This tutorial describes various ways to convert a Python string to an int and from an integer to a string. You may often need to perform such operations in day-to-day programming. Hence, you should know them to write better programs.
Also, an integer can be represented in different bases, so we’ll explain that too in this post. And there happen to be scenarios where conversion fails. Hence, you should consider such cases as well and can find a full reference given here with examples.
By the way, it will be useful if you have some elementary knowledge of Python data types. If not, please go through the linked tutorial.
Python String to Int and Int to String Conversion
It is essential for a programmer to know the different conversion methods. When you read or receive data from an external source like files, it could be a number but in string format. Sometimes, we use strings to display in a styled way.
Later, when it comes to manipulating or changing the number, you need to convert the string to an integer or in an appropriate type. So, let’s now see how to convert a Python string to int and check all +/-ve scenarios.
1. Using Int() to Convert Python String to Int
Python provides a standard integer class (class ‘int’) to handle numeric operations. It comes with the int() constructor method, which can be used for converting a string to int.
Let’s check out the int() function in action with the help of an example:
""" Python Example: Desc: Use Python int() function to convert string to int """ # Salary per year in string format SalaryPerYear = '1000000' # Test the data type of 'SalaryPerYear' variable print("Data Type of 'SalaryPerYear': {}".format(type(SalaryPerYear))) # Let's perform string to int conversion SalaryPerYear = int(SalaryPerYear) # Again, test the data type of 'SalaryPerYear' variable print("Data Type of 'SalaryPerYear': {}".format(type(SalaryPerYear)))
Below is the result after executing the above code:
Data Type of 'SalaryPerYear': <class 'str'> Data Type of 'SalaryPerYear': <class 'int'>
Convert an Integer from Different Bases
The default base for integer type values is 10. However, in some conditions, the string can contain a number in a different base other than 10.
A base or radix is the number of different digits or a combination of digits and letters that a system of counting uses to represent numbers.
If you wish to know more about the concept of a base, then refer to this – Base in Mathematics.
While converting such a number, you need to specify the correct base in the int() function for a successful conversion. It will assume the following syntax:
# Syntax int(input_str, base_arg)
The allowed range for the base argument is from 2 to 36.
The point to note here is that the result would always be an integer with Base 10. Check out how to concatenate strings in Python and the below code to understand string-to-int conversion from different bases.
""" Python Example: Desc: Use Python int() function to convert string to int from different bases """ # Machine Id in string format MachineIdBase10 = '10010' MachineIdBase8 = '23432' # 10010 => base 8 => 23432 MachineIdBase16 = '271A' # 10010 => base 16 => 271A # Convert machine id from base 10 to 10 MACHINE_ID = int(MachineIdBase10, 10) print("MachineID '{}' conversion from Base 10: {}".format(MachineIdBase10, MACHINE_ID)) # Convert machine id from base 8 (octal) to 10 MACHINE_ID = int(MachineIdBase8, 8) print("MachineID '{}' conversion from Base 8: {}".format(MachineIdBase8, MACHINE_ID)) # Convert machine id from base 16 (hexadecimal) to 10 MACHINE_ID = int(MachineIdBase16, 16) print("MachineID '{}' conversion from Base 16: {}".format(MachineIdBase16, MACHINE_ID))
When you execute the given code, it converts the strings (MACHINEIdBase) holding the same number but in different base formats. And the output value of MACHINE_ID is always in the base 10.

Handling Errors/Exceptions in Conversion
It is also possible to get an error or exception (ValueError) while converting a string to int. It usually happens when the value is not exactly a number.
For example, you are trying to convert a string containing a number formatted using commas. Or it stores a hexadecimal value, but you missed passing the base argument.
So, you may need to handle such errors and take some preventive actions. Try to use a Python try-except block. Please check out the below example to understand.
""" Python Example: Desc: Handle string to int conversion error/exception """ # Salary variable holds a number formatted using commas Salary = '1,000,000' try: print("Test Case: 1\n===========\n") numSalary = int(Salary) except ValueError as ex: print(" Exception: \n ", ex) newSalary = Salary.replace(',', '') print(" Action: ") numSalary = int(newSalary) print(" Salary (Int) after converting from String: {}".format(numSalary)) # MachineId MachineId = 'F4240' # 1,000,000 => base 16 => F4240 try: print("\nTest Case: 2\n===========\n") MACHINE_ID = int(MachineId) except ValueError as ex: print(" Exception: \n ", ex) print(" Action: ") MACHINE_ID = int(MachineId, 16) print(" MACHINE_ID (Int) after converting from String: {}".format(MACHINE_ID))
The example includes two test cases. The first shows an error when the string contains a formatted number. On the other hand, the second fails due to an incorrect base value. After running the code, you get the following result:
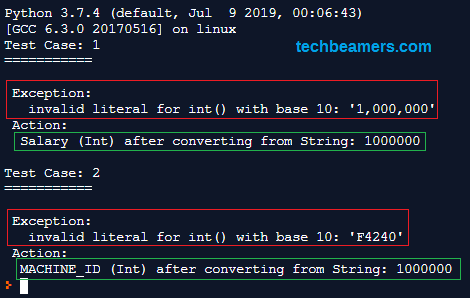
2. Using Str() to Convert Python Int to String
There is another Python standard library function called Str(). It simply takes a number value and converts it to a string.
However, Python 2.7 also had a function called Unicode() that was used to produce Unicode strings. But it isn’t available in Python 3 or later.
Check out the following examples:
""" Python Example: Desc: Use Python str() function to convert int to string """ # Numeric Machine Id in Hexadecimal Format MachineIdBase16 = 0x271A # 0x271A ==> 10010 # Convert numeric Machine Id to Integer MACHINE_ID = str(MachineIdBase16) print("\nMachineID ({}) conversion from Base 16: {}\n".format(hex(MachineIdBase16), MACHINE_ID)) print("MachineIdBase16 type: {} => Post CONVERT => MACHINE_ID type: {}\n".format(type(MachineIdBase16), type(MACHINE_ID)))
Below is the output snippet after executing the above code:

Key Notes
- Typecasting is the process of converting an object from one type to another.
- Python automatically performs the conversion known as Implicit Type Casting.
- Python ensures that no data loss occurs in type conversion.
- If you call functions to convert the types, then the process is called Explicit Type Casting.
- Explicit Casting may lead to loss of data as the user does it by force.