Check out 20 C# OOPS interview questions and answers for experienced. Answer each question and identify any gaps before appearing in an interview. Before you begin to read the interview questions, let’s quickly wrap up the concepts of classes and objects in C#.
The primary building blocks of object-oriented programming are the class & the object. A class outlines the available characteristics and behavior of a set of identical objects which a programmer creates in his code. Whereas an object is the abstract form of a class that turns concrete at run-time and takes on the class’s behavior.
Test Your C# OOPS Skills
Classes, Accessibility, and Modifiers in C#
A class declaration encompasses a header and body.
The class header carries attributes, modifiers, and the class keyword.
Whereas its body encapsulates the members of the class, which could be data and functions. Look at the syntax of a class declaration in C#.
Attributes accessibility modifiers class identifier: baselist { body }
Attributes extend the context to a class like adjectives, e.g. the Serializable attribute. Accessibility represents the visibility of the class. By default, a class has internal accessibility. Whereas its members are private by default. The following is the list of accessibility keywords in C#.
- Public – Visible in the current and referencing assembly.
- Private – visible inside the current class.
- Protected – Visible inside the current as well as in the derived class.
- Internal – Visible inside the containing assembly.
- Internal protected – Visible inside the containing assembly, also in the descendant of the current class.
Next, are the modifiers that refine the declaration of a class. Here is the list of all supported modifiers in C#.
- Sealed – The class stops inheriting any derived class.
- Static – The class contains only static members.
- Unsafe – The class that stores unsafe types like pointers.
- Abstract – No instance of the class if the Class is abstract.
In the below section, find out 20 C# OOPS interview questions for experienced.
20 C# OOPS interview questions and answers
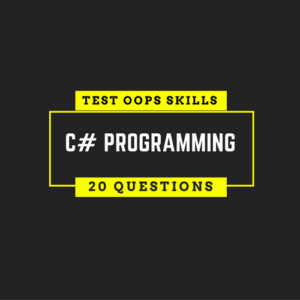
You can find the answer key at the end of this post.
Q-1. What will be the output of the following code snippet?
using System; public class emp { public static int age = 40; public static int salary = 25000; } public class record :emp { new public static int salary = 50000; static void Main(string[] args) { Console.WriteLine(emp.age + " " + emp.salary + " " + salary); } }
a) 40, 25000, 50000
b) 40, 25000, 25000
c) 40, 50000, 50000
d) Error
Q-2. Which of the following keyword, enables to modify the data and behavior of a base class by replacing its member with a new derived member?
a) overloads
b) overrides
c) new
d) base
Q-3. Which of the following is the correct way to overload “+” operator?
a) public sample operator + ( sample a, sample b)
b) public abstract operator + (sample a, sample b)
c) public static sample operator + (sample a, sample b)
d) All of the above
Q-4. What will be the correct order of execution of function func1(), func2() & func3() in the given code snippet?
class baseclass { public void func1() {} public virtual void func2() {} public virtual void func3() {} } class derivedclass :baseclass { new public void func1() {} public override void func2() {} public new void func3() {} } class Program { static void Main(string[] args) { baseclass b = new derivedclass(); b.func1 (); b.func2 (); b.func3 (); } }
a) func1() of derived class get executed
func2() of derived class get executed
func3() of base class get executed
b) func1() of base class get executed
func2() of derived class get executed
func3() of derived class get executed
c) func1() of derived class get executed
func2() of base class get executed
func3() of base class get executed
d) func1() of base class get executed
func2() of derived class get executed
func3() of base class get executed
Q-5. Which of the following statements are correct for C# language?
a) Every derived class does not define its own version of the virtual method.
b) By default, the access mode for all methods in C# is virtual.
c) If a derived class, does not define its own version of the virtual method, then the one present in the base class gets used.
d) All of the above.
Q-6. Which of the following code needs to be added for the overloaded operator “-” in C#?
class example { int x, y, z; public example() { } public example(int a ,int b ,int c) { x = a; y = b; z = c; } //Add correct set of code here public void display() { Console.WriteLine(x + " " + y + " " + z); } class program { static void Main(String[] args) { example obj1 = new example(5 ,6 ,8); example obj2 = new example(); obj2 = - obj1; obj2.display(); } } }
a)
public static example operator -(example obj1) { example t = new example(); t.x = obj1.x; t.y = obj1.y; t.z = -obj1.z; return t; }
b)
public static example operator -(example obj1) { example t = new example(); t.x = obj1.x; t.y = obj1.y; t.z = obj1.z; return t; }
c)
public static example operator -(example obj1) { example t = new example(); t.x = -obj1.x; t.y = -obj1.y; t.z = -obj1.z; return t; }
d) None of the above
Q-7. Which of the following is the correct name for selecting an appropriate method from a number of overloaded methods, by matching the arguments in terms of their number, type, order, and binding at compile time?
a) Late binding
b) Static binding.
c) Static Linking.
d) Compile time polymorphism.
Q-8. Which of the following statements is correct for run-time polymorphism?
a) The overridden base method should be virtual, abstract, or override.
b) An abstract method is implicitly a virtual method.
c) An abstract inherited property cannot be overridden in a derived class.
d) Both the override method and virtual method must have the same access level modifier.
Q-9. What will be the output of the following code snippet?
using System; class sample { public sample() { Console.WriteLine("constructor 1 called"); } public sample(int x) { int p = 2; int u; u = p + x; Console.WriteLine("constructor 2 called"); } } class Program { static void Main(string[] args) { sample s = new sample(4); sample t = new sample(); Console.ReadLine(); } }
a) constructor 1 called
constructor 2 called
b) constructor 2 called
constructor 1 called
c) constructor 2 called
constructor 2 called
d) error
Q-10. Which of the following keywords is used to refer base class constructor to subclass constructor?
a) this
b) static
c) base
d) extend
Q-11. What will be the output of the following code snippet?
using System; class sample { int i; public sample(int x) { i = x; Console.WriteLine("Tech"); } } class derived : sample { public derived(int x) :base(x) { Console.WriteLine("Beamers"); } } class Program { static void Main(string[] args) { derived k = new derived(12); Console.ReadLine(); } }
a) TechBeamers
b) 12 Tech
c) Beamers 12
d) Compile time error
Q-12. What will be the output of the following code snippet?
using System; class sample { int i; public sample(int num) { num = 12; int j = 12; int val = num * j; Console.WriteLine(val); } } class sample1 : sample { public sample1(int a) :base(a) { a = 13; int b = 13; Console.WriteLine(a + b); } } class sample2 : sample1 { public sample2(int k) :base(k) { k = 24; int o = 6 ; Console.WriteLine(k /o); } } class Program { public static void Main(string[] args) { sample2 t = new sample2(10); Console.ReadLine(); } }
a) 4, 26, 144
b) 26, 4, 144
c) 144, 26, 4
d) 0, 0, 0
Q-13. What will be the output of the following code snippet?
using System; class sample { static sample() { int num = 8; Console.WriteLine(num); } public sample(int a) { int val = 10; Console.WriteLine(val); } } class Program { static void Main(string[] args) { sample s = new sample(100); Console.ReadLine(); } }
a) 10, 10
b) 0, 10
c) 8, 10
d) 8, 8
Q-14. What will be the output of the following code snippet?
using System; class sample { int i; public sample(int num) { num = -25; int val; val = num > 0 ? num : num * -1; Console.WriteLine(val); } } class sample1 :sample { public sample1(int no) :base(no) { no = -1000; Console.WriteLine((no > 0 ? no : no * -1)); } } class Program { static void Main(string[] args) { sample1 s = new sample1(6); Console.ReadLine(); } }
a) 25
1000
b) -25
-1000
c) -25
1000
d) 25
-1000
Q-15. Which of the following options represents the type of class which does not have its own objects but acts as a base class for its subclass?
a) Static class
b) Sealed class
c) Abstract class
d) Derived class
Q-16. What will be the output of the following code snippet?
using System; namespace TechBeamers { abstract class baseclass { public int i ; public int j ; public abstract void print(); } class derived: baseclass { public int j = 5; public override void print() { this.j = 3; Console.WriteLine(i + " " + j); } } class Program { static void Main(string[] args) { derived obj = new derived(); obj.i = 1; obj.print(); Console.ReadLine(); } } }
a) 1, 5
b) 0, 5
c) 1, 0
d) 1, 3
Q-17. What will be the output of the following code snippet?
using System; namespace TechBeamers { abstract class baseclass { public int i; public abstract void print(); } class derived: baseclass { public int j; public int val; public override void print() { val = i + j; Console.WriteLine(+i + "\n" + +j); Console.WriteLine("sum is:" +val); } } class Program { static void Main(string[] args) { baseclass obj = new derived(); obj.i = 2; derived obj1 = new derived(); obj1.j = 10; obj.print(); Console.ReadLine(); } } }
a) 2 10
Sum is: 12
b) 0 10
Sum is: 10
c) 2 0
Sum is: 2
d) 0 0
Sum is: 0
Q-18. Which of the following represents a class that inherits an abstract class but it does not define all of its functions?
a) Abstract
b) A simple class
c) Static class
d) derived class
Q-19. What will be the output of the following code snippet?
using System; namespace TechBeamers { public abstract class sample { public int i = 7; public abstract void print(); } class sample1: sample { public int j; public override void print() { Console.WriteLine(i); Console.WriteLine(j); } } class Program { static void Main(string[] args) { sample1 obj = new sample1(); sample obj1 = new sample1(); obj.j = 1; obj1.i = 8; obj.print(); Console.ReadLine(); } } }
a) 0, 8
b) 1, 8
c) 1, 7
d) 7, 1
Q-20. What will be the output of the following code snippet?
using System; namespace TechBeamers { class sample { public int i; public void print() { Console.WriteLine(i); } } class sample1: sample { public int j; public void print() { Console.WriteLine(j); } } class Program { static void Main(string[] args) { sample1 obj = new sample1(); obj.j = 1; obj.i = 8; obj.print(); Console.ReadLine(); } } }
a) 8, 1
b) 8
c) 1
d) 1, 8
C# OOPS Interview Questions Answer Key
1. a 2. c 3. d 4. d 5. b 6. c 7. b, c, d 8. a, b, d 9. b 10. c | 11. a 12. c 13. c 14. a 15. c 16. d 17. c 18. a 19. d 20. c |
Summary – 20 C# OOPS Interview Questions for Experienced
In this post, we covered twenty C# OOPS interview questions and targeted a broad range of C# programming concepts.
We hope you have enjoyed going through the list of questions.
Check out more CSharp Q&A on various programming topics:
1. 50 CSharp Coding Interview Questions
2. 15 CSharp Programming Interview Questions
3. 15 CSharp Interview Questions Every Programmer
4. 15 CSharp Questions on For, While, and If Else
5. 15 CSharp Programming Questions on Classes
6. 20 CSharp OOPS Interview Questions Set-1
7. 20 CSharp OOPS Interview Questions Set-2
8. 25 CSharp OOPs Interview Questions Set-3
9. 35 CSharp Exception Handling Questions
Thanks for reading!
TechBeamers