Python list slicing is a powerful and flexible technique for working with lists. Slicing allows you to create new lists by extracting portions of an existing list. In this tutorial, we will explore the basics of list slicing, step by step, with various examples, including both numbers and strings.
Understand How Slicing Works in Python
One of the main reasons for Python to have list slicing is to make it easier to work with lists.
Before list slicing, there were only a few ways to access elements of a list, such as using indexing or iteration. List slicing provides a more concise and efficient way to access and modify sublists.
Try Yourself: The Best 30 Questions on Python List, Tuple, and Dictionary
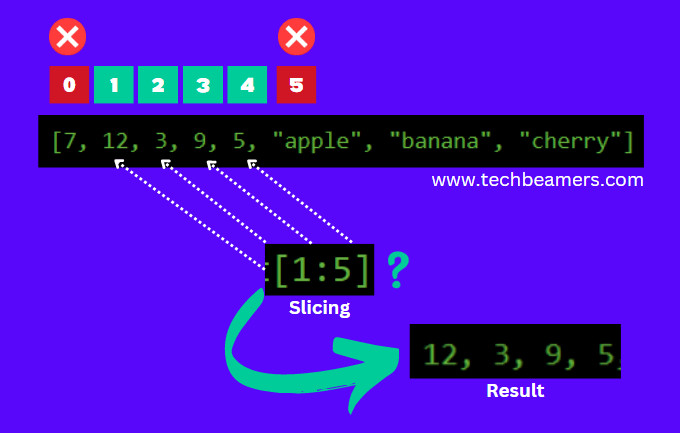
Sometimes, we might have to see if a Python list contains elements of another list or not. There are many such logical use cases we have to take care of as a programmer.
Introduction to Lists
Before we dive into list slicing, let’s quickly review what lists are. Lists are ordered collections of items in Python.
They can contain various data types, including integers, strings, or even other lists. Lists are defined using square brackets []
, with elements separated by commas.
my_list = [7, 12, 3, 9, 5, "apple", "banana", "cherry"]
Basic List Slicing
List slicing allows you to extract a subset of elements from a list. To perform basic list slicing in Python, you need the list and two indices: the start and end positions.
Also Read: Python List Sort Method
my_list = [7, 12, 3, 9, 5, "apple", "banana", "cherry"]
subset = my_list[1:5]
print(subset)
This code will print: [12, 3, 9, 5]
The start index is inclusive, and the end index is exclusive. So, the range [1:5]
includes elements at index 1, 2, 3, and 4, but not 5.
Python List Slicing Syntax
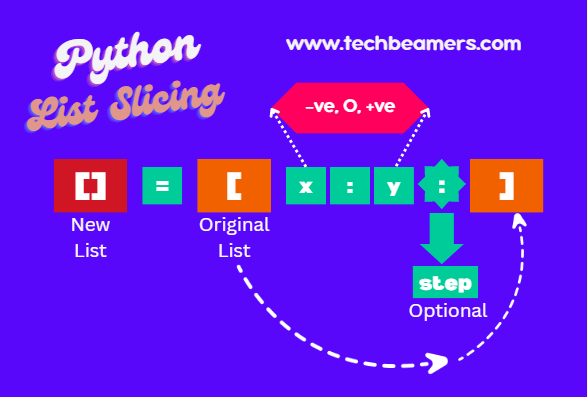
The syntax for list slicing is as follows:
new_list = original_list[start:end]
start
: The index of the first element you want to include.end
: The index of the first element you want to exclude.
Slicing with Negative Indices
You can also use negative indices for slicing. Firstly, there is no zero index in negative indices. Secondly, they count from the end of the list.
It means the last value is at -1, the second last is at -2, and so on. However, even with -ve indexing, the starting element (at -3 index) is inclusive whereas the last one is exclusive. This logic is similar to the basic slicing case. Here is the -ve Python list slicing example followed by its pictorial representation.
my_list = [7, 12, 3, 9, 5, "apple", "banana", "cherry"]
subset = my_list[-3:-1]
print(subset)
This code will print: ["apple", "banana"]
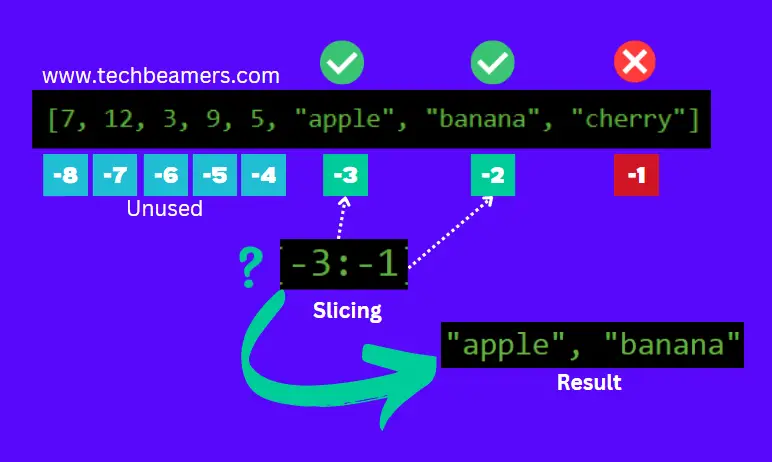
Slicing with Steps
You can also include an optional third parameter in Python list slicing, which defines the step size. This tells you how many elements to leave between each element.
my_list = [7, 12, 3, 9, 5, "apple", "banana", "cherry"]
subset = my_list[0:8:2]
print(subset)
This code will print: [7, 3, 5, "banana"]
In this example, the step size is 2, which means we’re skipping every second element from the list. Below is a picture depicting the list-slicing flow with steps.
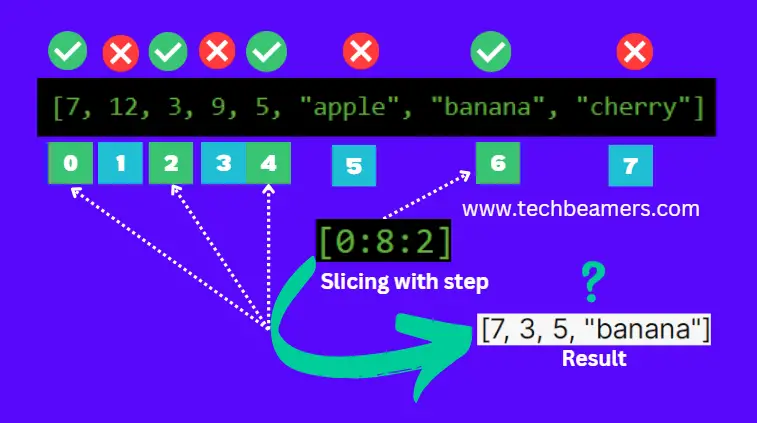
Use Slicing to Modify Lists
List slicing can also be used to modify the original list. For example, you can replace a portion of a list with new values.
my_list = [7, 12, 3, 9, 5, "apple", "banana", "cherry"]
my_list[1:5] = ["orange", "grape", "pear", "strawberry"]
print(my_list)
This code will print: [7, "orange", "grape", "pear", "strawberry", "apple", "banana", "cherry"]
Common List Slicing Tips
Here are some common list-slicing techniques with examples:
Sr. | Purpose | How To |
---|---|---|
1. | Copy a Python List | To create a copy of a Python list, you can use this: new_list = original_list[:] |
2. | Reverse a Python List | To reverse a list in Python, use this:reversed_list = original_list[::-1] . |
3. | Get the First N Elements | To get the first N elements, use this:subset = my_list[:3] . |
4. | Get the Last N Elements | To get the last N elements of a list, use this:subset = my_list[-2:] . |
5. | Remove Elements | To remove elements from a list, you can use slicing. |
6. | Splitting Lists | You can split a list into smaller lists using slicing. |
The 6 Best Python List Slicing Examples
List slicing is a core concept in Python and surfaces in various programming challenges. Here are the 6 best list-slicing problems along with their descriptions and sample Python code solutions.
Problem#1: Reversing a List
Description: Given a list, reverse it using list slicing.
Code:
lst = [1, 2, 3, 4, 5]
rev_lst = lst[::-1]
print(rev_lst)
Problem#2: Extracting a Sublist
Description: Given a list, extract a sublist that contains elements within a specified range.
Code:
lst = [10, 20, 30, 40, 50, 60]
sub = lst[2:5] # Extract elements at indices 2, 3, and 4
print(sub)
Problem#3: Removing Duplicates
Description: Given a list with duplicate elements, remove duplicates using list slicing.
Code:
lst = [1, 2, 2, 3, 4, 4, 5]
unique = list(set(lst)) # Using set to remove duplicates, then converting back to a list
print(unique)
Also Check: Python Program to Convert Lists into a Dictionary
Problem#4: Find Middle Element
Description: Given a list, find the middle element(s), or elements if the list has an even length.
Code:
lst = [1, 2, 3, 4, 5, 6]
mid = lst[len(lst) // 2] # For odd-length lists
print(mid)
mid_elements = lst[(len(lst) - 1) // 2:(len(lst) + 2) // 2] # For even-length lists
print(mid_elements)
Problem#5: Replace Element in Range
Description: Given a list, replace elements in a specified range with new values.
Code:
lst = [10, 20, 30, 40, 50]
lst[1:4] = [25, 35, 45] # Replace elements at indices 1, 2, and 3
print(lst)
Also Check: Find All Possible Permutation of a String in Python
Problem#6: Palindromic Substrings
Description: Given a string, find all palindromic substrings using list slicing.
Code:
def find_palindromic_subs(s):
subs = [s[i:j] for i in range(len(s)) for j in range(i + 1, len(s) + 1)]
pals = [sub for sub in subs if sub == sub[::-1]]
return pals
input_str = "abcbamadam"
pal_subs = find_palindromic_subs(input_str)
print(pal_subs)
These examples use shorter variable and function names while maintaining clarity and readability.
Before You Leave
That’s it! You’ve learned the basics of Python list slicing, including various examples with both numbers and strings. Slicing is a versatile tool for working with lists, and with practice, you’ll become proficient in using it for various tasks.
List slicing allows programmers to perform a variety of complex tasks on lists with just a few lines of code. This makes Python a very attractive language for data science and machine learning applications.
Lastly, our site needs your support to remain free. Share this post on social media (Linkedin/Twitter) if you gained some knowledge from this tutorial.
Happy coding,
TechBeamers.