Software testers who are planning to get a testing or test automation job should read this post. We’ve compiled the best 35 Selenium Webdriver questions to help you succeed in a job interview.
You’ll find a short and concise answer for all the Selenium Webdriver questions as most of the interviewers expect from an ideal candidate. There has been a lot of effort involved in preparing the perfect set of interview questions.
We believe that this questionnaire will not only benefit in face-to-face interviews but also in telephonic ones as well. It has been quite popular these days as the IT companies want to make sure the technical skills of a candidate before they invite him for a one-to-one discussion.
In addition to this post, you should also check out the most-read post on this blog which exclusively covers 100+ Selenium interview questions for beginners and experienced. It’ll make sure that you have a sufficient understanding of the Selenium Webdriver concepts.
35 Selenium Webdriver Questions to Crack Interviews
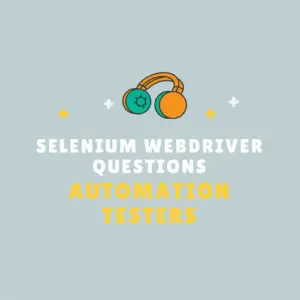
Let’s quickly go through the list of the top Selenium Webdriver questions specially designed for testing job seekers.
Core Concept Related Selenium Webdriver Questions.
Q: How many types of Webdriver APIs are available in Selenium?
Ans. Below is the list of driver classes that you can use for browser automation.
- AndroidDriver,
- ChromeDriver,
- EventFiringWebDriver,
- FirefoxDriver,
- HtmlUnitDriver,
- InternetExplorerDriver,
- IPhoneDriver,
- IPhoneSimulatorDriver,
- RemoteWebDriver.
Q: How would you make sure that a page is loaded using Selenium and Webdriver?
Ans. In Selenium, you can use the below lines of code to check for the successful loading of a web page. The best approach is to select an element from the page & stand by until it becomes clickable.
selenium.waitForPageToLoad("5000"); // Or while (!(selenium.isElementPresent("any page element ")==true)) { selenium.setSpeed("5"); Thread.sleep(5); }
Below is the Webdriver-specific code to achieve the same objective.
WebDriverWait check = new WebDriverWait(driver, 100); check.until(ExpectedConditions.anyElement(By.id(id)));
Q: How to launch a batch file in a Selenium Webdriver project?
Ans. It’s usually in a test suite to run a batch file or an executable file for setting up the prerequisites before starting the automation. You can use the below Java code for this purpose.
Process batch = Runtime.getRuntime.exec("path of the batch file"); batch.waitFor();
Q: How do you read a JavaScript variable in Selenium WebDriver?
Ans. It’s easy to access any JavaScript variable from the Selenium Webdriver test scripts. Just you need to use the below Java code snippet.
// Initialize the JS object. JavascriptExecutor JS = (JavascriptExecutor) webdriver; // Get the current site title. String sitetitle = (String)JS.executeScript("return document.title"); System.out.println("My Site Title: " + sitetitle);
Q: How to run the Selenium IDE test suite from the command line?
Ans. We’ve given the command to run the SIDE test suite in the next line.
Java -jar "C:\Selenium Webdriver Questions\selenium-server-standalone-2.33.0.jar" -htmlSuite "*firefox" "http://www.google.com" " “C:\Selenium Webdriver Questions\SeleniumSuite.HTML"
Q: How to run the Selenium Webdriver test from the command line?
Ans. We can run any Selenium Webdriver test written in Java using the following command.
java -classpath ".;selenium-server-standalone-2.33.0.jar" SampleClass
Q: What are the different exceptions you face in Selenium Webdriver?
Ans.
- WebDriverException,
- NoAlertPresentException,
- NoSuchWindowException,
- NoSuchElementException,
- TimeoutException.
Q: How would you automatically click a screenshot whenever an exception occurs?
Ans. For this, you will have to use the <EventFiringWebDriver> class and needs to implement the <onException> method of the <WebDriverEventListener> interface. See the code example given below.
WebDriver browser = new FirefoxDriver(); EventFiringWebDriver eventDriver = new EventFiringWebDriver(browser).register(new AbstractWebDriverEventListener() { @Override public void onException(Throwable throwable, WebDriver browser) { // Take the screenshot using the Webdriver. File screen = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); // Now you can copy the screenshot somewhere on your system. FileUtils.copyFile(screen, new File("c:\\Selenium Testing Questions\\screen.png")); } }); try { eventDriver.findElement(By.id("test")); fail("Caught the Expected exception."); // Intentionally causing the exception for demo. } catch (NoSuchElementException e) { // Triggering point for the <onException> event. }
Q: How would you select any particular text using the Selenium Webdriver?
Ans. It seems an easy one at first but you need to do a little more to achieve this.
driver.get("/"); WebElement item = driver.findElement(By.xpath("//p[contains(text(),'Selenium webdriver quesions')]")); Actions dummy = new Actions(driver); dummy.doubleClick(item).build().perform();
Q: Give an example to perform drag and drop action In Selenium WebDriver.
Ans. Yes, we can use the Advanced User Interactions API to perform drag-and-drop operations in a Selenium Webdriver project.
Code example:
Actions act = new Actions(driver); act.dragAndDrop(source_locator, target_locator).build().perform(); //Or you can use the below code style. (new Actions(driver)).dragAndDrop(source_locator, target_locator).perform();
Q: How would you fill a text field without calling the sendKeys()?
Ans. It’s a bit slower than the sendKeys() method but we do have means to type in a text field. See the Java code given below.
JavascriptExecutor JS = (JavascriptExecutor)webdriver; JS.executeScript("document.getElementById(User').value='admin@testmail.com'"); JS.executeScript("document.getElementById('Pass').value='######'");
Q: How can you check the state of a checkbox/radio button?
Ans. We can call the isSelected() method to test the status of these elements.
Example Code:
boolean test = driver.findElement(By.xpath("checkbox/radio button XPath")).isSelected();
Q: How would you handle the alert popups in Selenium Webdriver?
Ans. First, you’ve to switch the control to the pop up then press the ok or cancel button. After that, turn back to the source page screen.
Code Example:
String srcPage = driver.getWindowHandle(); Alert pop = driver.switchTo().alert(); // shift control to the alert popup. pop.accept(); // click on ok button. pop.dismiss(); // click on cancel button. // Move the control back to source page. driver.switchTo().window(srcPage); // move back to the source page.
Q: What is the process to start the IE/Chrome browser?
Ans. If you want to start a browser then, just set the system properties as mentioned below.
// For the IE web browser. System.setProperty("webdriver.ie.driver"," iedriver.exe file path"); WebDriver driver = new InternetExplorerDriver(); // For the Chrome web browser. System.setProperty("webdriver.chrome.driver","chrome.exe file path"); WebDriver driver = new ChromeDriver();
Q: How would you simulate the right-click operation in WebDriver?
Ans. You can make use of the Actions class features.
Actions test = new Actions(driver); // Here, driver is the object of WebDriver class. test.moveToElement(element).perform(); test.contextClick().perform();
Q: How would you select a menu item from a drop-down menu?
Ans. There can be the following two situations.
- If the menu is using the <select> tag then you can call the <selectByValue()> or <selectByIndex()> or <selectByVisibleText()> methods of the Select class.
- If the menu doesn’t use the <select> tag then simply find the XPath of that element and perform the click action for its selection.
Q: What is the FirefoxDriver, class, or interface? And which interface does it implement?
Ans. FirefoxDriver is a Java class, and it implements the <WebDriver> interface. It contains the implementations of all the methods available in the <WebDriver> interface.
Q: What is the name of the super interface of the Webdriver?
Ans. SearchContext.
Q: What is the main difference between the close() and quit() methods?
Ans.
close() – it closes the currently active browser window.
quit()- it will close all of the opened browser windows and the browser itself.
Q: What is the best way to check for the highlighted text on a web page?
Ans. Use the below code to verify the highlighted text for an element on the web page.
String clr = driver.findElement(By.xpath("//a[text()='TechBeamers']")).getCssValue("color"); String bkclr = driver.findElement(By.xpath("//a[text()='TechBeamers']")).getCssValue("background-color"); System.out.println(clr); System.out.println(bkclr);
Q: How would you use a Selenium variable say “size” from the JavaScript?
Ans. ${size}
Q: What is the Selenese command to show the value of a variable in the log file?
Ans. echo()
CSS/XPath Related Selenium Webdriver Questions.
Q: How would you differentiate between the absolute and relative XPath?
Ans.
A single slash “/” signifies an absolute XPath.
Double Slash “//” represents the relative XPath.
Q: What are the different ways to find the element by CSS class?
Ans. Say, the name of the CSS class is <MyTest>. Then, the most simple way to find the element is as follows.
//*[contains(@class, ‘MyTest')]
If you want an exact comparison to avoid matching texts like “OhhMyTest” or “MyTesting” then use this.
//*[contains(concat(' ', @class, ' '), ' MyTest ')]
You can even use the <normalize-space> function to remove any leading or trailing whitespaces.
//*[contains(concat(' ', normalize-space(@class), ' '), 'MyTest ')]
Q: How to specify a child element using XPath?
Ans.
//div/a
Q: How to specify a child element using CSS?
Ans.
css=div > a
Q: How to identify a sub-child element using XPath?
Ans.
//div//a
Q: How to identify a sub-child element using CSS?
Ans.
css=div a
Q: How do you access an element ID using CSS?
Ans.
css=div#sample a
Q: How would you get to the next sibling?
Ans. You may try this code.
tr/td[@class='user’]/following-sibling::td
Or you can use the below code.
tr[td[@class=’user'] ='age']/td[@class='weight']
TestNG Selenium Webdriver Questions.
Q: What is the time unit you use in TestNG time tests?
Ans. We use milliseconds as the time unit in @Test or @TestSuite methods.
Q: What is the use of priority in @Test methods and how do you set it?
Ans. We define the priority in @Test annotated methods to set the order of test execution. Please check the below example.
@Test1(priority=0) @Test2(priority=2) @Test3(priority=1)
The above test will run in the following order.
Test1 => Test3 => Test2
Q: What are the two different ways of generating reports in TestNG?
Ans. There are two ways to produce a report with Test NG, they are
- Use of Listeners: You can implement the
org.testng.ITestListener
interface and its methods. Reporting will start when the test begins, finishes, skips, and passes/fails test cases. - Use of Reporters: Another way to enable reporting is by implementing the
org.testng.Reporter
interface. Unlike the listener interface, here the reporting begins after the whole test suite reaches the end. The reporting class receives the object which contains the information of the whole test run.
Q: How to execute the TestNG test suite from the command line?
Ans. We don’t always need to open Eclipse to run the TestNG project, try using the below command instead.
java -cp “C:\Selenium Webdriver Questions\testng \lib\*;C:\Selenium Testing Questions\testng\bin” org.testng.TestNG testng.xml
Q: How to turn off the test output (generated test output folder) in TestNG?
Ans. In Eclipse, modify the launch/Run configuration and add an -usedefaultlisteners false
option to disable the test output.
Let’s now summarize the above post which includes the top 35 Selenium Webdriver questions and do a quick review of the content.
Final Word – 35 Selenium Webdriver Questions
We’ve tried to bring the most pertinent Selenium Webdriver questions in this post, but this topic belongs to an evolving subject. Hence, there would always be a scope for improvements and enhancements.
We’ll keep supporting all the new changes in the Selenium Webdriver technology and will add more and more questions that could add to your skills and boost your confidence when you face any job interview.
Apart from interview questions, we’ve also covered a wide range of Selenium quizzes on our blog. Attempt these and refresh your concepts.
Next, we like you to contribute to our knowledge drive. You can do this by sharing this list of top Selenium Webdriver questions among your friends and on social media using the sharing icons shown below.
Keep Learning,
TechBeamers