From this tutorial, you will learn about the Python list Remove method. You will see how to use it on sequences with the help of examples.
Note: The syntax used in the below section is for Python 3. You can change it to any other version of Python.
Python List Remove
To Learn about Lists – Read Python List
List Remove Method
The Remove Module is a built-in list method that allows you to delete values in a list.
It deletes the first occurrence of a value in a sequence, i.e., it won’t erase all the instances that exist in the list.
The remove() method has the following syntax:
# Syntax - How to use the Python list remove() method List_name.remove(<element_value>)
It takes the element_value as an input argument. The function searches the list for the matching element_value and removes the first occurrence of the element_value from the list.
It doesn’t have a return value. It only removes the element from a list without returning a value.
How does Python List Remove() method work?
When we pass an input value to the remove(), the list gets iterated through each element until the matching one gets found.
This matching element gets removed from the list, and the indexes of all list items are also updated. If an invalid or non-existent element is provided as input, then the function raises a ValueError exception.
The flowchart below attempts to explain it in a diagram:
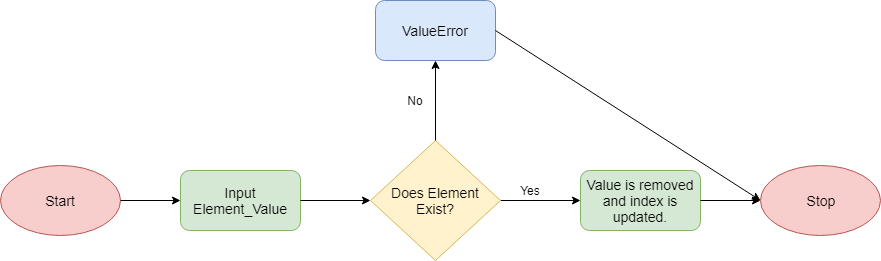
Program Examples
Removing an element from a list
List = [1,3,2,4,6] List.remove(3) print (List)
The result is as follows:
[1, 2, 4, 6]
Removing a tuple from the list
List = [1,2,(4,6),(25,4)] List.remove((4,6)) print (List)
The output is as follows:
[1, 2, (25, 4)]
Delete a string from the list
List = ["Unix", "PHP", "Golang"] List.remove("PHP") print (List)
The result is as follows:
['Unix', 'Golang']
Removing duplicate elements in a list
Social_Media = ["Whatsapp", "Hike", "Facebook", "Whatsapp", "Telegram"] Social_Media.remove("Whatsapp") print (Social_Media)
The result is as follows:
['Hike', 'Facebook', 'Whatsapp', 'Telegram']
Errors upon removing invalid items
List = [1,2, "Linux", "Java", 25, 4, 9] List.remove("PHP") print (List)
The result is as follows:
Traceback (most recent call last): File "C:\Python\Python35\test.py", line 3, in <module> List.remove("PHP") ValueError: list.remove(x): x not in list
Conclusion
In summary, the Python list remove() method in Python is an efficient way to remove specific elements from a list. It helps simplify code by removing unwanted items based on their values.
Finally, do keep in mind that it removes only the first occurrence of the given value. By learning and using this method, you can effectively modify lists in your Python programs.
Best,
TechBeamers