In our previous post, we covered basic C# object-oriented concepts along with a set of 20 questions. Now it’s time to share the second edition of C# object-oriented interview questions.
Before you begin to read the questions, let us tell you two crucial C# object-oriented concepts i.e. Multiple Class Declaration and Partial Classes.
Multiple Class Declaration – When you require multiple classes and need to use them interchangeably, then declare them inside a single namespace. In this way, you won’t have to add it separately to the project. Instead, you place it along with the main class of your program.
Partial classes – There could be a situation when multiple developers are working on a single class. Then, a question arises – how each of them can work with the class without interfering with others’ work? To solve this, C# provides a partial keyword allowing a class to span multiple files.
During compilation, the C# compiler bundles all the partial types into a single assembly. However, a developer has to make sure of the following rules while using the partial keyword.
- All partial types use the same accessibility.
- Each of the partial types should use the “partial” keyword as a prefix.
- If the partial type is sealed, then it must apply to the entire class.
Now you can check out the below C# interview questions.
Advanced C# Interview Questions: Object-Oriented Programming Edition
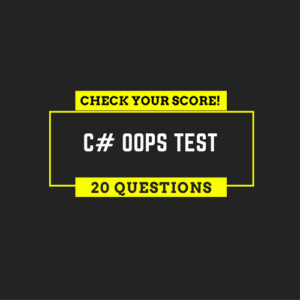
Q-1. Which of the following options defines the correct way of implementing interface data by the class employee?
a) class employee: data {}
b) class employee implements data {}
c) class employee imports data {}
d) None of the mentioned
Q-2. Which of the following correctly defines the implementation of the interface mentioned below?
interface a1 { int func(int i); }
a)
class a { int func(int i) as a1.fun { } }
b)
class a: implements a1 { int fun(int i) { } }
c)
class a: a1 { int a1.fun(int i) { } }
d) None of the mentioned
Q-3. Which of the following Access specifiers can be used for an interface?
a) Public
b) Protected
c) Private
d) All of the above
Q-4. Which of the following statements is correct for the below code snippet?
interface A { void func1(); int func2(); } class B : A { void func1() { } int A.func2() { } }
a) class B is an abstract class.
b) A method table would not be created for class B.
c) The definition of func1() in class B should be void A.func1().
d) All of the above.
Q-5. Which of the following statements is correct for the below code snippet?
interface A { String FirstName { get; set; } String LastName { get; set; } void print(); void assign(); int func(); }
a) interface A to contain function definition.
b) properties cannot be declared inside an interface.
c) code compiles successfully.
d) All of the above.
Q-6. What will be the output of the following code snippet?
interface A { void func(int i); } class B1 : A { public int x; public void func(int i) { x = i * i; } } class B2 : A { public int x; public void func(int i) { x = i / i; } } class Program { public static void Main(string[] args) { B1 b1 = new B1(); B2 b2 = new B2(); b1.x = 0; b2.x = 0; b1.func(2); b2.func(2); Console.WriteLine(b1.x + " " + b2.x); Console.ReadLine(); } }
a) 0 0
b) 4 1
c) 2 2
d) 1 4
Q-7. What will be the output of the following code snippet?
using System; interface A1 { void func(); } interface A2 { void func(); } public class B :A1, A2 { void A1.func() { Console.WriteLine("inside function definition for A1"); } void A2.func() { Console.WriteLine("inside function definition for A2"); } } class Program { static void Main(string[] args) { B b = new B(); A1 a1 = (A1) b; a1.func(); A2 a2 = (A2) b; a2.func(); } }
a) inside function definition for A1
inside function definition for A2
b) inside function definition for A1
c) inside function definition for A2
d) inside function definition for A2
inside function definition for A1
Q-8. Which of the following code snippet defines the correct way of representing an interface derived from two interfaces with a method with the same name and signature?
a)
interface A { void func(); } interface B { void func(); } interface C : A, B { } class sample :C { void A.func() { } void A.func() { } }
b)
interface A { void func(); } interface B { void func(); } interface C :A, B { } class sample :C { void A.B.func() { } }
c)
interface A { void func(); } interface B { void func(); } interface C : A, B { } class sample :C { void A.func() { } void B.func() { } }
d) None of the above
Q-9. Which of the following keywords can be used to access a member of the base class from the derived class?
a) upper
b) base
c) this
d) None of the above
Q-10. Which of the following statements are correct about the given code snippet?
using System; class baseSample { protected int num; public baseSample() { num = 0; } } class derivedSample: baseSample { public void sum() { num += 1; } } class Program { static void Main(string[] args) { derivedSample z = new derivedSample(); z.sum(); } }
a) ‘num’ variable should be declared as public if it is needed to be available in the inheritance chain.
b) ‘num’ should be declared as protected if it is to become available in the inheritance chain.
c) The constructor of the baseSample class does not get inherited in the derivedSample class.
d) While constructing an object referred to by z, firstly, the constructor of the baseSample class will be called followed by the constructor of the derivedSample class.
Q-11. What will be the output of the following code snippet?
using System; class baseSample { int i = 10; int j = 20; public void print() { Console.WriteLine("inside base method "); } } class derivedSample : baseSample { public int s = 30; } class Program { static void Main(string[] args) { derivedSample d = new derivedSample(); Console.WriteLine("{0}, {1}, {2}", d.i, d.j, d.s); d.print(); Console.ReadLine(); } }
a) 10, 20, 30
inside base method
b) 10, 20, 0
c) inside the base method
d) compile time error
`baseSample.i’ and `baseSample.j’ is inaccessible due to its protection level.
Q-12. What will be the size of the object created in the given code snippet?
using System; class baseSample { private int a; protected int b; public int c; } class derivedSample : baseSample { private int x; protected int y; public int z; } class Program { static Void Main(string[] args) { derivedSample d = new derivedSample(); } }
a) 20 bytes
b) 12 bytes
c) 16 bytes
d) 24 bytes
Q-13. What will be of the following code snippet?
using System; class baseSample { public baseSample() { Console.WriteLine("THIS IS BASE CLASS constructor"); } } public class derivedSample : baseSample { } class Program { static void Main(string[] args) { derivedSample d = new derivedSample(); Console.ReadLine(); } }
a) Code executes successfully and prints nothing
b) This is the base class constructor
c) Compile time error
d) None of the above
Inconsistent accessibility: base class `baseSample’ is less accessible than class `derivedSample’
Q-14. Which of the following statements should be included in the current code set to get the output as 10 20?
using System; public class baseSample { protected int a = 20; } public class derivedSample : baseSample { int a = 10; public void display() { /* add code here */ } } class Program { static void Main(string[] args) { derivedSample d = new derivedSample(); d.display(); Console.ReadLine(); } }
a) Console.WriteLine(a + ” ” + base.a);
b) Console.writeline( a + ” ” + this.a);
c) Console.Writeline( mybase.a + ” ” + a);
d) console.writeline(base.a + ” “+ a);
Q-15. What will be the output of the following code snippet?
using System; class baseSample { int a; public baseSample(int b) { a = b; Console.WriteLine(" a "); } class derivedSample : baseSample { public derivedSample (int b) : base(b) { Console.WriteLine(" b "); } } class program { public static void Main(string[] args) { derivedSample d = new derivedSample(20); } } }
a) Compile time error
b) a
b
c) b
a
d) 20
20
Q-16. Which of the following statements should be included in the given code set to get the output as “Welcome to TechBeamers”?
using System; class baseSample { public void print() { Console.WriteLine("Welcome to"); } } class derivedSample : baseSample { public void print() { /* add statement here */ Console.WriteLine("TechBeamers"); } } class program { static void Main(string[] args) { derivedSample d = new derivedSample(); d.print(); } }
a) base.print();
b) baseSample.print();
c) print();
d) baseSample::print();
Q-17. What will be the output of the following code snippet?
using System; class baseSample { public int i; protected int j; } class derivedSample : baseSample { public int j; public void print() { base.j = 3; Console.WriteLine(i + " " + j); } } class Program { static void Main(string[] args) { derivedSample d = new derivedSample(); d.i = 1; d.j = 2; d.print(); Console.ReadLine(); } }
a) 2 1
b) 1 3
c) 3 1
d) 1 2
Q-18. What will be the output of the following code snippet?
using System; class baseSample { public int i; public int j; } class derivedSample : baseSample { public void print() { int j = 3; base.i = base.j + 1; Console.WriteLine(base.i + " " + base.j); } } class Program { static void Main(string[] args) { derivedSample d = new derivedSample(); d.i = 1; d.j = 2; d.print(); Console.ReadLine(); } }
a) 1 2
b) 1 3
c) 3 2
d) 3 3
Q-19. Which of the following statements is correct for the base and derived classes?
a) If a base class consists of a member function fun() and a derived class do not have any function with this name. An object of the derived class can access the base class fun() directly.
b) Class D can be derived from class C, which in turn gets derived from class B which is derived from class A.
c) If a base and a derived class, both include a member function with the same name. The member function of the derived class can be called by an object of the derived class.
d) The size of a derived class object is equal to the sum of the sizes of data members in the base and derived class.
Q-20. What will be the output of the following code snippet?
using System; class Baseclass { public void fun() { Console.Write("Base class" + " "); } } class Derived1: Baseclass { new void fun() { Console.Write("Derived1 class" + " "); } } class Derived2: Derived1 { new void fun() { Console.Write("Derived2 class" + " "); } } class Program { public static void Main(string[ ] args) { Derived2 d = new Derived2(); d.fun(); } }
a) Base class
b) Derived1 class
c) Derived2 class
d) Base class Derived1 class
e) Base class Derived1 class Derived2 class
Wrapping Up – Object-Oriented Programming with C# Interview Questions
We think these questions are very helpful for people who are trying to get a job on C#/.NET. And we hope you’ll be able to get the most out of them.
However, if you’ll look up further on our blog, then you’ll see hundreds of other C# interview questions. Go through as many of them as you could and secure your seat in a top IT MNC.
Check out more CSharp Q&A on various programming topics:
1. 50 CSharp Coding Interview Questions
2. 15 CSharp Programming Interview Questions
3. 15 CSharp Interview Questions Every Programmer
4. 15 CSharp Questions on For, While, and If Else
5. 15 CSharp Programming Questions on Classes
6. 20 CSharp OOPS Interview Questions Set-1
7. 20 CSharp OOPS Interview Questions Set-2
8. 25 CSharp OOPs Interview Questions Set-3
9. 35 CSharp Exception Handling Questions
Keep Learning,
TechBeamers