Exceptions are runtime errors that can make a program behave abruptly if not handled in the right way. Hence, most programming languages add exception-handling capabilities. C# too has a robust object-oriented exception-handling framework. And a good C# programmer should know how to use C# exception handling techniques to ensure a good user experience. Interviewers often ask questions on exception handling during interviews.
Here we are laying down 35 C# interview questions on exception handling for C# programmers to identify rough edges. A programmer must be careful while handling exceptions in the application code. It could be a background service where you need to log errors in a file or an interactive user application expecting a proper error message. There might even be cases when you have to catch and rethrow an exception. For example, rethrowing an exception could be useful in rolling back a database transaction.
C# Exception Handling – Quick Review
Following are some of the key facts that you should know about exception handling in C#.
- In C# language, Exception (<System.Exception>) is the base class of all exceptions. And it itself is derived from the Object (<System.Object>) class.
- Other exception classes like <System.ApplicationException> and <System.SystemException> derive directly or indirectly from the Exception class.
- You can also create a user-defined or a custom exception in C#. With custom exceptions, you can decode a complex error into a meaningful message.
- Exception handling in C# mainly revolves around the four keywords.
// Try block try { // Program instructions Block. } // Catch block catch(ExceptionType e) { // Instructions to handle exception. } // Finally block finally { // Instructions to clean up. } // Throw keyword catch (Exception ex) { throw (ex); }
- A try block can cause more than one exception. By having a catch block without brackets, you can handle them all within the same catch block.
- To rethrow an exception, you can use the <throw> keyword.
- User-defined catch block must appear before any generic catch block. Otherwise, the runtime will ignore it from execution.
- In the absence of an exception handler, the program will abort with an error message.
If you are a real C# coding buff, then you may like to try the C# interview questions with 50 coding snippets we recently added.
Let’s now explore the C# Exception Handling Q&A section.
C# Exception Handling Questions for Programmers
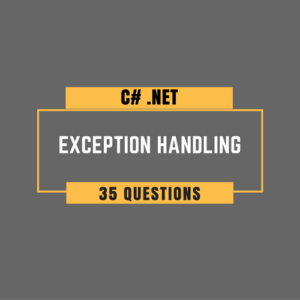
Q-1. Which of the following statements applies to the situation where Exception is not handled in the program:
a) The Compiler will not allow the program to run the code.
b) CLR will terminate the program execution at the point where it encounters an exception.
c) CLR will not show any output. However, the code will execute successfully.
d) The Code executes successfully, and an error message gets printed.
Q-2. Which of the following statements correctly defines the usage of <try-catch> blocks in exception handling:
a) Error-prone code is written inside the try block, and the exception handling code is written inside the catch block.
b) Error-prone code is written inside the try block, and the error-free code is written inside the catch block.
c) Exception handling code is written inside the try block and error code inside the catch block.
d) None of the above.
Q-3. Which of the following is NOT a valid DOT NET Exception class:
a) System.Exception
b) System.DivideByZeroException
c) System.OutOfMemoryException
d) System.InvalidOperationException
e) System.StackMemoryException
Q-4. Which of the following statements correctly defines the usage of the <finally> block in exception handling:
a) Code under the <finally> block gets executed only when try and catch block get executed.
b) Code under the <finally> block gets executed only if the catch block is not executed.
c) Code under the <finally> block is always executed irrespective of the <try-catch> block is executed or not.
d) Code under the <finally> block gets executed only if the catch block is executed.
Q-5. Which of the following statements correctly defines the purpose of return statements in a <try-catch> and <finally> blocks:
a) The return statement can be used in all to return data.
b) The return statement cannot be used in Catch and finally blocks.
c) The usage of a return statement is not supported in the <finally> block.
d) The return statement cannot be used in the catch block.
Q-6. Which of the following gives the number of catch blocks that can be defined for a single try block:
a) One
b) Two
c) Many
d) The try block can have any number of catch blocks. Just the most specific exception should precede the most generic one, i.e. all child catch blocks are to be defined first followed by the parent catch block.
Q-7. Which of the following keyword can be used to rethrow an exception in C#:
a) return
b) throw
c) re-throw
d) create
Q-8. Which of the following statements correctly justifies the exception given below:
Unhandled Exception: System.lndexOutOfRangeException: Index was outside the bounds of the array: at TechBeamers.MyProgram.Assign(Int32 index, Int32 val) in D:\Sample\ TechBeamers \MyProgram.cs:line 30 at TechBeamers.MyProgram.Main(String[] args) in D:\Sample\ TechBeamers \MyProgram.cs:line 25
a) The program did not handle an exception called IndexOutOfRangeException.
b) The class MyProgram belongs to the namespace TechBeamers.
c) The function Assign() was called from Main() in line number 25.
d) The exception occurred in line number 30 in the function Assign().
e) All of the above
Q-9. What will be the output of the following code snippet:
using System; class MyProgram { static void Main(string[] args) { int index = 6; int[] arr = new int[5]; try { arr[index] = 100; } catch(IndexOutOfRangeException e) { Console.WriteLine("Index out of bounds occurred"); } Console.WriteLine("Program execution continued after Exception Handling"); } }
a) Runtime error occurs
b) assigns 100 to arr[6]
c) Outputs: Index out of bounds occurred
d) Outputs: Program execution continued after Exception Handling
e) Outputs: Index out of bounds occurred
Program execution continued after Exception Handling
Q-10. What will be the output of the following code snippet, if the value entered for the index is a string like “ab”:
using System; class MyProgram { static void Main(string[] args) { int index; int value = 100; int[] arr = new int[10]; try { Console.Write("Enter a number: "); index = Convert.ToInt32(Console.ReadLine()); arr[index] = value; } catch(FormatException e) { Console.Write("Bad Format "); } catch(IndexOutOfRangeException e) { Console.Write("Index out of bounds "); } Console.Write("Remaining program "); } }
a) output: Bad Format
b) output: Remaining program
c) output: Index out of bounds
d) output: Bad Format Remaining program
e) output: Index out of bounds Remaining program
Q-11. What will be the result of the following code snippet, if the value entered for the index is 9:
using System; class MyProgram { static void Main(string[] args) { int index; int value = 100; int[] arr = new int[10]; try { Console.Write("Enter a number: "); index = Convert.ToInt32(Console.ReadLine()); arr[index] = value; } catch(Exception e) { Console.Write("Exception occurred"); } Console.Write("Remaining program "); } }
a) Runtime exception is thrown
b) output: Exception occurred
Remaining program
c) output: Remaining program
d) output: Index out of bounds
Remaining program
e) The value 100 will get assigned to <a[9]>
Q-12. What will be the output of the following code snippet:
using System; class MyProgram { static void Main(string[] args) { try { Console.WriteLine("Exception:" + " " + 1/Convert.ToInt32(0)); } catch(ArithmeticException e) { Console.WriteLine("Divide by Zero error"); } Console.ReadLine(); } }
a) Exception: Divide by Zero error
b) Divide by Zero error
c) Exception: 0
d) Runtime error
Q-13. Which of the following statements are correct about the exception handling <try-catch> blocks:
a) The try blocks cannot be nested.
b) In one function, there can be only one try block.
c) An exception must be caught in the same function in which it is thrown.
d) All values set up in the exception object are available in the catch block.
e) While throwing a user-defined exception multiple values can be set in the exception object.
Q-14. What will be the output of the following code snippet:
using System; class MyProgram { static void Main(string[] args) { try { Console.WriteLine("Exception:" + " " + 1/Convert.ToInt32(0)); } finally { Console.WriteLine("Divide by Zero error"); } Console.ReadLine(); } }
a) Runtime Error
b) Unhandled Exception
c) Exception: DivideByZeroException
d) Divide by Zero error
Q-15. What will be the output of the following code snippet:
using System; class MyProgram { static void Main(string[] args) { int val = 10; try { int i; for (i = -1; i < 3; ++i) val = (val / i); } catch (ArithmeticException e) { Console.WriteLine("0"); } Console.WriteLine(val); Console.ReadLine(); } }
a) 0
b) 0 10
c) 0 -10
d) 5
Q-16. What will be the output of the following code snippet:
using System; class MyProgram { static void Main(string[] args) { try { int []arr = {1, 2, 3, 4, 5}; for (int i = 0; i < 5; ++i) Console.WriteLine(arr[i]); int val = (1 / Convert.ToInt32(0)); } catch(IndexOutOfRangeException e) { Console.WriteLine("A"); } catch(ArithmeticException e) { Console.WriteLine("B"); } Console.ReadLine(); } }
a) 1234
b) Runtime Error
c) 12345A
d) 12345B
Q-17. What is the correct order of the catch blocks to allow the code to execute successfully:
try { int num = 90/n; } catch (Exception e) { { catch (ArithmeticException e) { } catch (DivideByZeroException e) { }
a) First catch block to be moved to the end.
b) First catch block to be moved to the end and the last catch block to be moved to the first place.
c) Last catch block to be moved to first place.
d) None of the above.
Q-18. What will be the output of the following code snippet:
using System; class MyProgram { static void Main(string[] args) { try { int []arr = {1, 2, 3, 4, 5}; for (int i = 0; i < 6; ++i) Console.WriteLine(arr[i]); } catch(IndexOutOfRangeException e) { Console.WriteLine("A"); } Console.ReadLine(); } }
a) 12345
b) 12345A
c) 12345AA
d) Run time error
Q-19. What will be the output of the following code snippet:
using System; class MyProgram { static void Main(string[] args) { try { int x, y; y = 0; x = 5 / y; Console.WriteLine("A"); } catch(ArithmeticException e) { Console.WriteLine("B"); } finally { Console.WriteLine("C"); } Console.ReadLine(); } }
a) Runtime error
b) ABC
c) B
d) BC
Q-20. What will be the output of the following code snippet:
using System; class MyProgram { public static void Main(string[] args) { int ret= Cal(10,0); Console.WriteLine(ret); } public static int Cal(int x, int y) { try { return(x/y); } catch(Exception e) { return 0; } Console.WriteLine("Exception Handled "); } }
a) Runtime error
b) Compile time error
c) 0
d) Exception Handled
Q-21. What will be the output of the following code snippet:
using System; class MyProgram { public static void Main(string[] args) { try { int x = args.Length; int y = 1 / x; Console.WriteLine("Hello"); } catch (ArithmeticException e) { Console.WriteLine("Exception Occurred"); } Console.ReadLine(); } }
a) Runtime error
b) Hello
c) Exception Occurred
d) Exception Occurred
Hello
Q-22. What will be the output of the following code snippet:
using System; class MyProgram { public static void Main(string[] args) { try { throw new NullReferenceException("C"); Console.WriteLine("Exception Occurred"); } catch (ArithmeticException e) { Console.WriteLine("Exception Caught"); } Console.ReadLine(); } }
a) Exception Occurred
b) Exception Caught
c) Runtime Error
d) Compile time Error
Q-23. What will be the output of the following code snippet:
using System; class MyProgram { public static void Main(string[] args) { try { int rand = 0; if (rand == 0) { throw new Exception ("Random = 0"); rand = 1; } if (rand ==1) { Console.WriteLine("Random = 1"); return; } Console.WriteLine("Random = 2"); } finally { Console.WriteLine("Flow reaches Finally"); } } }
a) Unhandled Exception: System.Exception:Random = 0 at MyProgram.Main()
Flow reaches Finally
b) Flow reaches Finally
c) Random = 0
Random = 1
Flow reaches Finally
d) Random = 0
Random = 1
Random = 2
Flow reaches Finally
Q-24. What will be the output of the following code snippet:
using System; class MyProgram { public static void Main(string[] args) { try { int num = 1; int value = 100; try { if (num == 1) num = num / num - num; if (num == 2) { int[] arr = { 1 }; arr[1] = value; } } finally { Console.WriteLine("Finally Block"); } } catch (IndexOutOfRangeException e) { Console.WriteLine("Exception Caught"); } Console.ReadLine(); } }
a) Finally Block
b) Exception Caught
c) Exception Caught
Finally Block
d) Runtime Error
Q-25. What will be the output of the following code snippet:
using System; class MyProgram { public static void Main(string[] args) { try { int num = args.Length; int val = 10 / num; Console.WriteLine(num); try { if (num == 1) num = num / num - num; if (num == 2) { int[] arr = { 1 }; arr[1] = 100; } } catch (IndexOutOfRangeException e) { Console.WriteLine("Index Out Of Range Exception"); } } catch (ArithmeticException e) { Console.WriteLine("Arithmetic Exception"); } Console.ReadLine(); } }
a) Runtime Error
b) Index Out Of Range Exception
c) Arithmetic Exception
d) Code compiles successfully, and nothing gets printed
Q-26. What will be the output of the following code snippet:
using System; class MyException : ApplicationException { public MyException(String Msg):base(Msg) { } } class MyClass { public static void Print() { throw new MyException("This is My Exception"); } } class MyProgram { static void Main(string[] args) { try { MyClass.Print(); } catch (MyException ex) { Console.WriteLine(ex.Message); } Console.ReadLine(); } }
a) Runtime error
b) Compile time error
c) This is My Exception
d) Code compiles successfully, and nothing gets printed
Q-27. Which of the following keywords does the calling function use to guard against the exception thrown by the called function:
a) try
b) throw
c) throws
d) catch
Q-28. What will be the output of the following code snippet:
using System;using System; public class InvalidAgeException : Exception { public InvalidAgeException(String message) : base(message) { } } public class MyProgram { static void verifyAge(int age) { if (age < 18) { throw new InvalidAgeException("Sorry, Age must be greater than 18"); } } public static void Main(string[] args) { try { verifyAge(10); } catch (InvalidAgeException e) { Console.WriteLine(e); } Console.WriteLine("Rest of the Code"); } }
a) Runtime error
b) Compile time error
c) Rest of the Code
d)
InvalidAgeException: Sorry, Age must be greater than 18 at MyProgram.verifyAge (Int32 age) [0x00000] in <filename unknown>:0 at MyProgram.Main (System.String[] args) [0x00000] in <filename unknown>:0 Rest of the Code
Q-29. What will be the output of the following code snippet:
using System; class MyProgram { public static void Main(string[] args) { try { int num = 1; int value = 100; try { if (num == 1) num = num / (num - num); if (num == 2) { int[] arr = { 1 }; arr[1] = value; } } catch ( IndexOutOfRangeException e) { Console.WriteLine("Inner Catch"); } } catch (ArithmeticException e) { Console.WriteLine("Outer Catch"); } Console.ReadLine(); } }
a) Inner Catch
b) Outer Catch
c) Runtime Error
d) Compile time Error
Q-30. What will be the output of the following code snippet:
using System; class MyProgram { static void Main() { try { A(); } catch (Exception ex) { Console.WriteLine(ex); } } static void A() { // Rethrow syntax. try { int value = 1 / int.Parse("0"); } catch { throw; } } }
a) Runtime Error
b) Compile time Error
c)
System.DivideByZeroException: Division by zero at MyProgram.A () [0x00000] in <filename unknown>:0
d) Program compiles successfully, and nothing is printed.
Q-31. What will be the output of the following code snippet:
using System; class MyProgram { static void Main() { try { B(); } catch (Exception ex) { Console.WriteLine(ex); } } static void B() { // Filtering exception types. try { int value = 1 / int.Parse("0"); } catch (DivideByZeroException ex) { throw ex; } } }
a) Runtime Error
b) Compile time Error
c)
System.DivideByZeroException: Division by zero at MyProgram.B () [0x00000] in <filename unknown>:0
d) The program compiles successfully, and nothing is printed.
Q-32. What will be the output of the following code snippet:
using System; class MyProgram { static void Main() { try { C(null); } catch (Exception ex) { Console.WriteLine(ex); } } static void C(string value) { // Generate new exception. if (value == null) { throw new ArgumentNullException("value"); } } }
a) Runtime Error
b) Compile time Error
c)
System.ArgumentNullException: Value cannot be null. Parameter name: value at Program.Main .....
d) The program compiles successfully, and nothing is printed.
Q-33. What will be the output of the following code snippet:
using System; class MyProgram { static void Main() { try { Y(); } catch (Exception ex) { Console.WriteLine(ex.TargetSite); } } static void Y() { try { int.Parse("?"); } catch (Exception ex) { throw ex; // [Throw captured ex variable] } } }
a) Runtime Error
b) Compile time Error
c) The program compiles successfully, and nothing is printed.
d) Int32 Parse(System.String)
Q-34. What will be the output of the following code snippet:
using System; class Program { static void Main() { try { X(); } catch (Exception ex) { Console.WriteLine(ex.TargetSite); } } static void X() { try { int.Parse("-9"); } catch (Exception) { throw; // [Rethrow construct] } } }
a) Runtime Error
b) Compile time Error
c) The program compiles successfully, and nothing is printed.
d)
void StringToNumber(System.String, System.Globalization.NumberStyles, NumberBuffer ByRef, System.Globalization.NumberFormatInfo, Boolean)
Q-35. What will be the output of the following code snippet:
using System; public class MyCustomException : System.ApplicationException { public MyCustomException( string message,Exception inner): base(message,inner) { } } public class Test { public static void Main( ) { Test t = new Test( ); t.TestFunc( ); } public void TestFunc( ) { try { ExceptionFunc1( ); } // if you catch a custom exception // print the exception history catch (MyCustomException e) { Console.WriteLine("\n{0}", e.Message); Console.WriteLine( "Retrieving exception history..."); Exception inner = e.InnerException; while (inner != null) { Console.WriteLine( "{0}",inner.Message); inner = inner.InnerException; } } } public void ExceptionFunc1( ) { try { ExceptionFunc2( ); } // if you catch any exception here // throw a custom exception catch(System.Exception e) { MyCustomException ex = new MyCustomException( "E3 - Custom Exception Situation!",e); throw ex; } } public void ExceptionFunc2( ) { try { ExceptionFunc3( ); } // if you catch a DivideByZeroException take some // corrective action and then throw a general exception catch (System.DivideByZeroException e) { Exception ex = new Exception( "E2 - Func2 caught divide by zero",e); throw ex; } } public void ExceptionFunc3( ) { try { ExceptionFunc4( ); } catch (System.ArithmeticException) { throw; } catch (System.Exception) { Console.WriteLine( "Exception handled here."); } } public void ExceptionFunc4( ) { throw new DivideByZeroException("E1 - DivideByZero Exception"); } }
a) Runtime Error
b) Compile time Error
c) The program compiles successfully, and nothing is printed.
d)
E3 - Custom Exception Situation! Retrieving exception history... E2 - Func2 caught divide by zero E1 - DivideByZero Exception
Summary – C# Exception Handling Interview Questions
Writing error-free code could be a challenge for many. However, with a proper exception-handling approach, you can still secure a good user experience.
We hope that our efforts above with a brief review of C# exception handling concepts and a set of C# interview questions would accelerate your endeavor to become a successful C# programmer.
Check out more CSharp Q&A on various programming topics:
Best,
TechBeamers