests.
In this example, the sample code would do the following.
1- While executing the below code, the Google Home Page will open in Chrome browser.
2- Then, we’ll validate the page title in our example.
2.1- Sample code for Running Webdriver Test Cases.
package com.techbeamers.exercises; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Assert; import org.testng.annotations.AfterClass; import org.testng.annotations.BeforeClass; import org.testng.annotations.Test; public class RunWebdriverInChrome { static String driverPath = "C:\\workspace\\tools\\selenium\\"; public WebDriver driver; @BeforeClass public void setUp() { System.out.println("*******************"); System.out.println("launching chrome browser"); System.setProperty("webdriver.chrome.driver", driverPath + "chromedriver.exe"); driver = new ChromeDriver(); driver.manage().window().maximize(); } @Test public void testGooglePageTitleInIEBrowser() { driver.navigate().to("http://www.google.com"); String strPageTitle = driver.getTitle(); System.out.println("Page title: - " + strPageTitle); Assert.assertTrue(strPageTitle.equalsIgnoreCase("Google"), "Page title doesn't match"); } @AfterClass public void tearDown() { if (driver != null) { System.out.println("Closing chrome browser"); driver.quit(); } } }
2.2- Execution Summary.
After you run the above code, it should give you the following output. Please refer to the below screenshot.
Hopefully, you will also see the same results. If you fall into any runtime issues, then do write us for a solution.
If you want more such posts like this “How to Use Chrome for Running Webdriver Test Cases“, then please visit here more often.
All the Best,
TechBeamers.