C# is undoubtedly one of the most interesting programming languages. And we’ve already covered some of its features in our earlier posts. Today, we are presenting a C# programming test with 15 questions and answers in classes.
There are a few compelling facts that you should know about C#. First of all, the obvious one, it’s an object-oriented language and is strongly typed.
With this strict type-checking feature, the bulk of programming errors including runtime as well as compile time gets uncovered at a very early stage.
It saves programmers from getting into any unforeseen issue later in the project life-cycle.
Test C# Programming Skills
Before you get into a war with the C# programming test, buy a little time to learn about the three most important data types in C#. These are none other than the trio – Object, Var, and Dynamic types. Let’s check out what draws a distinction among them.
Object
It took birth with C# 1.0. It creates a generic entity that can hold any type. A method can accept it as an argument and also return the object type. Without casting to an actual type, you won’t be able to use it. It’s primarily useful when the data type of the target entity is not known.
Var
It’s relatively new and was introduced with C# 3.0. Though, it too can store any value but requires initialization during declaration. It is type-safe and doesn’t make the compiler flag an error at runtime. Neither it supports to pass as a function argument nor can a function return it. The compiler relaxes it to work without being cast. You can utilize it to handle any of the anonymous types.
Dynamic
It’s the newest of the C# construct which got its shape with C# 4.0. It stores values in the same manner as the legacy Var does. It’s not type-safe. And the compiler won’t know anything about the type. There is no need to cast. But you must be aware of the properties and methods the stored type supports. Otherwise, you may get into runtime issues. It is fit for situations where there are requirements to use concepts like Reflection, Dynamic languages, or COM methods.
So now, you know the essentials of three object-oriented data types of C#. Please go ahead to the interview questions/answers section.
C# programming test with 15 questions on classes
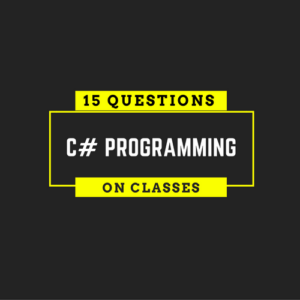
Q-1. Which of the following can be used to define the member of a class externally?
a) :
b) ::
c) #
d) None of the mentioned
Q-2. Which of the following operators can be used to access the member function of a class?
a) :
b) ::
c) .
d) #
Q-3. What will be the output of the following code snippet?
using System; class emp { public string name; public string address; public void display() { Console.WriteLine("{0} is in city {1}", name, address); } } class Program { static void Main(string[] args) { emp obj = new emp(); obj.name = "Akshay"; obj.address = "New Delhi"; obj.display(); Console.ReadLine(); } }
a) Syntax error
b) {0} is in city {1} Akshay New Delhi
c) Akshay is in New Delhi
d) Akshay is in the city of New Delhi
e) Executes successfully and prints nothing
Q-4. Which of the following statements is correct for the given code snippet:
shape obj; obj = new shape();
a) Creates an object of class shape.
b) To create an object of type shape on the heap or stack depending on its size.
c) Create a reference object of the class shape and an object of type shape on the heap.
d) Create an object of type shape on the stack.
Programmers Should Also Follow the Below Post.
Checkout: 15 C# Questions – For, While Loops, and If Else Statements
Q-5. Which of the following gives the correct count of the constructors that a class can define?
a) 1
b) 2
c) Any number
d) None of the above
Q-6. What will be the output of the following code snippet?
using System; class sample { public static void first() { Console.WriteLine("first method"); } public void second() { first(); Console.WriteLine("second method"); } public void second(int i) { Console.WriteLine(i); second(); } } class program { public static void Main() { sample obj = new sample(); sample.first(); obj.second(10); } }
a) second method
10
second method
first method
b) first method
10
first method
second method
c) first method
10
d) second method
10
first method.
Q-7. What will be the output of the following code snippet?
using System; class program { static void Main(string[] args) { int num = 2; fun1 (ref num); Console.WriteLine(num); Console.ReadLine(); } static void fun1(ref int num) { num = num * num * num; } }
a) 8
b) 0
c) 2
d) 16
Q-8. What will be the output of the following code snippet?
using System; class program { static void Main(string[] args) { int[] arr = new int[] {1 ,2 ,3 ,4 ,5 }; fun1(ref arr); Console.ReadLine(); } static void fun1(ref int[] array) { for (int i = 0; i < array.Length; i=i+2) { array[i] = array[i] + 10; } Console.WriteLine(string.Join(",", array)); } }
a) 1 2 3 4 5
b) 11 12 13 14 15
c) 11 2 13 4 15
d) 1 3 5 7 9
Q-9. Which of the following statements correctly tells the differences between ‘=’ and ‘==’ in C#?
a) ‘==’ operator is used to assign values from one variable to another variable
‘=’ operator is used to compare value between two variables
b) ‘=’ operator is used to assign values from one variable to another variable
‘==’ operator is used to compare value between two variables
c) No difference between both operators
d) None of the mentioned
Q-10. What will be the output of the following code snippet?
using System; class program { static void Main(string[] args) { int x = 4 ,b = 2; x -= b/= x * b; Console.WriteLine(x + " " + b); Console.ReadLine(); } }
a) 4 2
b) 0 4
c) 4 0
d) None of the mentioned
Q-11. What will be the output of the following code snippet?
using System; class program { static void Main(string[] args) { int x = 8; int b = 16; int c = 64; x /= c /= b; Console.WriteLine(x + " " + b+ " " +c); Console.ReadLine(); } }
a) 2 16 4
b) 4 8 16
c) 2 4 8
d) 8 16 64
Q-12. What is the correct name of a method that has the same name as that of class and is used to destroy objects?
Note – This method gets called automatically when the application is at the final stage waiting for the process to terminate.
a) Constructor
b) Finalize()
c) Destructor
d) End
Q-13. What will be the output of the following code snippet?
using System; class sample { int i; double k; public sample (int ii, double kk) { i = ii; k = kk; double j = (i) + (k); Console.WriteLine(j); } ~sample() { double j = i - k; Console.WriteLine(j); } } class Program { static void Main(string[] args) { sample s = new sample(9, 2.5); } }
a) 0 0
b) 11.5 0
c) Compile time error
d) 11.5 6.5
Don’t Miss to Check out this Awesome Post.
Checkout: 15 C# Interview Questions that Every Beginner Should Read Once
Q-14. What will be the output of the following code snippet?
using System; class sum { public int value; int num1; int num2; int num3; public sum ( int a, int b, int c) { this.num1 = a; this.num2 = b; this.num3 = c; } ~ sum() { value = this.num1 * this.num2 * this.num3; Console.WriteLine(value); } } class Program { public static void Main(string[] args) { sum s = new sum(4, 5, 9); } }
a) 0
b) 180
c) Compile time error
d) 18
Q-15. What will be the output of the following code snippet?
using System; class Program { static void Main(string[] args) { int num = 5; int square = 0, cube = 0; Mul (num, ref square, ref cube); Console.WriteLine(square + " & " +cube); Console.ReadLine(); } static void Mul (int num, ref int square, ref int cube) { square = num * num; cube = num * num * num; } }
a) 125 & 25
b) 25 & 125
c) Compile time error
d) 10 & 15
Summary – C# programming test with 15 questions on classes
We hope you enjoyed running through the C# programming test. And it might get you a quick brush up on your object-oriented skills.
It would be a pleasure for us to have your feedback on the questions.
Your valuable response, a question, or comments about this post are always welcome. Also, if you liked the above post, then don’t mind following us on our social media accounts.
Check out more CSharp Q&A on various programming topics:
1. 50 CSharp Coding Interview Questions
2. 15 CSharp Programming Interview Questions
3. 15 CSharp Interview Questions Every Programmer
4. 15 CSharp Questions on For, While, and If Else
5. 15 CSharp Programming Questions on Classes
6. 20 CSharp OOPS Interview Questions Set-1
7. 20 CSharp OOPS Interview Questions Set-2
8. 25 CSharp OOPs Interview Questions Set-3
9. 35 CSharp Exception Handling Questions
Best,
TechBeamers