If you enjoy getting through the coding problems, then you must attempt this Python online quiz. It packages 21 coding snippets focussing on Python classes.
With this quiz, you can quickly assess the technical depth of your Python programming ability.
Quiz on Python Classes and Objects
Those who are beginners and learning object-oriented programming should attempt this quiz on Python classes for beginners that we delivered a while ago.
Before diving into the quiz, let’s quickly know how to deal with Python classes. Though, you can use the below TOC to navigate to different sections of the post.
Create a class in Python
Python entitles the class keyword to produce a new class definition. Within a class, you can add member variables and methods. Here are a few facts about the accessibility.
Public
All members of a class are public by default in Python.
class ClipBoard: def __init__(self): self.target = None self.message = None def fill(self, text): self.message = text def clear(self): self.message = None
Now, you have a class ClipBoard. Following operations, you can do with it.
msWord = ClipBoard() msWord.target = "MSWORD" msWord.message = "Hello, World!" msWord.clear() msWord.fill("Any Text")
Protected
Unlike C++/Java, which supports protected members by design, Python allows implementing it by convention. Using underscore (_) as a prefix to any member, you mark it as protected.
Python doesn’t hold you from using a protected member. But it gives a symbolic way of doing that.
class ClipBoard: def __init__(self): self.target = None self._message = None # protected data def fill(self, text): self._message = text def clear(self): self._message = None
Private
You can use the name mangling technique to define a member as private in Python. It changes the way we usually access a class member. It states that if a member name begins with two underscores, then access the member in the following manner.
_<className><memberName>
class ClipBoard: def __init__(self, target): self.target = target self.__message = None # private data def fill(self, text): self.__message = text def clear(self): self.__message = None
To access the private member, you can do the following.
msWord = ClipBoard("MSWORD") msWord._ClipBoard__message = "Any Text"
If you use a private member differently, then Python will throw an error.
Next, Python provides a set of built-in class attributes which you should know.
Built-in Class Attributes
Python inculcates a lot of other features in classes. It does so with the help of a number of built-in attributes.
1. __doc__ – It returns None by default or the doc string if set already.
2. __module__ – It’s the name of the target module containing the class definition.
3. __name__ – Name of the class.
4. __dict__ – Represents the dictionary referencing to the class’s namespace in Python.
5. __bases__ – Mostly, it’s an empty Python tuple holding an ordered list of base classes.
Next, let’s have a look at the concept of inheritance in Python.
Inheritance in Python
You don’t need a keyword to set up an inheritance for a class in Python. See how easy it is to prepare a derived class.
class MyDerivedClass(MyBaseClass): pass
Let’s now extend the ClipBoard class, please see the below example.
class ExtendedClipBoard(ClipBoard): def __init__(self, target, message): ClipBoard.target = target ClipBoard.message = message self.store = None def save(self): self.store = ClipBoard.target + ClipBoard.message def remove(self): self.store = None
Now, use the below instructions to test our derived and base classes.
obj = ExtendedClipBoard("MSOutlook", "Email") obj.save() print(obj.store) obj.remove() print(obj.store)
When you club the above coding snippets and run the code, it’ll return the following output.
Python 2.7.10 (default, Jul 14 2015, 19:46:27) [GCC 4.8.2] on linux MSOutlook Email None
Overriding
Yes, you can override the parent class methods and modify their functionality. Also, you can override operators like +,-, and a list of generic class methods.
Here is a list of generic methods that you can override.
__init__ ( self [,args...] ) Constructor (supports optional arguments). Example : object = className(args) __del__( self ) Destructor (destroys an object). Example : del object __str__( self ) Computes the printable string representation. Example : str(object) __repr__( self ) Computes the "official" string representation. Example : repr(object) __cmp__ ( self, x ) Performs object comparison. Example : cmp(object, result)
We hope the brief intro to Python classes above will help. Now, it’s time to turn on the Python online quiz’s start button.
Python Online Quiz – 21 Questions for Experienced Programmers
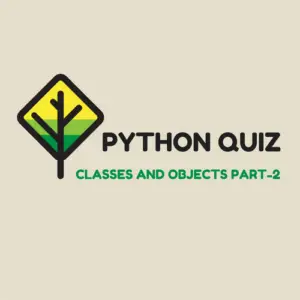
Q-1. What will be the output of the following code?
class Test: def __init__(self, s): self.s = s def print(self): print(s) a = Test("Python Class") a.print()
A. The program gives an error because there is no constructor for class Test.
B. The signature for the print method is incorrect, so an error is thrown.
C. The correct output is “Python Class”.
D. The above code will execute correctly on changing print(s) to print(self.s).
Q-2. What will be the output of the following code?
class Test: def __init__(self, s): self.s = s def print(self): print(self.s) msg = Test() msg.print()
A. The program has an error because the class Test does not have a constructor.
B. The above code produces an error because the definition of print(s) does not include.
C. It executes successfully but prints nothing.
D. The program has an error because the constructor call is made without an argument.
Q-3. What will be the output of the following code?
class Test: def __init__(self, s = "Welcome"): self.s = s def print(self): print(self.s) msg = Test() msg.print()
A. The program has an error because the constructor is not present in the class Test.
B. The above code produces an error because the definition of print(s) does not contain.
C. It executes successfully but prints nothing.
D. The program has an error because the constructor call is made without an argument.
E. The program executes successfully and prints Welcome.
Q-4. What will be the output of the following code snippet?
class Test: def __init__(self): self.x = 1 self.__y = 1 def getY(self): return self.__y val = Test() print(val.x)
A. The program has an error because x is private and cannot be accessed outside of the class.
B. The program has an error because val
is not declared.
C. The program runs fine and prints 1.
D. The program runs fine and prints nothing.
Q-5. What will be the output of the following code snippet?
class Test: def __init__(self): self.x = 1 self.__y = 1 def getY(self): return self.__y val = Test() print(val.__y)
A. The program has an error because y is private and should not be accessed from outside the class.
B. The program has an error because you cannot name a variable using __y.
C. The program runs fine and prints 1.
D. The program runs fine and prints nothing.
Q-6. What will be the output of the following code snippet?
class Test: def __init__(self): self.x = 1 self.__y = 1 def getY(self): return self.__y val = Test() val.x = 45 print(val.x)
A. The program has an error because x is private and should not be accessed from outside the class.
B. The program has an error because you cannot name a variable using __y.
C. The program runs fine and prints 1.
D. The program runs fine and prints 45.
Q-7. Which of the following is a private data field in the given code snippet?
class Test: def __init__(self): __a = 1 self.__b = 1 self.__c__ = 1 __d__ = 1
A. __a
B. __b
C. __c__
D. __d__
Q-8. What will be the output of the following code snippet?
class Test: def __init__(self): self.x = 1 self.__y = 1 def getY(self): return self.__y val= Test() val.__y = 45 print(val.getY())
A. The program has an error because y is private and should not be accessed from outside the class.
B. The program has an error because you cannot name a variable using __y.
C. The code runs fine and prints 1.
D. The code executes successfully and prints 45.
Q-9. What will be the output of the following code snippet?
def main(): myCounter = Counter() num = 0 for i in range(0, 100): increment(myCounter, num) print("myCounter.counter =", myCounter.counter, ", number of times =", num) def increment(c, num): c.counter += 1 num += 1 class Counter: def __init__(self): self.counter = 0 main()
A. counter is 101, number of times is 0
B. counter is 100, number of times is 0
C. counter is 100, number of times is 100
D. counter is 101, number of times is 101
Q-10. What code can we put at the third line of the definition of class B to invoke its superclass’s constructor?
class A: def __init__(self, i = 1): self.i = i class B(A): def __init__(self, j = 2): ___________________ self.j = j def main(): b = B() print(b.i, b.j) main()
A. super().__init__(self)
B. super().__init__()
C. A.__init__()
D. A.__init__(self)
Q-11. What will be the output of the following code snippet?
class A: def __init__(self, x = 1): self.x = x class B(A): def __init__(self, y = 2): super().__init__() self.y = y def main(): b = B() print(b.x, b.y) main()
A. 0 0
B. 0 1
C. 1 2
D. 0 2
E. 2 1
Q-12. What will be the output of the following code snippet?
class A: def __init__(self): self.__x = 1 self.y = 10 def print(self): print(self.__x, self.y) class B(A): def __init__(self): super().__init__() self.__x = 2 self.y = 20 c = B() c.print()
A. 1 10
B. 1 20
C. 2 10
D. 2 20
Q-13. What will be the output of the following code snippet?
class A: def __init__(self, x = 0): self.x = x def func1(self): self.x += 1 class B(A): def __init__(self, y = 0): A.__init__(self, 3) self.y = y def func1(self): self.y += 1 def main(): b = B() b.func1() print(b.x, b.y) main()
A. 2 0
B. 3 1
C. 4 0
D. 3 0
E. 4 1
Q-14. What will be the output of the following code snippet?
class A: def __new__(self): self.__init__(self) print("A's __new__() invoked") def __init__(self): print("A's __init__() invoked") class B(A): def __new__(self): print("B's __new__() invoked") def __init__(self): print("B's __init__() invoked") def main(): b = B() a = A() main()
A. B’s __new__() invoked A’s __init__() invoked
B. B’s __new__() invoked A’s __new__() invoked
C. B’s __new__() invoked A’s __init__() invoked A’s __new__() invoked
D. A’s __init__() invoked A’s __new__() invoked
Q-15. What will be the output of the following code snippet?
class A: def __init__(self, num): self.x = num class B(A): def __init__(self, num): self.y = num obj = B(11) print ("%d %d" % (obj.x, obj.y))
A. None None
B. None 11
C. 11 None
D. 11 11
E. AttributeError: ‘B’ object has no attribute ‘x’
Q-16. What will be the output of the following code snippet?
class A: def __init__(self): self.x = 1 def func(self): self.x = 10 class B(A): def func(self): self.x += 1 return self.x def main(): b = B() print(b.func()) main()
A. 1
B. 2
C. 10
D. x is not accessible from the object of class B.
Q-17. What will be the output of the following code snippet?
class A: def __str__(self): return "A" class B(A): def __str__(self): return "B" class C(B): def __str__(self): return "C" def main(): b = B() a = A() c = C() print(c, b, a) main()
A. A C B
B. A B C
C. C B A
D. B B B
Q-18. What will be the output of the following code snippet?
class A: def __str__(self): return "A" class B(A): def __init__(self): super().__init__() class C(B): def __init__(self): super().__init__() def main(): b = B() a = A() c = C() print(a, b, c) main()
A. B B B
B. A B C
C. C B A
D. A A A
Q-19. What will be the output of the following code snippet?
class A: def __init__(self, x = 2, y = 3): self.x = x self.y = y def __str__(self): return "A" def __eq__(self, num ): return self.x * self.y == num.x * num.y def main(): a = A(1, 2) b = A(2, 1) print(a == b) main()
A. True
B. False
C. 2
D. 1
Q-20. What will be the output of the following code snippet?
class A: def getInfo(self): return "A's getInfo is called" def printInfo(self): print(self.getInfo(), end = ' ') class B(A): def getInfo(self): return "B's getInfo is called" def main(): A().printInfo() B().printInfo() main()
A. A’s getInfo is called A’s getInfo is called
B. A’s getInfo is called B’s getInfo is called
C. B’s getInfo is called A’s getInfo is called
D. B’s getInfo is called B’s getInfo is called
Q-21. What will be the output of the following code snippet?
class A: def __getInfo(self): return "A's getInfo is called" def printInfo(self): print(self.__getInfo(), end = ' ') class B(A): def __getInfo(self): return "B's getInfo is called" def main(): A().printInfo() B().printInfo() main()
A. A’s getInfo is called A’s getInfo is called
B. A’s getInfo is called B’s getInfo is called
C. B’s getInfo is called A’s getInfo is called
D. B’s getInfo is called B’s getInfo is called
Summary – Python Online Quiz for Experienced Programmers
We are hopeful that you’ve liked the above Python online quiz and the idea to bring a brief tutorial of Python classes. You can also go through a number of other Python quizzes on our blog that could be useful for you.
Before leaving, if you like to share a topic of your choice, then please write it in the comment box. We’ll add it to our roadmap and try to deliver a post at the earliest.
Best,
TechBeamers.