Presenting today is the step-by-step tutorial for creating a Selenium 3 project to run UI automation tests in Firefox using Geckodriver. Let’s begin the interactive tutorial with a brief overview of Selenium 3 and the Geckodriver.
Selenium is the most favored UI automation tool for QA engineers. And it is continuously evolving since its inception in 2004. So far, Selenium 2 which introduced the WebDriver interface was the most famous version as it added native browser automation support.
Now, we have Selenium 3 recently launched with a list of new features. And, in this post, we’ll cover how to create a Selenium 3 project for Firefox using Geckodriver in Java.
Introduction to GeckoDriver
In Selenium 3, one of the major changes is that you can no longer access Firefox directly from the code. Instead, like the Chrome driver, now there is the new Gecko driver which you need to use for Firefox. So, it’s important to learn about the Geckodriver.
Please note if you are preparing for a Selenium testing interview, you must go through the following 35 Selenium interview questions at least once.
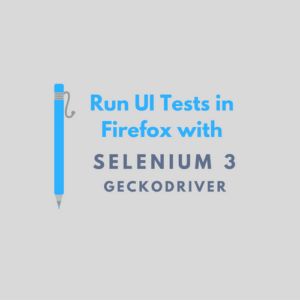
What is GeckoDriver and how does it work?
It’s a composite term that combines Gecko and Driver. Gecko is a proprietary web browser engine designed and developed by Mozilla.
GeckoDriver is the component that facilitates Selenium 3 tests to run in the Firefox browser. So basically, it replaced the default FF driver implementation in Selenium 3. It aimed to avoid compatibility issues that you might have seen with Selenium 2 and new versions of Firefox. Now Mozilla will also have to update the Geckodriver with every new release of its browser.
GeckoDriver in its physical form is an executable program that starts a server to accept commands from Selenium 3 tests. It uses the Marionette automation protocol to communicate with the browser by acting as a proxy. Hence, we may call it Marionette driver as well.
Note – There are several changes made to the Selenium 3 which you should check. Refer to Selenium 3 Changes.
How to move from Selenium 2.0 to Selenium 3.0?
First of all, you would need to download the latest version of the Selenium 3.0 package. And then, you will have to replace the Selenium 2.0 references with 3.0 libs under your project in Eclipse.
But there are some more steps you need to do. Because if you try to run the application, then it will give java.lang.IllegalStateException
. The description of the error is as follows.
The path to the driver executable must be set by webdriver.gecko.driver
system property.
java.lang.IllegalStateException: The path to the driver executable must be set by the webdriver.gecko.driver system property; at com.google.common.base.Preconditions.checkState(Preconditions.java:199) at org.openqa.selenium.remote.service.DriverService.findExecutable(DriverService.java:109) at org.openqa.selenium.firefox.GeckoDriverService.access$100(GeckoDriverService.java:38) ...
So, for a successful migration, first of all, you should download the latest version of the Geckodriver.
Click here to download the latest Geckodriver.
Next, you need to provide the Geckodriver path to the application. For this, you can adapt any of the following three approaches.
- Use system property to specify the Geckodriver path
- Add a persistent environment variable to set the Geckodriver path
- Add Geckodriver path via the browser’s desired capabilities
1. Use system property to specify the Geckodriver path.
The new Geckodriver for Selenium 3 accepts the following system property to set from the Java code.
System.setProperty("webdriver.gecko.driver", "Geckodriver Executable Path");
Below is the full code to launch the Firefox using Geckodriver.
package seleniumPrograms; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; public class Selenium3_test { public static void main(String[] args) throws InterruptedException { System.setProperty("webdriver.gecko.driver", "C:\\Selenium3\\work\\tools\\geckodriver.exe"); WebDriver driver = new FirefoxDriver(); driver.get("http://www.google.com"); Thread.sleep(5000); driver.quit(); } }
You can execute the above program in Eclipse and check if it is working all right. It’ll start Firefox and open the Google website.
2. Add a persistent environment variable to set the Geckodriver path.
Another simple method that one can use is by appending the path of Geckodriver in the PATH environment variable.
You can quickly do it from the command line using the SETX command in Windows 7.
setx PATH "C:\Selenium3\work\tools\geckodriver.exe;%PATH%;"
The above command will update the PATH in the global context. The significance of this method is that you won’t need to set the system property in each Selenium test.
Your code will work without setting up the "webdriver.gecko.driver"
Property.
3. Add the Geckodriver path via the browser’s desired capabilities.
Alternatively, we can make use of the Marionette capability parameter to run our Selenium3 tests. It is very easy to set it from the Java code.
Please check out the below Java code. With this method, it is also possible to run your tests remotely.
package seleniumPrograms; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.remote.DesiredCapabilities; public class Selenium3_test { public static void main(String[] args) throws InterruptedException { DesiredCapabilities dc = DesiredCapabilities.firefox(); dc.setCapability("marionette", true); WebDriver driver = new FirefoxDriver(dc); driver.get("http://www.google.com"); Thread.sleep(5000); driver.quit(); } }
TestNG Example – Launch Firefox using Geckodriver.
If any of you would like to use Geckodriver with a TestNG project, then refer to the below code.
package com.local; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.annotations.Test; public class Selenium3_test { String geckoPath = "<Provide Gecko Driver Executable Path>"; public WebDriver driver; @Test public void initSession() { System.out.println("Starting Firefox browser"); System.setProperty("webdriver.gecko.driver", geckoPath + "geckodriver.exe"); driver = new FirefoxDriver(); } @Test public void openURL() { driver.navigate().to("http://www.google.com/"); } @Test public void endSession() { if(driver!=null) { driver.close(); } } }
Also, if you are using the Maven tool to build your project, then you’ll need to update the POM file in your project.
Please see the sample POM file given below.
<dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>3.0.1</version> </dependency>
Summary – Selenium Project for Firefox using Geckodriver
We used Selenium 3 for this tutorial. However, you can use the code with the latest version of Selenium. If you encounter issues, we’ll help you resolve them.
Lastly, support our site if you want us to continue sharing knowledge. Share this post on social media (Facebook/Twitter) if you gained some knowledge from this tutorial.
Keep Learning,
TechBeamers.