Welcome to our comprehensive guide on how to reverse a list in Python! In this tutorial, we will walk you through step-by-step instructions, along with clear examples, to help you understand and implement efficient methods for reversing lists in Python.
Whether you’re a beginner or an experienced Python developer, this guide will equip you with the knowledge you need to confidently manipulate and reverse lists in your Python programs. Let’s dive in and unravel the secrets of reversing lists in Python!
How to Reverse a List in Python?
In Python, reversing a list is a common operation that can be easily accomplished using various methods. In this tutorial, we will explore different techniques to reverse a list in Python. We will cover both the built-in functions and manual approaches, providing you with a comprehensive understanding of the process.
Reversing a list or sequence in Python can be useful in various scenarios and can offer several benefits in real-time programming or projects.
Use cases for Reversing a List
Here are a few use cases where reversing a list can be helpful:
Changing Order: Reversing a list allows you to change the order of elements.
Iterating in Reverse: Reversing a list enables you to iterate over its elements in reverse order.
User Interface Display: Reversing a list can be used to display information in a visually appealing way, such as showing the most recent items first.
Algorithm Optimization: Some algorithms or computations can be more efficient when performed in reverse order.
Problem-Solving: Reversing a list can be useful in solving programming problems, finding patterns, or implementing specific requirements.
Let’s now explore the six unique ways to reverse a list in Python and understand through examples.
1. List.Reverse() Method
The reverse() method in Python is a built-in method that helps you to reverse the order of elements in a list. When applied to a list, it modifies the list in place, without creating a new list.
Its syntax is as follows:
List_name.reverse()
Parameters: This method does not take any parameters.
Return Value: It does not return anything. Instead, it modifies the list directly.
Usage: To reverse a list, you simply call the reverse() method on the list object. The elements in the list will be reversed, changing the original list itself.
How does the Python list reverse() function work?
This method doesn’t accept any argument and also has no return value. It only creates an updated list which is the reverse of the original sequence.
Please do not confuse the reverse() method with reversed(). The latter allows accessing the list in the reverse order whereas the reverse function merely transposes the order of the elements. The flowchart below attempts to explain it in a diagram:
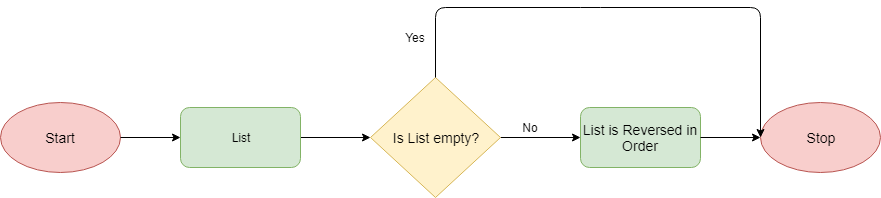
List.Reverse() Examples
Below are some examples to let you understand the usage of the reverse method. You can read our Python list tutorial to learn about its various other features.
a) Revere a list of numbers in Python
# Initilize the list of numbers Cubes = [1,8,27,64,125] Cubes.reverse() print (Cubes) # Output: [125, 64, 27, 8, 1]
b) A list of strings to reverse in Python
# Initialize the list of strings Mathematics = ["Number Theory", "Game Theory", "Statistics", "Set Theory"] Mathematics.reverse() print (Mathematics) # Output: ['Set Theory', 'Statistics', 'Game Theory', 'Number Theory']
c) Toggle the order in a list of boolean values
One unique but lesser-known usage of the list.reverse() method in Python is for toggling the order of boolean values stored in a list. By reversing the list, all True values become False, and all False values become True. This technique can come in handy in cases where you have a list representing the state of certain conditions or flags, and you want to toggle their values.
conditions = [True, False, True, True, False] conditions.reverse() print(conditions) # Output: [False, True, True, False, True]
2. Python Reversed() Function
It is a Python built-in function that doesn’t actually reverse a list. Instead, it provides an iterator for traversing the list in the reverse order. Please note the following points about this function.
- It works over a sequence, for example – A list, String, Tuple, etc.
- Its return value is an object of type “list_reverseiterator” – a reverse iterator.
Python reversed() function takes a single argument which can be a sequence or a custom object.
It has the following syntax in Python:
# Function # Call it directly on a sequence or list reversed(seq or custom object)
The reversed() function returns an iterator pointing to the last element in the list. We can use it to iterate the items in reverse order.
>>> type(reversed([])) <class 'list_reverseiterator'>
Python Reversed() List Examples
Here, we’ve got several practical examples of the Reversed() function operating on strings, lists, bytes, and tuples. Let’s dig each of them one by one.
""" Example: Demonstrate the application of Python Reversed() on String, List, Tuple, and Bytes """ def show(orig): print() print("Original: ", orig) print("Reversed: ", end="") for it in reversed(orig): print(it, end=' ') # Prepare Test Data str = "Machine Learning" list = ["Python", "Data Analysis", "Deep Learning", "Data Science"] tuple = ("Python", "CSharp", "Java") bytes = bytes("Python", "UTF-8") array = bytearray("Python", "UTF-8") # Run Tests show(str) show(list) show(tuple) show(bytes) show(array) # Output """ Original: Machine Learning Reversed: g n i n r a e L e n i h c a M Original: ['Python', 'Data Analysis', 'Deep Learning', 'Data Science'] Reversed: Data Science Deep Learning Data Analysis Python Original: ('Python', 'CSharp', 'Java') Reversed: Java CSharp Python Original: b'Python' Reversed: 110 111 104 116 121 80 Original: bytearray(b'Python') Reversed: 110 111 104 116 121 80 """
Here’s a more in-depth example that demonstrates the usage of the reversed() method to reverse a list.
Challenge: Given a list of numbers, reverse the list and compute the sum of the reversed elements.
def reverse_and_sum(numbers): reversed_numbers = list(reversed(numbers)) total = sum(reversed_numbers) return total # Test the function num_list = [1, 2, 3, 4, 5] result = reverse_and_sum(num_list) print(result) # Output: 15
In this example, we define a function called reverse_and_sum that takes a list of numbers as input. Within the function, we use the reversed() method to obtain a reversed iterator over the input list numbers. We convert this iterator to a list using the list() function and store it in the reversed_numbers variable.
Next, we compute the sum of the reversed numbers using the sum() function, which calculates the sum of all elements in the reversed_numbers list.
Finally, we return the total sum from the function. We then test the function by providing a list [1, 2, 3, 4, 5] as input. The result is the sum of the reversed numbers, which is 15.
This example showcases how the reversed() method can be utilized to reverse a list and solve a programming challenge involving reversed elements.
3. Slicing Operator to Reverse List
The most effective but lesser-known method to reverse a list in Python is by using the [::-1] slicing technique. It offers a concise and efficient way to reverse a list without the need for any additional functions or libraries.
Simply use the slicing syntax reversed_list = original_list[::-1] and the reversed list will be generated. This method creates a new reversed list, leaving the original list intact. It is not only highly effective but also widely regarded as a Pythonic approach for list reversal. Check its usage from the below example.
""" Function: Desc: Reverse list using Python slice operator Param: Input list Return: New list """ def invertList(input_list): out_list = input_list[::-1] return out_list # Unit test 1 input_list = [11, 27, -1, -5, 4, 3] print("Input list[id={}] items: {}".format(id(input_list), input_list)) out_list = invertList(input_list) print("Output list[id={}] items: {}".format(id(out_list), out_list))
After you run the above code, it outputs the following in the console:
Input list[id=139774228206664] items: [11, 27, -1, -5, 4, 3] Output list[id=139774228208840] items: [3, 4, -5, -1, 27, 11]
The result shows that the slice operator created a new copy as the id values of both input and output lists are different.
4. Reversing List Using For Loop in Python
Reversing a list using a for loop in Python can be done by iterating over the list and appending the elements in reverse order to a new list. Here’s an example that demonstrates how to reverse a list using a for loop:
""" Function: Desc: Reverse list using for loop Param: Input list Return: Modified input list """ def invertList(input_list): for item in range(len(input_list)//2): input_list[item], input_list[len(input_list)-1-item] = input_list[len(input_list)-1-item], input_list[item] return input_list # Unit test 1 input_list = [11, 27, -1, -5, 4, 3] print("Input list[id={}] items: {}".format(id(input_list), input_list)) input_list = invertList(input_list) print("Output list[id={}] items: {}".format(id(input_list), input_list))
After you run the above code, it outputs the following in the console:
Input list[id=140703238378568] items: [11, 27, -1, -5, 4, 3] Output list[id=140703238378568] items: [3, 4, -5, -1, 27, 11]
By using a for loop, we can manually iterate over the list and build a new list in reverse order. This approach is straightforward and does not require any additional modules or functions.
5. Deque Method to Reverse List in Python
An effective but lesser-known method to reverse a list in Python is by utilizing the deque class from the collections module. By converting the list into a deque object and using the reverse() method, we can efficiently reverse the list.
To reverse a list using the deque method, you can follow these steps:
- Import the deque class from the collections module.
- Create a deque object by passing the list as an argument to the deque constructor.
- Apply the reverse() method to the deque object.
- Convert the reversed deque back to a list using the list() function.
Here’s an example that demonstrates how to reverse a list using the deque method:
from collections import deque my_list = [1, 2, 3, 4, 5] reversed_list = list(deque(my_list).reverse()) print(reversed_list) # Output: [5, 4, 3, 2, 1]
In this example, we start by importing the deque class from the collections module. We then create a deque object called my_deque by passing the my_list to the deque constructor. The deque object allows us to perform efficient operations at both ends of the queue.
Next, we apply the reverse() method directly on the my_deque object. This reverses the order of elements within the deque object. Finally, we convert the reversed deque object back to a list using the list() function and store it in the reversed_list variable.
The result is a reversed list, which is printed as [5, 4, 3, 2, 1].
6. Reverse List Using List Comprehension
Another customized approach is to use the list comprehension technique to reserve the list. See the below code to understand this method.
""" Function: Desc: Reverse list using list comprehension method Param: Input list Return: Modified list object """ def invertList(input_list): input_list = [input_list[n] for n in range(len(input_list)-1,-1,-1)] return input_list # Unit test 1 input_list = [11, 27, -1, -5, 4, 3] print("Input list[id={}] items: {}".format(id(input_list), input_list)) input_list = invertList(input_list) print("Output list[id={}] items: {}".format(id(input_list), input_list))
After you run the above code, it outputs the following in the console:
Input list[id=140044877149256] items: [11, 27, -1, -5, 4, 3] Output list[id=140044877151560] items: [3, 4, -5, -1, 27, 11]
7. Using the Itertools Module and zip() Function
The itertools
module in Python provides a set of functions for efficient looping and iteration. The zip() function, in combination with the function, itertools.islice()
can be used to reverse a list efficiently. Here’s a detailed explanation of using the itertools
module and the zip() function to reverse a list:
import itertools my_list = [1, 2, 3, 4, 5] reversed_list = list(zip(*itertools.islice([reversed(my_list)], len(my_list))))[0] print(reversed_list) # Output: [5, 4, 3, 2, 1]
In this example, we first import the itertools
module. Then, we define our original list my_list as [1, 2, 3, 4, 5].
To reverse the list, we use itertools.islice() to create a reversed slice of the list. The reversed() function is applied to my_list to obtain an iterator that yields the elements of my_list in reverse order. We pass this reversed iterator to itertools
.islice(), along with the length of my_list, to get a sliced iterator.
Next, we use the zip() function on the sliced iterator, effectively transposing the elements in reverse order. To access the reversed list, we convert the resulting iterator back to a list using list(). Finally, we extract the first element from the list using indexing ([0]) and store it in reversed_list.
The output is the reversed list [5, 4, 3, 2, 1], demonstrating the reversal of the original list using the itertools
module and the zip() function.
Recommended: Python Program to Reverse a Number
Summary – Reversing a List in Python
In conclusion, we have explored seven unique ways to reverse a list in Python, each with its own advantages and use cases. By understanding these different approaches, you can choose the most suitable method for your specific needs.
Choose the method that aligns best with your needs in terms of readability, memory usage, and performance. With this knowledge, you have gained a valuable skill in handling list reversal tasks in Python.
Cheers!