Check out 15 C# programming questions every beginner should prepare before appearing in an interview for the C# developer position. It could help you clear the first round which is a written test held to assess your programming ability.
So if you are going for such interviews with top IT MNCs like Amazon, Flipkart, Oracle, Siemens, and Ericsson, then you should aim for solving these kinds of questions.
In this post, we’ve targeted a specific set of quick coding problems that can make you brush up your C# skills.
We hope to see you getting success in your forthcoming interviews. In case you have any queries, then please use the comment box to let us know.
Solve Quick C# Coding Problems
Apart from the C# programming questions, you can also read the below post to get through the second round of the interview. At this level, the interviewer puts up theoretical questions and expects a decisive answer from you.
Must Read: 15 C# Interview Questions for Beginners
Top 15 C# Programming Questions for Freshers
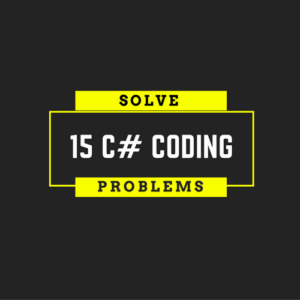
Q-1. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { int i = 0, j = 0; label: i++; j+=i; if (i < 10) { Console.Write(i +" "); goto label; } } } }
Output:
A. Prints 1 to 9
B. Prints 0 to 8
C. Prints 2 to 8
D. Prints 2 to 9
E. Compile error at label
Q-2. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { int i = 20 ; for( ; ; ) { Console.Write(i + " "); if (i >= -10) i -= 4; else break; } } } }
Output:
A. 20 16 12 84 0 -4 -8
B. 20 16 12 8 4 0
C. 20 16 12 8 4 0 -4 -8 -12
D. 16 12 8 4 0
E. 16 8 0 -8
Q-3. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { public enum day { Sun, Mon, Tue, Wed, Thur, Fri, Sat }; class SelectWeekDay { static void Main (string[ ] args) { day obj = day.Wed; switch (obj) { case day.Sun: Console.WriteLine(day.Sun); break; case day.Mon: Console.WriteLine(day.Mon); break; case day.Tue: Console.WriteLine(day.Tue); break; case day.Wed: Console.WriteLine(day.Wed); break; case day.Thur: Console.WriteLine(day.Thur); break; case day.Fri: Console.WriteLine(day.Fri); break; case day.Sat: Console.WriteLine(day.Sat); break; } } } }
Output:
A. Mon
B. Tue
C. Wed
D. 2
E. 3
Q-4. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { int num; for (num = -5; num <= 5; num++) { switch (num) { case 0: Console.Write ("Tech Beamers"); break; } if (num > 0) Console.Write ("+"); else if (num < 0) Console.Write ("*"); } } } }
Output:
A. +++++Tech Beamers
B. *****Tech Beamers+++++
C. Tech Beamers*****
D. +++++Tech Beamers*****
E. +++++*****
Q-5. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { char ch = Convert.ToChar ('c' | 'a' | 'b'); switch (ch) { case 'A': case 'a': Console.WriteLine ("case A | case a"); break; case 'B': case 'b': Console.WriteLine ("case B | case b"); break; case 'C': case 'c': case 'D': case 'd': Console.WriteLine ("case D | case d"); break; } } } }
Output:
A. case A | case a
B. case B | case b
C. case D | case d
D. Compile Error
Q-6. Which of the following code snippets correctly differentiate between Odd and Even number?
A.
int num; String out; if (num % 2 == 0) out = "Even"; else out = "Odd";
B.
int num; String out; if (num Mod 2 == 0) out = "Even"; else out = "Odd";
C.
int num; Console.WriteLine(num Mod 2 == 0 ? "Even": "Odd");
D.
int num; String out; num % 2 == 0 ? out = "Even" : out = "Odd"; Console.WriteLine(out);
Output:
A. 1, 3
B. 1 Only
C. 2, 3
D. 4 Only
E. All are correct
Q-7. Which of the following statements can be used to terminate a while loop and transfer control outside the loop?
1. exit while
2. continue
3. exit statement
4. break
5. goto
Output:
A. 1, 3
B. 2, 4
C. 3, 5
D. 4, 5
E. All of the above
Q-8. Which of the following statements is correct for the below code snippet?
if (age > 18 && no < 11) a = 25;
- The condition “no < 11” will be evaluated only if age > 18 evaluates to True.
- The statement “a = 25” will get executed if any one condition is True.
- The condition “no < 11” will be evaluated only if age > 18 evaluates to False.
- The statement “a = 25” will get executed if both the conditions are True.
- && is known as a short-circuiting logical operator.
Output:
A. 1, 3
B. 2, 5
C. 1, 4, 5
D. 3, 4, 5
E. All options are correct
Q-9. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { int a = 2, b = a; if (Convert.ToBoolean((a | b & 5) & (b - 25 * 1))) Console.WriteLine(1); else Console.WriteLine(0); } } }
Output:
A. 0
B. 1
C. Compile Error
D. Runtime Error
Q-10. Which of the following statements is correct for the given code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { int id; String val; switch (id) { case 5: val = "First"; break; case 6: val = "Second"; break; case 1: val = "Third"; break; case ls <4: val = "Fourth"; break ; case Else: val = "Fifth"; break; } } } }
Output:
A. The compiler will report an error in case “ls<4” as well as in case “Else”.
B. There is no error in the code.
C. The compiler will report an error in case “Else”.
D. The compiler will report an error as there is no default case.
E. The order of the first three cases should be cases 1, 5, and 6 (ascending order).
Q-11. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { int[] a = new int[3]; a[1] = 10; Object o = a; int[] b = (int[])o; b[1] = 100; Console.WriteLine(a[1]); ((int[])o)[1] = 1000; Console.WriteLine(a[1]); } } }
Output:
A. 1000
B. 100
C. 100
1000
D. Error
Q-12. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { String a = "TechBeamers"; String b = "TECHBEAMERS"; int val; val = b.CompareTo(a); Console.WriteLine(val); } } }
Output:
A. -1
B. 1
C. 0
D. Error
Q-13. What will be the output of the following code snippet?
using System; namespace ProgrammingExercise { class FindOutput { static void Main(string[] args) { char Obj = 'L'; char Num = Convert.ToChar(76); Obj--; Num++; Console.WriteLine( Obj+ " " + Num); Console.ReadLine(); } } }
Output:
A. K M
B. L M
C. K L
D. L L
Q-14. Which of the following statements is correct?
1. The switch statement is a control statement that handles multiple selections and enumerations by passing control to one of the case statements within its body.
2. The goto statement passes control to the next iteration of the enclosing loop statements in which it appears.
3. We induce Branching using jump statements which cause an immediate transfer of the program control.
4. We use the continue statement to transfer the program control, either to the specified switch-case label or to its default case.
5. The do statement executes a statement or a block of statements enclosed in “{}” repeatedly until the defined expression evaluates to false.
A. 1, 2, 4
B. 1, 3, 5
C. 2, 3, 4
D. 3, 4, 5
E. None of these
Q-15. Which of the following statements are correct about the given code snippet?
if (obj > 18 || num < 11) res = 25;
1. The condition num < 11 will get evaluated only if obj > 18 evaluates to False.
2. The condition num < 11 will get evaluated if obj > 18 evaluates to True.
3. The statement res = 25 will be evaluated if any one of the two conditions is True.
4. || is known as a short-circuiting logical operator.
5. The statement res = 25 will be evaluated only if both the conditions are True.
A. 1, 4, 5
B. 2, 4
C. 1, 3, 4
D. 2, 3, 5
E. None of these
Summary – C# Programming Questions for Beginners
Hopefully, you would have now got good exposure to the kinds of C# programming questions asked in interviews.
In our next post, we’ll come up with a new topic that will help you learn more about C# programming.
If you liked the above questions, then do share this post on your social media accounts.
Check out more CSharp Q&A on various programming topics:
1. 50 CSharp Coding Interview Questions
2. 15 CSharp Programming Interview Questions
3. 15 CSharp Interview Questions Every Programmer
4. 15 CSharp Questions on For, While, and If Else
5. 15 CSharp Programming Questions on Classes
6. 20 CSharp OOPS Interview Questions Set-1
7. 20 CSharp OOPS Interview Questions Set-2
8. 25 CSharp OOPs Interview Questions Set-3
9. 35 CSharp Exception Handling Questions
All the Best,
TechBeamers