The DataProvider is your go-to annotation when your tests require inputs from external sources like Excel, CSV, or a database. This tutorial explains the use of TestNG DataProvider in detail. It covers – what is DataProvider in TestNG, its purpose, and its features. Furthermore, we’ll cover what is the easiest as well as the best method methods to use in Java.
Understanding TestNG DataProvider
Let’s begin to understand one of the most important annotations in TestNG, i.e., DataProvider.
What is DataProvider in TestNG?
The DataProvider in TestNG is a built-in annotation for passing input from external data sources to your test cases. This is useful for data-driven testing, where you execute the same test case with different data to verify different scenarios.
Purpose of DataProvider
The purpose of DataProvider is to simplify data-driven testing and make it more efficient. Without DataProvider, you would need to create a separate test case for every different set of data you want to test. This could be time-consuming and error-prone.
Is DataProvider the same in all versions of TestNG?
The DataProvider feature has been available in all versions of TestNG. However, some new features have been added in later versions. For example, in TestNG 7.0, you can now use DataProvider to pass parameters to test methods in other classes.
TestNG, data provider, and Selenium Workflow
Here is a simplified pictorial representation of the flow among TestNG, DataProvider, and Selenium in the context of automated testing.
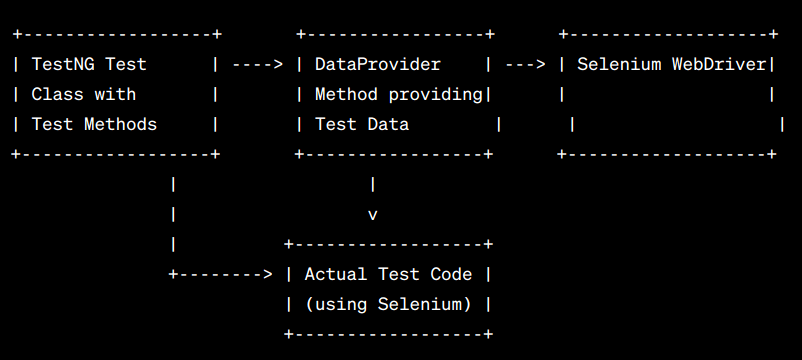
You’ll understand more about this flow in the next sections.
How to use DataProvider in Java?
To use DataProvider in Java, you need to follow these steps:
- Create a method that returns a two-dimensional array of objects. This array will contain the test data.
- Annotate the method with the
@DataProvider
annotation. - Annotate your test method with the
@Test
annotation and specify the name of the DataProvider method in thedataProvider
attribute.
Here is a basic example of a DataProvider method in Java. You should now be able to realize how easy it is to add a data provider in your own code.
@DataProvider
public static Object[][] loginTestData() {
return new Object[][] {
{"username1", "password1"},
{"username2", "password2"},
{"username3", "password3"}
};
}
This DataProvider method returns a two-dimensional array of objects, where each row represents a set of login credentials.
Here is an example of a test method that uses the DataProvider method from above:
@Test(dataProvider = "loginTestData")
public void testLogin(String username, String password) {
// Perform login
// Assert that login is successful
}
When you run this test method, TestNG will execute it three times, once for each set of login credentials in the DataProvider method.
Also Check: How to Add TestNG Assertions in Your Java Code?
How to use DataProvider in Python?
TestNG does not have built-in support for DataProvider in Python. However, there are third-party libraries that we can use for this purpose. One such library is pytest-data-provider
.
To use pytest-data-provider
, you need pip to install its package:
pip install pytest-data-provider
Once installed, you can use pytest-data-provider
to create DataProvider methods and use them in your test cases.
Here is an example of a DataProvider method in Python using pytest-data-provider
:
Python code:
@pytest.mark.parametrize("login_data", [
("username1", "password1"),
("username2", "password2"),
("username3", "password3")
])
def test_login(login_data):
username, password = login_data
# Perform login
# Assert that login is successful
This test method will be executed three times, once for each set of login credentials in the login_data
parameter.
Different things we can do with DataProvider
DataProvider can be used to pass different types of data to your test cases, such as:
- Login credentials
- Product information
- Test data for different scenarios
You can also use DataProvider to pass complex data structures to your test cases, such as JSON objects and XML documents.
Here are some examples of how to use DataProvider in TestNG:
Testing a login form with different sets of credentials:
This Java code illustrates how to log in with a different set of credentials by using the DataProvider in TestNG. This example also shows how you can run the tests with different sets of inputs.
@DataProvider
public static Object[][] loginTestData() {
return new Object[][] {
{"username1", "password1"},
{"username2", "password2"},
{"username3", "password3"}
};
}
@Test(dataProvider = "loginTestData")
public void testLogin(String username, String password) {
// Perform login
// Assert that login is successful
}
This test will execute three times, once for each set of login credentials.
Testing a product page with different products:
In this code, we are simply using the data provider to verify whether the products are displaying with a correct price tag or not. Furthermore, this example demonstrates the case where we may have to provide multiple parameters to our test cases.
@DataProvider
public static Object[][] productTestData() {
return new Object[][] {
{"product1", "100"},
{"product2", "200"},
{"product3", "300"}
};
}
@Test(dataProvider = "productTestData")
public void testProductPage(String product, String price) {
// Navigate to the product page
// Verify that the product name and price are displayed correctly
}
This test will be executed three times, once for each product.
Testing a search functionality with different search queries:
We’ll demonstrate how to use the DataProvider to search 3 different products and verify their listing one after the other.
@DataProvider
public static Object[][] searchTestData() {
return new Object[][] {
{"product1"},
{"product2"},
{"product3"}
};
}
@Test(dataProvider = "searchTextData")
public void testSearch(String query) {
// Enter the search query
// Click on the search button
// Verify that the search results are displayed correctly
}
This test will execute three times, once for each search query.
Check This: 5 Most Asked Selenium Questions You Must Know How to Answer
Unique examples of using DataProvider in TestNG
Here are some unique examples of how to use DataProvider in TestNG:
Testing a file upload functionality with different file types
The below Java code is a placeholder for you to improve further so that you can test whether a file of a certain type was uploaded or not.
@DataProvider
public static Object[][] fileTypeTest() {
return new Object[][] {
{"image.jpg"},
{"document.pdf"},
{"video.mp4"}
};
}
@Test(dataProvider = "fileTypeTest")
public void testFileUpload(String filename) {
// Upload the file
// Verify that the file is uploaded successfully
}
This test will execute three times, once for each file type.
Testing an API with different request payloads
This Java code utilizes TestNG DataProvider to verify different types and sizes of payloads that can pass into an HTTP request.
@DataProvider
public static Object[][] apiPayload() {
return new Object[][] {
{"{\"name\": \"Hello World\"}"},
{"{\"email\": \"hello.world@test.com\"}"},
{"{\"phone\": \"793-111-1313\"}"}
};
}
@Test(dataProvider = "apiPayload")
public void testApi(String payload) {
// Make a POST request to the API with the given payload
// Verify that the response is successful and contains the expected data
}
This test will execute three times, once for each request payload.
Generating dynamic test cases using TestNG
Check another example of DataProvider generating test cases dynamically for specific criteria.
@DataProvider(name = "dynTest")
public Object[][] dynTest() {
// Generate test cases dynamically
return new Object[][]{
{"Input1", "Result1"},
{"Input2", "Result2"}
};
}
@Test(dataProvider = "dynTest")
public void testDynTestCase(String input, String expectedResult) {
// Run the dynamic test cases
}
Testing a login functionality with different sets of credentials
Here is a snapshot of the fully working Java code to showcase how the data provider tackles the most common login use case.
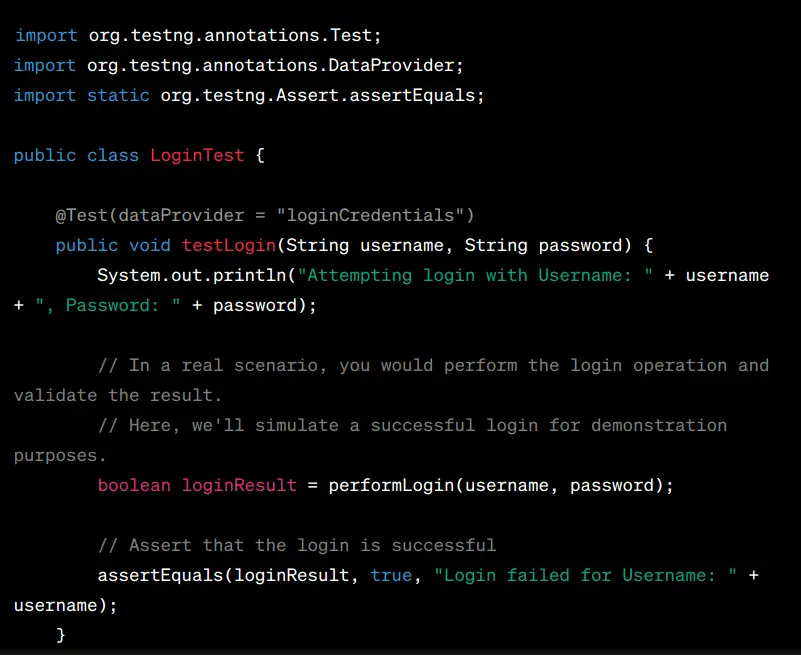
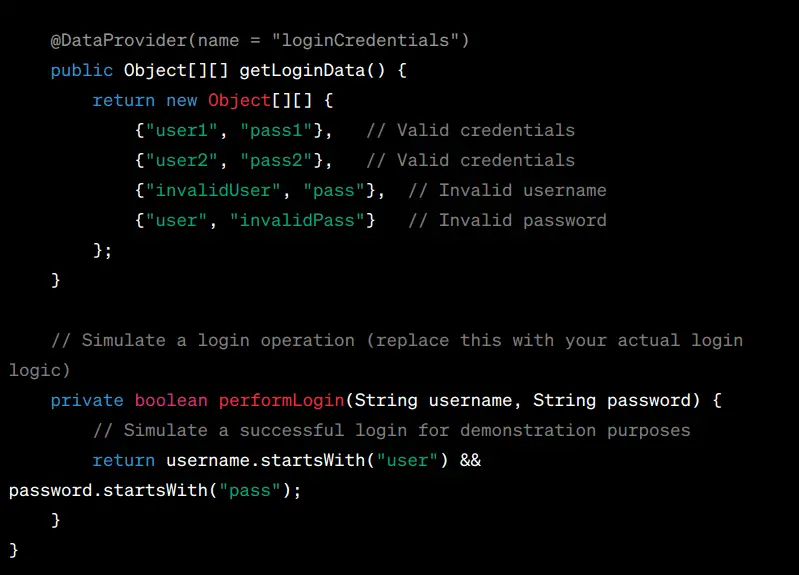
Must Read: 35 Best TestNG Interview Questions to Get Results
We request you go through the above TestNG interview questions. It is because you can see and analyze any gaps from your own TestNG journey from the beginning to the end. You would find 4 crucial sections there fully dedicated to covering every inch of info you need to master this tech.
Summary – How to Use DataProvider in TestNG
We hope today you learned a lot about how to make the most out of the TestNG data provider. By using this unique annotation, you can not only improve your testing experience but also avoid the need to create separate test cases for each set of data that you want to test. This is how you can save time and write more reusable code.
However, if you have even a little query, don’t hesitate to email us. We’ll make sure that it is answered the next day. If you want us to further enrich this tutorial or update some part of it, then also let us know. We like making changes as we learn more from you.
Before you leave, render your support for us to continue. If you like our tutorials, share this post on social media like Facebook/Twitter.
Happy testing,
TechBeamers.